Getting Began with SQL in 5 Steps
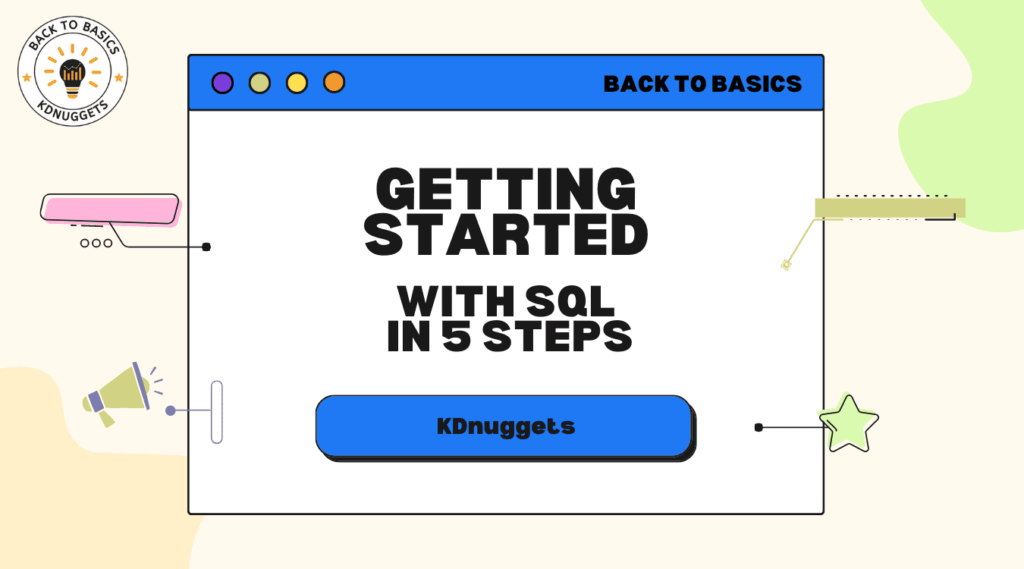
In terms of managing and manipulating information in relational databases, Structured Question Language (SQL) is the most important title within the sport. SQL is a serious domain-specific language which serves because the cornerstone for database administration, and which supplies a standardized solution to work together with databases. With information being the driving power behind decision-making and innovation, SQL stays a vital know-how demanding top-level consideration from information analysts, builders, and information scientists.
SQL was initially developed by IBM within the Nineteen Seventies, and have become standardized by ANSI and ISO within the late Eighties. All kinds of organizations — from small companies to universities to main companies — depend on SQL databases reminiscent of MySQL, SQL Server, and PostgreSQL to deal with large-scale information. SQL’s significance continues to develop with the enlargement of data-driven industries. Its common utility makes it an important ability for numerous professionals, within the information realm and past.
SQL permits customers to carry out numerous data-related duties, together with:
- Querying information
- Inserting new information
- Updating present information
- Deleting information
- Creating and modifying tables
This tutorial will provide a step-by-step walkthrough of SQL, specializing in getting began with intensive hands-on examples.
Selecting a SQL Database Administration System (DBMS)
Earlier than diving into SQL queries, you may want to decide on a database administration system (DBMS) that fits your undertaking’s wants. The DBMS serves because the spine in your SQL actions, providing completely different options, efficiency optimizations, and pricing fashions. Your alternative of a DBMS can have a major influence on the way you work together together with your information.
- MySQL: Open supply, broadly adopted, utilized by Fb and Google. Appropriate for quite a lot of purposes, from small initiatives to enterprise-level purposes.
- PostgreSQL: Open supply, sturdy options, utilized by Apple. Identified for its efficiency and requirements compliance.
- SQL Server Categorical: Microsoft’s entry-level possibility. Best for small to medium purposes with restricted necessities for scalability.
- SQLite: Light-weight, serverless, and self-contained. Best for cell apps and small initiatives.
Set up Information for MySQL
For the sake of this tutorial, we are going to deal with MySQL because of its widespread utilization and complete characteristic set. Putting in MySQL is a simple course of:
- Go to MySQL’s website and obtain the installer acceptable in your working system.
- Run the installer, following the on-screen directions.
- Through the setup, you may be prompted to create a root account. Be certain that to recollect or securely retailer the foundation password.
- As soon as set up is full, you possibly can entry the MySQL shell by opening a terminal and typing
mysql -u root -p
. You may be prompted to enter the foundation password. - After profitable login, you may be greeted with the MySQL immediate, indicating that your MySQL server is up and operating.
