Hyperparameter Optimization With Hyperopt — Intro & Implementation | by Farzad Mahmoodinobar | Jun, 2023
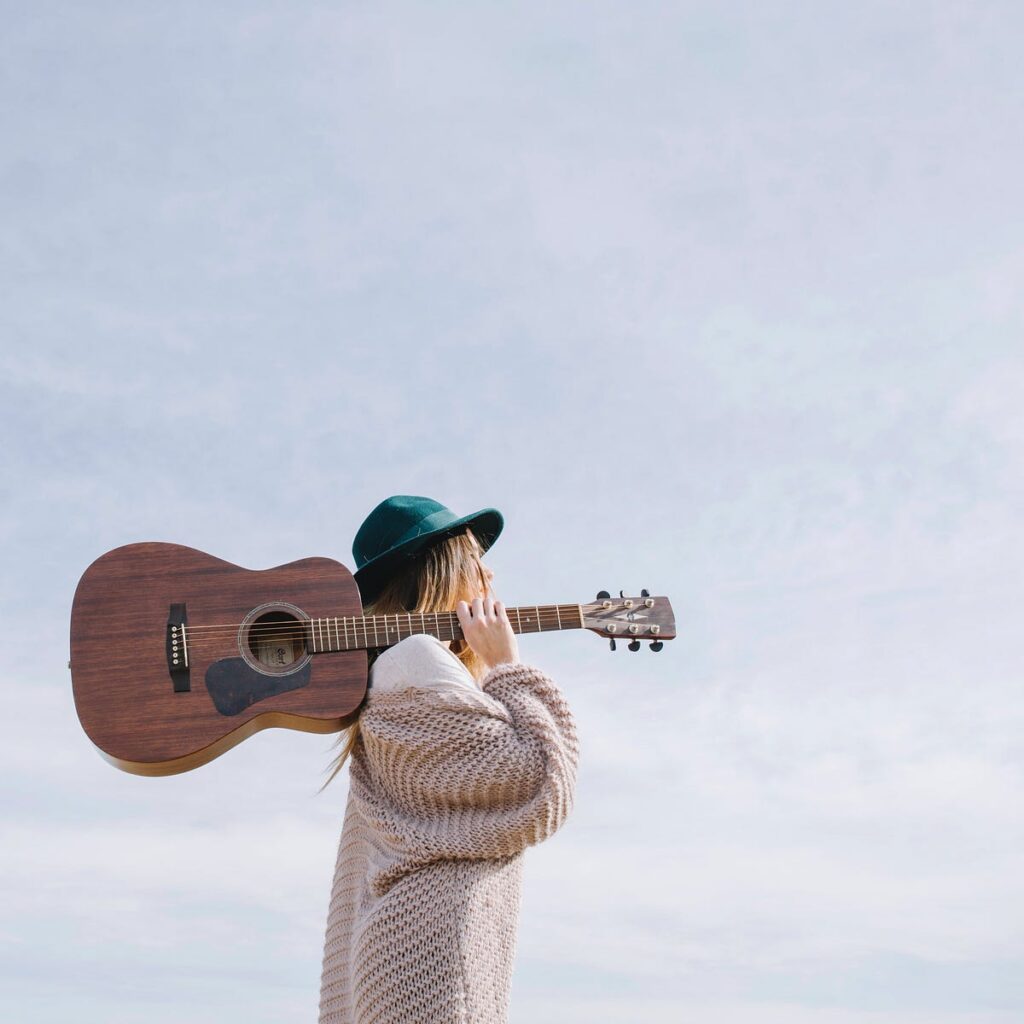
2.1. Help Vector Machines and Iris Knowledge Set
In a earlier post I used Grid Search, Random Search and Bayesian Optimization for hyperparameter optimization utilizing the Iris data set provided by scikit-learn. Iris knowledge set consists of 3 completely different irises petal and sepal lengths and is a commonly-used knowledge set for classification workouts. On this submit, we are going to use the identical knowledge set however we are going to use a Help Vector Machine (SVM) as a mannequin with two parameters that we will optimize as follows:
C
: Regularization parameter, which trades off misclassification of coaching examples towards simplicity of the choice floor.gamma
: Kernel coefficient, which defines how a lot affect a single coaching instance has. The bigger gamma is, the nearer different examples should be to be affected.
Because the purpose of this train is to undergo the hyperparameter optimization, I cannot go deeper into what SVMs do however in case you are , I discover this scikit-learn submit useful.
We are going to typically comply with the identical steps that we used within the easy instance earlier however may also visualize the method on the finish:
1. Import needed libraries and packages
2. Outline the target perform and the search house
3. Run the optimization course of
4. Visualize the optimization
2.1.1. Step 1 — Import Libraries and Packages
Let’s import the libraries and packages after which load the info set.
# Import libraries and packages
from sklearn import datasets
from sklearn.svm import SVC
from sklearn.model_selection import cross_val_score# Load Iris dataset
iris = datasets.load_iris()
X = iris.knowledge
y = iris.goal
2.1.2. Step 2 — Outline Goal Operate and Search Area
Let’s first begin with defining the target perform, which can prepare an SVM and returns the detrimental of the cross-validation rating — that’s what we need to decrease. Notice that we’re minimizing the detrimental of cross-validation rating to be in line with the final purpose of “minimizing” the target perform (as a substitute of “maximizing” the cross-validation rating).
def objective_function(parameters):
clf = SVC(**parameters)
rating = cross_val_score(clf, X, y, cv=5).imply()
return -score
Subsequent we are going to outline the search house, which consists of the values that our parameters of C
and gamma
can take. Notice that we are going to use Hyperopt’s hp.uniform(label, low, excessive)
, which returns a price uniformly between “low” and “excessive” (source).
# Search Area
search_space = {
'C': hp.uniform('C', 0.1, 10),
'gamma': hp.uniform('gamma', 0.01, 1)
}
2.1.3. Run Optimization
Similar as the easy instance earlier, we are going to use a TPE algorithm and retailer the ends in a Trials
object.
# Trials object to retailer the outcomes
trials = Trials()# Run optimization
finest = fmin(fn=objective_function, house=search_space, algo=tpe.counsel, trials=trials, max_evals=100)
Outcomes: