Visualizing Knowledge Instantly from Numpy Arrays
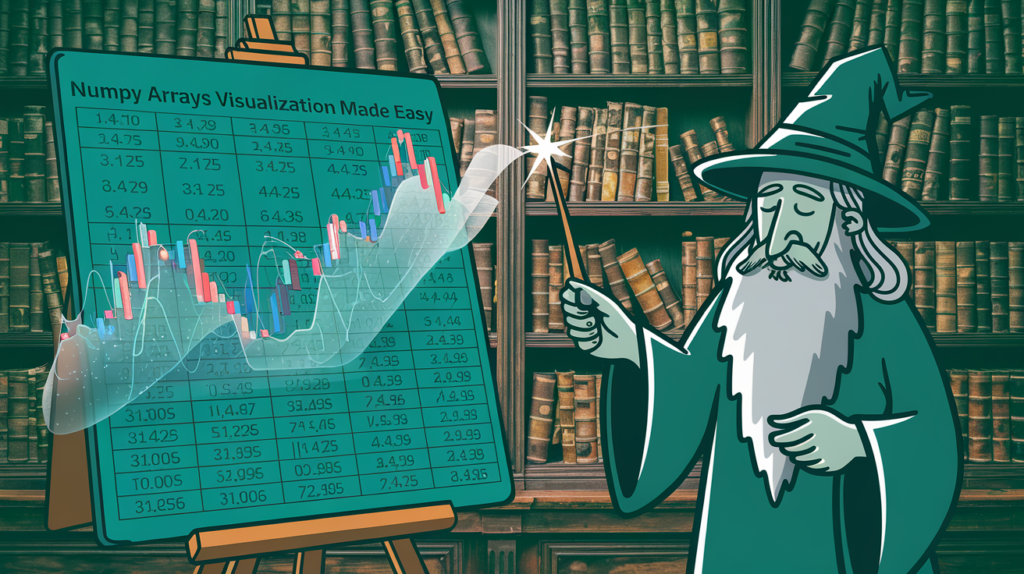

Picture by Writer | Ideogram
This Python tutorial covers sensible step-by-step examples of visualizing information contained in NumPy, a standard Python information construction to effectively deal with massive datasets.
The tutorial showcases various kinds of information visualizations utilizing a well-liked plotting library: matplotlib. This library gives intuitive instruments to plot, customise, and interpret information, facilitating perception drawing from NumPy arrays. If you’re on the lookout for DIY examples for buying a fast basis for visualizing information in Python, this tutorial is for you.
Tutorial Examples
To hold out the beneath examples of visualizing information contained in NumPy arrays, you may solely must import two libraries at the beginning of your Python script or program: NumPy and matplotlib.
import numpy as np
import matplotlib.pyplot as plt
Let’s dive now into the real-world information examples we have ready for you.
Visualizing 1D Knowledge: Inventory Costs Over Time
Our first instance visualizes day by day inventory costs over a month (30 days) utilizing a easy line plot.
days = np.arange(1, len(stock_prices) + 1)
# Array of day by day inventory costs (30 components)
stock_prices = [102.5, 105.2, 103.8, 101.9, 104.7, 106.3, 107.1, 105.5,
108.2, 109.0, 107.8, 106.5, 108.9, 109.5, 110.2, 109.8,
111.5, 112.3, 110.9, 113.1, 111.8, 114.2, 113.5, 115.0,
114.7, 116.2, 115.8, 117.5, 116.9, 118.1]
# Plot the array in a line plot
plt.plot(days, stock_prices)
plt.xlabel('Day')
plt.ylabel('Worth ($)')
plt.title('Inventory Costs Over Time')
plt.present()
The above code creates two NumPy arrays: one referred to as days
, containing the times of the month (used for the x-axis of the plot), and the principle information array stock_prices
containing the values to characterize (y-axis). When these two arrays are handed as arguments to plt.plot()
, by default matplotlib builds a easy line plot. Extra attributes could be optionally set so as to add axes titles and a plot title. This easy strategy is good to visualise time collection information contained in a NumPy array.
