Construct a strong query answering bot with Amazon SageMaker, Amazon OpenSearch Service, Streamlit, and LangChain
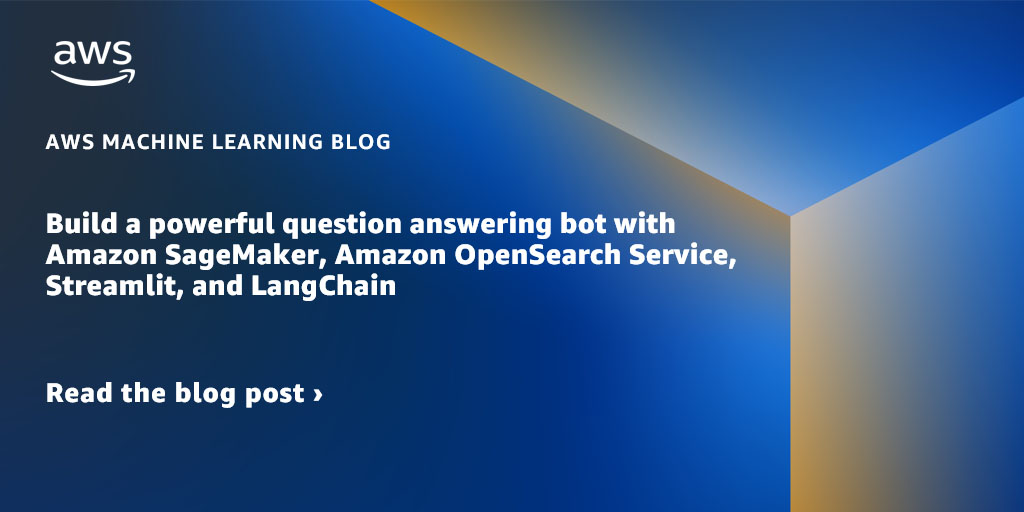
One of the crucial widespread purposes of generative AI and enormous language fashions (LLMs) in an enterprise atmosphere is answering questions based mostly on the enterprise’s information corpus. Amazon Lex supplies the framework for constructing AI based chatbots. Pre-trained basis fashions (FMs) carry out properly at pure language understanding (NLU) duties such summarization, textual content era and query answering on a broad number of matters however both battle to supply correct (with out hallucinations) solutions or utterly fail at answering questions on content material that they haven’t seen as a part of their coaching knowledge. Moreover, FMs are educated with a time limit snapshot of information and haven’t any inherent skill to entry contemporary knowledge at inference time; with out this skill they may present responses which are probably incorrect or insufficient.
A generally used strategy to deal with this downside is to make use of a way referred to as Retrieval Augmented Era (RAG). Within the RAG-based strategy we convert the consumer query into vector embeddings utilizing an LLM after which do a similarity seek for these embeddings in a pre-populated vector database holding the embeddings for the enterprise information corpus. A small variety of comparable paperwork (sometimes three) is added as context together with the consumer query to the “immediate” supplied to a different LLM after which that LLM generates a solution to the consumer query utilizing data supplied as context within the immediate. RAG fashions had been launched by Lewis et al. in 2020 as a mannequin the place parametric reminiscence is a pre-trained seq2seq mannequin and the non-parametric reminiscence is a dense vector index of Wikipedia, accessed with a pre-trained neural retriever. To grasp the general construction of a RAG-based strategy, confer with Question answering using Retrieval Augmented Generation with foundation models in Amazon SageMaker JumpStart.
On this put up we offer a step-by-step information with all of the constructing blocks for creating an enterprise prepared RAG software comparable to a query answering bot. We use a mixture of various AWS providers, open-source basis fashions (FLAN-T5 XXL for textual content era and GPT-j-6B for embeddings) and packages comparable to LangChain for interfacing with all of the parts and Streamlit for constructing the bot frontend.
We offer an AWS Cloud Formation template to face up all of the assets required for constructing this answer. We then show the way to use LangChain for tying all the pieces collectively:
- Interfacing with LLMs hosted on Amazon SageMaker.
- Chunking of data base paperwork.
- Ingesting doc embeddings into Amazon OpenSearch Service.
