Carry out batch transforms with Amazon SageMaker Jumpstart Text2Text Era massive language fashions
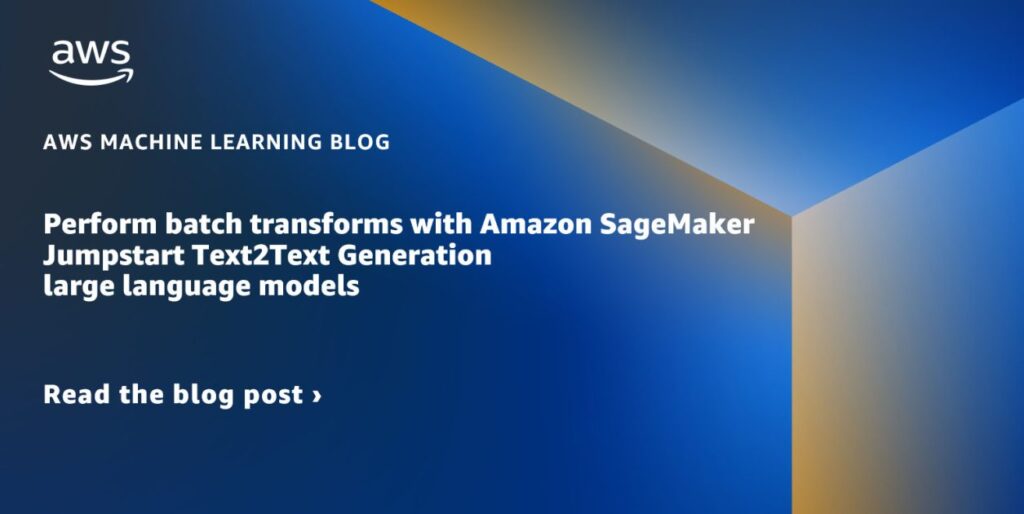
Right this moment we’re excited to announce you can now carry out batch transforms with Amazon SageMaker JumpStart massive language fashions (LLMs) for Text2Text Era. Batch transforms are helpful in conditions the place the responses don’t have to be actual time and due to this fact you are able to do inference in batch for big datasets in bulk. For batch rework, a batch job is run that takes batch enter as a dataset and a pre-trained mannequin, and outputs predictions for every information level within the dataset. Batch rework is cost-effective as a result of in contrast to real-time hosted endpoints which have persistent {hardware}, batch rework clusters are torn down when the job is full and due to this fact the {hardware} is just used throughout the batch job.
In some use instances, real-time inference requests will be grouped in small batches for batch processing to create real-time or near-real-time responses. For instance, if you must course of a steady stream of knowledge with low latency and excessive throughput, invoking a real-time endpoint for every request individually would require extra assets and might take longer to course of all of the requests as a result of the processing is being completed serially. A greater strategy can be to group a few of the requests and name the real-time endpoint in batch inference mode, which processes your requests in a single ahead go of the mannequin and returns the majority response for the request in actual time or near-real time. The latency of the response will rely on what number of requests you group collectively and occasion reminiscence dimension, due to this fact you possibly can tune the batch dimension per your small business necessities for latency and throughput. We name this real-time batch inference as a result of it combines the idea of batching whereas nonetheless offering real-time responses. With real-time batch inference, you possibly can obtain a stability between low latency and excessive throughput, enabling you to course of massive volumes of knowledge in a well timed and environment friendly method.
Jumpstart batch rework for Text2Text Era fashions permits you to go the batch hyperparameters by way of atmosphere variables that additional enhance throughput and decrease latency.
JumpStart offers pretrained, open-source fashions for a variety of downside varieties that will help you get began with machine studying (ML). You may incrementally practice and tune these fashions earlier than deployment. JumpStart additionally offers resolution templates that arrange infrastructure for widespread use instances, and executable instance notebooks for ML with Amazon SageMaker. You may entry the pre-trained fashions, resolution templates, and examples by way of the JumpStart touchdown web page in Amazon SageMaker Studio. You too can entry JumpStart fashions utilizing the SageMaker Python SDK.
On this publish, we reveal easy methods to use the state-of-the-art pre-trained text2text FLAN T5 models from Hugging Face for batch rework and real-time batch inference.
Resolution overview
The pocket book exhibiting batch rework of pre-trained Text2Text FLAN T5 fashions from Hugging Face in accessible within the following GitHub repository. This pocket book makes use of information from the Hugging Face cnn_dailymail dataset for a textual content summarization job utilizing the SageMaker SDK.
The next are the important thing steps for implementing batch rework and real-time batch inference:
- Arrange conditions.
- Choose a pre-trained mannequin.
- Retrieve artifacts for the mannequin.
- Specify batch rework job hyperparameters.
- Put together information for the batch rework.
- Run the batch rework job.
- Consider the summarization utilizing a ROUGE (Recall-Oriented Understudy for Gisting Analysis) rating.
- Carry out real-time batch inference.
