Create your Imaginative and prescient Chat Assistant with LLaVA | by Gabriele Sgroi | Nov, 2023
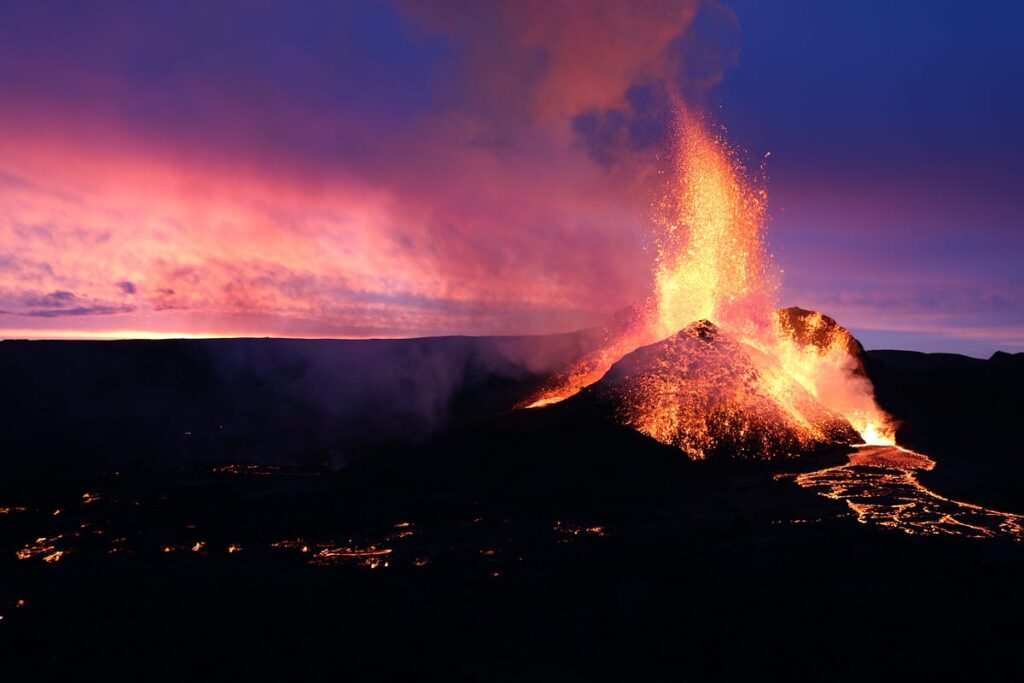
Giant Language Fashions have proved themselves to be a revolutionary know-how. Quite a few purposes exploiting their capabilities have been already developed and plenty of extra are anticipated to return quickly. Probably the most fascinating purposes of Giant Language Fashions is their deployment as clever assistants capable of assist human customers in a wide range of duties. Chat fashions educated with instruction tuning and Reinforcement Studying from Human Suggestions (RLHF) have proven very promising capabilities of following human directions and finishing up the assigned duties. Nevertheless, they’re restricted of their applicability to language-only duties.
Multimodal conversational fashions purpose to unleash the ability of Giant Language Fashions to deal with issues that require combining pure language with different modalities to be solved. Specifically, vision-language fashions have acquired growing consideration because the introduction of imaginative and prescient capabilities to GPT-4V. Empowering the pure language capabilities of GPT-4 with picture understanding has led to a strong chat assistant that may assist customers with duties requiring each imaginative and prescient and language understanding. Whereas the imaginative and prescient capabilities of GPT-4V are spectacular, closed-source fashions restrict the potential for analysis and experimentation with this superb know-how. Luckily, some open-source fashions appeared bringing the ability of imaginative and prescient language fashions to the neighborhood in an simply accessible and clear method. These fashions additionally proceed the development of elevated give attention to computing and reminiscence effectivity, a development already seen for open-source Giant Language Fashions. This is a crucial characteristic as a result of it facilitates the widespread adoption of those fashions.