- Implementing the query answering activity.
We will use the identical structure to swap the open-source fashions with the Amazon Titan fashions. After Amazon Bedrock launches, we’ll publish a follow-up put up displaying the way to implement comparable generative AI purposes utilizing Amazon Bedrock, so keep tuned.
Answer overview
We use the SageMaker docs because the information corpus for this put up. We convert the HTML pages on this website into smaller overlapping chunks (to retain some context continuity between chunks) of data after which convert these chunks into embeddings utilizing the gpt-j-6b mannequin and retailer the embeddings in OpenSearch Service. We implement the RAG performance inside an AWS Lambda operate with Amazon API Gateway to deal with routing all requests to the Lambda. We implement a chatbot software in Streamlit which invokes the operate through the API Gateway and the operate does a similarity search within the OpenSearch Service index for the embeddings of consumer query. The matching paperwork (chunks) are added to the immediate as context by the Lambda operate after which the operate makes use of the flan-t5-xxl mannequin deployed as a SageMaker endpoint to generate a solution to the consumer query. All of the code for this put up is on the market within the GitHub repo.
The next determine represents the high-level structure of the proposed answer.
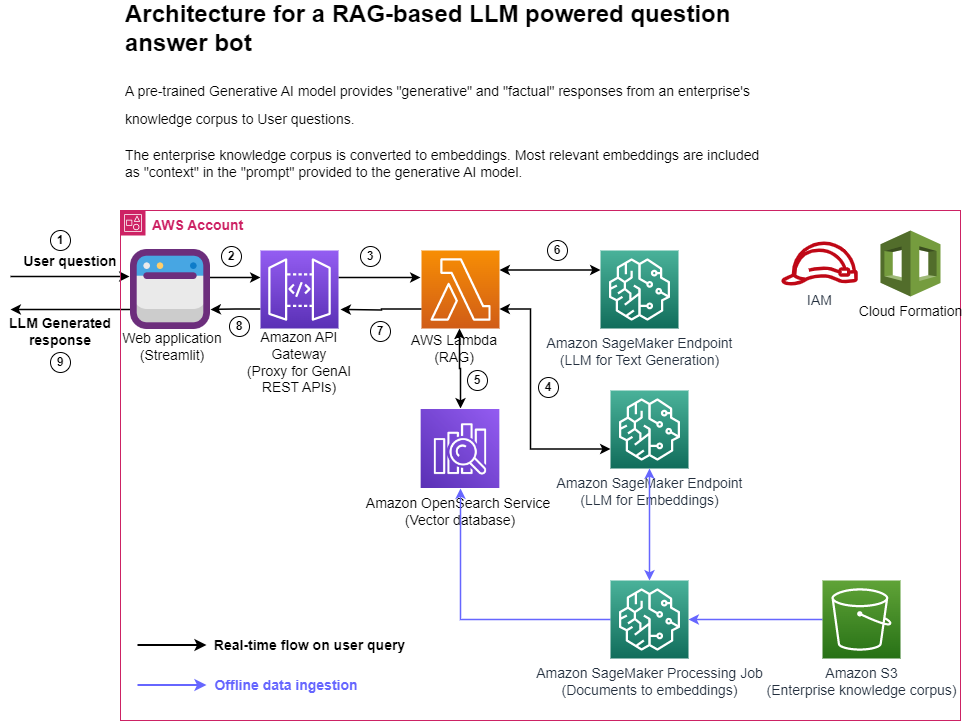
Determine 1: Structure
Step-by-step rationalization:
- The Person supplies a query through the Streamlit net software.
- The Streamlit software invokes the API Gateway endpoint REST API.
- The API Gateway invokes the Lambda operate.
- The operate invokes the SageMaker endpoint to transform consumer query into embeddings.
- The operate invokes invokes an OpenSearch Service API to search out comparable paperwork to the consumer query.
- The operate creates a “immediate” with the consumer question and the “comparable paperwork” as context and asks the SageMaker endpoint to generate a response.
- The response is supplied from the operate to the API Gateway.
- The API Gateway supplies the response to the Streamlit software.
