Getting Began with Constructing RAG Programs Utilizing Haystack
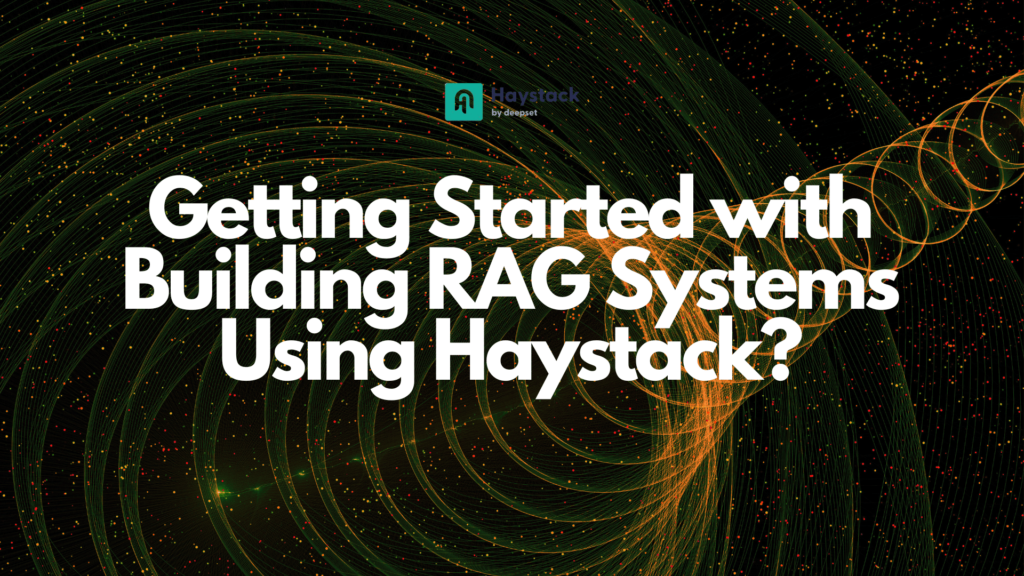

Picture by Writer
Introduction
Massive language fashions, or LLMs for brief, have been a sensational subject up to now few months, with a number of LLMs being constructed and deployed day by day. These fashions, constructed on an unlimited quantity of information, have a outstanding capability to know and generate human-like textual content, making them invaluable for varied duties and even advanced decision-making.
They’ve eased beforehand daunting challenges, whether or not breaking language obstacles with seamless translations or serving to companies ship personalised buyer help at lightning velocity. Past simply comfort, LLMs have opened doorways to innovation in ways in which have been exhausting to think about a decade in the past.
Nevertheless, LLMs aren’t all-knowing oracles. Their data comes from huge quantities of textual content information used throughout coaching, however that information has its limits—each in scope and freshness. Think about making an attempt to fill an encyclopedia with the whole lot in regards to the world however stopping earlier than at the moment’s newest information. Naturally, gaps will emerge, resulting in blind spots, and the LLM will battle to offer significant solutions.
These gaps are significantly noticeable once we ask questions that depend on present occasions, very particular area experience, or experiences exterior the LLM’s dataset. Let’s check out this instance, ChatGPT would possibly stumble when requested in regards to the newest developments in AI post-2023. It is not that the mannequin is “ignorant” within the human sense; somewhat, it’s like asking somebody who hasn’t learn the most recent ebook or studied a uncommon topic; they merely don’t have that data to attract from.
To resolve this downside, LLMS wants up-to-date coaching and supplemental sources, like related databases or search capabilities, to bridge these gaps. This led to the arrival of Retrieval-Augmented Technology (RAG), a captivating strategy that mixes the ability of knowledge retrieval with the creativity of generative fashions. At its core, RAG combines the ability of pre-trained language fashions with a retrieval system to offer extra correct, context-aware solutions.
Think about you’re utilizing a big language mannequin to search out particulars a few area of interest subject, however the mannequin doesn’t have all of the up-to-date data you want. As an alternative of relying solely on its pre-trained data, RAG retrieves related data from an exterior database or paperwork after which makes use of that to craft a well-informed, coherent response. This mix of retrieval and technology ensures that responses aren’t solely inventive but additionally grounded in real-time information or particular sources.
Are you aware what’s extra thrilling about RAG? It has the potential to adapt in real-time. As an alternative of working with static data, it could fetch essentially the most up-to-date or particular data wanted for a process from trusted sources whereas lowering the chance of hallucination.
There are three essential phases in RAG, retrieval, augmentation, and technology. Let’s break these down one after the other.
Retrieval Course of
This is step one the place we dive into the search. Think about you are searching for solutions in an unlimited library—earlier than leaping to conclusions, you first want to assemble the suitable books. Within the RAG, this implies querying a big database or data base to fetch related items of knowledge. These could possibly be paperwork, articles, or every other type of information that may make clear the query or process at hand. The aim right here is to retrieve essentially the most pertinent data that can enable you to construct a stable basis for the following steps.
Augmentation Course of
As soon as we have now the related data, it is time to improve it. This implies refining the information we retrieved, reformatting it, and even combining a number of sources to kind a extra complete and detailed reply. The retrieved information is not at all times excellent by itself, so it could want some tweaks or extra context to be actually helpful.
The augmentation course of can contain filtering out irrelevant data, merging info, or rephrasing to make the information extra digestible. That is the place we begin shaping the uncooked data into one thing extra significant.
Technology Course of
Now that we have now the improved information, it’s time to generate the ultimate output. With the augmented data, we use language fashions (like GPT) to craft a response or an output that immediately solutions the question or solves the issue. This step ensures the reply is coherent, human-like, and related.