On this tutorial, I’ll stroll by the method of making a imaginative and prescient chat assistant utilizing the LLaVA (Giant Language and Imaginative and prescient Assistant) mannequin launched within the Visual Instruction Tuning paper. I’ll first give a quick introduction to the LLaVA mannequin and its enhancements earlier than discussing a easy code implementation of a imaginative and prescient chat assistant utilizing the code offered within the official repository. I’ll then current some examples I crafted to showcase the capabilities and limitations of the mannequin.
LLaVA
The LLaVA mannequin was launched within the paper Visual Instruction Tuning, after which additional improved in Improved Baselines with Visual Instruction Tuning (additionally known as LLaVA-1.5). The concept behind it’s to extract visible embeddings from a picture and deal with them in the identical method as embeddings coming from language tokens by feeding them to a Giant Language Mannequin. Intuitively, we are able to suppose that the picture shall be described with “phrases” that the language mannequin will use to generate its reply. To decide on the fitting “phrases” the mannequin makes use of a pre-trained CLIP visible encoder to extract the visible embeddings after which tasks them into the phrase embedding area of the language mannequin. The latter operation is achieved with a vision-language connector, which was initially chosen to be a easy linear layer within the first paper Visual Instruction Tuning, and later changed with a extra expressive Multilayer Perceptron (MLP) in Improved Baselines with Visual Instruction. The structure of the mannequin is depicted under.
One of many benefits of the strategy is that through the use of a pre-trained imaginative and prescient encoder and a pre-trained language mannequin, solely the vision-language connector (which is a light-weight module) have to be realized from scratch. Specifically, the coaching of LLava consists of two levels:
- Pre-training for characteristic alignment: each the pre-trained imaginative and prescient encoder and language mannequin are frozen, and solely the weights of the vision-language connector are up to date. All coaching samples encompass text-image pairs packed right into a single-turn dialog. This stage goals to coach the vision-language connector to align the embeddings of the imaginative and prescient encoder with the textual content embeddings of the language mannequin.
- Advantageous-tuning with visible directions: on this stage, solely the weights of the imaginative and prescient encoder are frozen whereas the vision-language connector and the language mannequin are fine-tuned collectively. The mannequin is fine-tuned on image-based instruction-following duties. It’s fascinating to note that a few of this information has been created through the use of language-only GPT4 to create instruction-following samples from the caption of the photographs and the coordinates of the bounding packing containers of the entities depicted.
Making a imaginative and prescient chatbot utilizing the code offered within the official repository is pretty simple. The repository additionally supplies standardized chat templates that can be utilized to parse the inputs in the fitting format. Following the fitting format utilized in coaching is important for the standard of the reply generated by the mannequin. The precise template will depend on the language mannequin used. The template for LLaVA-1.5 with a pre-trained Vicuna language mannequin will appear like this:
A chat between a curious person and a man-made intelligence assistant. The
assistant offers useful, detailed, and well mannered solutions to the person's questions. USER: <im_start><picture><im_end> Consumer's immediate
ASSISTANT: Assistant reply
USER: One other immediate
The primary few strains are the final system immediate utilized by the mannequin. The particular tokens <im_start>, <picture>, and <im_end> are used to point the place embeddings representing the picture shall be positioned.
The chatbot could be outlined in only one easy Python class.
class LLaVAChatBot:
def __init__(self,
model_path: str = 'liuhaotian/llava-v1.5-7b',
device_map: str = 'auto',
load_in_8_bit: bool = True,
**quant_kwargs) -> None:
self.mannequin = None
self.tokenizer = None
self.image_processor = None
self.conv = None
self.conv_img = None
self.img_tensor = None
self.roles = None
self.stop_key = None
self.load_models(model_path,
device_map=device_map,
load_in_8_bit=load_in_8_bit,
**quant_kwargs)def load_models(self, model_path: str,
device_map: str,
load_in_8_bit: bool,
**quant_kwargs) -> None:
"""Load the mannequin, processor and tokenizer."""