- The Person is ready to view the response on the Streamlit software,
As illustrated within the structure diagram, we use the next AWS providers:
By way of open-source packages used on this answer, we use LangChain for interfacing with OpenSearch Service and SageMaker, and FastAPI for implementing the REST API interface within the Lambda.
The workflow for instantiating the answer offered on this put up in your individual AWS account is as follows:
- Run the CloudFormation template supplied with this put up in your account. This can create all the mandatory infrastructure assets wanted for this answer:
- SageMaker endpoints for the LLMs
- OpenSearch Service cluster
- API Gateway
- Lambda operate
- SageMaker Pocket book
- IAM roles
- Run the data_ingestion_to_vectordb.ipynb pocket book within the SageMaker pocket book to ingest knowledge from SageMaker docs into an OpenSearch Service index.
- Run the Streamlit software on a terminal in Studio and open the URL for the appliance in a brand new browser tab.
- Ask your questions on SageMaker through the chat interface supplied by the Streamlit app and consider the responses generated by the LLM.
These steps are mentioned intimately within the following sections.
Stipulations
To implement the answer supplied on this put up, it is best to have an AWS account and familiarity with LLMs, OpenSearch Service and SageMaker.
We want entry to accelerated cases (GPUs) for internet hosting the LLMs. This answer makes use of one occasion every of ml.g5.12xlarge and ml.g5.24xlarge; you possibly can verify the supply of those cases in your AWS account and request these cases as wanted through a Sevice Quota enhance request as proven within the following screenshot.
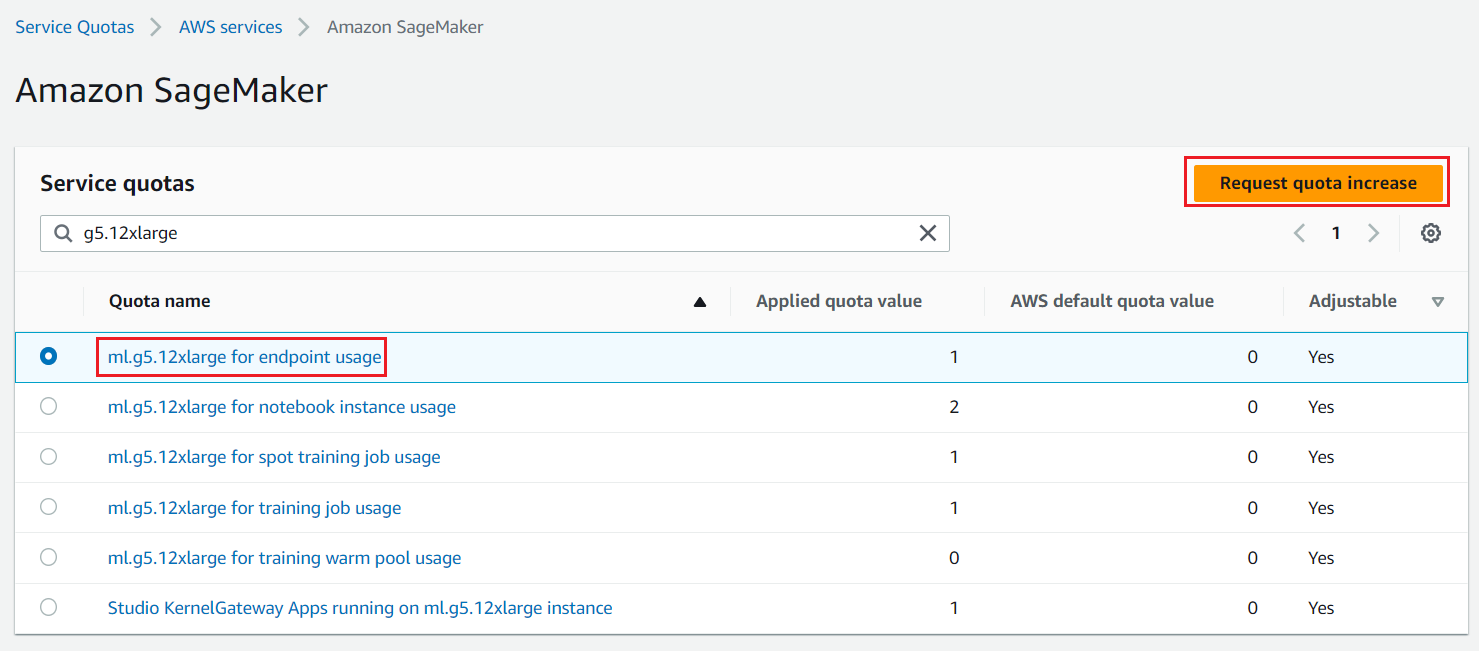
Determine 2: Service Quota Improve Request
Use AWS Cloud Formation to create the answer stack
We use AWS CloudFormation to create a SageMaker pocket book referred to as aws-llm-apps-blog
and an IAM function referred to as LLMAppsBlogIAMRole
. Select Launch Stack for the Area you need to deploy assets to. All parameters wanted by the CloudFormation template have default values already stuffed in, apart from the OpenSearch Service password which you’d have to supply. Make a remark of the OpenSearch Service username and password, we use these in subsequent steps. This template takes about quarter-hour to finish.
