Lists, Tuples, Dictionaries, And Knowledge Frames in Python: The Full Information | by Federico Trotta | Could, 2023
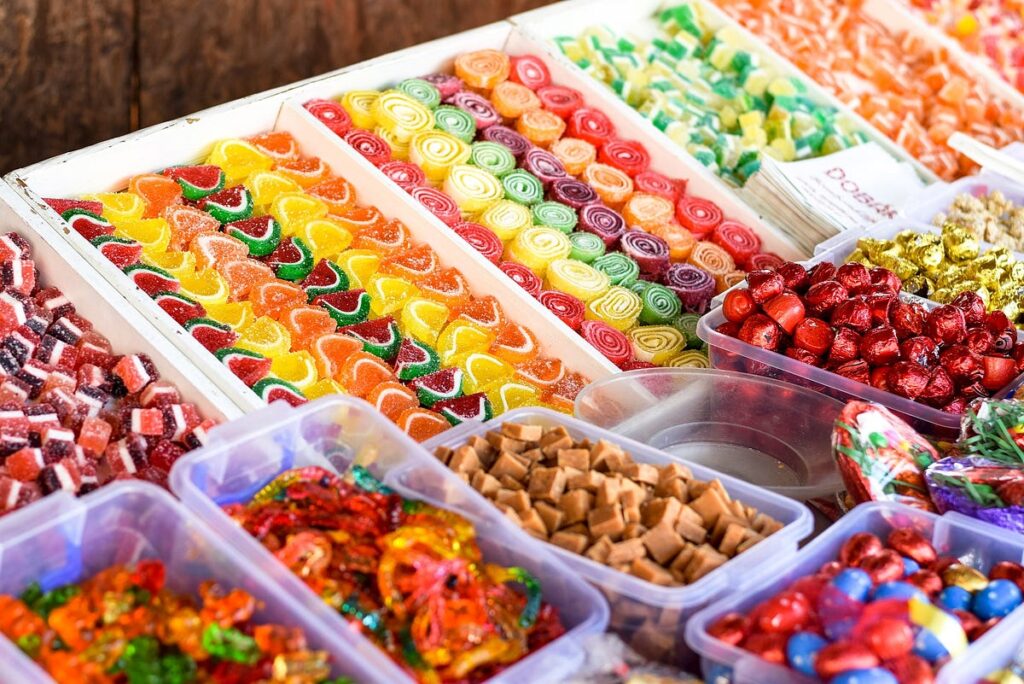
Definition and creation examples
In Python, an inventory is a group of ordered components that may be of any sort: strings, integers, floats, and so forth…
To create an inventory, the objects have to be inserted between sq. brackets and separated by a comma. For instance, right here’s how we are able to create an inventory of integers:
# Create record of integers
my_integers = [1, 2, 3, 4, 5, 6]
However lists may have “blended” sorts saved inside them. For instance, let’s create an inventory with each integers and strings:
# Create a blended record
mixed_list = [1, 3, "dad", 101, "apple"]
To create an inventory, we are able to additionally use the Python built-in operate record()
. That is how we are able to use it:
# Create record and print it
my_list = record((1, 2, 3, 4, 5))
print(my_list)>>>
[1, 2, 3, 4, 5]
This built-in operate may be very helpful in some specific instances. For instance, let’s say we wish to create an inventory of numbers within the vary (1–10). Right here’s how we are able to accomplish that:
# Create an inventory in a variety
my_list = record(vary(1, 10))
print(my_list)>>>
[1, 2, 3, 4, 5, 6, 7, 8, 9]
NOTE:Keep in mind that the built-in operate "vary" contains the primary worth,
and excludes the final one.
Now, let’s see how we are able to manipulate lists.
Lists manipulation
Due to the truth that lists are mutable, we’ve a number of potentialities to govern them. For instance, let’s say we’ve an inventory of names, however we made a mistake and we wish to change one. Right here’s how we are able to accomplish that:
# Checklist of names
names = ["James", "Richard", "Simon", "Elizabeth", "Tricia"]
# Change the incorrect title
names[0] = "Alexander"
# Print record
print(names)>>>
['Alexander', 'Richard', 'Simon', 'Elizabeth', 'Tricia']
So, within the above instance, we’ve modified the primary title of the record from James to Alexander.
NOTE:
In case you did not know, be aware that in Python the primary factor
is at all times accessed by "0", concerning of the kind we're manipulating.
So, within the above instance, "names[0]" represents the primary factor
of the record "names".
