A Light Introduction to Rust for Python Programmers
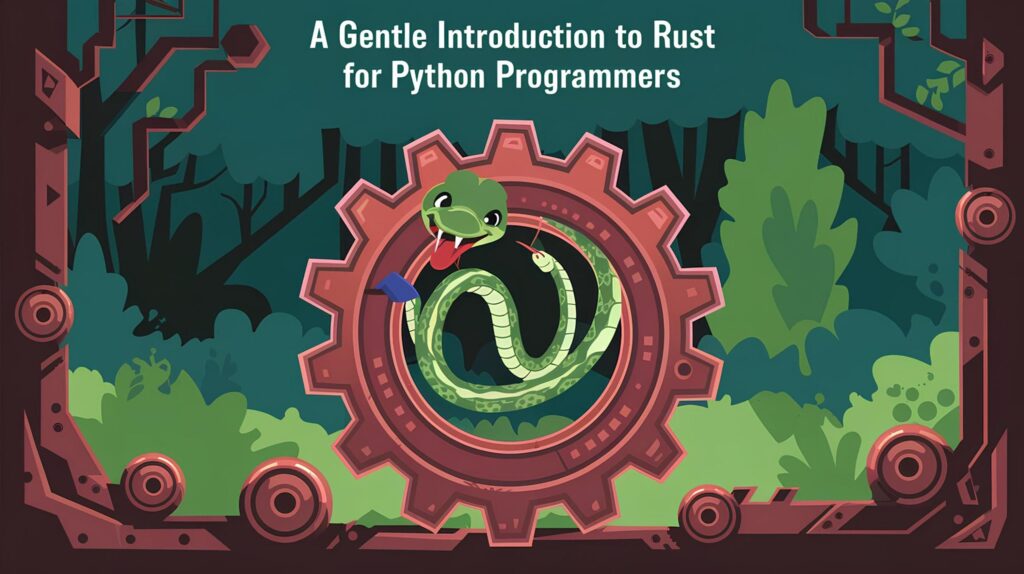

Picture by Editor (Kanwal Mehreen) | Ideogram.ai
Rust is a techniques programming language that emphasizes security and efficiency, making it a superb alternative for constructing quick, resource-efficient purposes. Whereas it affords low-level management over assets, it may be difficult for Python builders attributable to its handbook reminiscence administration. In contrast to Python, Rust doesn’t depend on a rubbish collector, and its design ensures each security and concurrency. This text will clarify Rust to Python programmers, highlighting the important thing variations and challenges.
Why Be taught Rust?
Listed here are some explanation why Python builders would possibly wish to be taught Rust:
- Efficiency: Rust is as quick as C. It’s good for duties like system programming, internet servers, and knowledge processing.
- Reminiscence Security: Rust’s possession system prevents reminiscence errors. It stops points like null pointer bugs and reminiscence leaks that occur in languages like C or C++.
- Concurrency: Rust handles multi-threading safely. Its possession system prevents knowledge races. This makes it nice for concurrent programming.
- Interoperability: Rust works effectively with Python. You may write the components that want excessive efficiency in Rust and use Python for the remainder. PyO3 and rust-cpython assist combine Rust with Python.
Setting Up Rust
First, you’ll want to put in it in your machine. You are able to do so utilizing the official Rust toolchain installer, rustup:
- Go to the official Rust website.
- Comply with the set up directions to your platform.
- Confirm the set up by working rustc –version within the terminal.
Whats up World
To begin with Rust, write a “Whats up World” program. Right here’s the way you do it:
fn foremost() {
println!("Whats up, World!");
}
Variables and Information Sorts
Rust requires specific sorts. Python is dynamically typed. In Rust, you declare variables with sorts. Here is the way it works:
Variables
In Rust, variables are immutable by default. To make a variable mutable, you utilize the mut key phrase.
fn foremost() {
let x = 5; // Immutable variable
println!("x is: {}", x);
let mut y = 10; // Mutable variable
println!("y is: {}", y);
y = 20; // This works as a result of y is mutable
println!("y is now: {}", y);
}
Information Sorts
Rust is statically typed. You need to specify sorts or let the compiler infer them. Listed here are some widespread Rust knowledge sorts:
- Integers: i32, u32, and so forth. for entire numbers.