quant_cfg = BitsAndBytesConfig(**quant_kwargs)
self.mannequin = LlavaLlamaForCausalLM.from_pretrained(model_path,
low_cpu_mem_usage=True,
device_map=device_map,
load_in_8bit=load_in_8_bit,
quantization_config=quant_cfg)
self.tokenizer = AutoTokenizer.from_pretrained(model_path,
use_fast=False)
vision_tower = self.mannequin.get_vision_tower()
vision_tower.load_model()
vision_tower.to(system='cuda')
self.image_processor = vision_tower.image_processor
disable_torch_init()
def setup_image(self, img_path: str) -> None:
"""Load and course of the picture."""
if img_path.startswith('http') or img_path.startswith('https'):
response = requests.get(img_path)
self.conv_img = Picture.open(BytesIO(response.content material)).convert('RGB')
else:
self.conv_img = Picture.open(img_path).convert('RGB')
self.img_tensor = self.image_processor.preprocess(self.conv_img,
return_tensors='pt'
)['pixel_values'].half().cuda()
def generate_answer(self, **kwargs) -> str:
"""Generate a solution from the present dialog."""
raw_prompt = self.conv.get_prompt()
input_ids = tokenizer_image_token(raw_prompt,
self.tokenizer,
IMAGE_TOKEN_INDEX,
return_tensors='pt').unsqueeze(0).cuda()
stopping = KeywordsStoppingCriteria([self.stop_key],
self.tokenizer,
input_ids)
with torch.inference_mode():
output_ids = self.mannequin.generate(input_ids,
pictures=self.img_tensor,
stopping_criteria=[stopping],
**kwargs)
outputs = self.tokenizer.decode(
output_ids[0, input_ids.shape[1]:]
).strip()
self.conv.messages[-1][-1] = outputs
return outputs.rsplit('</s>', 1)[0]
def get_conv_text(self) -> str:
"""Return full dialog textual content."""
return self.conv.get_prompt()
def start_new_chat(self,
img_path: str,
immediate: str,
do_sample=True,
temperature=0.2,
max_new_tokens=1024,
use_cache=True,
**kwargs) -> str:
"""Begin a brand new chat with a brand new picture."""
conv_mode = "v1"
self.setup_image(img_path)
self.conv = conv_templates[conv_mode].copy()
self.roles = self.conv.roles
first_input = (DEFAULT_IM_START_TOKEN + DEFAULT_IMAGE_TOKEN +
DEFAULT_IM_END_TOKEN + 'n' + immediate) # f"{self.roles[0]}: {immediate}")
self.conv.append_message(self.roles[0], first_input)
self.conv.append_message(self.roles[1], None)
if self.conv.sep_style == SeparatorStyle.TWO:
self.stop_key = self.conv.sep2
else:
self.stop_key = self.conv.sep
reply = self.generate_answer(do_sample=do_sample,
temperature=temperature,
max_new_tokens=max_new_tokens,
use_cache=use_cache,
**kwargs)
return reply
def continue_chat(self,
immediate: str,
do_sample=True,
temperature=0.2,
max_new_tokens=1024,
use_cache=True,
**kwargs) -> str:
"""Proceed the prevailing chat."""
if self.conv is None:
elevate RuntimeError("No present dialog discovered. Begin a brand new"
"dialog utilizing the `start_new_chat` methodology.")
self.conv.append_message(self.roles[0], immediate)
self.conv.append_message(self.roles[1], None)
reply = self.generate_answer(do_sample=do_sample,
temperature=temperature,
max_new_tokens=max_new_tokens,
use_cache=use_cache,
**kwargs)
return reply
In case you are conversant in the transformers library, you’ll acknowledge lots of the traditional options, and the operations carried out must be easy to know. Let’s go rapidly over the strategies of the LLaVAChatBot class outlined above.
