Whisper JAX vs PyTorch: Uncovering the Reality about ASR Efficiency on GPUs | by Luís Roque | Apr, 2023
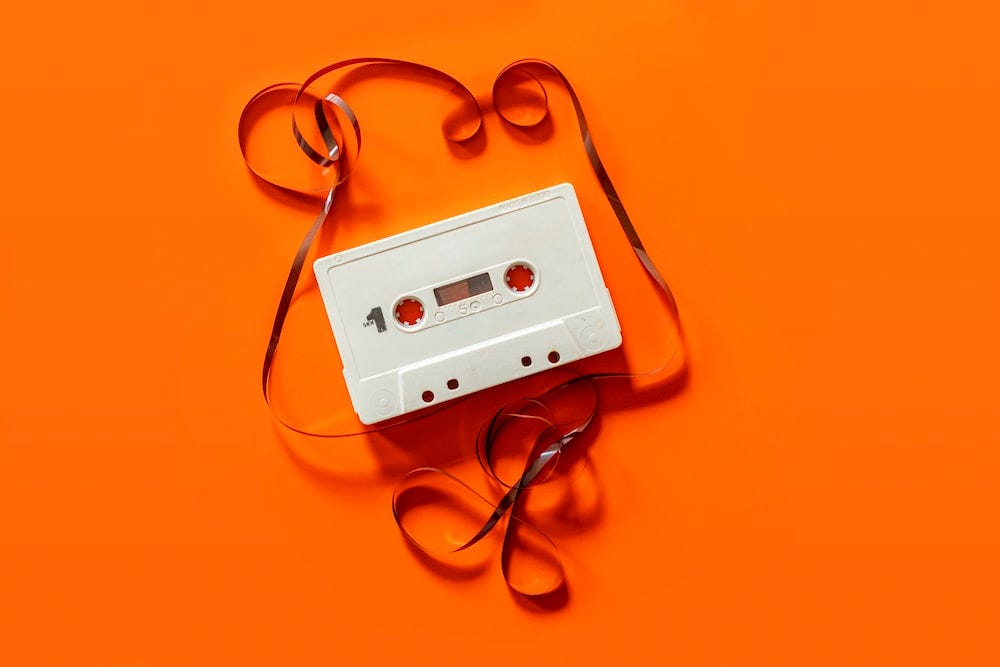
Deep Dive into Computerized Speech Recognition: Benchmarking Whisper JAX and PyTorch Implementations Throughout Platforms
On the earth of Computerized Speech Recognition (ASR), velocity and accuracy are of nice significance. The dimensions of the information and fashions has been rising considerably just lately, making it arduous to be environment friendly. Nonetheless, the race is simply beginning, and we see new developments each week. On this article, we concentrate on Whisper JAX, a latest implementation of Whisper utilizing a special backend framework that appears to run 70 occasions quicker than OpenAI’s PyTorch implementation. We examined each CPU and GPU implementations and measured accuracy and execution time. Additionally, we outlined experiments for small and large-size fashions whereas parametrizing batch measurement and information varieties to see if we may enhance it additional.
As we noticed in our previous article, Whisper is a flexible speech recognition mannequin that excels in a number of speech-processing duties. It might probably carry out multilingual speech recognition, translation, and even voice exercise detection. It makes use of a Transformer sequence-to-sequence structure to foretell phrases and duties collectively. Whisper works as a meta-model for speech-processing duties. One of many downsides of Whisper is its effectivity; it’s typically discovered to be pretty gradual in comparison with different state-of-the-art fashions.
Within the following sections, we undergo the small print of what modified with this new strategy. We examine Whisper and Whisper JAX, spotlight the primary variations between PyTorch and JAX, and develop a pipeline to judge the velocity and accuracy between each implementations.
This text belongs to “Massive Language Fashions Chronicles: Navigating the NLP Frontier”, a brand new weekly collection of articles that may discover find out how to leverage the facility of huge fashions for varied NLP duties. By diving into these cutting-edge applied sciences, we purpose to empower builders, researchers, and fans to harness the potential of NLP and unlock new prospects.
Articles revealed thus far:
- Summarizing the latest Spotify releases with ChatGPT
- Master Semantic Search at Scale: Index Millions of Documents with Lightning-Fast Inference Times using FAISS and Sentence Transformers
- Unlock the Power of Audio Data: Advanced Transcription and Diarization with Whisper, WhisperX, and PyAnnotate
As all the time, the code is obtainable on my Github.