AWS Area | Hyperlink |
---|---|
us-east-1 |
![]() |
us-west-2 |
![]() |
eu-west-1 |
![]() |
ap-northeast-1 |
![]() |
After the stack is created efficiently, navigate to the stack’s Outputs tab on the AWS CloudFormation console and notice the values for OpenSearchDomainEndpoint
and LLMAppAPIEndpoint
. We use these within the subsequent steps.
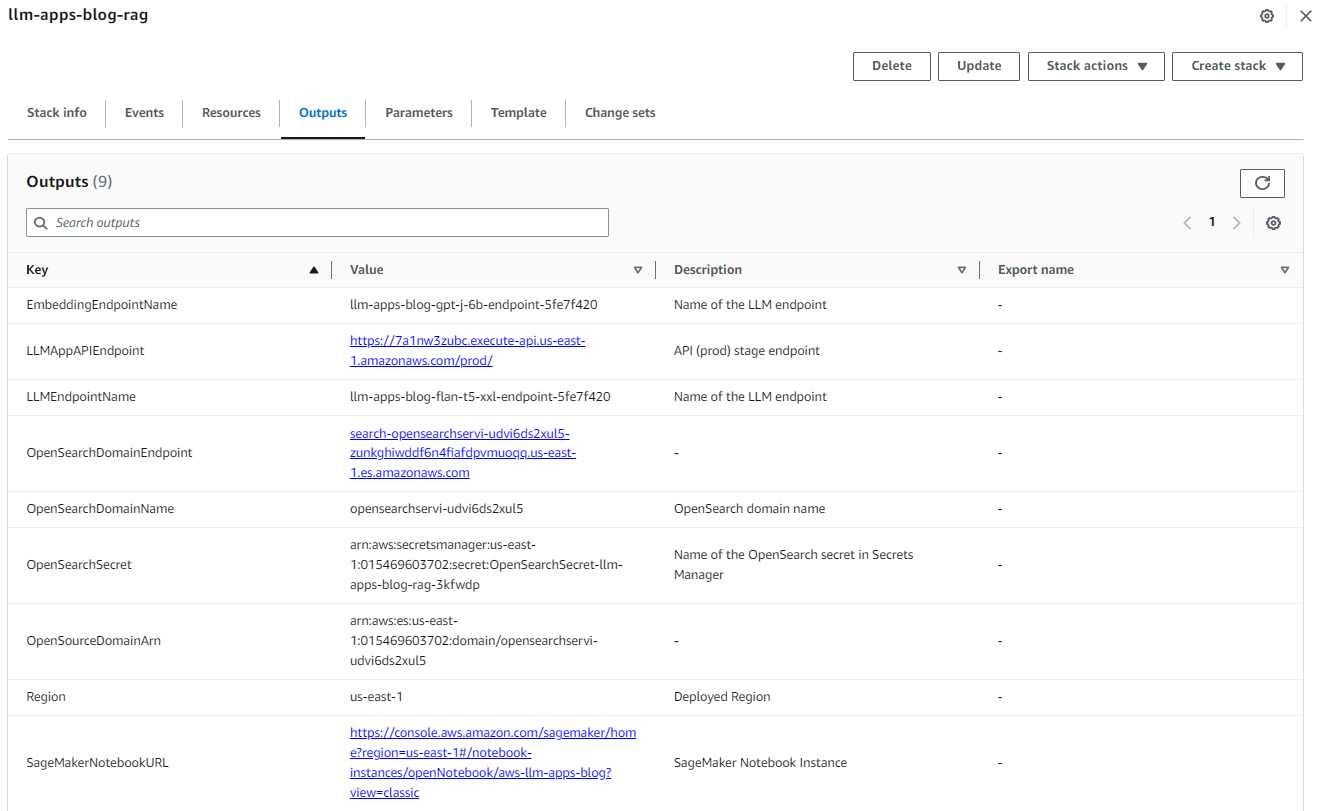
Determine 3: Cloud Formation Stack Outputs
Ingest the info into OpenSearch Service
To ingest the info, full the next steps:
- On the SageMaker console, select Notebooks within the navigation pane.