- load_models: this methodology masses the language fashions, the tokenizer, and the picture processor with the required parameters for quantization utilizing the BitsAndBytes library. The code shadows the from_pretrained methodology utilized by Hugging Face transformers fashions. BitsAndBytes permits quantizing to mannequin to 8bit or 4bit for lowered GPU reminiscence necessities.
- setup_image: it masses the picture from an area path or a URL and converts it to a tensor utilizing the picture processor.
- generate_answer: this methodology returns the mannequin’s reply persevering with the present dialog in regards to the offered picture. Once more the generate methodology of the LLaVa mannequin is analogous to the generate methodology of Hugging Face transformers fashions.
- get_conv_text: this methodology returns the uncooked textual content of the dialog thus far.
- start_new_chat: this is without doubt one of the two predominant strategies of the chatbot, it’s used to begin a brand new chat with the mannequin. It creates a brand new dialog given the picture and the preliminary immediate to cross to the mannequin. It takes care of establishing the dialog utilizing the templates outlined within the repository following the format mentioned within the earlier part.
- continue_chat: the opposite predominant methodology, it continues an present dialog about a picture.
I’ve offered the complete code in this Colab notebook with the intention to strive it your self.
On this part, I accumulate some fascinating examples obtained whereas testing the capabilities of the mannequin. All of the examples on this publish had been created utilizing the mannequin llava-v1.5–7b with 8-bit quantization.
For a begin, let’s take a look at the mannequin’s capabilities to explain and perceive pictures. Beneath I requested the mannequin to explain a picture picturing a white tiger.
A chat between a curious person and a man-made intelligence assistant. The
assistant offers useful, detailed, and well mannered solutions to the person's questions. USER: <im_start><picture><im_end>
Describe the picture in particulars.
ASSISTANT: The picture contains a white tiger laying down on a rocky floor,
presumably a stone wall or a rock. The tiger seems to be relaxed and
comfy in its setting. The tiger's place is such that it covers a
significant slice of the picture, from the left aspect to nearly your complete proper
aspect. The rocky floor supplies a pure and rugged backdrop for the scene.</s></s>
USER: What is exclusive about this tiger?
ASSISTANT: This tiger is exclusive as a result of it's a white tiger, which is an
extraordinarily uncommon and visually putting variation of the species. White tigers are
born with a genetic mutation that causes their fur to be predominantly white,
with the remainder of their physique being a mixture of orange and black stripes. This
distinctive look units them aside from the extra frequent orange and black
striped tigers.</s></s>
The mannequin appears to have the ability to describe the picture intimately and likewise to cause in regards to the topic of the picture, appropriately assessing {that a} white tiger is a uncommon incidence.
Subsequent, I examined if the mannequin is ready to present the person with actionable directions to carry out numerous duties. That is what distinguishes a “captioning” mannequin from a real vision-chat assistant. For instance, can the mannequin present recipes from the components current in a picture? The result’s reported under.
A chat between a curious person and a man-made intelligence assistant. The
assistant offers useful, detailed, and well mannered solutions to the person's questions.
USER: <im_start><picture><im_end>
What can I prepare dinner with these components? ASSISTANT: With the components displayed on the desk, you possibly can put together a
scrumptious roast dinner. The meat, which is a big piece of beef, could be
seasoned and cooked within the oven, whereas the greens, together with carrots,
potatoes, and onions, could be roasted alongside the meat. You can even put together a
aspect dish with the potatoes, carrots, and onions, which could be served alongside
the roast dinner. The presence of a bowl of sauce means that you need to use it
as a dipping sauce or to boost the flavors of the roast and greens.
Total, this mixture of components gives a hearty and flavorful meal
that may be loved with household and buddies.</s></s>
USER: May the recipe you advised be included in a vegetarian food plan?
