LGBMClassifier: A Getting Began Information

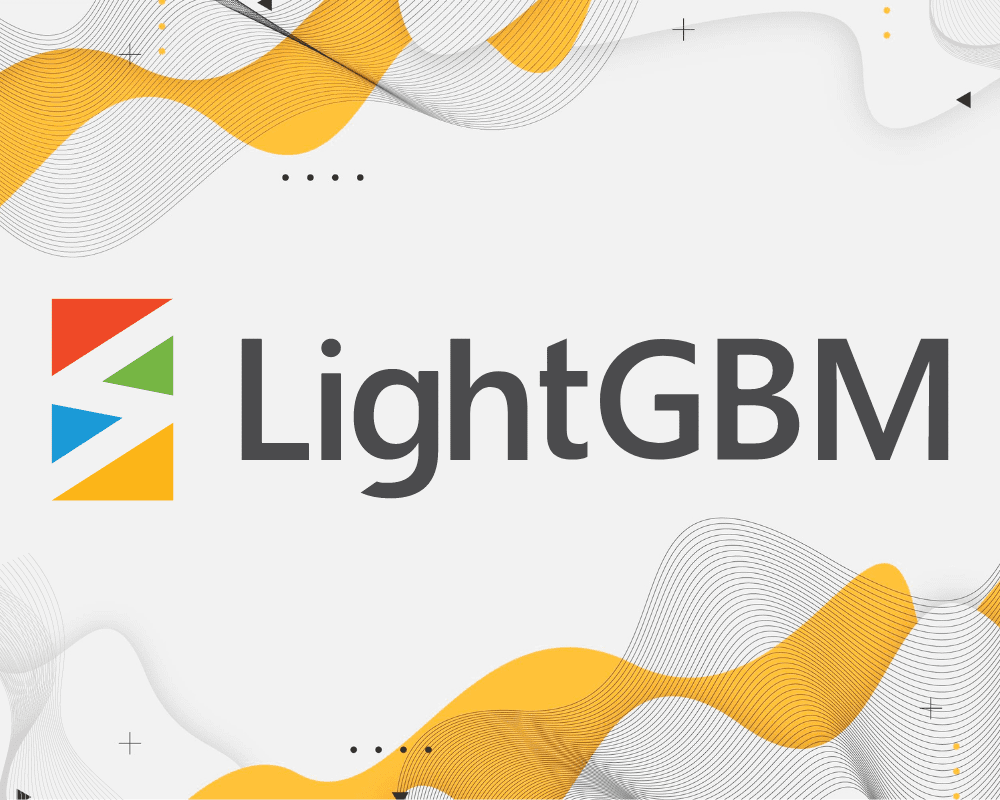
Picture by Editor
There are an unlimited variety of machine studying algorithms which can be apt to mannequin particular phenomena. Whereas some fashions make the most of a set of attributes to outperform others, others embody weak learners to make the most of the rest of attributes for offering further data to the mannequin, often known as ensemble fashions.
The premise of the ensemble fashions is to enhance the mannequin efficiency by combining the predictions from completely different fashions by decreasing their errors. There are two well-liked ensembling strategies: bagging and boosting.
Bagging, aka Bootstrapped Aggregation, trains a number of particular person fashions on completely different random subsets of the coaching information after which averages their predictions to supply the ultimate prediction. Boosting, however, includes coaching particular person fashions sequentially, the place every mannequin makes an attempt to appropriate the errors made by the earlier fashions.
Now that we now have context concerning the ensemble fashions, allow us to double-click on the boosting ensemble mannequin, particularly the Mild GBM (LGBM) algorithm developed by Microsoft.
LGBMClassifier stands for Mild Gradient Boosting Machine Classifier. It makes use of determination tree algorithms for rating, classification, and different machine-learning duties. LGBMClassifier makes use of a novel strategy of Gradient-based One-Aspect Sampling (GOSS) and Unique Function Bundling (EFB) to deal with large-scale information with accuracy, successfully making it sooner and decreasing reminiscence utilization.
What’s Gradient-based One-Aspect Sampling (GOSS)?
Conventional gradient boosting algorithms use all the info for coaching, which may be time-consuming when coping with massive datasets. LightGBM’s GOSS, however, retains all of the cases with massive gradients and performs random sampling on the cases with small gradients. The instinct behind that is that cases with massive gradients are more durable to suit and thus carry extra data. GOSS introduces a relentless multiplier for the info cases with small gradients to compensate for the knowledge loss throughout sampling.
What’s Unique Function Bundling (EFB)?
In a sparse dataset, many of the options are zeros. EFB is a near-lossless algorithm that bundles/combines mutually unique options (options that aren’t non-zero concurrently) to scale back the variety of dimensions, thereby accelerating the coaching course of. Since these options are “unique”, the unique characteristic area is retained with out important data loss.
The LightGBM package deal may be put in instantly utilizing pip – python’s package deal supervisor. Sort the command shared beneath both on the terminal or command immediate to obtain and set up the LightGBM library onto your machine:
Anaconda customers can set up it utilizing the “conda set up” command as listed beneath.
conda set up -c conda-forge lightgbm
Primarily based in your OS, you possibly can select the set up technique utilizing this guide.
