Methods to Write Clear Python Code as a Newbie
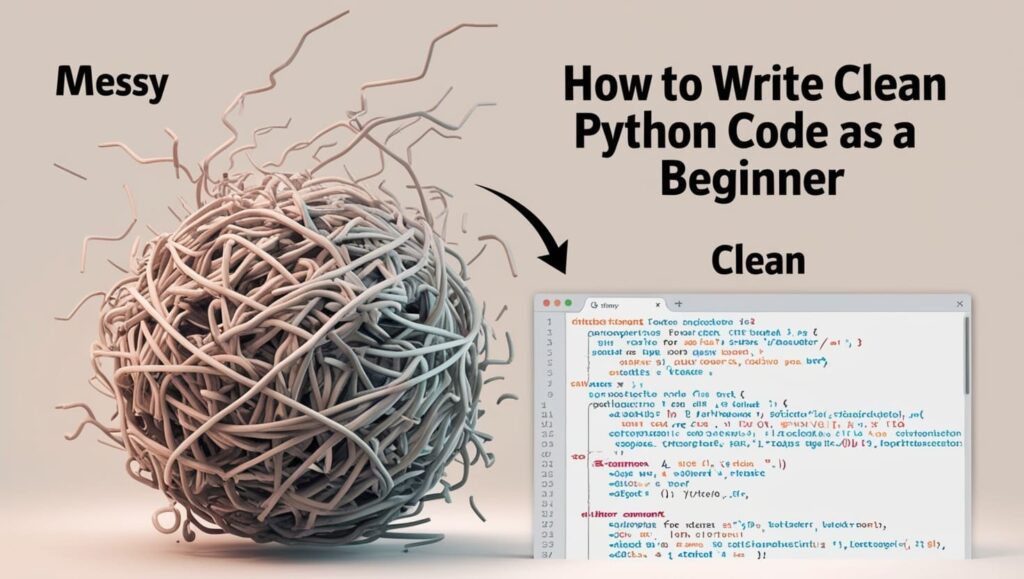
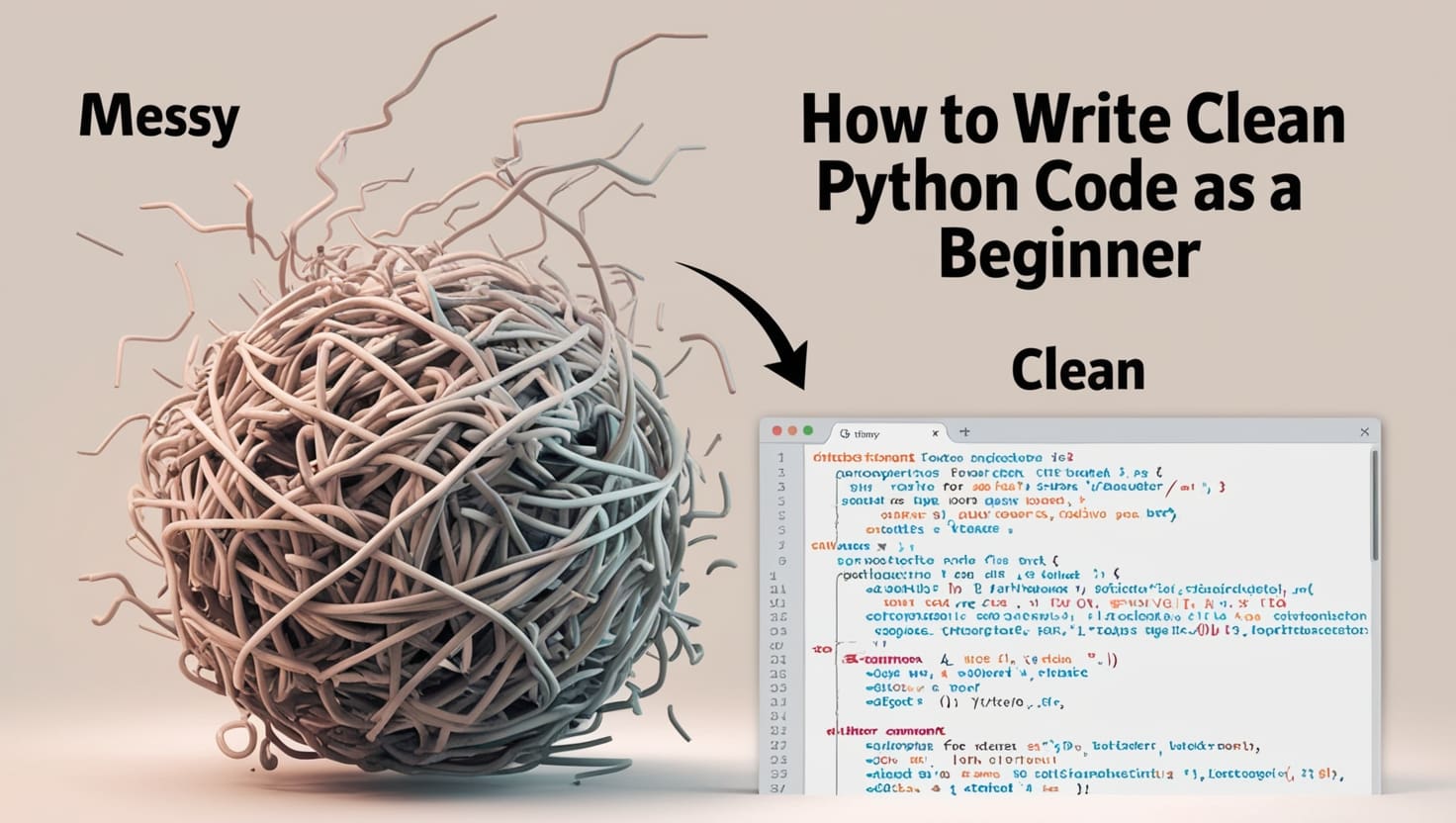
Picture by Creator| Leonardo.ai
Python is a good newbie language—easy and clear. However as you get extra snug with it, it is easy to slide into the dangerous behavior of writing convoluted, unreadable code that may be a developer’s worst nightmare to debug. Clear code is not nearly making it nice to search for another person—it’s going to prevent a ton of complications when you must cope with it once more. Take my phrase on this, taking the time to put in writing neat code will make your personal life a hell of so much simpler in the long term.
On this article, we’ll have a look at some suggestions and finest practices that may allow you to put in writing clear and readable Python code even if you’re a newbie.
Key Practices for Writing Clear, Newbie-Pleasant Python Code
1. Comply with the PEP 8 Model Information
PEP 8 is the principle fashion information for Python, and it offers conventions for writing clear and readable code. Listed here are a number of necessary factors made in PEP 8:
- Use 4 areas per indentation degree:
- Restrict line size to 79 characters: Preserve your code readable by not writing overly lengthy traces. If a line will get too lengthy, break it into smaller elements utilizing parentheses or backslashes.
- Use clean traces to separate code blocks: Add clean traces between capabilities or courses to enhance readability:
- Use snake_case for variable and performance names: Variables and capabilities must be lowercase with phrases separated by underscores:
def greet():
print("Hiya, World!") # Indented with 4 areas
def add(a, b):
return a + b
def subtract(a, b):
return a - b
my_variable = 10
def calculate_average(numbers):
return sum(numbers) / len(numbers)
2. Use Significant Names for Variables and Capabilities