Within the upcoming sections of this text, we are going to get hands-on in constructing a RAG system utilizing a highly regarded device known as Haystack.
What’s Haystack?
Haystack, constructed by Deepset AI, is an open-source framework for constructing production-ready LLM purposes, retrieval-augmented generative pipelines, and state-of-the-art search programs that work intelligently over massive doc collections.

Picture by Writer
With use circumstances spanning multimodal AI, conversational AI, content material technology, agentic pipelines, and superior RAG. Haystack is modularly designed to be able to combine the perfect applied sciences from OpenAI, Chroma, Marqo, and different open-source tasks like Hugging Face’s Transformers or Elasticsearch.
Haystack’s cutting-edge LLMs and NLP fashions could also be used to create personalised search experiences and permit your customers to question in pure language. The latest launch of Haystack 2.0 has introduced a serious replace to the design of Haystack parts, Doc Shops, and pipelines.
Preparation
Conditions
- Python 3.8 or larger
- Haystack 2.0
- OpenAI
Set up
We are able to set up Haystack by way of both conda or pip.
Utilizing pip:
Utilizing conda:
conda config --add channels conda-forge/label/haystack-ai_rc
conda set up haystack-ai
Import Libraries
Earlier than diving into the code, obligatory libraries and modules are imported. These embody os
for surroundings variables, Pipeline
and PredefinedPipeline
from Haystack to create and use pipelines, and urllib.request
to deal with file downloads.
import os
from haystack import Pipeline, PredefinedPipeline
import urllib.request
Set the API Key & Obtain the Information
On this step, the OpenAI API secret’s set as an surroundings variable, and a pattern textual content file (containing details about Leonardo da Vinci) is downloaded to function enter information for indexing.
os.environ["OPENAI_API_KEY"]
units up authentication for the LLM used within the pipeline- urllib.request.urlretrieve downloads the file davinci.txt from an internet supply and saves it domestically
os.environ["OPENAI_API_KEY"] = "Your OpenAI API Key"
urllib.request.urlretrieve("https://archive.org/stream/leonardodavinci00brocrich/leonardodavinci00brocrich_djvu.txt","davinci.txt")
Creating Our RAG System
Create and Run an Indexing Pipeline
Right here, a predefined indexing pipeline is created and executed. The indexing pipeline processes the davinci.txt file, making its content material searchable for future queries.
Pipeline.from_template(PredefinedPipeline.INDEXING)
initializes a pipeline for indexing information.run(information={"sources": ["davinci.txt"]})
processes the enter textual content file to index its content material
indexing_pipeline = Pipeline.from_template(PredefinedPipeline.INDEXING)
indexing_pipeline.run(information={"sources": ["davinci.txt"]})
Create the RAG Pipeline
This step initializes the RAG pipeline, which is designed to retrieve related data from the listed information and generate a response utilizing an LLM.
Question the Pipeline and Generate a Response
A question is handed to the RAG pipeline, which retrieves related data and generates a response.
question
shops the query you wish to replyrag_pipeline.run(information={"prompt_builder": {"question":question}, "text_embedder": {"textual content": question}})
sends the question by way of the pipelineprompt_builder
specifies the question to be answeredtext_embedder
helps create embeddings for the enter questionend result["llm"]["replies"][0]
extracts and prints the LLM-generated reply
question = "How previous was he when he died?"
end result = rag_pipeline.run(information={"prompt_builder": {"question":question}, "text_embedder": {"textual content": question}})
print(end result["llm"]["replies"][0])
Full code:
import os
from haystack import Pipeline, PredefinedPipeline
import urllib.request
os.environ["OPENAI_API_KEY"] = "Your OpenAI Key"
urllib.request.urlretrieve("https://archive.org/stream/leonardodavinci00brocrich/leonardodavinci00brocrich_djvu.txt",
"davinci.txt")
indexing_pipeline = Pipeline.from_template(PredefinedPipeline.INDEXING)
indexing_pipeline.run(information={"sources": ["davinci.txt"]})
rag_pipeline = Pipeline.from_template(PredefinedPipeline.RAG)
question = "How previous was he when he died?"
end result = rag_pipeline.run(information={"prompt_builder": {"question":question}, "text_embedder": {"textual content": question}})
print(end result["llm"]["replies"][0])
Output:
Leonardo da Vinci was born in 1452 and died on Might 2, 1519. Due to this fact, he was 66 years previous when he handed away.
Conclusion
On this article, we explored step-by-step tips on how to construct a Retrieval-Augmented Technology (RAG) pipeline utilizing Haystack. We began by importing important libraries and establishing the surroundings, together with the OpenAI API key for the language mannequin integration. Subsequent, we demonstrated tips on how to obtain a textual content file containing details about Leonardo da Vinci, which served as the information supply for our pipeline.
The walkthrough then lined the creation and execution of an indexing pipeline to course of and retailer the textual content information, enabling it to be searched effectively. We adopted that with the setup of a RAG pipeline designed to mix retrieval and language technology seamlessly. Lastly, we confirmed tips on how to question the RAG pipeline with a query about Leonardo da Vinci’s age on the time of his loss of life and retrieved the reply—66 years previous.
This hands-on information not solely defined how the RAG course of works but additionally walked you thru sensible steps to implement it.
Assets
Shittu Olumide is a software program engineer and technical author obsessed with leveraging cutting-edge applied sciences to craft compelling narratives, with a eager eye for element and a knack for simplifying advanced ideas. You may also discover Shittu on Twitter.