Exposing sklearn machine studying fashions in Energy BI | by Mark Graus | Could, 2023
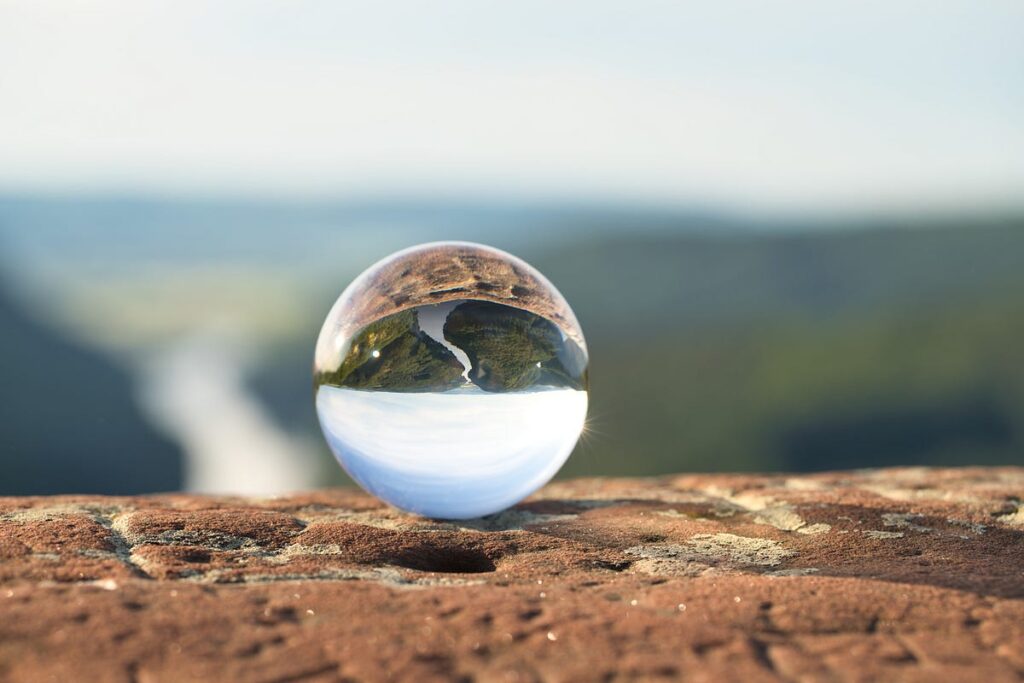
In some instances we wish to have a supervised studying mannequin to mess around with. Whereas any information scientist can fairly simply construct an SKLearn mannequin and mess around with it in a Jupyter pocket book, once you wish to produce other stakeholders work together together with your mannequin you’ll have to create a little bit of a front-end. This may be achieved in a easy Flask webapp, offering an internet interface for folks to feed information into an sklearn mannequin or pipeline to see the anticipated output. This put up nevertheless will concentrate on find out how to use Python visuals in Energy BI to work together with a mannequin.
The put up will include two fundamental elements:
- Constructing the SKLearn Mannequin / Constructing a Pipeline
- Constructing the Energy BI Interface
The code is actually simple and you may copypaste no matter you want from this put up, however it’s also accessible on my Github. To make use of it, you must do two issues. Run the code within the Python Pocket book to serialize the pipeline and alter the trail to that pipeline within the Energy BI file.
For this instance we’ll use the Titanic dataset and construct a easy predictive mannequin. The mannequin might be a classification mannequin, utilizing one categorical (‘intercourse’) and one numeric function (‘age’) as predictors. To exhibit the method we are going to use the RandomForestClassifier because the classification mannequin. It’s because a Random Forest Classifier is a bit more durable to implement in Energy BI than for instance a logistic regression that might be coded in MQuery or DAX. As well as, since this put up isn’t geared toward actually constructing the very best mannequin, I’m counting on parts of the scikit-learn documentation fairly a bit and I cannot be taking a look at efficiency that a lot.
The code we create does a few issues. To start with, it masses and preprocesses the Titanic dataset. As talked about earlier than, we’re solely utilizing the ‘intercourse’ and the ‘age’ options, however these nonetheless must be processed. The specific variable ‘intercourse’ needs to be remodeled into Dummy Variables or needs to be One Sizzling Encoded (i.e. the one column needs to be recoded right into a set of columns) for any sklearn mannequin to have the ability to deal with it. For the numerical function ‘age’ we do a regular MinMaxScaling, because it goes from about 0 to 80, whereas ‘intercourse’ goes from 0 to 1. As soon as all of that’s achieved, we drop all observations with lacking values, do a Practice/Take a look at break up and construct and serialize the pipeline.
#Importsfrom sklearn.datasets import fetch_openml
from sklearn.pipeline import Pipeline
from sklearn.compose import ColumnTransformer
from sklearn.preprocessing import OneHotEncoder
#Load the dataset
X,y = fetch_openml("titanic", model = 1, as_frame=True, return_X_y=True)
#Create the OneHotEncoding for the specific variable 'intercourse'
categorical_feature = ["sex"]
categorical_transformer = Pipeline(
steps = [
("encoder",OneHotEncoder(drop="first"))
])
preprocessor = ColumnTransformer(
transformers = [
("categorical", categorical_transformer, categorical_feature)
])
#Creating the Pipeline, with preprocessing and the Random Forest Classifier
clf = Pipeline(
steps = [
("preprocessor", preprocessor),
("classifier", RandomForestClassifier())
]
)
#Choose solely age and intercourse as predictors
X = X[["age","sex"]]
#Drop rows with lacking values
X = X.dropna()
#Preserve solely observations equivalent to rows with out lacking values
y = y[X.index]
#Create Practice/Take a look at Cut up
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state=1)
#Match the Pipeline
clf.match(X_train, y_train)
#Rating the Pipeline
y_pred = clf.predict(X_test)
print(classification_report(y_test, y_pred))
The code above creates a mannequin that scores probably not good, however ok for the aim of this put up.
precision recall f1-score assist0 0.85 0.84 0.85 159
1 0.76 0.77 0.76 103
accuracy 0.81 262
macro avg 0.80 0.80 0.80 262
weighted avg 0.81 0.81 0.81 262
What’s going to assist us later, is to test how the mannequin predicts. To try this we create a DataFrame with the Cartesian product age and intercourse (i.e. all attainable ‘age’/’intercourse’ combos). We use that DataFrame to calculate predictions from the pipeline and we subsequently plot these predictions as a heatmap. The code to do this seems to be as follows.
from pandas import DataFrame# Create a DataFrame with all attainable ages
ages = DataFrame({'age':vary(1,80,1)})
# Create a DataFrame with all attainable sexes
sexes = DataFrame({'intercourse':["male","female"]})
# Create a DataFrame with all attainable combos.
combos = ages.merge(sexes, how='cross')
# Predict survival for combos
combiations["predicted_survival"] = clf.predict(combos)
# Plot the Heatmap
sns.heatmap(pd.pivot_table(outcomes, values="predicted_survival", index=["age"],columns=["sex"]), annot=True)
The corresponding heatmap seems to be as follows and reveals that for instance for females from 13–33 years previous, the prediction is survival (1). Whereas a feminine aged precisely 37 is predicted to not survive. For males, the predictions are largely no survival, apart from age 12 and a few youthful ages. This info might be helpful when debugging the Energy BI report.
Now that that is achieved, we will serialize the mannequin to begin embedding it right into a Energy BI report.
from joblib import dump
dump(clf, "randomforest.joblib")
Creating the Energy BI Interface consists of two steps. Step one is that of making the controls to feed information into the mannequin. The second is that of making the visualization that takes the inputs from the controls, feeds it into the mannequin and reveals the prediction.
2a. Controls
A few ideas are essential to concentrate on when utilizing Energy BI. To start with, there are Parameters, or variables that comprise values in Energy BI. These Parameters will be managed via slicers and the values they comprise will be accessed via visualization parts in Energy BI, which in our case might be a Python visualization.