- Floating-point numbers: f32, f64 for decimal values.
- Boolean: bool for true or false.
- Characters: char for a single character.
- Strings: String for textual content knowledge.
- Tuples: Group several types of values collectively.
- Arrays: Mounted-size collections of parts.
Management Circulation
Rust’s management stream statements (equivalent to if, else, and loop) work equally to Python, however there are just a few variations.
If Statements
In Rust, if expressions can return values:
fn foremost() {
let x = 10;
if x > 5 {
println!("x is bigger than 5");
} else {
println!("x is lower than or equal to five");
}
}
Loops
Rust supplies a number of loop constructs. The loop key phrase is an infinite loop, and you should use break and proceed to regulate the stream.
fn foremost() {
let mut counter = 0;
loop {
counter += 1;
if counter == 5 {
println!("Breaking out of the loop!");
break;
}
}
// Utilizing some time loop
let mut n = 0;
whereas n
Capabilities
Capabilities in Rust work like Python however have some variations:
- Return Kind: Rust requires you to specify the return sort explicitly.
- Parameters: You need to outline sorts for perform parameters in Rust.
- Operate Syntax: Rust makes use of fn to outline a perform.
fn foremost() {
let consequence = add(2, 3);
println!("2 + 3 = {}", consequence);
}
fn add(a: i32, b: i32) -> i32 {
a + b
}
Right here, the perform add takes two i32 integers as parameters and returns an i32 consequence. The -> i32 syntax specifies the return sort.
Error Dealing with
In Python, you utilize try to besides to deal with errors. In Rust, error dealing with is completed in another way:
- Rust makes use of Outcome for capabilities which may succeed or fail.
- Rust makes use of Possibility for values that may be there or may be lacking.
Outcome Kind
The Outcome sort is used for capabilities that may return an error. It has two variants: Okay for fulfillment and Err for failure. That you must explicitly deal with each instances to make sure your code works safely.
fn divide(x: i32, y: i32) -> Outcome {
if y == 0 {
Err("Can not divide by zero".to_string())
} else {
Okay(x / y)
}
}
fn foremost() {
match divide(10, 2) {
Okay(consequence) => println!("Outcome: {}", consequence),
Err(e) => println!("Error: {}", e),
}
}
Possibility Kind
The Possibility sort is used for instances the place a price would possibly or won’t be current. It has two variants: Some and None. That is helpful for capabilities that will not return a consequence.
fn find_number(arr: &[i32], goal: i32) -> Possibility {
for &num in arr.iter() {
if num == goal {
return Some(num); // Return the discovered quantity wrapped in Some
}
}
None // Return None if the quantity is just not discovered
}
fn foremost() {
let numbers = [1, 2, 3, 4, 5];
// Seek for a quantity that exists within the array
match find_number(&numbers, 3) {
Some(num) => println!("Discovered the quantity: {}", num),
None => println!("Quantity not discovered"),
}
// Seek for a quantity that doesn't exist within the array
match find_number(&numbers, 6) {
Some(num) => println!("Discovered the quantity: {}", num),
None => println!("Quantity not discovered"),
}
}
Conclusion
Rust is a programming language designed for security, efficiency, and concurrency, providing extra management over reminiscence and duties in comparison with Python. Whereas studying Rust may be difficult initially, it excels in high-performance purposes. Rust additionally integrates effectively with Python, permitting you to make use of Python for common duties and Rust for performance-critical components.
With clear guidelines for variables, knowledge sorts, and capabilities, it successfully manages errors and ensures protected concurrency. Though totally different from Python, it supplies important benefits for particular duties. Begin with small packages to get snug with its syntax and options.
Studying Rust will help you write safer, quicker code. Completely happy coding!
Jayita Gulati is a machine studying fanatic and technical author pushed by her ardour for constructing machine studying fashions. She holds a Grasp’s diploma in Laptop Science from the College of Liverpool.