Using Pandas AI for Knowledge Evaluation
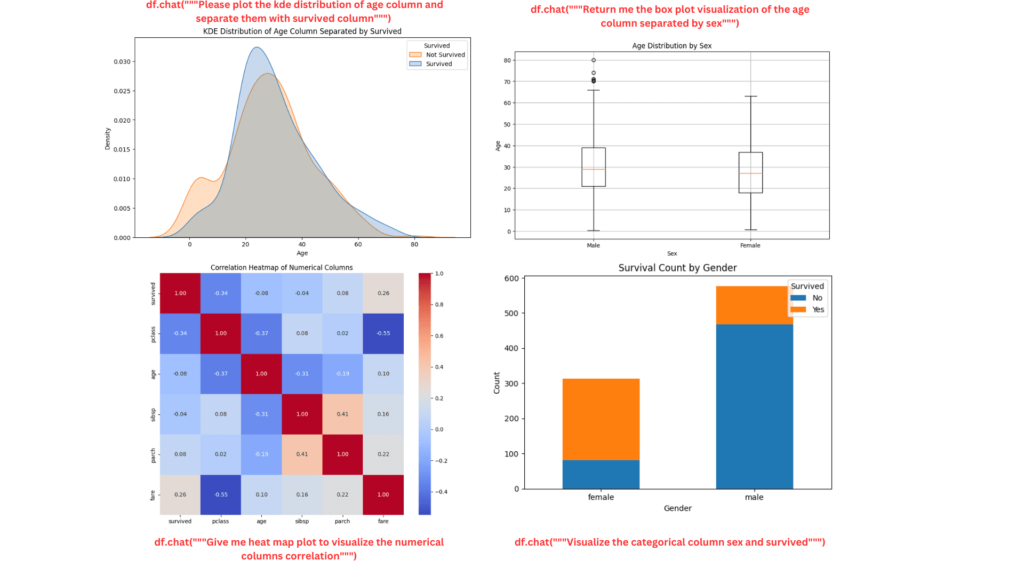
Are you proficient within the knowledge discipline utilizing Python? In that case, I guess most of you utilize Pandas for knowledge manipulation.
If you happen to don’t know, Pandas is an open-source Python bundle particularly developed for knowledge evaluation and manipulation. It’s one of many most-used packages and one you normally study when beginning an information science journey in Python.
So, what’s Pandas AI? I suppose you’re studying this text since you need to find out about it.
Effectively, as you realize, we’re in a time when Generative AI is all over the place. Think about when you can carry out knowledge evaluation in your knowledge utilizing Generative AI; issues can be a lot simpler.
That is what Pandas AI brings. With easy prompts, we will rapidly analyze and manipulate our dataset with out sending our knowledge someplace.
This text will discover learn how to make the most of Pandas AI for Knowledge Evaluation duties. Within the article, we’ll study the next:
- Pandas AI Setup
- Knowledge Exploration with Pandas AI
- Knowledge Visualization with Pandas AI
- Pandas AI Superior utilization
In case you are able to study, let’s get into it!
Pandas AI is a Python bundle that implements a Giant Language Mannequin (LLM) functionality into Pandas API. We will use customary Pandas API with Generative AI enhancement that turns Pandas right into a conversational device.
We primarily need to use Pandas AI due to the straightforward course of that the bundle offers. The bundle might routinely analyze knowledge utilizing a easy immediate with out requiring advanced code.
Sufficient introduction. Let’s get into the hands-on.
First, we have to set up the bundle earlier than the rest.
Subsequent, we should arrange the LLM we need to use for Pandas AI. There are a number of choices, corresponding to OpenAI GPT and HuggingFace. Nevertheless, we’ll use the OpenAI GPT for this tutorial.
Setting the OpenAI mannequin into Pandas AI is easy, however you would wish the OpenAI API Key. If you happen to don’t have one, you may get on their website.
If every little thing is prepared, let’s arrange the Pandas AI LLM utilizing the code under.
from pandasai.llm import OpenAI
llm = OpenAI(api_token="Your OpenAI API Key")
You are actually able to do Knowledge Evaluation with Pandas AI.
