The Newbie’s Information to Pure Language Processing with Python
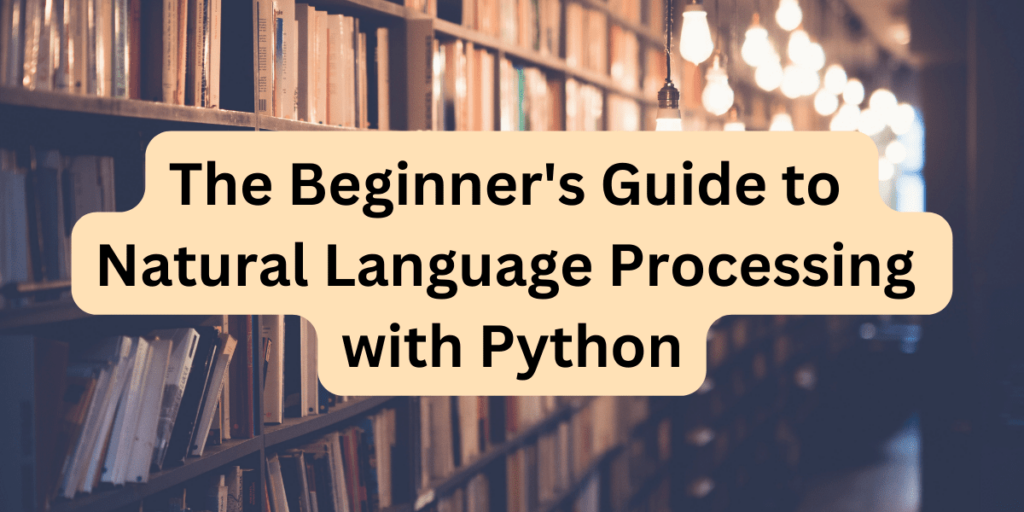
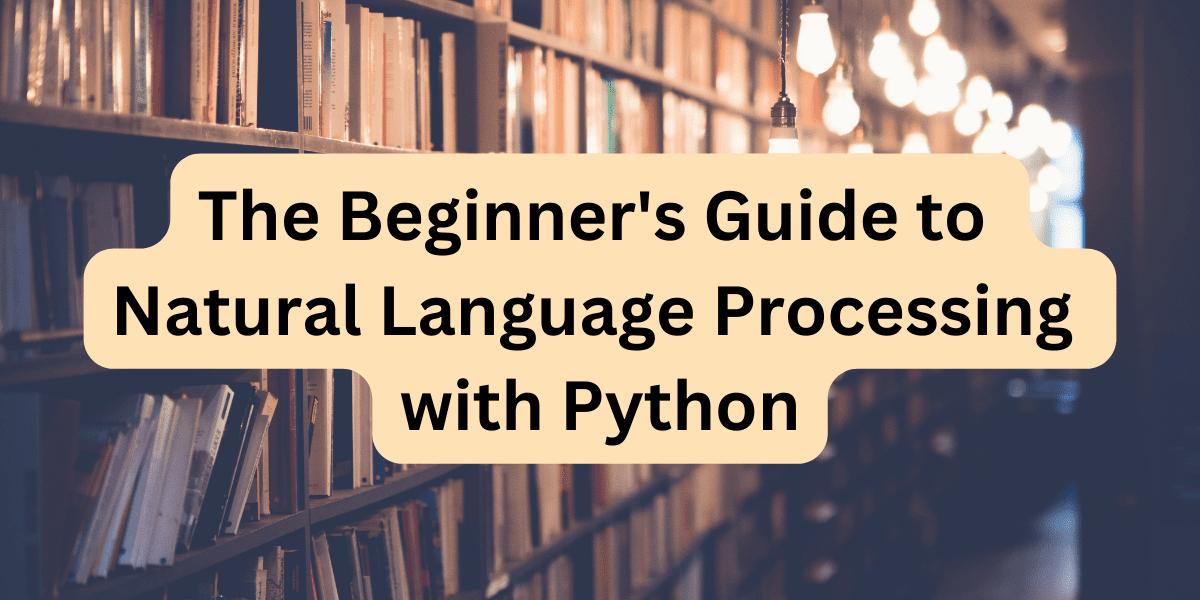
The Newbie’s Information to Pure Language Processing with Python
Picture by Writer | Created on Canva
Studying pure language processing could be a tremendous helpful addition to your developer toolkit. From the fundamentals to constructing LLM-powered functions, you may stand up to hurry pure language processing—in a number of weeks—one small step at a time. And this text will aid you get began.