The Machine Studying neighborhood extensively makes use of highly effective libraries like PyTorch and JAX. Whereas they share some similarities, their internal works are fairly completely different. Let’s perceive the primary variations.
The AI Analysis Lab at Meta developed PyTorch and actively maintains it as we speak. It’s an open-source library based mostly on the Torch library. Researchers broadly use PyTorch as a result of its dynamic computation graph, intuitive interface, and stable debugging capabilities. The truth that it makes use of dynamic graphs provides it better flexibility in constructing new fashions and simplifying the modification of such fashions throughout runtime. It’s nearer to Python and particularly to the NumPy API. The principle distinction is that we aren’t working with arrays however with tensors, which might run on GPU, and helps auto differentiation.
JAX is a high-performance library developed by Google. Conversely to PyTorch, JAX combines the advantages of static and dynamic computation graphs. It does this via its just-in-time compilation characteristic, which supplies flexibility and efficiency. We will consider JAX being a stack of interpreters that progressively rewrite your program. It will definitely offloads the precise computation to XLA — the Accelerated Linear Algebra compiler, additionally designed and developed by Google, to speed up Machine Studying computations.
Let’s begin by constructing a category to deal with audio transcriptions utilizing Whisper with PyTorch (OpenAI’s implementation) or Whisper with JAX. Our class is a wrapper for the fashions and an interface to simply arrange experiments. We need to carry out a number of experiments, together with specifying the gadget, mannequin sort, and extra hyperparameters for Whisper JAX. Be aware that we used a singleton sample to make sure that as we run a number of experiences, we don’t find yourself with a number of situations of the mannequin consuming our reminiscence.
class Transcription:
"""
A category to deal with audio transcriptions utilizing both the Whisper or Whisper JAX mannequin.Attributes:
audio_file_path (str): Path to the audio file to transcribe.
model_type (str): The kind of mannequin to make use of for transcription, both "whisper" or "whisper_jax".
gadget (str): The gadget to make use of for inference (e.g., "cpu" or "cuda").
model_name (str): The precise mannequin to make use of (e.g., "base", "medium", "giant", or "large-v2").
dtype (Optionally available[str]): The info sort to make use of for Whisper JAX, both "bfloat16" or "bfloat32".
batch_size (Optionally available[int]): The batch measurement to make use of for Whisper JAX.
"""
_instance = None
def __new__(cls, *args, **kwargs):
if cls._instance is None:
cls._instance = tremendous().__new__(cls)
return cls._instance
def __init__(
self,
audio_file_path: str,
model_type: str = "whisper",
gadget: str = "cpu",
model_name: str = "base",
dtype: Optionally available[str] = None,
batch_size: Optionally available[int] = None,
):
self.audio_file_path = audio_file_path
self.gadget = gadget
self.model_type = model_type
self.model_name = model_name
self.dtype = dtype
self.batch_size = batch_size
self.pipeline = None
The set_pipeline
technique units up the pipeline for the required mannequin sort. Relying on the worth of the model_type
attribute, the tactic initializes the pipeline utilizing both by instantiating the FlaxWhisperPipline
class for Whisper JAX or by calling the whisper.load_model()
perform for the PyTorch implementation of Whisper.
def set_pipeline(self) -> None:
"""
Arrange the pipeline for the required mannequin sort.Returns:
None
"""
if self.model_type == "whisper_jax":
pipeline_kwargs = {}
if self.dtype:
pipeline_kwargs["dtype"] = getattr(jnp, self.dtype)
if self.batch_size:
pipeline_kwargs["batch_size"] = self.batch_size
self.pipeline = FlaxWhisperPipline(
f"openai/whisper-{self.model_name}", **pipeline_kwargs
)
elif self.model_type == "whisper":
self.pipeline = whisper.load_model(
self.model_name,
torch.gadget("cuda:0") if self.gadget == "gpu" else self.gadget,
)
else:
increase ValueError(f"Invalid mannequin sort: {self.model_type}")
The run_pipeline
technique transcribes the audio file and returns the outcomes as an inventory of dictionaries containing the transcribed textual content and timestamps. Within the case of Whisper JAX, it considers non-compulsory parameters like information sort and batch measurement, if offered. Discover which you can set return_timestamps
to False
if you’re solely occupied with getting the transcription. The mannequin output is completely different if we run the transcription course of with the PyTorch implementation. Thus, we should create a brand new object that aligns each return objects.
def run_pipeline(self) -> Record[Dict[str, Union[Tuple[float, float], str]]]:
"""
Run the transcription pipeline a second time.Returns:
An inventory of dictionaries, every containing textual content and a tuple of begin and finish timestamps.
