Newbie’s Information to Unit Testing Python Code with PyTest
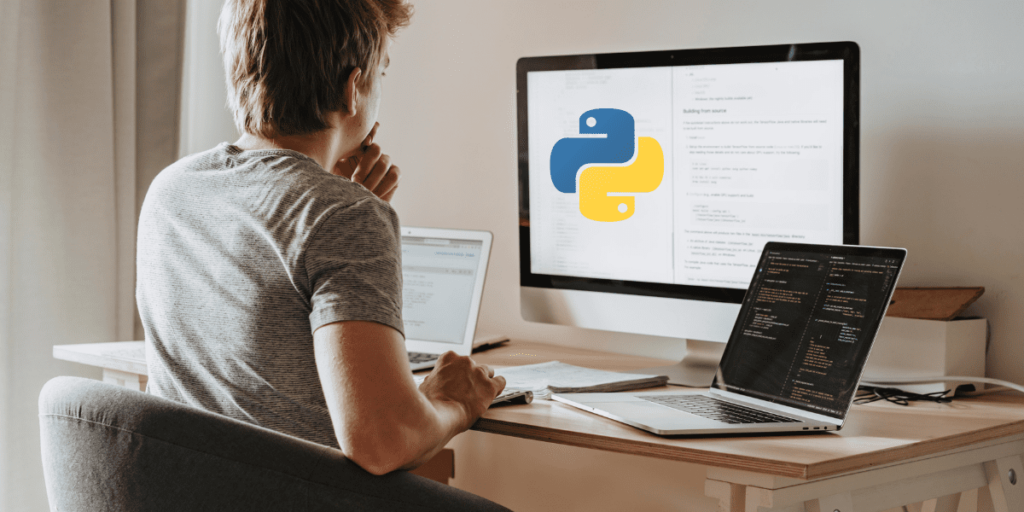
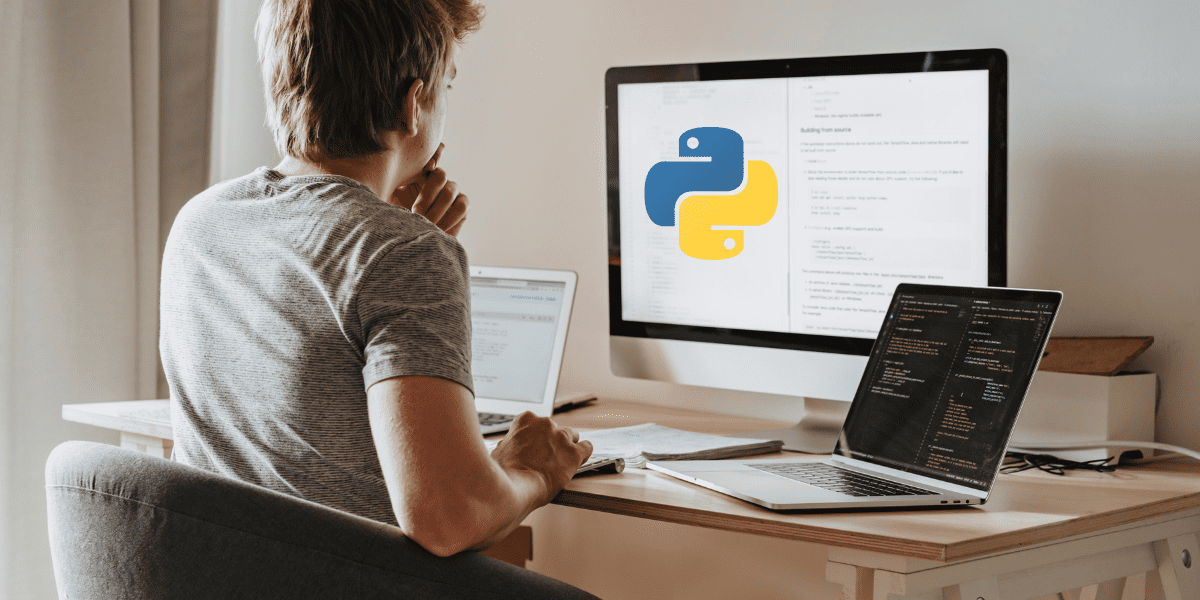
Picture by Creator | Created on Canva
In software program growth, unit testing is vital to examine that particular person parts of your code work accurately. At its core, unit testing is the observe of testing particular person models—features, strategies, courses—of your code to make sure they work as anticipated. Consider it as zooming in on small sections of your code and asking, “Does this piece of code do what it’s presupposed to?”
Good unit assessments assist you make sure that bugs are caught early, code stays maintainable, and you may refactor with out introducing new points. On this tutorial, we’ll do the next:
- Construct a easy TO-DO record supervisor
- Write unit assessments for various functionalities utilizing PyTest
- Run assessments and interpret the outcomes
Let’s get began.
Setting Up the Challenge
▶️ You will discover the code for this tutorial on GitHub.
Earlier than we leap into coding, let’s arrange a clear folder construction for our mission. It will assist arrange the code and assessments correctly. Right here’s the beneficial folder construction:
todo_project/
│
├── todo/
│ ├── __init__.py
│ └── todo_manager.py
│
├── assessments/
│ ├── __init__.py
│ └── test_todo_manager.py
│
└── necessities.txt
Right here’s a fast breakdown:
todo/
: This folder accommodates the core software logic: theToDoManager
class that we’ll write. We additionally add an empty__init__.py
file so we are able to import and use features and courses on this module.assessments/
: This folder holds all of the check recordsdata. We’ll place our unit assessments right here, maintaining them separate from the principle code.