Arrange conditions
Earlier than you run the pocket book, you could full some preliminary setup steps. Let’s arrange the SageMaker execution function so it has permissions to run AWS providers in your behalf:
Choose a pre-trained mannequin
We use the huggingface-text2text-flan-t5-large mannequin as a default mannequin. Optionally, you possibly can retrieve the listing of obtainable Text2Text fashions on JumpStart and select your most popular mannequin. This technique offers a simple solution to choose totally different mannequin IDs utilizing similar pocket book. For demonstration functions, we use the huggingface-text2text-flan-t5-large mannequin:
Retrieve artifacts for the mannequin
With SageMaker, we are able to carry out inference on the pre-trained mannequin, even with out fine-tuning it first on a brand new dataset. We begin by retrieving the deploy_image_uri
, deploy_source_uri
, and model_uri
for the pre-trained mannequin:
Specify batch rework job hyperparameters
It’s possible you’ll go any subset of hyperparameters as atmosphere variables to the batch rework job. You too can go these hyperparameters in a JSON payload. Nevertheless, in case you’re setting atmosphere variables for hyperparameters like the next code reveals, then the superior hyperparameters from the person examples within the JSON strains payload is not going to be used. If you wish to use hyperparameters from the payload, you might need to set the hyper_params_dict
parameter as null as a substitute.
Put together information for batch rework
Now we’re able to load the cnn_dailymail dataset from Hugging Face:
We go over every information entry and create the enter information within the required format. We create an articles.jsonl
file as a check information file containing articles that have to be summarized as enter payload. As we create this file, we append the immediate "Briefly summarize this textual content:"
to every check enter row. If you wish to have totally different hyperparameters for every check enter, you possibly can append these hyperparameters as a part of creating the dataset.
We create highlights.jsonl
as the bottom fact file containing highlights of every article saved within the check file articles.jsonl
. We retailer each check information in an Amazon Simple Storage Service (Amazon S3) bucket. See the next code:
Run the batch rework job
While you begin a batch rework job, SageMaker launches the required compute assets to course of the information, together with CPU or GPU situations relying on the chosen occasion sort. In the course of the batch rework job, SageMaker routinely provisions and manages the compute assets required to course of the information, together with situations, storage, and networking assets. When the batch rework job is full, the compute assets are routinely cleaned up by SageMaker. Which means that the situations and storage used in the course of the job are stopped and eliminated, liberating up assets and minimizing value. See the next code:
The next is one instance document from the articles.jsonl
check file. Notice that document on this file has an ID that matched with predict.jsonl
file data that reveals a summarized document as output from the Hugging Face Text2Text mannequin. Equally, the bottom fact file additionally has an identical ID for the information document. The matching ID throughout the check file, floor fact file, and output file permits linking enter data with output data for straightforward interpretation of the outcomes.
The next is the instance enter document offered for summarization:
The next is the expected output with summarization:
The next is the bottom fact summarization for mannequin analysis functions:
Subsequent, we use the bottom fact and predicted outputs for mannequin analysis.
Consider the mannequin utilizing a ROUGE rating¶
ROUGE, or Recall-Oriented Understudy for Gisting Analysis, is a set of metrics and a software program bundle used for evaluating computerized summarization and machine translation in pure language processing. The metrics examine an routinely produced abstract or translation in opposition to a reference (human-produced) abstract or translation or a set of references.
Within the following code, we mix the expected and unique summaries by becoming a member of them on the widespread key id
and use this to compute the ROUGE rating:
Carry out real-time batch inference
Subsequent, we present you easy methods to run real-time batch inference on the endpoint by offering the inputs as an inventory. We use the identical mannequin ID and dataset as earlier, besides we take a couple of data from the check dataset and use them to invoke a real-time endpoint.
The next code reveals easy methods to create and deploy a real-time endpoint for real-time batch inference:
Subsequent, we put together our enter payload. For this, we use the information that we ready earlier and extract the primary 10 check inputs and append the textual content inputs with hyperparameters that we need to use. We offer this payload to the real-time invoke_endpoint
. The response payload is then returned as an inventory of responses. See the next code:
Clear up
After you’ve got examined the endpoint, ensure you delete the SageMaker inference endpoint and delete the mannequin to keep away from incurring expenses.
Conclusion
On this pocket book, we carried out a batch rework to showcase the Hugging Face Text2Text Generator mannequin for summarization duties. Batch rework is advantageous in acquiring inferences from massive datasets with out requiring a persistent endpoint. We linked enter data with inferences to help in consequence interpretation. We used the ROUGE rating to match the check information summarization with the model-generated summarization.
Moreover, we demonstrated real-time batch inference, the place you possibly can ship a small batch of knowledge to a real-time endpoint to attain a stability between latency and throughput for eventualities like streaming enter information. Actual-time batch inference helps enhance throughput for real-time requests.
Check out the batch rework with Text2Text Era fashions in SageMaker at this time and tell us your suggestions!
Concerning the authors
Hemant Singh is a Machine Studying Engineer with expertise in Amazon SageMaker JumpStart and Amazon SageMaker built-in algorithms. He obtained his masters from Courant Institute of Mathematical Sciences and B.Tech from IIT Delhi. He has expertise in engaged on a various vary of machine studying issues inside the area of pure language processing, laptop imaginative and prescient, and time collection evaluation.
Rachna Chadha is a Principal Options Architect AI/ML in Strategic Accounts at AWS. Rachna is an optimist who believes that the moral and accountable use of AI can enhance society in future and produce financial and social prosperity. In her spare time, Rachna likes spending time together with her household, mountain climbing, and listening to music.
Dr. Ashish Khetan is a Senior Utilized Scientist with Amazon SageMaker built-in algorithms and helps develop machine studying algorithms. He obtained his PhD from College of Illinois Urbana-Champaign. He’s an energetic researcher in machine studying and statistical inference, and has printed many papers in NeurIPS, ICML, ICLR, JMLR, ACL, and EMNLP conferences.