Growing Sturdy ETL Pipelines for Knowledge Science Tasks
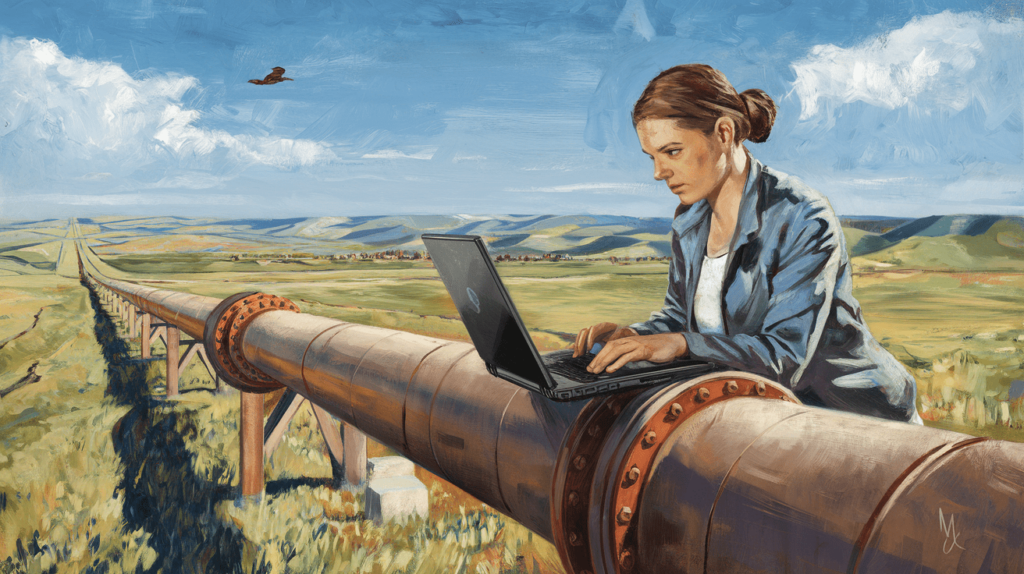

Picture by Editor | Ideogram
Good-quality knowledge is essential in knowledge science, nevertheless it usually comes from many locations and in messy codecs. Some knowledge comes from databases, whereas others come from information or web sites. This uncooked knowledge is tough to make use of straight away, and so we have to clear and manage it first.
ETL is the method that helps with this. ETL stands for Extract, Rework, and Load. Extract means gathering knowledge from totally different sources. Rework means cleansing and formatting the information. Load means storing the information in a database for simple entry. Constructing ETL pipelines automates this course of. A powerful ETL pipeline saves time and makes knowledge dependable.
On this article, we’ll have a look at tips on how to construct ETL pipelines for knowledge science tasks.
What’s an ETL Pipeline?
An ETL pipeline strikes knowledge from the supply to a vacation spot. It really works in three phases:
- Extract: Gather knowledge from a number of sources, like databases or information.
- Rework: Clear and rework the information for evaluation.
- Load: Retailer the cleaned knowledge in a database or one other system.
Why ETL Pipelines are Essential
ETL pipelines are necessary for a number of causes:
- Knowledge High quality: Transformation helps clear knowledge by dealing with lacking values and fixing errors.
- Knowledge Accessibility: ETL pipelines carry knowledge from many sources into one place for simple entry.
- Automation: Pipelines automate repetitive duties and lets knowledge scientists give attention to evaluation.
Now, let’s construct a easy ETL pipeline in Python.
Knowledge Ingestion
First, we have to get the information. We are going to extract it from a CSV file.
import pandas as pd
# Perform to extract knowledge from a CSV file
def extract_data(file_path):
attempt:
knowledge = pd.read_csv(file_path)
print(f"Knowledge extracted from {file_path}")
return knowledge
besides Exception as e:
print(f"Error in extraction: {e}")
return None
# Extract worker knowledge
employee_data = extract_data('/content material/employees_data.csv')
# Print the primary few rows of the information
if employee_data just isn't None:
print(employee_data.head())
Knowledge Transformation
After gathering the information, we have to rework it. This implies cleansing the information and making it right. We additionally change the information right into a format that’s prepared for evaluation. Listed here are some widespread transformations:
- Dealing with Lacking Knowledge: Take away or fill in lacking values.
- Creating Derived Options: Make new columns, like wage bands or age teams.
- Encoding Classes: Change knowledge like division names right into a format computer systems can use.