Setting Up a SQL IDE
An Built-in Improvement Atmosphere (IDE) can considerably improve your SQL coding expertise by offering options like auto-completion, syntax highlighting, and database visualization. An IDE just isn’t strictly essential for operating SQL queries, however it’s extremely advisable for extra complicated duties and bigger initiatives.
- DBeaver: Open supply and helps a variety of DBMS, together with MySQL, PostgreSQL, SQLite, and SQL Server.
- MySQL Workbench: Developed by Oracle, that is the official IDE for MySQL and presents complete instruments tailor-made for MySQL.
After downloading and putting in your chosen IDE, you may want to attach it to your MySQL server. This often includes specifying the server’s IP deal with (localhost
if the server is in your machine), the port quantity (often 3306 for MySQL), and the credentials for a licensed database consumer.
Testing Your Setup
Let’s ensure that all the pieces is working appropriately. You are able to do this by operating a easy SQL question to show all present databases:
If this question returns a listing of databases, and no errors, then congratulations! Your SQL surroundings has been efficiently arrange, and you’re prepared to begin SQL programming.
Making a Database and Tables
Earlier than including or manipulating information, you’ll first want each a database and one desk, at minimal. Making a database and a desk is completed by:
CREATE DATABASE sql_tutorial;
USE sql_tutorial;
CREATE TABLE prospects (
id INT PRIMARY KEY AUTO_INCREMENT,
title VARCHAR(50),
e-mail VARCHAR(50)
);
Manipulating Information
Now you’re prepared for information manipulation. Let’s take a look on the primary CRUD operations:
- Insert:
INSERT INTO prospects (title, e-mail) VALUES ('John Doe', 'john@e-mail.com');
- Question:
SELECT * FROM prospects;
- Replace:
UPDATE prospects SET e-mail="john@newemail.com" WHERE id = 1;
- Delete:
DELETE FROM prospects WHERE id = 1;
Filtering and Sorting
Filtering in SQL includes utilizing situations to selectively retrieve rows from a desk, typically utilizing the WHERE
clause. Sorting in SQL arranges the retrieved information in a selected order, usually utilizing the ORDER BY
clause. Pagination in SQL divides the end result set into smaller chunks, displaying a restricted variety of rows per web page.
- Filter:
SELECT * FROM prospects WHERE title="John Doe";
- Type:
SELECT * FROM prospects ORDER BY title ASC;
- Paginate:
SELECT * FROM prospects LIMIT 10 OFFSET 20;
Information Sorts and Constraints
Understanding information varieties and constraints is essential for outlining the construction of your tables. Information varieties specify what sort of information a column can maintain, reminiscent of integers, textual content, or dates. Constraints implement limitations to make sure information integrity.
- Integer Sorts: INT, SMALLINT, TINYINT, and so forth. Used for storing complete numbers.
- Decimal Sorts: FLOAT, DOUBLE, DECIMAL. Appropriate for storing numbers with decimal locations.
- Character Sorts: CHAR, VARCHAR, TEXT. Used for textual content information.
- Date and Time: DATE, TIME, DATETIME, TIMESTAMP. Designed for storing date and time data.
CREATE TABLE workers (
id INT PRIMARY KEY AUTO_INCREMENT,
first_name VARCHAR(50) NOT NULL,
last_name VARCHAR(50) NOT NULL,
birth_date DATE,
e-mail VARCHAR(50) UNIQUE,
wage FLOAT CHECK (wage > 0)
);
Within the above instance, the NOT NULL
constraint ensures {that a} column can’t have a NULL worth. The UNIQUE
constraint ensures that each one values in a column are distinctive. The CHECK
constraint validates that the wage have to be better than zero.
Becoming a member of Tables
Joins are used to mix rows from two or extra tables based mostly on a associated column between them. They’re important while you need to retrieve information that’s unfold throughout a number of tables. Understanding joins is essential for complicated SQL queries.
- INNER JOIN:
SELECT * FROM orders JOIN prospects ON orders.customer_id = prospects.id;
- LEFT JOIN:
SELECT * FROM orders LEFT JOIN prospects ON orders.customer_id = prospects.id;
- RIGHT JOIN:
SELECT * FROM orders RIGHT JOIN prospects ON orders.customer_id = prospects.id;
Joins will be complicated however are extremely highly effective when it is advisable pull information from a number of tables. Let’s undergo an in depth instance to make clear how several types of joins work.
Take into account two tables: Staff and Departments.