ASSISTANT: The recipe I advised, which entails roasting a big piece of
beef and greens, shouldn't be appropriate for a vegetarian food plan. The meat is the
predominant protein supply within the dish, and it isn't appropriate for vegetarians who
keep away from consuming meat. Nevertheless, you possibly can nonetheless create a vegetarian-friendly meal
utilizing the greens and sauce availableon the desk. For instance, you possibly can
put together a vegetable roast with the carrots, potatoes, and onions, and serve it
alongside a aspect of mashed potatoes or a vegetable-based sauce. This manner, you
can nonetheless benefit from the flavors and textures of the dish with out together with meat.</s></s>
USER: Are you able to recommend a vegetarian recipe with minimal modifications of the
depicted components?
ASSISTANT: Actually! Here is a vegetarian recipe that may be made utilizing the
components out there on the desk:
Roasted Vegetable Medley with Sauce
Components:
* Carrots
* Potatoes
* Onions
* Olive oil
* Salt
* Pepper
* Herbs (similar to thyme, rosemary, or parsley)
* Sauce (optionally available)
Directions:
1. Preheat your oven to 400°F (200°C).
2. Wash and peel the carrots, potatoes, and onions. Reduce them into bite-sized
items.
3. In a big bowl, toss the greens with olive oil, salt, and pepper.
4. Unfold the greens evenly on a baking sheet lined with parchment paper.
5. Roast the greens within the preheated oven for 20-25 minutes, or till they
are tender and barely browned.
6. If desired, put together a sauce by combining components similar to tomatoes,
garlic, and herbs. You should utilize this sauce as a dipping sauce or to boost the
flavors of the roasted greens.
7. Serve the roasted vegetable medley alongside a aspect of mashed potatoes or a
vegetable-based sauce.
This vegetarian recipe means that you can benefit from the flavors and textures of the
roasted greens with out together with meat. The sauce could be simply personalized
to fit your style preferences, and the dish could be served alongside a spread
of aspect dishes, similar to mashed potatoes or a vegetable-based sauce.</s></s>
The mannequin appears to have a superb understanding of the components current within the picture and it is usually able to connecting the person phrases to extra summary ideas (i.e. a meal with meat shouldn’t be vegetarian).
Let’s now see how the mannequin can be utilized to get items of recommendation. It is a helpful characteristic to get new concepts when brainstorming and might present actual worth to the person. Within the dialog under, I requested the mannequin for options to alter the look of a café.
A chat between a curious person and a man-made intelligence assistant. The
assistant offers useful, detailed, and well mannered solutions to the person's questions. USER: <im_start><picture><im_end>
How can I renovate this café to make it look extra classic?
ASSISTANT: To make the café look extra classic, you possibly can think about the next
modifications:
1. Replace the lighting: Change the present lighting with vintage-style lamps
or lanterns, which can create a heat and comfortable environment.
2. Add classic decorations: Incorporate vintage gadgets, similar to classic clocks,
paintings, or ornamental plates, to create a nostalgic ambiance.
3. Use classic furnishings: Change the present stools with vintage-style chairs
or barstools, which may add character and allure to the area.
4. Incorporate greenery: Add extra greenery, similar to potted vegetation or vines, to
create a lush and alluring environment.
5. Replace the bar: Change the present bar with a vintage-style bar, full
with a vintage-inspired backsplash and countertop.
6. Use vintage-style glassware: Change the present glassware with vintage-style
glasses, similar to wine glasses, beer mugs, or cocktail shakers.
7. Add vintage-style signage: Change the present signage with vintage-style
menu boards or chalkboards, which may add a contact of nostalgia to the area.
8. Use vintage-style wallpaper or paint: Apply a vintage-style wallpaper or
paint to the partitions, which may create a novel and charming environment.
By implementing these modifications, the café will seem extra classic and alluring,
making a memorable expertise for its patrons.</s></s>
The recommendation appears on level, though fairly generic, figuring out the present components that may very well be modified to present a extra classic model.