Now, suppose we’ve forgotten a reputation. We will add it to our record like so:
# Checklist of names
names = ["James", "Richard", "Simon", "Elizabeth", "Tricia"]
# Append one other title
names.append("Alexander")
# Print record
print(names) >>>
['James', 'Richard', 'Simon', 'Elizabeth', 'Tricia', 'Alexander']
If we have to concatenate two lists, we’ve two potentialities: the concatenate
methodology or the prolong()
one. Let’s see them:
# Create list1
list1 = [1, 2, 3]
# Create list2
list2 = [4, 5, 6]
# Concatenate lists
concatenated_list = list1 + list2
# Print concatenated record
print(concatenated_list)>>>
[1, 2, 3, 4, 5, 6]
So, this methodology creates an inventory that’s the sum of different lists. Let’s see the prolong()
methodology:
# Create list1
list1 = [1, 2, 3]
# Create list2
list2 = [4, 5, 6]
# Prolong list1 with list2
list1.prolong(list2)
# Print new list1
print(list1)>>>
[1, 2, 3, 4, 5, 6]
As we are able to see, the outcomes are the identical, however the syntax is completely different. This methodology extends list1
with list2
.
If we wish to take away components, we’ve two potentialities: we are able to use the take away()
methodology or del
. Let’s see them:
# Create record
my_list = [1, 2, 3, 'four', 5.0]
# Take away one factor and print
my_list.take away('4')
print(my_list)>>>
[1, 2, 3, 5.0]
Let’s see the opposite methodology:
# Create record
my_list = [1, 2, 3, 'four', 5.0]
# Delete one factor and print
del my_list[3]
print(my_list)>>>
[1, 2, 3, 5.0]
So, we get the identical outcomes with each strategies, however take away()
provides us the chance to explicitly write the factor to take away, whereas del
must entry the place of the factor of the record.
NOTE:
In case you've gained familiarity with accessing positions, within the above
instance my_list[3] = '4'. As a result of, keep in mind: in Python we begin counting
positions from 0.
Checklist comprehension
There are plenty of instances the place we have to create lists ranging from current lists, typically making use of some filters to the prevailing knowledge. To take action, we’ve two potentialities:
- We use loops and statements.
- We use record comprehension.
Virtually, they’re each the identical strategy to write the identical factor, however record comprehension is extra concise and stylish.
However earlier than we talk about these strategies, you could want a deep overview of loops and statements. Listed below are a few articles I wrote previously that will provide help to:
Now, let’s see a few examples utilizing loops and statements immediately.
Suppose we’ve a procuring record. We wish our program to print that we love one fruit and that we don’t just like the others on the record. Right here’s how we are able to accomplish that:
# Create procuring record
shopping_list = ["banana", "apple", "orange", "lemon"]
# Print the one I like
for fruit in shopping_list:
if fruit == "lemon":
print(f"I like {fruit}")
else:
print(f"I do not like {fruit}")>>>
I do not like banana
I do not like apple
I do not like orange
I like lemon
One other instance might be the next. Suppose we’ve an inventory of numbers and we wish to print simply the even ones. Right here’s how we are able to accomplish that:
# Create record
numbers = [1,2,3,4,5,6,7,8]
# Create empty record
even_list = []
# Print even numbers
for even in numbers:
if even %2 == 0:
even_list.append(even)
else:
crossprint(even_list)
>>>
[2, 4, 6, 8]
NOTE:If you're not acquainted with the sintax %2 == 0 it signifies that we're
dividing a quantity by 2 and count on a reminder of 0. In different phrases,
we're asking our program to intercept the even numbers.
So, within the above instance, we’ve created an inventory of numbers. Then, we’ve created an empty record that’s used after the loop to append all of the even numbers. This manner, we’ve created an inventory of even numbers from an inventory with “basic” numbers.
Now… this fashion of making new lists with loops and statements is somewhat “heavy”. I imply: it requires plenty of code. We will achieve the identical ends in a extra concise method utilizing record comprehension.
For instance, to create an inventory with even numbers we are able to use record comprehension like so:
# Create record
numbers = [1,2,3,4,5,6,7,8]
# Create record of even numbers
even_numbers = [even for even in numbers if even %2 == 0]
# Print even record
print(even_numbers)>>>
[2, 4, 6, 8]
So, record comprehension creates immediately a brand new record and we outline the situation inside it. As we are able to see, we achieve the identical end result as earlier than, however in only one line of code: not unhealthy!