"""
if not hasattr(self, "pipeline"):
increase ValueError("Pipeline not initialized. Name set_pipeline() first.")
if self.model_type == "whisper_jax":
outputs = self.pipeline(
self.audio_file_path, process="transcribe", return_timestamps=True
)
return outputs["chunks"]
elif self.model_type == "whisper":
end result = self.pipeline.transcribe(self.audio_file_path)
formatted_result = [
{
"timestamp": (segment["start"], section["end"]),
"textual content": section["text"],
}
for section in end result["segments"]
]
return formatted_result
else:
increase ValueError(f"Invalid mannequin sort: {self.model_type}")
Lastly, the transcribe_multiple()
technique allows the transcription of a number of audio recordsdata. It takes an inventory of audio file paths and returns an inventory of transcriptions for every audio file, the place every transcription is an inventory of dictionaries containing textual content and a tuple of begin and finish timestamps.
def transcribe_multiple(
self, audio_file_paths: Record[str]
) -> Record[List[Dict[str, Union[Tuple[float, float], str]]]]:
"""
Transcribe a number of audio recordsdata utilizing the required mannequin sort.Args:
audio_file_paths (Record[str]): An inventory of audio file paths to transcribe.
Returns:
Record[List[Dict[str, Union[Tuple[float, float], str]]]]: An inventory of transcriptions for every audio file, the place every transcription is an inventory of dictionaries containing textual content and a tuple of begin and finish timestamps.
"""
transcriptions = []
for audio_file_path in audio_file_paths:
self.audio_file_path = audio_file_path
self.set_pipeline()
transcription = self.run_pipeline()
transcriptions.append(transcription)
return transcriptions
Experimental Setup
We used a protracted audio clip with greater than half-hour to judge the efficiency of Whisper variants, with a PyTorch and JAX implementation. The researchers that developed Whisper JAX declare that the distinction is extra important when transcribing lengthy audio recordsdata.
Our experimental {hardware} setup consists of the next key parts. For the CPU, we now have an x86_64 structure with a complete of 112 cores, powered by an Intel(R) Xeon(R) Gold 6258R CPU operating at 2.70GHz. Concerning GPU, we use an NVIDIA Quadro RTX 8000 with 48 GB of VRAM.
Outcomes and Dialogue
On this part, we talk about the outcomes obtained from the experiments to check the efficiency of Whisper JAX and PyTorch implementations. Our outcomes present insights into the velocity and effectivity of the 2 implementations on each GPU and CPU platforms.
Our first experiment concerned operating a protracted audio (over half-hour) utilizing GPU and the bigger Whisper mannequin (large-v2) that requires roughly 10GB of VRAM. Opposite to the declare made by the authors of Whisper JAX, our outcomes point out that the JAX implementation is slower than the PyTorch model. Even with the incorporation of half-precision and batching, we couldn’t surpass the efficiency of the PyTorch implementation utilizing Whisper JAX. Whisper JAX took virtually twice the time in comparison with the PyTorch implementation to carry out the same transcription. We additionally noticed an unusually lengthy transcription time when each half-precision and batching have been employed.
Then again, when evaluating the CPU efficiency, our outcomes present that Whisper JAX outperforms the PyTorch implementation. The speedup issue was roughly two occasions quicker for Whisper JAX in comparison with the PyTorch model. We noticed this sample for the bottom and important mannequin variations.
Concerning the declare made by the authors of Whisper JAX that the second transcription must be a lot quicker, our experiments didn’t present supporting proof. The distinction in velocity between the primary and second transcriptions was not important. Plus, we discovered that the sample was comparable between each Whisper and Whisper JAX implementations.
On this article, we introduced a complete evaluation of the Whisper JAX implementation, evaluating its efficiency to the unique PyTorch implementation of Whisper. Our experiments aimed to judge the claimed 70x velocity enchancment utilizing quite a lot of setups, together with completely different {hardware} and hyperparameters for the Whisper JAX mannequin.
The outcomes confirmed that Whisper JAX outperformed the PyTorch implementation on CPU platforms, with a speedup issue of roughly two fold. Nonetheless, our experiments didn’t assist the authors’ claims that Whisper JAX is considerably quicker on GPU platforms. Really, the PyTorch implementation carried out higher when transcribing lengthy audio recordsdata utilizing a GPU.
Moreover, we discovered no important distinction within the velocity between the primary and second transcriptions, a declare made by the Whisper JAX authors. Each implementations exhibited the same sample on this regard.
Keep up a correspondence: LinkedIn