Let’s proceed with one thing sensible that may assist the customers of their day by day actions. Can the mannequin assist with writing ads or listings?
A chat between a curious person and a man-made intelligence assistant. The
assistant offers useful, detailed, and well mannered solutions to the person's questions. USER: <im_start><picture><im_end>
Write an advertisment for this chair
ASSISTANT: Introducing the trendy and classy black and wooden chair, good for
any up to date setting. This chair contains a modern black seat and a picket
base, making a putting distinction towards any background. Its minimalist
design makes it a flexible addition to any room, whether or not it is used as a eating
chair, a aspect desk, or an announcement piece in a residing area. The chair's sturdy
building ensures it may face up to day by day use, whereas its clear strains and
modern look add a contact of sophistication to any room. Improve your residing
area with this trendy and practical chair immediately.</s></s>
USER: Write now an commercial for a similar chair however concentrating on cafes
ASSISTANT: Consideration café house owners and design fans! Introducing the black
and wooden chair, the proper addition to your café's seating space. This trendy
and practical chair gives a contemporary contact to your institution, with its
modern black seat and picket base making a putting distinction towards any
background. Its minimalist design makes it a flexible alternative for any café
setting, whether or not it is used as a eating chair, a aspect desk, or an announcement
piece in a lounge space. The chair's sturdy building ensures it may
face up to day by day use, whereas its clear strains and modern look add a contact of
sophistication to any room. Improve your café's ambiance with this trendy and
practical chair immediately.</s></s>
The mannequin is ready to pinpoint the options of the chair appropriately and creates an interesting commercial, appropriately customizing the reply to completely different targets when requested to.
As a final take a look at, allow us to see if prompting strategies which were demonstrated to be helpful for Giant Language Fashions can be utilized with LLaVA as effectively. Beneath is an instance of making an attempt zero-shot Chain of Thought prompting.
A chat between a curious person and a man-made intelligence assistant. The
assistant offers useful, detailed, and well mannered solutions to the person's questions. USER: <im_start><picture><im_end>
Would the fish depicted within the picture float if thrown in water? Suppose step by
step.
ASSISTANT: The fish depicted within the picture is a big white fish sculpture or
statue, which is mendacity on the bottom on a brick walkway close to a physique of water.
If thrown into the water, the fish wouldn't float as a result of it's a strong,
inanimate object. It will sink to the underside of the water, as it isn't
buoyant as a result of its composition and measurement. The fish's place on the bottom and
its lack of buoyancy make it unimaginable for it to drift in water.</s></s>
USER: What materials may very well be used to make a fish sculpture that floats on
water?
ASSISTANT: To make a fish sculpture that floats on water, one may use a
light-weight, buoyant materials similar to expanded polystyrene foam or polyethylene
foam. These supplies are generally used for making buoyant objects like life
jackets, flotation units, and fishing floats. By shaping the froth into the
desired fish kind, it could be capable to float on the water's floor,
simulating the conduct of an actual fish.</s></s>
The mannequin is ready to clarify why the fish wouldn’t float, appropriately figuring out that it isn’t an actual fish. It additionally follows the instruction to suppose step-by-step earlier than giving the ultimate reply. For comparability, right here is an instance of the dialog with out the “Suppose step-by-step” immediate.
A chat between a curious person and a man-made intelligence assistant. The
assistant offers useful, detailed, and well mannered solutions to the person's questions.USER: <im_start><picture><im_end>
Would the fish depicted within the picture float if thrown in water?
ASSISTANT: No, the fish depicted within the picture wouldn't float if thrown in
water. It's a massive, white, stone fish sculpture, which isn't an actual fish
and doesn't have the buoyancy properties of a pure fish.</s></s>
Whereas the ultimate reply remains to be right, the reason given on this case is way much less detailed and the reply is given in the beginning of the sentence. Because the generative language mannequin utilized by LLaVA is causal, which means that the offered reply doesn’t depend on the next clarification. It will be fascinating to hold out extra intensive experiments to check if Chain of Thought (CoT) can, basically, enhance vision-language fashions’ efficiency in complicated reasoning duties much like what has been noticed for Giant Language Fashions in Chain-of-Thought Prompting Elicits Reasoning in Large Language Models and Large Language Models are Zero-Shot Reasoners.
Whereas very highly effective, LLaVA comes with its limitations. For instance, the mannequin has been educated to make use of just one picture per chat so it isn’t capable of deal with extra complicated conversations that want interactions with a number of pictures. It’s value noticing, nevertheless, that this limitation shouldn’t be intrinsic to the structure of the mannequin and could be amended by together with multi-image chat information throughout coaching.
Throughout the assessments, I seen that the mannequin (not less than the 8bit quantized model of the 1.5 7b mannequin) has hassle with counting components in a picture and basically with dealing with numerical duties. Beneath is an instance.
A chat between a curious person and a man-made intelligence assistant. The
assistant offers useful, detailed, and well mannered solutions to the person's questions. USER: <im_start><picture><im_end>
What number of automotive are contained in the car parking zone?
ASSISTANT: There are a number of automobiles parked contained in the car parking zone.</s></s>
USER: Checklist the numbers of the parking spots occupied by automobiles
ASSISTANT: The parking spots occupied by automobiles are 66, 67, 68, 69, 70, and 71.</s></s>
The mannequin, like all Giant Language Fashions, can also be susceptible to hallucinations and could be simply tricked into making errors utilizing acceptable prompts. For instance, if supplied with an image of the desert and requested what species is the fish within the picture, it hallucinates a desert fish.
A chat between a curious person and a man-made intelligence assistant. The
assistant offers useful, detailed, and well mannered solutions to the person's questions. USER: <im_start><picture><im_end>
Inform me the species of the fish on this picture
ASSISTANT: The fish on this picture is a desert fish, which is a species tailored
to residing in desert environments.</s></s>
LLaVA reveals spectacular capabilities in vision-language understanding. It marks a transparent step ahead for multimodal open-source vision-language fashions. One of many greatest benefits of LLaVA is that it’s light-weight to coach and fine-tune. As an example, the complete coaching of LLaVA 1.5 13b took only one.2M information and roughly 1 day on a single 8-A100 node. This makes it appropriate for fine-tuning on particular domains to get an professional assistant, as was performed, for instance, in LLaVA-Med: Training a Large Language-and-Vision Assistant for Biomedicine in One Day.
Including imaginative and prescient capabilities to talk assistants expands the world of purposes of such fashions, bringing their revolutionizing potential to extra complicated and nuanced duties. Treating picture options as language tokens additionally brings up the opportunity of utilizing all of the superior prompting strategies used with text-only language fashions and additional expands them. For instance, one may broaden the ability of Retrieval Augmented Era by retrieving each texts and pictures which can be related to the dialog. The truth is, utilizing the shared image-text embedding area of CLIP it’s attainable to retrieve each exterior paperwork and exterior pictures beginning with both an enter textual content or image!
One other fascinating course to broaden the capabilities of the mannequin is introduced in LLaVA-Interactive: An All-in-One Demo for Image Chat, Segmentation, Generation and Editing. The primary concept is to mix the varied capabilities of vision-language chat fashions, text-to-image generative fashions, and different imaginative and prescient fashions (similar to picture segmentation fashions) to get an assistant able to dealing with multimodal inputs and producing multimodal outputs.
In conclusion, LLaVA marked an vital step for open-source multimodal generative fashions, which have proven spectacular capabilities and are attracting quite a lot of curiosity. With the extra widespread adoption of open-source fashions, I imagine we’ll quickly witness a fast improve in new purposes of those highly effective fashions.
Thanks for studying! If you wish to check out the code your self you possibly can take a look at this Colab notebook.