Now, let’s import LightGBM and different crucial libraries:
import numpy as np
import pandas as pd
import seaborn as sns
import lightgbm as lgb
from sklearn.metrics import classification_report
from sklearn.model_selection import train_test_split
Getting ready the Dataset
We’re utilizing the favored Titanic dataset, which accommodates details about the passengers on the Titanic, with the goal variable signifying whether or not they survived or not. You’ll be able to obtain the dataset from Kaggle or use the next code to load it instantly from Seaborn, as proven beneath:
titanic = sns.load_dataset('titanic')
Drop pointless columns akin to “deck”, “embark_town”, and “alive” as a result of they’re redundant or don’t contribute to the survival of any individual on the ship. Subsequent, we noticed that the options “age”, “fare”, and “embarked” have lacking values – word that completely different attributes are imputed with acceptable statistical measures.
# Drop pointless columns
titanic = titanic.drop(['deck', 'embark_town', 'alive'], axis=1)
# Exchange lacking values with the median or mode
titanic['age'] = titanic['age'].fillna(titanic['age'].median())
titanic['fare'] = titanic['fare'].fillna(titanic['fare'].mode()[0])
titanic['embarked'] = titanic['embarked'].fillna(titanic['embarked'].mode()[0])
Lastly, we convert the specific variables to numerical variables utilizing pandas’ categorical codes. Now, the info is ready to begin the mannequin coaching course of.
# Convert categorical variables to numerical variables
titanic['sex'] = pd.Categorical(titanic['sex']).codes
titanic['embarked'] = pd.Categorical(titanic['embarked']).codes
# Cut up the dataset into enter options and the goal variable
X = titanic.drop('survived', axis=1)
y = titanic['survived']
Coaching the LGBMClassifier Mannequin
To start coaching the LGBMClassifier mannequin, we have to break up the dataset into enter options and goal variables, in addition to coaching and testing units utilizing the train_test_split perform from scikit-learn.
# Cut up the dataset into coaching and testing units
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Let’s label encode categorical (“who”) and ordinal information (“class”) to make sure that the mannequin is equipped with numerical information, as LGBM doesn’t eat non-numerical information.
class_dict = {
"Third": 3,
"First": 1,
"Second": 2
}
who_dict = {
"youngster": 0,
"lady": 1,
"man": 2
}
X_train['class'] = X_train['class'].apply(lambda x: class_dict[x])
X_train['who'] = X_train['who'].apply(lambda x: who_dict[x])
X_test['class'] = X_test['class'].apply(lambda x: class_dict[x])
X_test['who'] = X_test['who'].apply(lambda x: who_dict[x])
Subsequent, we specify the mannequin hyperparameters as arguments to the constructor, or we are able to move them as a dictionary to the set_params technique.
The final step to provoke the mannequin coaching is to load the dataset by creating an occasion of the LGBMClassifier class and becoming it to the coaching information.
params = {
'goal': 'binary',
'boosting_type': 'gbdt',
'num_leaves': 31,
'learning_rate': 0.05,
'feature_fraction': 0.9
}
clf = lgb.LGBMClassifier(**params)
clf.match(X_train, y_train)
Subsequent, allow us to consider the skilled classifier’s efficiency on the unseen or check dataset.
predictions = clf.predict(X_test)
print(classification_report(y_test, predictions))
precision recall f1-score help
0 0.84 0.89 0.86 105
1 0.82 0.76 0.79 74
accuracy 0.83 179
macro avg 0.83 0.82 0.82 179
weighted avg 0.83 0.83 0.83 179
Hyperparameter Tuning
The LGBMClassifier permits for a lot flexibility through hyperparameters which you’ll tune for optimum efficiency. Right here, we are going to briefly talk about a few of the key hyperparameters:
- num_leaves: That is the primary parameter to regulate the complexity of the tree mannequin. Ideally, the worth of num_leaves must be lower than or equal to 2^(max_depth).
- min_data_in_leaf: This is a vital parameter to forestall overfitting in a leaf-wise tree. Its optimum worth is dependent upon the variety of coaching samples and num_leaves.
- max_depth: You need to use this to restrict the tree depth explicitly. It is best to tune this parameter in case of overfitting.
Let’s tune these hyperparameters and prepare a brand new mannequin:
mannequin = lgb.LGBMClassifier(num_leaves=31, min_data_in_leaf=20, max_depth=5)
mannequin.match(X_train, y_train)
predictions = mannequin.predict(X_test)
print(classification_report(y_test, predictions))
precision recall f1-score help
0 0.85 0.89 0.87 105
1 0.83 0.77 0.80 74
accuracy 0.84 179
macro avg 0.84 0.83 0.83 179
weighted avg 0.84 0.84 0.84 179
Notice that the precise tuning of hyperparameters is a course of that includes trial and error and may additionally be guided by expertise and a deeper understanding of the boosting algorithm and material experience (area data) of the enterprise drawback you are engaged on.
On this submit, you discovered concerning the LightGBM algorithm and its Python implementation. It’s a versatile method that’s helpful for numerous varieties of classification issues and must be part of your machine-learning toolkit.
Vidhi Chugh is an AI strategist and a digital transformation chief working on the intersection of product, sciences, and engineering to construct scalable machine studying techniques. She is an award-winning innovation chief, an writer, and a global speaker. She is on a mission to democratize machine studying and break the jargon for everybody to be part of this transformation.