Constructing Your First Chatbot: A Palms-On Tutorial with Open-Supply Instruments
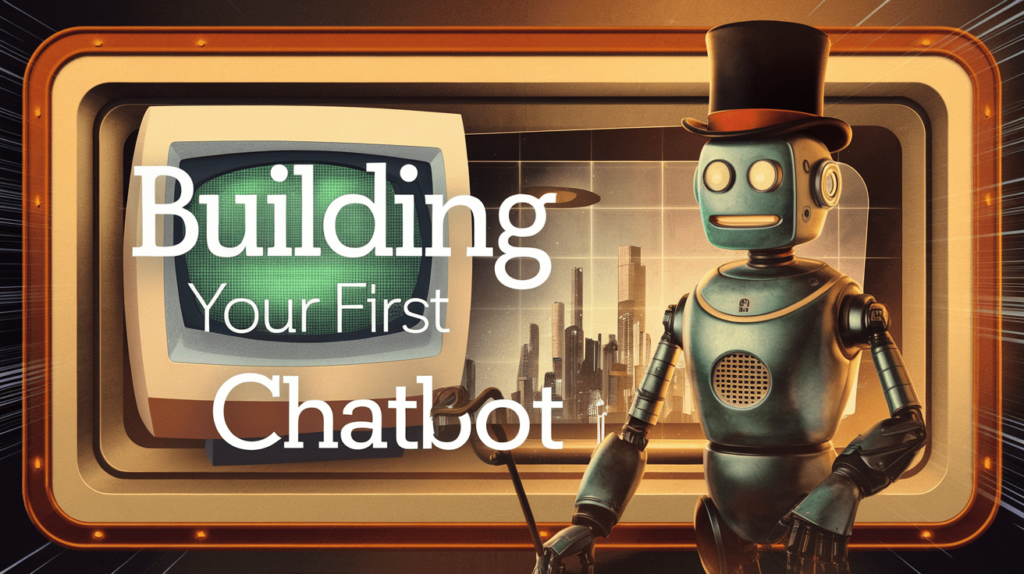
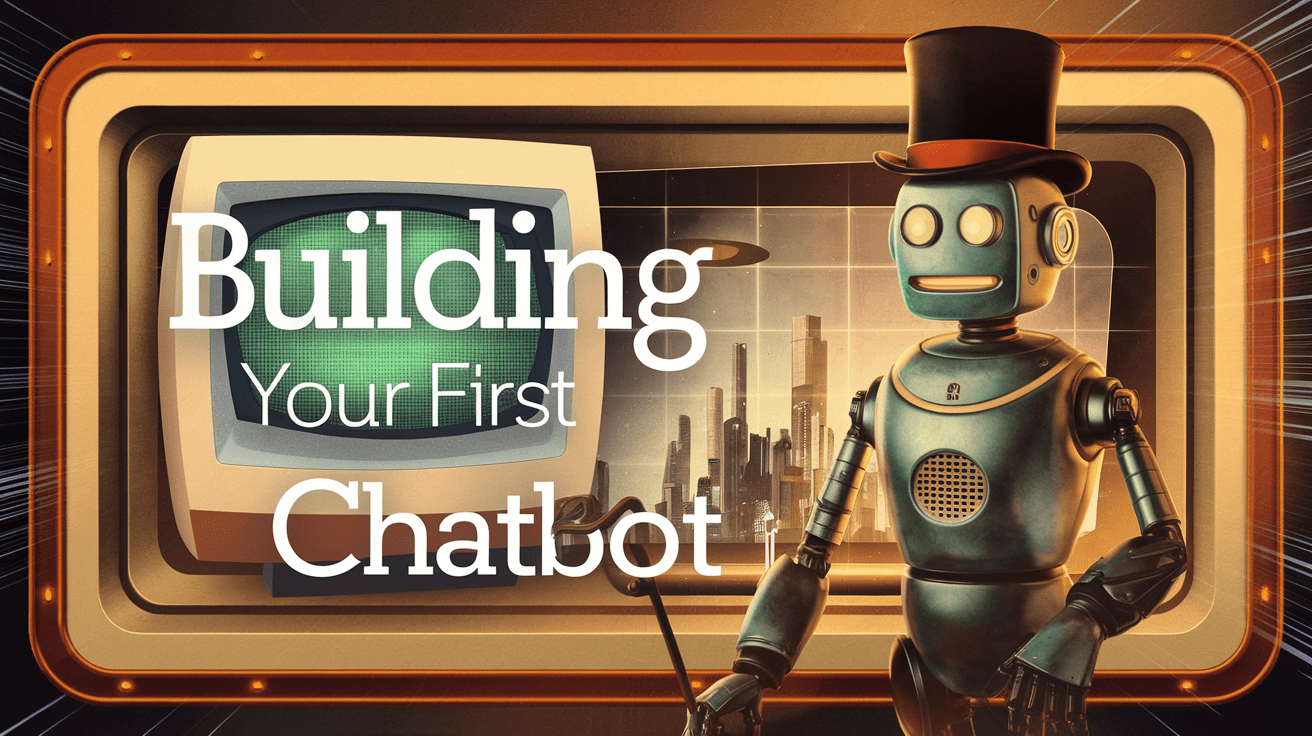
Constructing Your First Chatbot: A Palms-On Tutorial with Open-Supply Instruments
Picture by Editor | Ideogram
A chatbot is a pc program that may speak to individuals. It might reply questions and assist customers anytime. You don’t must know lots about coding to make one. There are free instruments that make it easy and enjoyable.
