Filling the Gaps: A Comparative Information to Imputation Strategies in Machine Studying
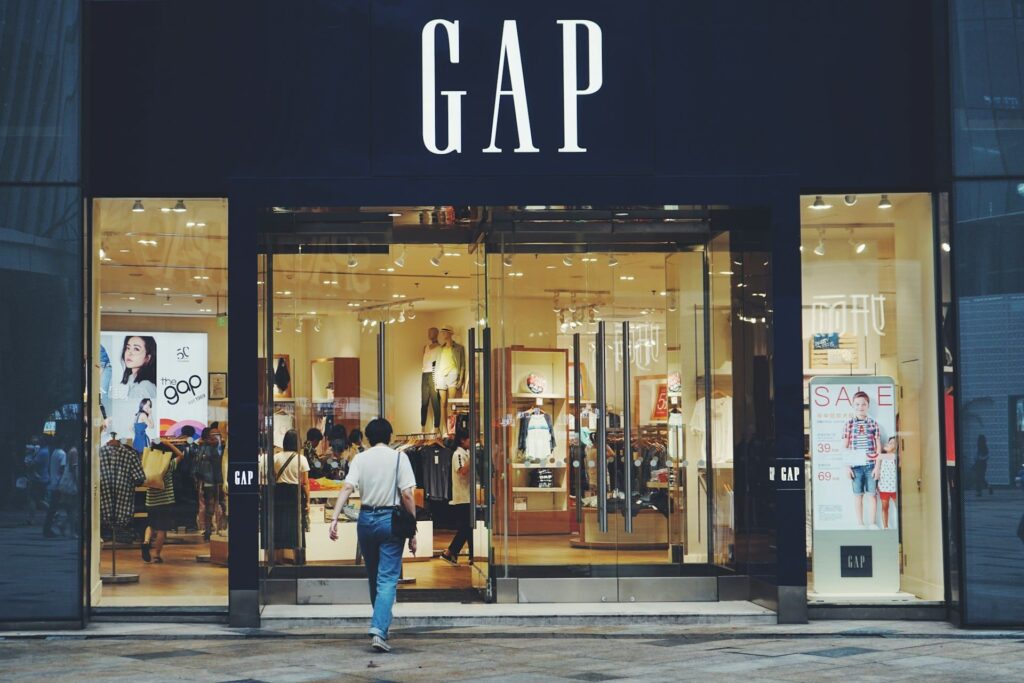
In our earlier exploration of penalized regression fashions corresponding to Lasso, Ridge, and ElasticNet, we demonstrated how successfully these fashions handle multicollinearity, permitting us to make the most of a broader array of options to reinforce mannequin efficiency. Constructing on this basis, we now handle one other essential facet of knowledge preprocessing—dealing with lacking values. Lacking knowledge can considerably compromise the accuracy and reliability of fashions if not appropriately managed. This put up explores varied imputation methods to handle lacking knowledge and embed them into our pipeline. This method permits us to additional refine our predictive accuracy by incorporating beforehand excluded options, thus profiting from our wealthy dataset.
Let’s get began.
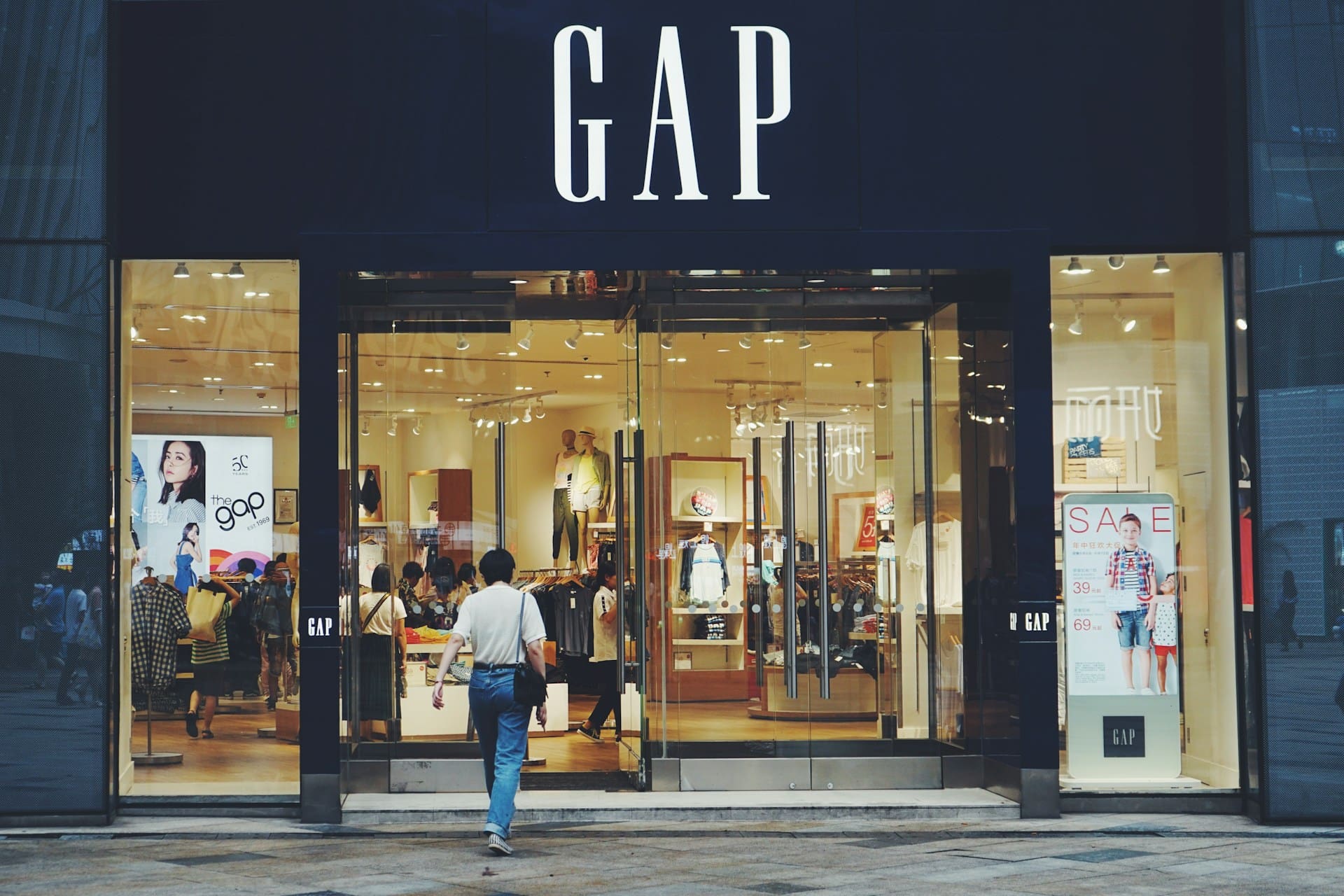
Filling the Gaps: A Comparative Information to Imputation Strategies in Machine Studying
Picture by lan deng. Some rights reserved.
Overview
This put up is split into three elements; they’re:
- Reconstructing Handbook Imputation with SimpleImputer
- Advancing Imputation Strategies with IterativeImputer
- Leveraging Neighborhood Insights with KNN Imputation
Reconstructing Handbook Imputation with SimpleImputer
Partially certainly one of this put up, we revisit and reconstruct our earlier guide imputation strategies utilizing SimpleImputer
. Our earlier exploration of the Ames Housing dataset offered foundational insights into using the data dictionary to sort out lacking knowledge. We demonstrated guide imputation methods tailor-made to completely different knowledge sorts, contemplating area data and knowledge dictionary particulars. For instance, categorical variables lacking within the dataset usually point out an absence of the function (e.g., a lacking ‘PoolQC’ may imply no pool exists), guiding our imputation to fill these with “None” to protect the dataset’s integrity. In the meantime, numerical options have been dealt with otherwise, using strategies like imply imputation.
Now, by automating these processes with scikit-learn’s SimpleImputer