On this article, we’ll be taught the fundamentals of pure language processing with Python—taking a code-first strategy utilizing NLTK or the Natural Language Toolkit (NLTK). Let’s start!
▶️ Hyperlink to the Google Colab notebook for this tutorial
Putting in NLTK
Earlier than diving into NLP duties, we have to set up the Natural Language Toolkit (NLTK). NLTK offers a collection of textual content processing instruments—tokenizers, lemmatizers, POS taggers, and preloaded datasets. It’s extra like a Swiss military knife of NLP. Setting it up includes putting in the library and downloading the mandatory datasets and fashions.
Set up the NLTK Library
Run the next command in your terminal or command immediate to put in NLTK:
This installs the core NLTK library, which incorporates the principle modules wanted for textual content processing duties.
Obtain NLTK Sources
After set up, obtain NLTK’s pre-packaged datasets and instruments. These embrace stopword lists, tokenizers, and lexicons like WordNet:
import nltk
# Obtain important datasets and fashions nltk.obtain(‘punkt’) # Tokenizers for sentence and phrase tokenization nltk.obtain(‘stopwords’) # Record of frequent cease phrases nltk.obtain(‘wordnet’) # WordNet lexical database for lemmatization nltk.obtain(‘averaged_perceptron_tagger_eng’) # Half-of-speech tagger nltk.obtain(‘maxent_ne_chunker_tab’) # Named Entity Recognition mannequin nltk.obtain(‘phrases’) # Phrase corpus for NER nltk.obtain(‘punkt_tab’) |
Textual content Preprocessing
Textual content preprocessing is an important step in NLP, reworking uncooked textual content right into a clear and structured format that makes it simpler to research. The purpose is to zero in on the significant parts of the textual content whereas additionally breaking down the textual content into chunks that may be processed.
On this part, we cowl three necessary preprocessing steps: tokenization, cease phrase elimination, and stemming.
Tokenization
Tokenization is among the frequent preprocessing duties. It includes splitting textual content into smaller models—tokens. These tokens might be phrases, sentences, and even sub-word models, relying on the duty.
- Sentence tokenization splits the textual content into sentences
- Phrase tokenization splits the textual content into phrases and punctuation marks
Within the following code, we use NLTK’s sent_tokenize to separate the enter textual content into sentences, and word_tokenize to interrupt it down into phrases. However we additionally do a brilliant easy prerpocessing step of eradicating all punctuation from the textual content:
import string from nltk.tokenize import word_tokenize, sent_tokenize
textual content = “Pure Language Processing (NLP) is cool! Let’s discover it.”
# Take away punctuation utilizing string.punctuation cleaned_text = ”.be part of(char for char in textual content if char not in string.punctuation) print(“Textual content with out punctuation:”, cleaned_text)
# Sentence Tokenization sentences = sent_tokenize(cleaned_text) print(“Sentences:”, sentences)
# Phrase Tokenization phrases = word_tokenize(cleaned_text) print(“Phrases:”, phrases) |
This permits us to research the construction of the textual content at each the sentence and phrase ranges.
On this instance, sent_tokenize(textual content) splits the enter string into sentences, returning an inventory of sentence strings. The output of this operate is an inventory with two components: one for every sentence within the unique textual content.
Subsequent, the word_tokenize(textual content) operate is utilized to the identical textual content. It breaks down the textual content into particular person phrases and punctuation, treating issues like parentheses and exclamation marks as separate tokens. However we’ve eliminated all punctuation, so the output is as follows:
Textual content with out punctuation: Pure Language Processing NLP is cool Lets discover it Sentences: [‘Natural Language Processing NLP is cool Lets explore it’] Phrases: [‘Natural’, ‘Language’, ‘Processing’, ‘NLP’, ‘is’, ‘cool’, ‘Lets’, ‘explore’, ‘it’] |
Stopwords Elimination
Stopwords are frequent phrases reminiscent of “the,” “and,” or “is” that happen regularly however carry little which means in most analyses. Eradicating these phrases helps give attention to the extra significant phrases within the textual content.