Knowledge Exploration with Pandas AI
Let’s begin with a pattern dataset and take a look at the info exploration with Pandas AI. I might use the Titanic knowledge from the Seaborn bundle on this instance.
import seaborn as sns
from pandasai import SmartDataframe
knowledge = sns.load_dataset('titanic')
df = SmartDataframe(knowledge, config = {'llm': llm})
We have to cross them into the Pandas AI Good Knowledge Body object to provoke the Pandas AI. After that, we will carry out conversational exercise on our DataFrame.
Let’s attempt a easy query.
response = df.chat("""Return the survived class in share""")
response
The share of passengers who survived is: 38.38%
From the immediate, Pandas AI might provide you with the answer and reply our questions.
We will ask Pandas AI questions that present solutions within the DataFrame object. For instance, listed below are a number of prompts for analyzing the info.
#Knowledge Abstract
abstract = df.chat("""Are you able to get me the statistical abstract of the dataset""")
#Class share
surv_pclass_perc = df.chat("""Return the survived in share breakdown by pclass""")
#Lacking Knowledge
missing_data_perc = df.chat("""Return the lacking knowledge share for the columns""")
#Outlier Knowledge
outlier_fare_data = response = df.chat("""Please present me the info rows that
incorporates outlier knowledge based mostly on fare column""")

Picture by Creator
You’ll be able to see from the picture above that the Pandas AI can present data with the DataFrame object, even when the immediate is sort of advanced.
Nevertheless, Pandas AI can’t deal with a calculation that’s too advanced because the packages are restricted to the LLM we cross on the SmartDataFrame object. Sooner or later, I’m positive that Pandas AI might deal with rather more detailed evaluation because the LLM functionality is evolving.
Knowledge Visualization with Pandas AI
Pandas AI is helpful for knowledge exploration and may carry out knowledge visualization. So long as we specify the immediate, Pandas AI will give the visualization output.
Let’s attempt a easy instance.
response = df.chat('Please present me the fare knowledge distribution visualization')
response
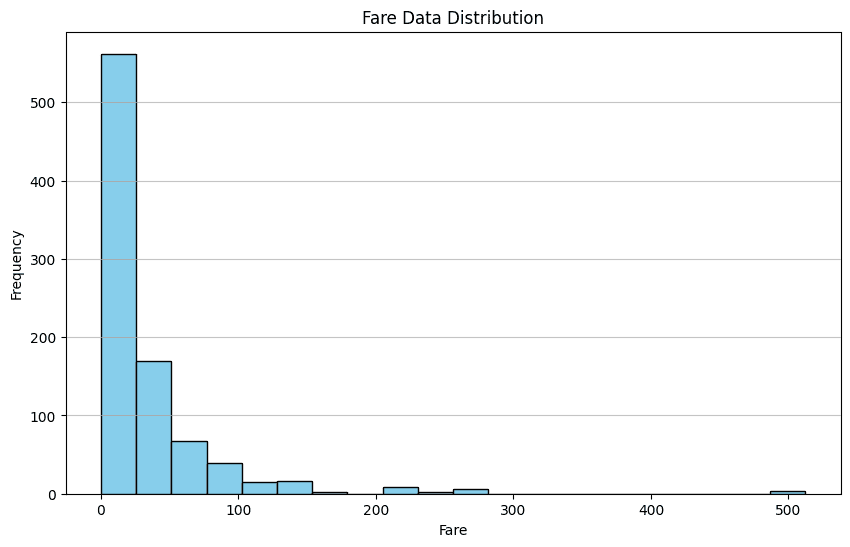
Picture by Creator
Within the instance above, we ask Pandas AI to visualise the distribution of the Fare column. The output is the Bar Chart distribution from the dataset.
Identical to Knowledge Exploration, you possibly can carry out any type of knowledge visualization. Nevertheless, Pandas AI nonetheless can’t deal with extra advanced visualization processes.
Listed below are another examples of Knowledge Visualization with Pandas AI.
kde_plot = df.chat("""Please plot the kde distribution of age column and separate them with survived column""")
box_plot = df.chat("""Return me the field plot visualization of the age column separated by intercourse""")
heat_map = df.chat("""Give me warmth map plot to visualise the numerical columns correlation""")
count_plot = df.chat("""Visualize the specific column intercourse and survived""")
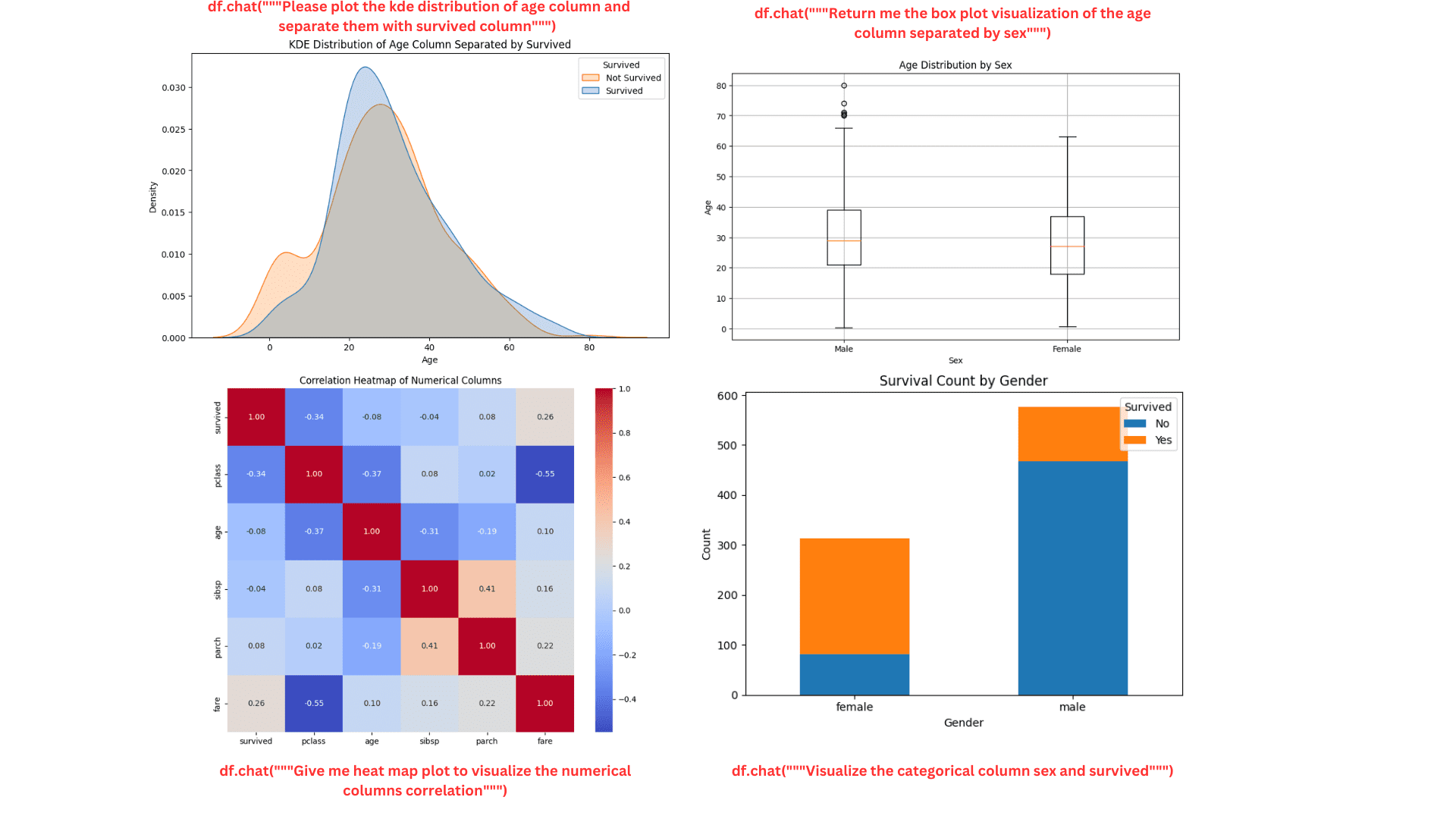
Picture by Creator
The plot appears to be like good and neat. You’ll be able to maintain asking the Pandas AI for extra particulars if crucial.
