Construct a multilingual computerized translation pipeline with Amazon Translate Lively Customized Translation
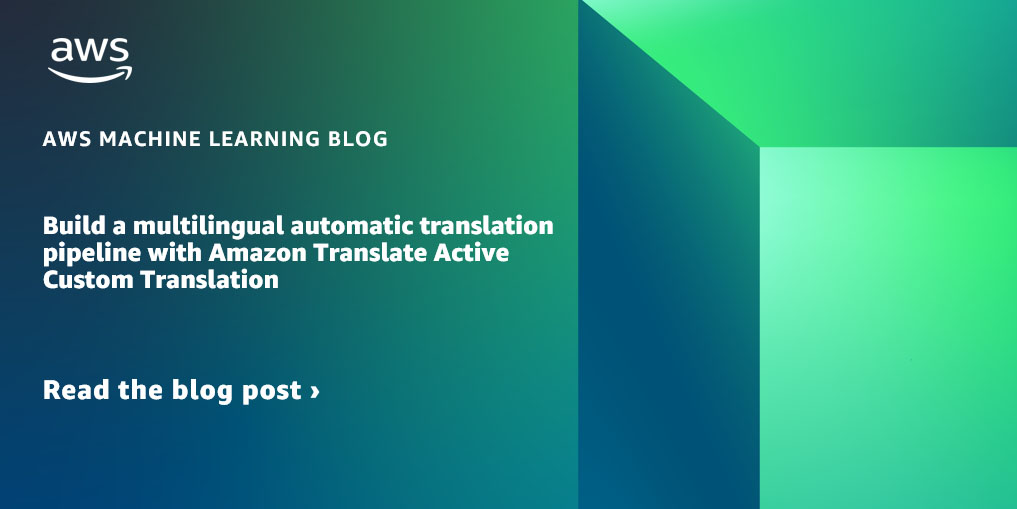
Dive into Deep Studying (D2L.ai) is an open-source textbook that makes deep studying accessible to everybody. It options interactive Jupyter notebooks with self-contained code in PyTorch, JAX, TensorFlow, and MXNet, in addition to real-world examples, exposition figures, and math. Thus far, D2L has been adopted by greater than 400 universities around the globe, such because the College of Cambridge, Stanford College, the Massachusetts Institute of Know-how, Carnegie Mellon College, and Tsinghua College. This work can also be made obtainable in Chinese language, Japanese, Korean, Portuguese, Turkish, and Vietnamese, with plans to launch Spanish and different languages.
It’s a difficult endeavor to have a web-based e book that’s constantly stored updated, written by a number of authors, and obtainable in a number of languages. On this publish, we current an answer that D2L.ai used to handle this problem through the use of the Active Custom Translation (ACT) feature of Amazon Translate and constructing a multilingual computerized translation pipeline.
We reveal easy methods to use the AWS Management Console and Amazon Translate public API to ship computerized machine batch translation, and analyze the translations between two language pairs: English and Chinese language, and English and Spanish. We additionally suggest finest practices when utilizing Amazon Translate on this computerized translation pipeline to make sure translation high quality and effectivity.
Resolution overview
We constructed computerized translation pipelines for a number of languages utilizing the ACT characteristic in Amazon Translate. ACT permits you to customise translation output on the fly by offering tailor-made translation examples within the type of parallel data. Parallel information consists of a group of textual examples in a supply language and the specified translations in a number of goal languages. Throughout translation, ACT mechanically selects essentially the most related segments from the parallel information and updates the interpretation mannequin on the fly primarily based on these phase pairs. This ends in translations that higher match the model and content material of the parallel information.
The structure accommodates a number of sub-pipelines; every sub-pipeline handles one language translation akin to English to Chinese language, English to Spanish, and so forth. A number of translation sub-pipelines may be processed in parallel. In every sub-pipeline, we first construct the parallel information in Amazon Translate utilizing the high-quality dataset of tailed translation examples from the human-translated D2L books. Then we generate the custom-made machine translation output on the fly at run time, which achieves higher high quality and accuracy.
Within the following sections, we reveal easy methods to construct every translation pipeline utilizing Amazon Translate with ACT, together with Amazon SageMaker and Amazon Simple Storage Service (Amazon S3).
First, we put the supply paperwork, reference paperwork, and parallel information coaching set in an S3 bucket. Then we construct Jupyter notebooks in SageMaker to run the interpretation course of utilizing Amazon Translate public APIs.
Stipulations
To comply with the steps on this publish, ensure you have an AWS account with the next:
- Entry to AWS Identity and Access Management (IAM) for position and coverage configuration
- Entry to Amazon Translate, SageMaker, and Amazon S3
- An S3 bucket to retailer the supply paperwork, reference paperwork, parallel information dataset, and output of translation
Create an IAM position and insurance policies for Amazon Translate with ACT
Our IAM position must include a customized belief coverage for Amazon Translate:
This position should even have a permissions coverage that grants Amazon Translate learn entry to the enter folder and subfolders in Amazon S3 that include the supply paperwork, and browse/write entry to the output S3 bucket and folder that accommodates the translated paperwork:
To run Jupyter notebooks in SageMaker for the interpretation jobs, we have to grant an inline permission coverage to the SageMaker execution position. This position passes the Amazon Translate service position to SageMaker that enables the SageMaker notebooks to have entry to the supply and translated paperwork within the designated S3 buckets:
Put together parallel information coaching samples
The parallel information in ACT must be skilled by an enter file consisting of an inventory of textual instance pairs, as an example, a pair of supply language (English) and goal language (Chinese language). The enter file may be in TMX, CSV, or TSV format. The next screenshot reveals an instance of a CSV enter file. The primary column is the supply language information (in English), and the second column is the goal language information (in Chinese language). The next instance is extracted from D2L-en e book and D2L-zh e book.