# Perform to remodel worker knowledge
def transform_data(knowledge):
attempt:
# Guarantee wage and age are numeric and deal with any errors
knowledge['Salary'] = pd.to_numeric(knowledge['Salary'], errors="coerce")
knowledge['Age'] = pd.to_numeric(knowledge['Age'], errors="coerce")
# Take away rows with lacking values
knowledge = knowledge.dropna(subset=['Salary', 'Age', 'Department'])
# Create wage bands
knowledge['Salary_band'] = pd.reduce(knowledge['Salary'], bins=[0, 60000, 90000, 120000, 1500000], labels=['Low', 'Medium', 'High', 'Very High'])
# Create age teams
knowledge['Age_group'] = pd.reduce(knowledge['Age'], bins=[0, 30, 40, 50, 60], labels=['Young', 'Middle-aged', 'Senior', 'Older'])
# Convert division to categorical
knowledge['Department'] = knowledge['Department'].astype('class')
print("Knowledge transformation full")
return knowledge
besides Exception as e:
print(f"Error in transformation: {e}")
return None
employee_data = extract_employee_data('/content material/employees_data.csv')
# Rework the worker knowledge
if employee_data just isn't None:
transformed_employee_data = transform_data(employee_data)
# Print the primary few rows of the reworked knowledge
print(transformed_employee_data.head())
Knowledge Storage
The ultimate step is to load it right into a database. This makes it simple to go looking and analyze.
Right here, we use SQLite. It’s a light-weight database that shops knowledge. We are going to create a desk referred to as staff within the SQLite database. Then, we are going to insert the reworked knowledge into this desk.
import sqlite3
# Perform to load reworked knowledge into SQLite database
def load_data_to_db(knowledge, db_name="employee_data.db"):
attempt:
# Connect with SQLite database (or create it if it does not exist)
conn = sqlite3.join(db_name)
cursor = conn.cursor()
# Create desk if it does not exist
cursor.execute('''
CREATE TABLE IF NOT EXISTS staff (
employee_id INTEGER PRIMARY KEY,
first_name TEXT,
last_name TEXT,
wage REAL,
age INTEGER,
division TEXT,
salary_band TEXT,
age_group TEXT
)
''')
# Insert knowledge into the workers desk
knowledge.to_sql('staff', conn, if_exists="substitute", index=False)
# Commit and shut the connection
conn.commit()
print(f"Knowledge loaded into {db_name} efficiently")
# Question the information to confirm it was loaded
question = "SELECT * FROM staff"
outcome = pd.read_sql(question, conn)
print("nData loaded into the database:")
print(outcome.head()) # Print the primary few rows of the information from the database
conn.shut()
besides Exception as e:
print(f"Error in loading knowledge: {e}")
load_data_to_db(transformed_employee_data)
Operating the Full ETL Pipeline
Since we now have the extract, rework, and cargo steps, we’re capable of mix them. This creates a full ETL pipeline. The pipeline will get the worker knowledge. It can clear and alter the information. Lastly, it should save the information within the database.
def run_etl_pipeline(file_path, db_name="employee_data.db"):
# Extract
knowledge = extract_employee_data(file_path)
if knowledge just isn't None:
# Rework
transformed_data = transform_employee_data(knowledge)
if transformed_data just isn't None:
# Load
load_data_to_db(transformed_data, db_name)
# Run the ETL pipeline
run_etl_pipeline('/content material/employees_data.csv', 'employee_data.db')
And there you’ve it: our ETL pipeline has been carried out and might now be executed.
Finest Practices for ETL Pipelines
Listed here are some finest practices to observe for environment friendly and dependable ETL pipelines:
- Use Modularity: Break the pipeline into smaller, reusable features.
- Error Dealing with: Add error dealing with to log points throughout extraction, transformation, or loading.
- Optimize Efficiency: Optimize queries and handle reminiscence for big datasets.
- Automated Testing: Check transformations and knowledge codecs robotically to make sure accuracy.
Conclusion
ETL pipelines are key to any knowledge science undertaking. They assist course of and retailer knowledge for correct evaluation. We confirmed tips on how to get knowledge from a CSV file. Then, we cleaned and adjusted the information. Lastly, we saved it in a SQLite database.
A superb ETL pipeline retains the information organized. This pipeline might be improved to deal with extra advanced knowledge and storage wants. It helps create scalable and dependable knowledge options.
Jayita Gulati is a machine studying fanatic and technical author pushed by her ardour for constructing machine studying fashions. She holds a Grasp’s diploma in Laptop Science from the College of Liverpool.