, we improve reproducibility and effectivity. Our pipeline method not solely incorporates imputation but in addition scales and encodes options, getting ready them for regression evaluation with fashions corresponding to Lasso, Ridge, and ElasticNet:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 |
# Import the required libraries import pandas as pd from sklearn.pipeline import Pipeline from sklearn.impute import SimpleImputer from sklearn.compose import ColumnTransformer from sklearn.preprocessing import StandardScaler, OneHotEncoder, FunctionTransformer from sklearn.linear_model import Lasso, Ridge, ElasticNet from sklearn.model_selection import cross_val_rating
# Load the dataset Ames = pd.read_csv(‘Ames.csv’)
# Exclude ‘PID’ and ‘SalePrice’ from options and particularly deal with the ‘Electrical’ column numeric_features = Ames.select_dtypes(embrace=[‘int64’, ‘float64’]).drop(columns=[‘PID’, ‘SalePrice’]).columns categorical_features = Ames.select_dtypes(embrace=[‘object’]).columns.distinction([‘Electrical’]) electrical_feature = [‘Electrical’] # Particularly deal with the ‘Electrical’ column
# Helper perform to fill ‘None’ for lacking categorical knowledge def fill_none(X): return X.fillna(“None”)
# Pipeline for numeric options: Impute lacking values then scale numeric_transformer = Pipeline(steps=[ (‘impute_mean’, SimpleImputer(strategy=‘mean’)), (‘scaler’, StandardScaler()) ])
# Pipeline for normal categorical options: Fill lacking values with ‘None’ then apply one-hot encoding categorical_transformer = Pipeline(steps=[ (‘fill_none’, FunctionTransformer(fill_none, validate=False)), (‘onehot’, OneHotEncoder(handle_unknown=‘ignore’)) ])
# Particular transformer for ‘Electrical’ utilizing the mode for imputation electrical_transformer = Pipeline(steps=[ (‘impute_electrical’, SimpleImputer(strategy=‘most_frequent’)), (‘onehot_electrical’, OneHotEncoder(handle_unknown=‘ignore’)) ])
# Mixed preprocessor for numeric, normal categorical, and electrical knowledge preprocessor = ColumnTransformer( transformers=[ (‘num’, numeric_transformer, numeric_features), (‘cat’, categorical_transformer, categorical_features), (‘electrical’, electrical_transformer, electrical_feature) ])
# Goal variable y = Ames[‘SalePrice’]
# All options X = