Deploy and fine-tune basis fashions in Amazon SageMaker JumpStart with two traces of code
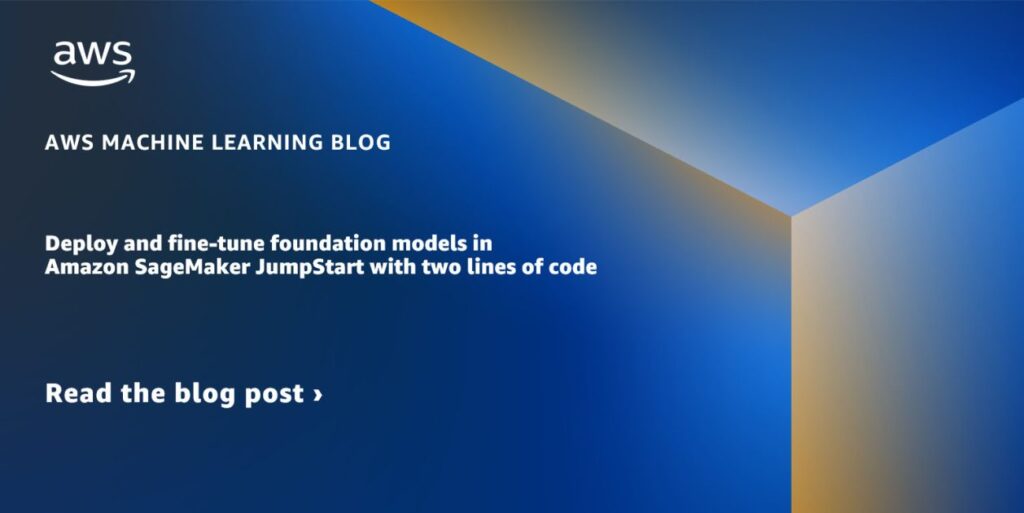
We’re excited to announce a simplified model of the Amazon SageMaker JumpStart SDK that makes it easy to construct, practice, and deploy basis fashions. The code for prediction can be simplified. On this submit, we show how you should use the simplified SageMaker Jumpstart SDK to get began with utilizing basis fashions in simply a few traces of code.
For extra details about the simplified SageMaker JumpStart SDK for deployment and coaching, seek advice from Low-code deployment with the JumpStartModel class and Low-code fine-tuning with the JumpStartEstimator class, respectively.
Answer overview
SageMaker JumpStart offers pre-trained, open-source fashions for a variety of drawback varieties that can assist you get began with machine studying (ML). You may incrementally practice and fine-tune these fashions earlier than deployment. JumpStart additionally offers answer templates that arrange infrastructure for frequent use instances, and executable instance notebooks for ML with Amazon SageMaker. You may entry the pre-trained fashions, answer templates, and examples by the SageMaker JumpStart touchdown web page in Amazon SageMaker Studio or use the SageMaker Python SDK.
To show the brand new options of the SageMaker JumpStart SDK, we present you tips on how to use the pre-trained Flan T5 XL mannequin from Hugging Face for textual content era for summarization duties. We additionally showcase how, in only a few traces of code, you’ll be able to fine-tune the Flan T5 XL mannequin for summarization duties. You should use some other mannequin for textual content era like Llama2, Falcon, or Mistral AI.
You will discover the pocket book for this answer utilizing Flan T5 XL within the GitHub repo.
Deploy and invoke the mannequin
Basis fashions hosted on SageMaker JumpStart have mannequin IDs. For the complete checklist of mannequin IDs, seek advice from Built-in Algorithms with pre-trained Model Table. For this submit, we use the mannequin ID of the Flan T5 XL textual content era mannequin. We instantiate the mannequin object and deploy it to a SageMaker endpoint by calling its deploy
technique. See the next code:
Subsequent, we invoke the mannequin to create a abstract of the supplied textual content utilizing the Flan T5 XL mannequin. The brand new SDK interface makes it easy so that you can invoke the mannequin: you simply have to cross the textual content to the predictor and it returns the response from the mannequin as a Python dictionary.
The next is the output of the summarization activity:
Fantastic-tune and deploy the mannequin
The SageMaker JumpStart SDK offers you with a brand new class, JumpStartEstimator
, which simplifies fine-tuning. You may present the situation of fine-tuning information and optionally cross validations datasets as properly. After you fine-tune the mannequin, use the deploy technique of the Estimator object to deploy the fine-tuned mannequin:
Customise the brand new courses within the SageMaker SDK
The brand new SDK makes it easy to deploy and fine-tune JumpStart fashions by defaulting many parameters. You continue to have the choice to override the defaults and customise the deployment and invocation primarily based in your necessities. For instance, you’ll be able to customise enter payload format kind, occasion kind, VPC configuration, and extra on your atmosphere and use case.
The next code reveals tips on how to override the occasion kind whereas deploying your mannequin:
The SageMaker JumpStart SDK deploy
perform will robotically choose a default content material kind and serializer for you. If you wish to change the format kind of the enter payload, you should use serializers
and content_types
objects to introspect the choices obtainable to you by passing the model_id
of the mannequin you’re working with. Within the following code, we set the payload enter format as JSON by setting JSONSerializer
as serializer
and software/json
as content_type
:
Subsequent, you’ll be able to invoke the Flan T5 XL mannequin for the summarization activity with a payload of the JSON format. Within the following code, we additionally cross inference parameters within the JSON payload for making responses extra correct:
For those who’re searching for extra methods to customise the inputs and different choices for internet hosting and fine-tuning, seek advice from the documentation for the JumpStartModel and JumpStartEstimator courses.
Conclusion
On this submit, we confirmed you ways you should use the simplified SageMaker JumpStart SDK for constructing, coaching, and deploying task-based and basis fashions in only a few traces of code. We demonstrated the brand new courses like JumpStartModel
and JumpStartEstimator
utilizing the Hugging Face Flan T5-XL mannequin for example. You should use any of the opposite SageMaker JumpStart basis fashions to be used instances equivalent to content material writing, code era, query answering, summarization, classification, data retrieval, and extra. To see the entire checklist of fashions obtainable with SageMaker JumpStart, seek advice from Built-in Algorithms with pre-trained Model Table. SageMaker JumpStart additionally helps task-specific models for a lot of common drawback varieties.
We hope the simplified interface of the SageMaker JumpStart SDK will make it easier to get began rapidly and allow you to ship quicker. We stay up for listening to how you employ the simplified SageMaker JumpStart SDK to create thrilling purposes!
In regards to the authors
Evan Kravitz is a software program engineer at Amazon Net Providers, engaged on SageMaker JumpStart. He’s within the confluence of machine studying with cloud computing. Evan obtained his undergraduate diploma from Cornell College and grasp’s diploma from the College of California, Berkeley. In 2021, he introduced a paper on adversarial neural networks on the ICLR convention. In his free time, Evan enjoys cooking, touring, and occurring runs in New York Metropolis.
Rachna Chadha is a Principal Answer Architect AI/ML in Strategic Accounts at AWS. Rachna is an optimist who believes that moral and accountable use of AI can enhance society sooner or later and convey financial and social prosperity. In her spare time, Rachna likes spending time along with her household, climbing, and listening to music.
Jonathan Guinegagne is a Senior Software program Engineer with Amazon SageMaker JumpStart at AWS. He received his grasp’s diploma from Columbia College. His pursuits span machine studying, distributed methods, and cloud computing, in addition to democratizing the usage of AI. Jonathan is initially from France and now lives in Brooklyn, NY.
Dr. Ashish Khetan is a Senior Utilized Scientist with Amazon SageMaker built-in algorithms and helps develop machine studying algorithms. He received his PhD from College of Illinois Urbana-Champaign. He’s an lively researcher in machine studying and statistical inference, and has printed many papers in NeurIPS, ICML, ICLR, JMLR, ACL, and EMNLP conferences.