Now, let’s create an inventory with feedback on the fruit I like (and the fruit I don’t) with record comprehension:
# Create transport record
shopping_list = ["banana", "apple", "orange", "lemon"]
# Create commented record and print it
commented_list = [f"I love {fruit}" if fruit == "banana"
else f"I don't like {fruit}"
for fruit in shopping_list]
print(commented_list)>>>
['I love banana', "I don't like apple", "I don't like orange",
"I don't like lemon"]
So, we gained the identical end result as earlier than, however with only a line of code. The one distinction is that right here we’ve printed an inventory (as a result of record comprehension creates one!), whereas earlier than we simply printed the outcomes.
Checklist of lists
There may be additionally the chance to create lists of lists, which are lists nested into one record. This risk is helpful after we wish to signify listed knowledge as a singular record.
For instance, contemplate we wish to create an inventory of scholars and their grades. We might create one thing like that:
# Create lis with college students and their grades
college students = [
["John", [85, 92, 78, 90]],
["Emily", [77, 80, 85, 88]],
["Michael", [90, 92, 88, 94]],
["Sophia", [85, 90, 92, 87]]
]
It is a helpful notation if, for instance, we wish to calculate the imply grade for every scholar. We will do it like so:
# Iterate over the record
for scholar in college students:
title = scholar[0] # Entry names
grades = scholar[1] # Entry grades
average_grade = sum(grades) / len(grades) # Calculate imply grades
print(f"{title}'s common grade is {average_grade:.2f}")>>>
John's common grade is 86.25
Emily's common grade is 82.50
Michael's common grade is 91.00
Sophia's common grade is 88.50
Tuples are one other knowledge construction sort in Python. They’re outlined with spherical brackets and, as lists, can comprise any knowledge sort separated by a comma. So, for instance, we are able to outline a tuple like so:
# Outline a tuple and print it
my_tuple = (1, 3.0, "John")
print(my_tuple)>>>
(1, 3.0, 'John')
The distinction between a tuple and an inventory is {that a} tuple is immutable. Because of this the weather of a tuple cannot be modified. So, for instance, if we attempt to append a price to a tuple we get an error:
# Create a tuple with names
names = ("James", "Jhon", "Elizabeth")
# Attempt to append a reputation
names.append("Liza")>>>
AttributeError: 'tuple' object has no attribute 'append'
So, since we are able to’t modify tuples, they’re helpful after we need our knowledge to be immutable; for instance, in conditions the place we don’t wish to make errors.
A sensible instance will be the cart of an e-commerce. We might want this type of knowledge to be immutable in order that we don’t make any errors when manipulating it. Think about somebody purchased a shirt, a pair of footwear, and a watch from our e-commerce. We might report this knowledge with amount and worth into one tuple:
# Create a chart as a tuple
cart = (
("Shirt", 2, 19.99),
("Sneakers", 1, 59.99),
("Watch", 1, 99.99)
)
In fact, to be exact, it is a tuple of tuples.
Since lists are immutable, they’re extra environment friendly when it comes to efficiency, that means they save our pc’s assets. However on the subject of manipulation, we are able to use the very same code as we’ve seen for lists, so we received’t write it once more.
Lastly, equally to lists, we are able to create a tuple with the built-in operate tuple()
like so:
# Create a tuple in a variety
my_tuple = tuple(vary(1, 10))
print(my_tuple)>>>
(1, 2, 3, 4, 5, 6, 7, 8, 9)
A dictionary is a strategy to retailer knowledge which are coupled as keys and values. That is how we are able to create one:
# Create a dictionary
my_dictionary = {'key_1':'value_1', 'key_2':'value_2'}
So, we create a dictionary with curly brackets and we retailer in it a few keys and values separated by a colon. The {couples} keys-values are then separated by a comma.
Now, let’s see how we are able to manipulate dictionaries.
Dictionaries manipulation
Each keys and values of a dictionary may be of any sort: strings, integers, or floats. So, for instance, we are able to create a dictionary like so:
# Create a dictionary of numbers and print it
numbers = {1:'one', 2:'two', 3:'three'}
print(numbers)>>>
{1: 'one', 2: 'two', 3: 'three'}
However we are able to create one additionally like that:
# Create a dictionary of numbers and print it
numbers = {'one':1, 'two':2.0, 3:'three'}
print(numbers)>>>
{'one': 1, 'two': 2.0, 3: 'three'}
Selecting the kind for values and keys is determined by the issue we have to resolve. Anyway, contemplating the dictionary we’ve seen earlier than, we are able to entry each values and keys like so:
# Entry values and keys
keys = record(numbers.keys())
values = tuple(numbers.values())
# Print values and keys
print(f"The keys are: {keys}")
print(f"The values are: {values}")>>>
The keys are: ['one', 'two', 3]
The values are: (1, 2.0, 'three')
So, if our dictionary is known as numbers
we entry its key with numbers.keys()
. And with numbers.values()
we entry its values. Additionally, be aware that we’ve created an inventory with the keys and a tuple with the values utilizing the notation we’ve seen earlier than.