Don’t use obscure or one-letter names (like `x` or `y` if it isn’t for easy and brief loops. This isn’t helpful to the code. As a substitute, give names that specify what the variable or the perform does.
- Dangerous Instance:
- Good Instance:
def a(x, y):
return x + y
def add_numbers(first_number, second_number):
return first_number + second_number
3. Use Clear Feedback (However Do not Overdo It)
Feedback justify why your code does one thing however not what it does. In case your codebase is clear and doesn’t include obscure naming, you wouldn’t must remark as a lot. The code ought to, in precept, converse for itself! Nonetheless, when mandatory, use feedback to make clear your intentions.
4. Preserve Capabilities Quick and Centered
A perform ought to do one factor and do it properly. If a perform is simply too lengthy or handles a number of duties, take into account breaking it into smaller capabilities.
5. Deal with Errors Gracefully
As a newbie, it’s tempting to skip error dealing with, however it’s an necessary a part of writing good code. Use attempt to besides blocks to deal with potential errors.
strive:
quantity = int(enter("Enter a quantity: "))
print(f"The quantity is {quantity}")
besides ValueError:
print("That’s not a sound quantity!")
This ensures your program doesn’t crash unexpectedly.
6. Keep away from Hardcoding Values
Hardcoding values (e.g., numbers or strings) immediately into your code could make it tough to replace or reuse. As a substitute, use variables or constants.
- Dangerous Instance:
- Good Instance:
print("The overall value with tax is: $105") # Hardcoded tax and whole value
PRICE = 100 # Base value of the product
TAX_RATE = 0.05 # 5% tax charge
# Calculate the overall value
total_price = PRICE + (PRICE * TAX_RATE)
print(f"The overall value with tax is: ${total_price:.2f}")
This makes your code extra versatile and straightforward to switch.
7. Keep away from World Variables
Counting on world variables could make your code tougher to know and debug. As a substitute, encapsulate state inside courses or capabilities.