- Choose the pocket book
aws-llm-apps-blog
and select Open JupyterLab.Determine 4: Open JupyterLab
- Select data_ingestion_to_vectordb.ipynb to open it in JupyterLab. This pocket book will ingest the SageMaker docs to an OpenSearch Service index referred to as
llm_apps_workshop_embeddings
.Determine 5: Open Knowledge Ingestion Pocket book
- When the pocket book is open, on the Run menu, select Run All Cells to run the code on this pocket book. This can obtain the dataset regionally into the pocket book after which ingest it into the OpenSearch Service index. This pocket book takes about 20 minutes to run. The pocket book additionally ingests the info into one other vector database referred to as FAISS. The FAISS index information are saved regionally and the uploaded to Amazon Easy Storage Service (S3) in order that they will optionally be utilized by the Lambda operate as an illustration of utilizing an alternate vector database.
Determine 6: Pocket book Run All Cells
Now we’re prepared to separate the paperwork into chunks, which may then be transformed into embeddings to be ingested into OpenSearch. We use the LangChain RecursiveCharacterTextSplitter
class to chunk the paperwork after which use the LangChain SagemakerEndpointEmbeddingsJumpStart
class to transform these chunks into embeddings utilizing the gpt-j-6b LLM. We retailer the embeddings in OpenSearch Service through the LangChain OpenSearchVectorSearch
class. We bundle this code into Python scripts which are supplied to the SageMaker Processing Job through a customized container. See the data_ingestion_to_vectordb.ipynb pocket book for the total code.
- Create a customized container, then set up in it the LangChain and opensearch-py Python packages.
- Add this container picture to Amazon Elastic Container Registry (ECR).
- We use the SageMaker ScriptProcessor class to create a SageMaker Processing job that may run on a number of nodes.
- The information information obtainable in Amazon S3 are robotically distributed throughout within the SageMaker Processing job cases by setting
s3_data_distribution_type="ShardedByS3Key"
as a part of theProcessingInput
supplied to the processing job. - Every node processes a subset of the information and this brings down the general time required to ingest the info into OpenSearch Service.
- Every node additionally makes use of Python multiprocessing to internally additionally parallelize the file processing. Due to this fact, there are two ranges of parallelization taking place, one on the cluster degree the place particular person nodes are distributing the work (information) amongst themselves and one other on the node degree the place the information in a node are additionally cut up between a number of processes operating on the node.
- The information information obtainable in Amazon S3 are robotically distributed throughout within the SageMaker Processing job cases by setting
- Shut the pocket book in spite of everything cells run with none error. Your knowledge is now obtainable in OpenSearch Service. Enter the next URL in your browser’s handle bar to get a depend of paperwork within the
llm_apps_workshop_embeddings
index. Use the OpenSearch Service area endpoint from the CloudFormation stack outputs within the URL under. You’d be prompted for the OpenSearch Service username and password, these can be found from the CloudFormations stack.