In fact, we are able to additionally iterate over dictionaries. For instance, suppose we wish to print the values which are higher than a sure threshold:
# Create a procuring record with fruits and costs
shopping_list = {'banana':2, 'apple':1, 'orange':1.5}
# Iterate over the values
for values in shopping_list.values():
# Values higher than threshold
if values > 1:
print(values)>>>
2
1.5
Like lists, dictionaries are mutable. So, if we wish to add a price to a dictionary we’ve to outline the important thing and the worth so as to add to it. We will do it like so:
# Create the dictionary
individual = {'title': 'John', 'age': 30}
# Add worth and key and print
individual['city'] = 'New York'
print(individual)>>>
{'title': 'John', 'age': 30, 'metropolis': 'New York'}
To change a price of a dictionary, we have to entry its key:
# Create a dictionary
individual = {'title': 'John', 'age': 30}
# Change age worth and print
individual['age'] = 35
print(individual)>>>
{'title': 'John', 'age': 35}
To delete a pair key-value from a dictionary, we have to entry its key:
# Create dictionary
individual = {'title': 'John', 'age': 30}
# Delete age and print
del individual['age']
print(individual)>>>
{'title': 'John'}
Nested dictionaries
We’ve got seen earlier than that we are able to create lists of lists and tuples of tuples. Equally, we are able to create nested dictionaries. Suppose, for instance, we wish to create a dictionary to retailer the information associated to a category of scholars. We will do it like so:
# Create a classroom dictionary
classroom = {
'student_1': {
'title': 'Alice',
'age': 15,
'grades': [90, 85, 92]
},
'student_2': {
'title': 'Bob',
'age': 16,
'grades': [80, 75, 88]
},
'student_3': {
'title': 'Charlie',
'age': 14,
'grades': [95, 92, 98]
}
So, the information of every scholar are represented as a dictionary and all of the dictionaries are saved in a singular dictionary, representing the classroom. As we are able to see, the values of a dictionary may even be lists (or tuples, if we’d like). On this case, we’ve used lists to retailer the grades of every scholar.
To print the values of 1 scholar, we simply must do not forget that, from the attitude of the classroom dictionary, we have to entry the important thing and, on this case, the keys are the scholars themselves. This implies we are able to do it like so:
# Entry student_3 and print
student_3 = classroom['student_3']
print(student_3)>>>
{'title': 'Charlie', 'age': 14, 'grades': [95, 92, 98]}
Dictionaries comprehension
Dictionary comprehension permits us to create dictionaries concisely and effectively. It’s just like record comprehension however, as a substitute of making an inventory, it creates a dictionary.
Suppose we’ve a dictionary the place we’ve saved some objects and their costs. We wish to know the objects that price lower than a sure threshold. We will do it like so:
# Outline preliminary dictionary
merchandise = {'footwear': 100, 'watch': 50, 'smartphone': 250, 'pill': 120}
# Outline threshold
max_price = 150
# Filter for threshold
products_to_buy = {fruit: worth for fruit, worth in merchandise.objects() if worth <= max_price}
# Print filtered dictionary
print(products_to_buy)>>>
{'footwear': 100, 'watch': 50, 'pill': 120}
So, the syntax to make use of dictionary comprehension is:
new_dict = {key:worth for key, worth in iterable}
The place iterable is any iterable Python object. It may be an inventory, a tuple, one other dictionary, and so forth…
Creating dictionaries with the “commonplace” methodology would require plenty of code, with situations, loops, and statements. As a substitute, as we are able to see, dictionary comprehension permits us to create a dictionary, primarily based on situations, with only one line of code.
Dictionary comprehension is particularly helpful when we have to create a dictionary retrieving knowledge from different sources or knowledge buildings. For instance, say we have to create a dictionary retrieving values from two lists. We will do it like so:
# Outline names and ages in lists
names = ['John', 'Jane', 'Bob', 'Alice']
cities = ['New York', 'Boston', 'London', 'Rome']
# Create dictionary from lists and print outcomes
name_age_dict = {title: metropolis for title, metropolis in zip(names, cities)}
print(name_age_dict)>>>
{'John': 'New York', 'Jane': 'Boston', 'Bob': 'London', 'Alice': 'Rome'}
A knowledge body is a two-dimensional knowledge construction consisting of columns and rows. So, it’s in some way just like a spreadsheet or a desk in an SQL database. They’ve the next traits:
- Every row represents a person remark or report.
- Every column represents a variable or a particular attribute of the information.
- They’ve labeled rows (known as indexes) and columns, making it simple to govern the information.
- The columns can comprise various kinds of knowledge, like integers, strings, or floats. Even a single column can comprise completely different knowledge sorts.
Whereas knowledge frames are the everyday knowledge construction used within the context of Knowledge Evaluation and Knowledge Science, it’s not unusual {that a} Python Software program Engineer might have to govern an information body, and this is the reason we’re having an summary of information frames.
Right here’s how an information body seems:
So, on the left (within the blue rectangle) we are able to see the indexes, that means the row counts. We will then see {that a} knowledge body can comprise various kinds of knowledge. Particularly, the column “Age” accommodates completely different knowledge sorts (one string and two integers).
Primary knowledge frames manipulation with Pandas
Whereas just lately a brand new library to govern knowledge frames known as “Polars” began circulating, right here we’ll see some knowledge manipulation with Pandas which remains to be probably the most used as of at this time.
Initially, typically, we are able to create knowledge frames by importing knowledge from .xlsx
or .cvs
information. In Pandas we are able to do it like so:
import pandas as pd# Import cvs file
my_dataframe = pd.read_csv('a_file.csv')
# Import xlsx
my_dataframe_2 = pd.read_excel('a_file_2.xlsx')
If we wish to create an information body:
import pandas as pd# Create a dictionary with various kinds of knowledge
knowledge = {
'Identify': ['John', 'Alice', 'Bob'],
'Age': ['twenty-five', 30, 27],
'Metropolis': ['New York', 'London', 'Sydney'],
'Wage': [50000, 60000.50, 45000.75],
'Is_Employed': [True, True, False]
}
# Create the dataframe
df = pd.DataFrame(knowledge)
That is the information body we’ve proven above. So, as we are able to see, we first create a dictionary, after which we convert it to an information body with the strategy pd.DataFrame()
.
We’ve got three potentialities to visualise an information body. Suppose we’ve an information body known as df
:
- The primary one is
print(df)
. - The second is
df.head()
that may present the primary 5 rows of our knowledge body. In case we’ve an information body with plenty of rows, we are able to present greater than the primary 5. For instance,df.head(20)
reveals the primary 20. - The third one is
df.tail()
that works precisely likehead()
, however this reveals the final rows.
On the aspect of visualization, utilizing the above df
, that is what df.head()
reveals:
And that is what print(df)
reveals:
Within the case of small knowledge units like this one, the distinction is simply a matter of style (I choose head()
as a result of it “reveals the tabularity” of information). However within the case of huge knowledge units, head()
is method significantly better. Strive it, and let me know!
Contemplate that Pandas is a really vast library, that means it permits us to govern tabular knowledge in a wide range of methods, so it’d have to be handled alone. Right here we wish to present simply the very fundamentals, so we’ll see how we are able to add and delete a column (the columns of an information body are additionally known as “Pandas collection”).
Suppose we wish to add a column to the information body df
we’ve seen above that’s telling us if persons are married or not. We will do it like so:
# Add marital standing
df["married"] = ["yes", "yes", "no"]
NOTE:this is similar notation we used so as to add values to a dictionary.
Return again on the article and examine the 2 strategies.
And exhibiting the top we’ve:
To delete one column:
# Delete the "Is_Employed" column
df = df.drop('Is_Employed', axis=1)
And we get:
Be aware that we have to use axis=1
as a result of right here we’re telling Pandas to take away columns and since an information body is a two-dimensional knowledge construction, axis=1
represents the vertical route.
As a substitute, if we wish to drop a row, we have to use axis=0
. For instance, suppose we wish to delete the row related to the index 1 ( that’s the second row as a result of, once more, we begin counting from 0):
# Delete the second row
df = df.drop(1, axis=0)
And we get:
To date, we’ve seen probably the most used knowledge buildings in Python. These will not be the one ones, however absolutely probably the most used.
Additionally, there is no such thing as a proper or incorrect in utilizing one reasonably than one other: we simply want to grasp what knowledge we have to retailer and use the most effective knowledge construction for this kind of process.
I hope this text helped you perceive the utilization of those knowledge buildings and when to make use of them.