On this article, we’ll use a device referred to as ChatterBot. You’ll learn to set it up and practice it to reply.
Working of a Chatbot
Chatbots work through the use of algorithms to know what customers say. They take heed to consumer enter and discover the perfect response. When a consumer sorts a query, the chatbot processes it. It appears for key phrases and phrases to know the that means. Then, it selects a solution based mostly on its coaching knowledge.
The extra the chatbot interacts, the higher it turns into. It learns from every dialog. This permits it to enhance responses over time. Some chatbots use pure language processing (NLP) to know language higher. This makes conversations really feel extra pure.
ChatterBot
ChatterBot is a Python library for making chatbots. It helps you create good bots that may speak. The library makes use of machine studying to generate responses. This implies the bot can study from conversations. It’s straightforward to make use of, even for novices. ChatterBot supplies completely different storage choices. You should utilize SQL or MongoDB to save lots of knowledge. This allows you to decide what works greatest for you. The library can be customizable. You may change how the bot responds to suit your wants.
ChatterBot is open-source. This implies it’s free to make use of and modify. Anybody can use it to construct chatbots. It consists of built-in datasets for coaching. You should utilize English dialog knowledge to assist your bot study. This makes it an amazing device for creating partaking chatbots.
Setting Up Your Surroundings
Earlier than you begin, you have to arrange your atmosphere. Comply with these steps:
- Set up Python: Obtain and set up Python from the official website. Be sure to get Python 3.5 or later.
- Create a Digital Surroundings: This helps you handle your challenge. Run these instructions in your terminal:
python –m venv chatbot–env supply chatbot–env/bin/activate # On Home windows, use `chatbot-envScriptsactivate` |
Putting in ChatterBot
Subsequent, you have to set up ChatterBot. To create a chatbot, it’s also needed to put in the ChatterBot Corpus.
pip set up chatterbot pip set up chatterbot–corpus |
Let’s import the Chatbot class of the chatterbot module.
from chatterbot import ChatBot |
Initializing the ChatterBot
After you have the ChatterBot library put in, you can begin creating your chatbot.
# Create object of ChatBot class bot = ChatBot(‘MyChatBot’) |
Storage is necessary for a chatbot. It helps the bot keep in mind what it learns. With storage, the bot can maintain monitor of conversations. It might recall previous interactions. This improves the bot’s responses over time. You may select several types of storage. Choices embrace SQL and MongoDB. SQL storage saves knowledge in a database. This makes it simpler to handle and retrieve later.
from chatterbot import ChatBot
# Create a chatbot with SQL storage bot = ChatBot( ‘MyChatBot’, storage_adapter=‘chatterbot.storage.SQLStorageAdapter’, database_uri=‘sqlite:///database.sqlite3’ ) |
Setting Up the Coach
ChatterBot may be skilled with varied datasets. The ChatterBotCorpusTrainer lets you practice your chatbot with the built-in conversational datasets.
To coach your chatbot utilizing the English corpus, you should use the next code:
from chatterbot.trainers import ChatterBotCorpusTrainer
# Prepare the chatbot with the English corpus coach.practice(“chatterbot.corpus.english”) |
Customizing Your Chatbot
You may customise your chatbot in a number of methods:
Change Response Logic
ChatterBot makes use of logic adapters to decide on responses. You may change this habits. Use the BestMatch adapter:
chatbot = ChatBot( ‘MyBot’, logic_adapters=[ ‘chatterbot.logic.BestMatch’ ] ) |
Add Extra Coaching Information
Extra coaching knowledge improves your bot. You may create your individual knowledge file. Put it aside as custom_corpus.yml with pairs of questions and solutions.
– – How are you? – I‘m doing nicely, thank you!
– – What is your title? – I am MyBot. |
Prepare your bot with this practice knowledge:
coach.practice(‘path/to/custom_corpus.yml’) |
Implement Customized Logic
You may add customized logic for particular responses. Right here’s an instance of a easy customized adapter:
from chatterbot.logic import LogicAdapter
class CustomLogicAdapter(LogicAdapter): def can_process(self, assertion): return ‘climate’ in assertion.textual content
def course of(self, assertion, additional_response_selection_parameters=None): return ‘I can’t present climate data proper now.’ |
Testing Your Chatbot
As soon as your chatbot is skilled, you can begin interacting with it to check its responses. The next code creates a easy loop for chatting together with your bot:
print(“Chat with the bot! Sort ‘stop’ to exit.”)
whereas True: user_input = enter(“You: “) if user_input.decrease() == ‘stop’: break response = chatbot.get_response(user_input) print(“Bot:”, response) |
Chat with the bot! Sort ‘stop’ to exit. You: Hello there! Bot: Hey! You: How are you? Bot: I‘m doing nicely, thank you! You: stop |
Deploying Your Chatbot for Interplay
If you wish to make your chatbot accessible on-line, take into account integrating it with an internet software. Right here’s a easy approach to combine your ChatterBot with a Flask internet software:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route(“/chat”, strategies=[“POST”]) def chat(): user_input = request.json.get(“message”) response = chatbot.get_response(user_input) return jsonify({“response”: str(response)})
if __name__ == “__main__”: app.run(debug=True) |
This setup makes it straightforward to deploy your chatbot. Customers can chat with it on-line. You may ship messages to the chatbot via an internet software.
Conclusion
With instruments like ChatterBot, you can also make your individual chatbot rapidly. As you study extra about how you can use ChatterBot, you possibly can add a few of its further options. You may make your chatbot perceive language higher, and you’ll have it join it with different apps to do extra issues. It will make your chatbot smarter and extra useful.