-- Staff Desk
CREATE TABLE Staff (
id INT PRIMARY KEY,
title VARCHAR(50),
department_id INT
);
INSERT INTO Staff (id, title, department_id) VALUES
(1, 'Winifred', 1),
(2, 'Francisco', 2),
(3, 'Englebert', NULL);
-- Departments Desk
CREATE TABLE Departments (
id INT PRIMARY KEY,
title VARCHAR(50)
);
INSERT INTO Departments (id, title) VALUES
(1, 'R&D'),
(2, 'Engineering'),
(3, 'Gross sales');
Let’s discover several types of joins:
-- INNER JOIN
-- Returns information which have matching values in each tables
SELECT E.title, D.title
FROM Staff E
INNER JOIN Departments D ON E.department_id = D.id;
-- LEFT JOIN (or LEFT OUTER JOIN)
-- Returns all information from the left desk,
-- and the matched information from the best desk
SELECT E.title, D.title
FROM Staff E
LEFT JOIN Departments D ON E.department_id = D.id;
-- RIGHT JOIN (or RIGHT OUTER JOIN)
-- Returns all information from the best desk
-- and the matched information from the left desk
SELECT E.title, D.title
FROM Staff E
RIGHT JOIN Departments D ON E.department_id = D.id;
Within the above examples, the INNER JOIN returns solely the rows the place there’s a match in each tables. The LEFT JOIN returns all rows from the left desk, and matching rows from the best desk, filling with NULL if there isn’t any match. The RIGHT JOIN does the alternative, returning all rows from the best desk and matching rows from the left desk.
Grouping and Aggregation
Aggregation features carry out a calculation on a set of values and return a single worth. Aggregations are generally used alongside GROUP BY clauses to section information into classes and carry out calculations on every group.
- Depend:
SELECT customer_id, COUNT(id) AS total_orders FROM orders GROUP BY customer_id;
- Sum:
SELECT customer_id, SUM(order_amount) AS total_spent FROM orders GROUP BY customer_id;
- Filter group:
SELECT customer_id, SUM(order_amount) AS total_spent FROM orders GROUP BY customer_id HAVING total_spent > 100;
Subqueries and Nested Queries
Subqueries permit you to carry out queries inside queries, offering a solution to fetch information that will likely be utilized in the principle question as a situation to additional prohibit the info that’s retrieved.
SELECT *
FROM prospects
WHERE id IN (
SELECT customer_id
FROM orders
WHERE orderdate > '2023-01-01'
);
Transactions
Transactions are sequences of SQL operations which are executed as a single unit of labor. They’re essential for sustaining the integrity of database operations, significantly in multi-user techniques. Transactions comply with the ACID ideas: Atomicity, Consistency, Isolation, and Sturdiness.
BEGIN;
UPDATE accounts SET steadiness = steadiness - 500 WHERE id = 1;
UPDATE accounts SET steadiness = steadiness + 500 WHERE id = 2;
COMMIT;
Within the above instance, each UPDATE statements are wrapped inside a transaction. Both each execute efficiently, or if an error happens, neither execute, guaranteeing information integrity.
Understanding Question Efficiency
Question efficiency is essential for sustaining a responsive database system. An inefficient question can result in delays, affecting the general consumer expertise. Listed below are some key ideas:
- Execution Plans: These plans present a roadmap of how a question will likely be executed, permitting for evaluation and optimization.
- Bottlenecks: Figuring out gradual components of a question can information optimization efforts. Instruments just like the SQL Server Profiler can help on this course of.
Indexing Methods
Indexes are information buildings that improve the velocity of knowledge retrieval. They’re very important in giant databases. This is how they work:
- Single-Column Index: An index on a single column, typically utilized in WHERE clauses;
CREATE INDEX idx_name ON prospects (title);
- Composite Index: An index on a number of columns, used when queries filter by a number of fields;
CREATE INDEX idx_name_age ON prospects (title, age);
- Understanding When to Index: Indexing improves studying velocity however can decelerate insertions and updates. Cautious consideration is required to steadiness these elements.
Optimizing Joins and Subqueries
Joins and subqueries will be resource-intensive. Optimization methods embrace:
- Utilizing Indexes: Making use of indexes on be part of fields improves be part of efficiency.
- Decreasing Complexity: Decrease the variety of tables joined and the variety of rows chosen.