Pandas AI Advances Utilization
We will use a number of in-built APIs from Pandas AI to enhance the Pandas AI expertise.
Cache clearing
By default, all of the prompts and outcomes from the Pandas AI object are saved within the native listing to cut back the processing time and minimize the time the Pandas AI must name the mannequin.
Nevertheless, this cache might typically make the Pandas AI consequence irrelevant as they take into account the previous consequence. That’s why it’s good apply to clear the cache. You’ll be able to clear them with the next code.
import pandasai as pai
pai.clear_cache()
You may also flip off the cache at the start.
df = SmartDataframe(knowledge, {"enable_cache": False})
On this method, no immediate or result’s saved from the start.
Customized Head
It’s potential to cross a pattern head DataFrame to Pandas AI. It’s useful when you don’t need to share some non-public knowledge with the LLM or simply need to present an instance to Pandas AI.
To try this, you should utilize the next code.
from pandasai import SmartDataframe
import pandas as pd
# head df
head_df = knowledge.pattern(5)
df = SmartDataframe(knowledge, config={
"custom_head": head_df,
'llm': llm
})
Pandas AI Abilities and Brokers
Pandas AI permits customers to cross an instance operate and execute it with an Agent choice. For instance, the operate under combines two totally different DataFrame, and we cross a pattern plot operate for the Pandas AI agent to execute.
import pandas as pd
from pandasai import Agent
from pandasai.expertise import ability
employees_data = {
"EmployeeID": [1, 2, 3, 4, 5],
"Title": ["John", "Emma", "Liam", "Olivia", "William"],
"Division": ["HR", "Sales", "IT", "Marketing", "Finance"],
}
salaries_data = {
"EmployeeID": [1, 2, 3, 4, 5],
"Wage": [5000, 6000, 4500, 7000, 5500],
}
employees_df = pd.DataFrame(employees_data)
salaries_df = pd.DataFrame(salaries_data)
# Operate doc string to present extra context to the mannequin to be used of this ability
@ability
def plot_salaries(names: record[str], salaries: record[int]):
"""
Shows the bar chart having identify on x-axis and salaries on y-axis
Args:
names (record[str]): Workers' names
salaries (record[int]): Salaries
"""
# plot bars
import matplotlib.pyplot as plt
plt.bar(names, salaries)
plt.xlabel("Worker Title")
plt.ylabel("Wage")
plt.title("Worker Salaries")
plt.xticks(rotation=45)
# Including depend above for every bar
for i, wage in enumerate(salaries):
plt.textual content(i, wage + 1000, str(wage), ha="heart", va="backside")
plt.present()
agent = Agent([employees_df, salaries_df], config = {'llm': llm})
agent.add_skills(plot_salaries)
response = agent.chat("Plot the worker salaries in opposition to names")
The Agent would determine if they need to use the operate we assigned to the Pandas AI or not.
Combining Ability and Agent provides you a extra controllable consequence to your DataFrame evaluation.
Now we have discovered how simple it’s to make use of Pandas AI to assist our knowledge evaluation work. Utilizing the facility of LLM, we will restrict the coding portion of the info evaluation works and as a substitute concentrate on the crucial works.
On this article, we’ve discovered learn how to arrange Pandas AI, carry out knowledge exploration and visualization with Pandas AI, and advance utilization. You are able to do rather more with the bundle, so go to their documentation to study additional.
Cornellius Yudha Wijaya is an information science assistant supervisor and knowledge author. Whereas working full-time at Allianz Indonesia, he likes to share Python and knowledge ideas through social media and writing media. Cornellius writes on a wide range of AI and machine studying subjects.