In essence, you filter out cease phrases to scale back noise within the dataset. We will use NLTK’s stopwords corpus to establish and take away cease phrases from the record of tokens obtained after tokenization:
from nltk.corpus import stopwords
# Load NLTK’s stopwords record stop_words = set(stopwords.phrases(‘english’))
# Filter out cease phrases filtered_words = [word for word in words if word.lower() not in stop_words] print(“Filtered Phrases:”, filtered_words) |
Right here, we load the set of English cease phrases utilizing stopwords.phrases(‘english’) from NLTK. Then, we use an inventory comprehension to iterate over the record of tokens generated by word_tokenize. By checking whether or not every token (transformed to lowercase) is within the set of cease phrases, we take away frequent phrases that don’t contribute to the which means of the textual content.
Right here’s the filtered consequence:
Filtered Phrases: [‘Natural’, ‘Language’, ‘Processing’, ‘NLP’, ‘cool’, ‘Lets’, ‘explore’] |
Stemming
Stemming is the method of lowering phrases to their root kind by eradicating affixes like suffixes and prefixes. The basis kind might not all the time be a sound phrase within the dictionary, however it helps in standardizing variations of the identical phrase.
Porter Stemmer is a typical stemming algorithm that works by eradicating suffixes. Let’s use NLTK’s PorterStemmer to stem the filtered glossary:
from nltk.stem import PorterStemmer
# Initialize the Porter Stemmer stemmer = PorterStemmer()
# Apply stemming to filtered phrases stemmed_words = [stemmer.stem(word) for word in filtered_words] print(“Stemmed Phrases:”, stemmed_words) |
Right here, we initialize the PorterStemmer and use it to course of every phrase within the record filtered_words.
The stemmer.stem() operate strips frequent suffixes like “-ing,” “-ed,” and “-ly” from phrases to scale back them to their root kind. Whereas stemming helps scale back the variety of variations of phrases, it’s necessary to notice that the outcomes might not all the time be legitimate dictionary phrases.
Stemmed Phrases: [‘natur’, ‘languag’, ‘process’, ‘nlp’, ‘cool’, ‘let’, ‘explor’] |
Earlier than we proceed, right here’s a abstract of the textual content preprocessing steps:
- Tokenization breaks textual content into smaller models.
- Cease phrase elimination filters out frequent, non-meaningful phrases to give attention to extra important phrases within the evaluation.
- Stemming reduces phrases to their root types, simplifying variations and serving to standardize textual content for evaluation.
With these preprocessing steps accomplished, you may transfer on to study lemmatization, part-of-speech tagging, and named entity recognition.
Lemmatization
Lemmatization is much like stemming in that it additionally reduces phrases to their base kind. However in contrast to stemming, lemmatization returns legitimate dictionary phrases. Lemmatization elements within the context reminiscent of its a part of speech (POS) to scale back the phrase to its lemma. For instance, the phrases “operating” and “ran” could be diminished to “run.”
Lemmatization usually produces extra correct outcomes than stemming, because it retains the phrase in a recognizable kind. The commonest device for lemmatization in NLTK is the WordNetLemmatizer, which makes use of the WordNet lexical database.
- Lemmatization reduces a phrase to its lemma by contemplating its which means and context—not simply by chopping off affixes.
- WordNetLemmatizer is the NLTK device generally used for lemmatization.
Within the code snippet beneath, we use NLTK’s WordNetLemmatizer to lemmatize phrases from the beforehand filtered record:
from nltk.stem import WordNetLemmatizer
# Initialize the Lemmatizer lemmatizer = WordNetLemmatizer()
# Lemmatize every phrase lemmatized_words = [lemmatizer.lemmatize(word, pos=‘v’) for word in filtered_words] print(“Lemmatized Phrases:”, lemmatized_words) |
Right here, we initialize the WordNetLemmatizer and use its lemmatize() methodology to course of every phrase within the filtered_words record. We specify pos=’v’ to inform the lemmatizer that we’d like to scale back verbs within the textual content to their root kind. This helps the lemmatizer perceive the a part of speech and apply the right lemmatization rule.