SELECT prospects.title, COUNT(orders.id) AS total_orders
FROM prospects
JOIN orders ON prospects.id = orders.customer_id
GROUP BY prospects.title
HAVING orders > 2;
Database Normalization and Denormalization
Database design performs a major function in efficiency:
- Normalization: Reduces redundancy by organizing information into associated tables. This will make queries extra complicated however ensures information consistency.
- Denormalization: Combines tables to enhance learn efficiency at the price of potential inconsistency. It is used when learn velocity is a precedence.
Monitoring and Profiling Instruments
Using instruments to observe efficiency ensures that the database runs easily:
- MySQL’s Efficiency Schema: Gives insights into question execution and efficiency.
- SQL Server Profiler: Permits monitoring and capturing of SQL Server occasions, serving to in analyzing efficiency.
Finest Practices in Writing Environment friendly SQL
Adhering to finest practices makes SQL code extra maintainable and environment friendly:
- Keep away from SELECT *: Choose solely required columns to scale back load.
- Decrease Wildcards: Use wildcards sparingly in LIKE queries.
- Use EXISTS As a substitute of COUNT: When checking for existence, EXISTS is extra environment friendly.
SELECT id, title
FROM prospects
WHERE EXISTS (
SELECT 1
FROM orders
WHERE customer_id = prospects.id
);
Database Upkeep
Common upkeep ensures optimum efficiency:
- Updating Statistics: Helps the database engine make optimization selections.
- Rebuilding Indexes: Over time, indexes turn out to be fragmented. Common rebuilding improves efficiency.
- Backups: Common backups are important for information integrity and restoration.
Efficiency Finest Practices
Optimizing the efficiency of your SQL queries and database is essential for sustaining a responsive and environment friendly system. Listed below are some efficiency finest practices:
- Use Indexes Properly: Indexes velocity up information retrieval however can decelerate information modification operations like insert, replace, and delete.
- Restrict Outcomes: Use the
LIMIT
clause to retrieve solely the info you want. - Optimize Joins: All the time be part of tables on listed or main key columns.
- Analyze Question Plans: Understanding the question execution plan will help you optimize queries.
Safety Finest Practices
Safety is paramount when coping with databases, as they typically comprise delicate data. Listed below are some finest practices for enhancing SQL safety:
- Information Encryption: All the time encrypt delicate information earlier than storing it.
- Person Privileges: Grant customers the least quantity of privileges they should carry out their duties.
- SQL Injection Prevention: Use parameterized queries to guard towards SQL injection assaults.
- Common Audits: Conduct common safety audits to establish vulnerabilities.
Combining Efficiency and Safety
Hanging the best steadiness between efficiency and safety is commonly difficult however essential. For instance, whereas indexing can velocity up information retrieval, it may well additionally make delicate information extra accessible. Due to this fact, all the time contemplate the safety implications of your efficiency optimization methods.
Instance: Safe and Environment friendly Question
-- Utilizing a parameterized question to each optimize
-- efficiency and forestall SQL injection
PREPARE secureQuery FROM 'SELECT * FROM customers WHERE age > ? AND age < ?';
SET @min_age = 18, @max_age = 35;
EXECUTE secureQuery USING @min_age, @max_age;
This instance makes use of a parameterized question, which not solely prevents SQL injection but additionally permits MySQL to cache the question, enhancing efficiency.
This getting began information has lined the elemental ideas and common sensible purposes of SQL. From getting up and operating to mastering complicated queries, this information ought to have offered you with the talents it is advisable navigate information administration by way of using detailed examples and with a sensible strategy. As information continues to form our world, mastering SQL opens the door to quite a lot of fields, together with information analytics, machine studying, and software program improvement.
As you progress, contemplate extending your SQL ability set with extra assets. Websites like w3schools SQL Tutorial and SQL Practice Exercises on SQLBolt present extra examine supplies and workouts. Moreover, HackerRank’s SQL problems present goal-oriented question apply. Whether or not you are constructing a posh information analytics platform or creating the following technology of internet purposes, SQL is a ability you’ll positively be utilizing recurrently. Do not forget that the journey to SQL mastery traverses a protracted street, and is a journey that’s enriched by constant apply and studying.
Matthew Mayo (@mattmayo13) holds a Grasp’s diploma in pc science and a graduate diploma in information mining. As Editor-in-Chief of KDnuggets, Matthew goals to make complicated information science ideas accessible. His skilled pursuits embrace pure language processing, machine studying algorithms, and exploring rising AI. He’s pushed by a mission to democratize information within the information science neighborhood. Matthew has been coding since he was 6 years outdated.