In case you have an inventory of dependencies, you may create a necessities.txt file and set up the libraries in a digital atmosphere like so:
For this tutorial, we solely want PyTest. So you may create and activate a digital atmosphere:
$ python3 -m venv v1
$ supply v1/bin/activate
Then set up PyTest utilizing pip:
Now that we’ve put in PyTest, let’s begin coding!
Creating the To-Do Checklist Supervisor
We’ll now construct the To-Do record app. The ToDoManager
class permits customers so as to add duties, take away duties, mark them as accomplished, and retrieve duties primarily based on their completion standing:
# todo/todo_manager.py
class ToDoManager:
def __init__(self):
self.duties = []
def add_task(self, activity):
if not activity:
elevate ValueError("Job can't be empty.")
self.duties.append({"activity": activity, "accomplished": False})
return activity
def remove_task(self, activity):
for t in self.duties:
if t["task"] == activity:
self.duties.take away(t)
return activity
elevate ValueError("Job not discovered.")
def mark_completed(self, activity):
for t in self.duties:
if t["task"] == activity:
t["completed"] = True
return activity
elevate ValueError("Job not discovered.")
def get_tasks(self, accomplished=None):
if accomplished is None:
return self.duties
return [t for t in self.tasks if t["completed"] == accomplished]
The add_task
methodology provides a brand new activity to the record. It raises an error if the duty is empty. The remove_task
methodology removes a activity from the record. Raises an error if the duty doesn’t exist.
The mark_completed
marks a activity as accomplished. It raises an error if the duty isn’t discovered. The get_tasks(accomplished=None)
methodology retrieves duties from the record. You’ll be able to filter by accomplished or pending duties utilizing the accomplished
parameter.
Writing Unit Checks with PyTest
Now that we now have a working class, it’s time to write down unit assessments for it. We are going to write our assessments within the assessments/test_todo_manager.py
file. Let’s write assessments for every methodology in our ToDoManager
class.
We create a fixture that initializes the ToDoManager
earlier than every check. This ensures that every check has a clear occasion of the category and prevents unwanted side effects.
# assessments/test_todo_manager.py
import pytest
from todo.todo_manager import ToDoManager
@pytest.fixture
def todo_manager():
return ToDoManager()
It’s now time to write down the check instances—every check case specializing in one methodology:
Technique | Description |
test_add_task | Checks if duties are added accurately |
test_add_empty_task | Ensures that an empty activity raises an error |
test_remove_task | Checks if a activity may be efficiently eliminated |
test_remove_nonexistent_task | Checks if an error is raised when attempting to take away a non-existent activity |
test_mark_completed | Checks if duties may be marked as accomplished |
test_get_tasks | Retrieves all duties and filters accomplished and pending duties |
# assessments/test_todo_manager.py
import pytest
from todo.todo_manager import ToDoManager
...
def test_add_task(todo_manager):
todo_manager.add_task("Purchase groceries")
assert todo_manager.duties == [{"task": "Buy groceries", "completed": False}]
def test_add_empty_task(todo_manager):
with pytest.raises(ValueError, match="Job can't be empty."):
todo_manager.add_task("")
def test_remove_task(todo_manager):
todo_manager.add_task("Purchase groceries")
todo_manager.remove_task("Purchase groceries")
assert todo_manager.duties == []
def test_remove_nonexistent_task(todo_manager):
with pytest.raises(ValueError, match="Job not discovered."):
todo_manager.remove_task("Do laundry")
def test_mark_completed(todo_manager):
todo_manager.add_task("Go for a stroll")
todo_manager.mark_completed("Go for a stroll")
assert todo_manager.duties == [{"task": "Go for a walk", "completed": True}]
def test_get_tasks(todo_manager):
todo_manager.add_task("Job 1")
todo_manager.add_task("Job 2")
todo_manager.mark_completed("Job 1")
all_tasks = todo_manager.get_tasks()
completed_tasks = todo_manager.get_tasks(accomplished=True)
pending_tasks = todo_manager.get_tasks(accomplished=False)
assert len(all_tasks) == 2
assert completed_tasks == [{"task": "Task 1", "completed": True}]
assert pending_tasks == [{"task": "Task 2", "completed": False}]
Working the Checks
Let’s now run the assessments. Navigate to the basis of your mission (right here todo_project
) and run the next command:
PyTest will auto-discover the assessments within the assessments/
folder and execute them. If the whole lot is appropriate, it’s best to see output like this:
============================= check session begins ==============================
platform linux -- Python 3.11.4, pytest-8.3.3, pluggy-1.5.0
rootdir: /dwelling/balapriya/pytest_t
collected 6 objects
assessments/test_todo_manager.py ...... [100%]
============================== 6 handed in 0.02s ===============================
This implies all our assessments handed efficiently!
Wrapping Up
Unit assessments help you catch bugs early within the growth cycle—saving time and sources. Once you make modifications or refactor code, unit assessments make sure that the code works as anticipated. Writing unit assessments forces you to design your code in a modular and testable approach.
On this tutorial, we walked by constructing a To-Do record app and writing unit assessments utilizing PyTest. By following finest practices for organizing your mission, you may scale your software and assessments because it grows. Unit testing, particularly with PyTest, makes your code extra strong, maintainable, and bug-free.
Completely satisfied unit testing!
Bala Priya C is a developer and technical author from India. She likes working on the intersection of math, programming, knowledge science, and content material creation. Her areas of curiosity and experience embody DevOps, knowledge science, and pure language processing. She enjoys studying, writing, coding, and low! At the moment, she’s engaged on studying and sharing her data with the developer group by authoring tutorials, how-to guides, opinion items, and extra. Bala additionally creates participating useful resource overviews and coding tutorials.