The browser window ought to present an output just like the next. This output exhibits that 5,667 paperwork had been ingested into the llm_apps_workshop_embeddings index. {"depend":5667,"_shards":{"whole":5,"profitable":5,"skipped":0,"failed":0}}
Run the Streamlit software in Studio
Now we’re able to run the Streamlit net software for our query answering bot. This software permits the consumer to ask a query after which fetches the reply through the /llm/rag
REST API endpoint supplied by the Lambda operate.
Studio supplies a handy platform to host the Streamlit net software. The next steps describes the way to run the Streamlit app on Studio. Alternatively, you could possibly additionally comply with the identical process to run the app in your laptop computer.
- Open Studio after which open a brand new terminal.
- Run the next instructions on the terminal to clone the code repository for this put up and set up the Python packages wanted by the appliance:
- The API Gateway endpoint URL that’s obtainable from the CloudFormation stack output must be set within the webapp.py file. That is executed by operating the next
sed
command. Change thereplace-with-LLMAppAPIEndpoint-value-from-cloudformation-stack-outputs
within the shell instructions with the worth of theLLMAppAPIEndpoint
subject from the CloudFormation stack output after which run the next instructions to begin a Streamlit app on Studio. - When the appliance runs efficiently, you’ll see an output just like the next (the IP addresses you will notice can be completely different from those proven on this instance). Notice the port quantity (sometimes 8501) from the output to make use of as a part of the URL for app within the subsequent step.
- You possibly can entry the app in a brand new browser tab utilizing a URL that’s just like your Studio area URL. For instance, in case your Studio URL is
https://d-randomidentifier.studio.us-east-1.sagemaker.aws/jupyter/default/lab?
then the URL in your Streamlit app can behttps://d-randomidentifier.studio.us-east-1.sagemaker.aws/jupyter/default/proxy/8501/webapp
(discover thatlab
is changed withproxy/8501/webapp
). If the port quantity famous within the earlier step is completely different from 8501 then use that as a substitute of 8501 within the URL for the Streamlit app.
The next screenshot exhibits the app with a few consumer questions.
A better have a look at the RAG implementation within the Lambda operate
Now that we have now the appliance working finish to finish, lets take a better have a look at the Lambda operate. The Lambda operate makes use of FastAPI to implement the REST API for RAG and the Mangum bundle to wrap the API with a handler that we bundle and deploy within the operate. We use the API Gateway to route all incoming requests to invoke the operate and deal with the routing internally inside our software.
The next code snippet exhibits how we discover paperwork within the OpenSearch index which are just like the consumer query after which create a immediate by combining the query and the same paperwork. This immediate is then supplied to the LLM for producing a solution to the consumer query.
Clear up
To keep away from incurring future fees, delete the assets. You are able to do this by deleting the CloudFormation stack as proven within the following screenshot.

Determine 7: Cleansing Up
Conclusion
On this put up, we confirmed the way to create an enterprise prepared RAG answer utilizing a mixture of AWS service, open-source LLMs and open-source Python packages.
We encourage you to study extra by exploring JumpStart, Amazon Titan fashions, Amazon Bedrock, and OpenSearch Service and constructing an answer utilizing the pattern implementation supplied on this put up and a dataset related to your online business. When you have questions or strategies, depart a remark.
In regards to the Authors
Amit Arora is an AI and ML Specialist Architect at Amazon Net Companies, serving to enterprise clients use cloud-based machine studying providers to quickly scale their improvements. He’s additionally an adjunct lecturer within the MS knowledge science and analytics program at Georgetown College in Washington D.C.
Dr. Xin Huang is a Senior Utilized Scientist for Amazon SageMaker JumpStart and Amazon SageMaker built-in algorithms. He focuses on creating scalable machine studying algorithms. His analysis pursuits are within the space of pure language processing, explainable deep studying on tabular knowledge, and strong evaluation of non-parametric space-time clustering. He has revealed many papers in ACL, ICDM, KDD conferences, and Royal Statistical Society: Sequence A.
Navneet Tuteja is a Knowledge Specialist at Amazon Net Companies. Earlier than becoming a member of AWS, Navneet labored as a facilitator for organizations searching for to modernize their knowledge architectures and implement complete AI/ML options. She holds an engineering diploma from Thapar College, in addition to a grasp’s diploma in statistics from Texas A&M College.