Output:
Alternatively, for experiment functions, you may generate your 1D array of inventory costs randomly, as follows:
days = np.arange(1, 31)
stock_prices = np.random.regular(100, 5, measurement=days.form)
Visualizing Two 1D Knowledge Arrays: Top vs. Weight
Suppose we now have two information variables collected from 100 people: their peak in cm and their weight in kg, every saved in a separate NumPy array. If needed to visualise these two variables collectively -for occasion, to research correlations-, a scatter plot is the answer.
This instance randomly generates two arrays: peak
, and weight
, of measurement 100 every. It then makes use of matplotlib’s scatter
technique to create a scatter plot upon each arrays.
peak = np.random.regular(170, 10, 100) # Random heights generated utilizing a traditional distribution with imply 170 and stdev 10
weight = np.random.regular(70, 8, 100) # Random heights generated utilizing a traditional distribution with imply 70 and stdev 8
plt.scatter(peak, weight)
plt.xlabel('Top (cm)')
plt.ylabel('Weight (kg)')
plt.title('Top vs. Weight')
plt.present()
Output:
Visualizing a 2D array: temperatures throughout places
Suppose you have got collected temperature recordings over a variety of 10 equidistant latitudes and 10 equidistant longitudes in an oblong space. As an alternative of utilizing 1D NumPy arrays, it’s extra acceptable to make use of one 2D NumPy array for these information. The beneath instance reveals learn how to visualize this “information grid” of temperatures utilizing a heatmap: an fascinating kind of visualization that maps information values to colours in an outlined colour scale. For simplicity, the temperature information are generated randomly, following a uniform distribution with values between 15ºC and 30ºC.
# 2D information grid: 10x10 temperature recordings over an oblong space
temperatures = np.random.uniform(low=15, excessive=30, measurement=(10, 10)) # Temperature in °C
plt.imshow(temperatures, cmap='scorching', interpolation='nearest')
plt.colorbar(label="Temperature (°C)")
plt.title('Temperature Heatmap')
plt.present()
Be aware that plt.imshow
is used to create the heatmap, specifying the 2D array to visualise, a particular colour scale (within the instance, ‘scorching’), and an interpolation technique, crucial when the info granularity and the picture decision differ.
Output:
Visualizing A number of 1D Arrays: Monetary Time Sequence
Again to the primary instance about inventory costs, let’s suppose we now have three totally different monetary shares and need to visualize their day by day evolution collectively in a easy plot. If every inventory time collection is contained in a 1D array of equal measurement, the method is just not very totally different from what we did earlier.
days = np.arange(1, 31)
stock_A = np.random.regular(100, 5, measurement=days.form)
stock_B = np.random.regular(120, 10, measurement=days.form)
stock_C = np.random.regular(90, 8, measurement=days.form)
plt.plot(days, stock_A, label="Inventory A")
plt.plot(days, stock_B, label="Inventory B")
plt.plot(days, stock_C, label="Inventory C")
plt.xlabel('Day')
plt.ylabel('Worth ($)')
plt.title('Inventory Costs Over Time')
plt.legend()
plt.present()
The distinction? We invoke plt.plot
three consecutive occasions, as soon as for every inventory time collection. This does not generate three plots. Matplotlib creates the plot within the ultimate instruction plt.present()
: all the things in earlier directions is like “artifacts” that can be added to the ensuing visualization.
Output:
Wrapping Up
Via 4 insightful examples of various complexity, this tutorial has illustrated learn how to simply visualize various kinds of information contained in NumPy arrays utilizing a number of visualization strategies, from less complicated instruments like the road plot to extra refined approaches like heatmaps.
Iván Palomares Carrascosa is a pacesetter, author, speaker, and adviser in AI, machine studying, deep studying & LLMs. He trains and guides others in harnessing AI in the true world.