Lemmatized Phrases: [‘Natural’, ‘Language’, ‘Processing’, ‘NLP’, ‘cool’, ‘Lets’, ‘explore’] |
So why is lemmatization useful? Lemmatization is especially helpful if you need to scale back phrases to their base kind however nonetheless retain their which means. It’s a extra correct and context-sensitive methodology in comparison with stemming. Which makes it superb for duties that require excessive accuracy, reminiscent of textual content classification or sentiment evaluation.
Half-of-Speech (POS) Tagging
Half-of-speech (POS) tagging includes figuring out the grammatical class of every phrase in a sentence, reminiscent of nouns, verbs, adjectives, adverbs, and extra. POS tagging additionally helps perceive the syntactic construction of a sentence, enabling higher dealing with of duties reminiscent of textual content parsing, data extraction, and machine translation.
The POS tags assigned to phrases might be primarily based on a regular set such because the Penn Treebank POS tags. For instance, within the sentence “The canine runs quick,” “canine” could be tagged as a noun (NN), “runs” as a verb (VBZ), and “quick” as an adverb (RB).
- POS tagging assigns labels to phrases in a sentence.
- Tagging helps analyze the syntax of the sentence and perceive phrase features in context.
With NLTK, you may carry out POS tagging utilizing the pos_tag operate, which tags every phrase in an inventory of tokens with its a part of speech. Within the following instance, we first tokenize the textual content after which use NLTK’s pos_tag operate to assign POS tags to every phrase.
from nltk import pos_tag
# Tokenize the textual content into phrases textual content = “She enjoys enjoying soccer on weekends.”
# Tokenization (phrases) phrases = word_tokenize(textual content)
# POS tagging tagged_words = pos_tag(phrases) print(“Tagged Phrases:”, tagged_words) |
This could output:
Tagged Phrases: [(‘She’, ‘PRP’), (‘enjoys’, ‘VBZ’), (‘playing’, ‘VBG’), (‘soccer’, ‘NN’), (‘on’, ‘IN’), (‘weekends’, ‘NNS’), (‘.’, ‘.’)] |
POS tagging is important for understanding sentence construction and for duties that contain syntactic evaluation, reminiscent of named entity recognition (NER) and machine translation.
Named Entity Recognition (NER)
Named Entity Recognition (NER) is an NLP activity used to establish and classify named entities in a textual content, such because the names of individuals, organizations, areas, and dates. This method is important for understanding and extracting helpful data from textual content.
Right here is an instance:
from nltk import ne_chunk, pos_tag, phrase_tokenize
# Pattern textual content textual content = “We will go to the Eiffel Tower on our trip to Paris.”
# Tokenize the textual content into phrases phrases = word_tokenize(textual content)
# Half-of-speech tagging tagged_words = pos_tag(phrases)
# Named Entity Recognition named_entities = ne_chunk(tagged_words) print(“Named Entities:”, named_entities) |
On this case, NER helps extract geographical references, such because the landmark and the town.
Named Entities: (S We/PRP shall/MD go to/VB the/DT (ORGANIZATION Eiffel/NNP Tower/NNP) on/IN our/PRP$ trip/NN to/TO (GPE Paris/NNP) ./.) |
This may then be utilized in varied duties, reminiscent of summarizing articles, extracting data for data graphs, and extra.
Wrap-Up & Subsequent Steps
On this information, we’ve lined important ideas in pure language processing utilizing NLTK—from fundamental textual content preprocessing to barely extra concerned strategies like lemmatization, POS tagging, and named entity recognition.
So the place do you go from right here? As you proceed your NLP journey, listed here are a number of subsequent steps to contemplate:
- Work on easy textual content classification issues utilizing algorithms like logistic regression, assist vector machine, and Naive Bayes.
- Strive sentiment evaluation with instruments like VADER or by coaching your personal classifier.
- Dive deeper into subject modeling or textual content summarization duties.
- Discover different NLP libraries reminiscent of spaCy or Hugging Face’s Transformers for state-of-the-art fashions.
What would you wish to be taught subsequent? Tell us! Preserve coding!