- Dangerous Instance (utilizing a world variable):
- Good Instance (utilizing a category):
whole = 0
def add_to_total(worth):
world whole
whole += worth
class Calculator:
def __init__(self):
self.whole = 0
def add_value(self, worth):
self.whole += worth
Encapsulating knowledge inside objects or capabilities ensures that your code is modular, testable, and fewer error-prone.
8. Use f-Strings for String Formatting
f-Strings (launched in Python 3.6) are a clear and readable strategy to format strings.
- Dangerous Instance (concatenating strings):
- Good Instance (utilizing f-strings):
title = "Alice"
age = 25
print("My title is " + title + " and I'm " + str(age) + " years outdated")
title = "Alice"
age = 25
print(f"My title is {title} and I'm {age} years outdated")
f-Strings are usually not solely extra readable but additionally extra environment friendly than different string formatting strategies.
9. Use Constructed-in Capabilities and Libraries
Python comes with many highly effective built-in options. Use these to put in writing environment friendly and correct code as a substitute of coding it from scratch.
- Dangerous Instance (manually discovering the utmost):
- Good Instance (utilizing max):
def find_max(numbers):
max_number = numbers[0]
for num in numbers:
if num > max_number:
max_number = num
return max_number
def find_max(numbers):
return max(numbers)
10. Use Pythonic Code
“Pythonic” code refers to writing code that takes benefit of Python’s simplicity and readability. Keep away from overly advanced or verbose options when less complicated choices can be found.
- Dangerous Instance:
- Good Instance:
numbers = [1, 2, 3, 4, 5]
doubled = []
for num in numbers:
doubled.append(num * 2)
numbers = [1, 2, 3, 4, 5]
doubled = [num * 2 for num in numbers]
Utilizing record comprehensions, built-in capabilities, and readable idioms makes your code extra elegant.
11. Use Model Management
Whilst a newbie, it’s a good suggestion to start out utilizing instruments like Git for model management. It means that you can monitor modifications, collaborate with others, and keep away from shedding progress if one thing goes improper.
Study the fundamentals of Git:
- Save your code with git add and git commit
- Experiment with out concern, realizing you’ll be able to revert to a earlier model.
12. Construction Your Mission Nicely
As your codebase grows, organizing your information and directories turns into important. A well-structured mission makes it simpler to navigate, debug, and scale your code.
Right here is an instance of a regular mission construction:
my_project/
├── README.md # Mission documentation
├── necessities.txt # Mission dependencies
├── setup.py # Package deal configuration for distribution
├── .gitignore # Git ignore file
├── src/ # Primary supply code listing
│ └── my_project/ # Your package deal listing
│ ├── __init__.py # Makes the folder a package deal
│ ├── predominant.py # Primary utility file
│ ├── config.py # Configuration settings
│ └── constants.py # Mission constants
├── checks/ # Take a look at information
│ ├── __init__.py
│ ├── test_main.py
│ └── test_utils.py
├── docs/ # Documentation information
│ ├── api.md
│ └── user_guide.md
└── scripts/ # Utility scripts
└── setup_db.py
A structured method ensures your mission stays clear and manageable because it grows in dimension.
13. Take a look at Your Code
All the time take a look at your code to make sure it really works as anticipated. Even easy scripts can profit from testing. For automated testing, learners can begin with the built-in unittest module.
import unittest
def add_numbers(a, b):
return a + b
class TestAddNumbers(unittest.TestCase):
def test_add(self):
self.assertEqual(add_numbers(2, 3), 5)
self.assertEqual(add_numbers(-1, 1), 0)
if __name__ == "__main__":
unittest.predominant()
Testing helps you catch bugs early and ensures your code works accurately.
Keep in mind:
- Write code for people, not simply computer systems
- Preserve it easy
- Keep constant together with your fashion
- Take a look at your code frequently
- Refactor when wanted
Wrapping Up
Do it little by little, carry on studying, and shortly clear code will probably be your second nature. This text is for full Python newbies. To be taught superior methods on the way to write higher maintainable code in Python, learn my article: Mastering Python: 7 Strategies For Writing Clear, Organized, and Efficient Code
Kanwal Mehreen Kanwal is a machine studying engineer and a technical author with a profound ardour for knowledge science and the intersection of AI with medication. She co-authored the e book “Maximizing Productiveness with ChatGPT”. As a Google Technology Scholar 2022 for APAC, she champions range and educational excellence. She’s additionally acknowledged as a Teradata Range in Tech Scholar, Mitacs Globalink Analysis Scholar, and Harvard WeCode Scholar. Kanwal is an ardent advocate for change, having based FEMCodes to empower ladies in STEM fields.