Carry out customized parallel information coaching in Amazon Translate
First, we arrange the S3 bucket and folders as proven within the following screenshot. The source_data
folder accommodates the supply paperwork earlier than the interpretation; the generated paperwork after the batch translation are put within the output folder. The ParallelData
folder holds the parallel information enter file ready within the earlier step.
After importing the enter recordsdata to the source_data
folder, we will use the CreateParallelData API to run a parallel information creation job in Amazon Translate:
To replace current parallel information with new coaching datasets, we will use the UpdateParallelData API:
S3_BUCKET = “YOUR-S3_BUCKET-NAME”
pd_name = “pd-d2l-short_test_sentence_enzh_all”
pd_description = “Parallel Information for English to Chinese language”
pd_fn = “d2l_short_test_sentence_enzh_all.csv”
response_t = translate_client.update_parallel_data(
Title=pd_name, # pd_name is the parallel information identify
Description=pd_description, # pd_description is the parallel information description
ParallelDataConfig={
'S3Uri': 's3://'+S3_BUCKET+'/Paralleldata/'+pd_fn, # S3_BUCKET is the S3 bucket identify outlined within the earlier step
'Format': 'CSV'
},
)
print(pd_name, ": ", response_t['Status'], " up to date.")
We will test the coaching job progress on the Amazon Translate console. When the job is full, the parallel information standing reveals as Lively and is able to use.
Run asynchronized batch translation utilizing parallel information
The batch translation may be carried out in a course of the place a number of supply paperwork are mechanically translated into paperwork in goal languages. The method includes importing the supply paperwork to the enter folder of the S3 bucket, then making use of the StartTextTranslationJob API of Amazon Translate to provoke an asynchronized translation job:
We chosen 5 supply paperwork in English from the D2L e book (D2L-en) for the majority translation. On the Amazon Translate console, we will monitor the interpretation job progress. When the job standing adjustments into Accomplished, we will discover the translated paperwork in Chinese language (D2L-zh) within the S3 bucket output folder.
Consider the interpretation high quality
To reveal the effectiveness of the ACT characteristic in Amazon Translate, we additionally utilized the standard methodology of Amazon Translate real-time translation with out parallel information to course of the identical paperwork, and in contrast the output with the batch translation output with ACT. We used the BLEU (BiLingual Analysis Understudy) rating to benchmark the interpretation high quality between the 2 strategies. The one strategy to precisely measure the standard of machine translation output is to have an knowledgeable assessment and grade the standard. Nonetheless, BLEU gives an estimate of relative high quality enchancment between two output. A BLEU rating is often a quantity between 0–1; it calculates the similarity of the machine translation to the reference human translation. The upper rating represents higher high quality in pure language understanding (NLU).
We’ve examined a set of paperwork in 4 pipelines: English into Chinese language (en to zh), Chinese language into English (zh to en), English into Spanish (en to es), and Spanish into English (es to en). The next determine reveals that the interpretation with ACT produced the next common BLEU rating in all the interpretation pipelines.
We additionally noticed that, the extra granular the parallel information pairs are, the higher the interpretation efficiency. For instance, we use the next parallel information enter file with pairs of paragraphs, which accommodates 10 entries.
For a similar content material, we use the next parallel information enter file with pairs of sentences and 16 entries.
We used each parallel information enter recordsdata to assemble two parallel information entities in Amazon Translate, then created two batch translation jobs with the identical supply doc. The next determine compares the output translations. It reveals that the output utilizing parallel information with pairs of sentences out-performed the one utilizing parallel information with pairs of paragraphs, for each English to Chinese language translation and Chinese language to English translation.
In case you are desirous about studying extra about these benchmark analyses, seek advice from Auto Machine Translation and Synchronization for “Dive into Deep Learning”.
Clear up
To keep away from recurring prices sooner or later, we suggest you clear up the sources you created:
- On the Amazon Translate console, choose the parallel information you created and select Delete. Alternatively, you should use the DeleteParallelData API or the AWS Command Line Interface (AWS CLI) delete-parallel-data command to delete the parallel information.
- Delete the S3 bucket used to host the supply and reference paperwork, translated paperwork, and parallel information enter recordsdata.
- Delete the IAM position and coverage. For directions, seek advice from Deleting roles or instance profiles and Deleting IAM policies.
Conclusion
With this resolution, we goal to scale back the workload of human translators by 80%, whereas sustaining the interpretation high quality and supporting a number of languages. You should utilize this resolution to enhance your translation high quality and effectivity. We’re engaged on additional bettering the answer structure and translation high quality for different languages.
Your suggestions is all the time welcome; please go away your ideas and questions within the feedback part.
Concerning the authors
Yunfei Bai is a Senior Options Architect at AWS. With a background in AI/ML, information science, and analytics, Yunfei helps prospects undertake AWS companies to ship enterprise outcomes. He designs AI/ML and information analytics options that overcome complicated technical challenges and drive strategic targets. Yunfei has a PhD in Digital and Electrical Engineering. Exterior of labor, Yunfei enjoys studying and music.
Rachel Hu is an utilized scientist at AWS Machine Studying College (MLU). She has been main just a few course designs, together with ML Operations (MLOps) and Accelerator Laptop Imaginative and prescient. Rachel is an AWS senior speaker and has spoken at prime conferences together with AWS re:Invent, NVIDIA GTC, KDD, and MLOps Summit. Earlier than becoming a member of AWS, Rachel labored as a machine studying engineer constructing pure language processing fashions. Exterior of labor, she enjoys yoga, final frisbee, studying, and touring.
Watson Srivathsan is the Principal Product Supervisor for Amazon Translate, AWS’s pure language processing service. On weekends, you’ll find him exploring the outside within the Pacific Northwest.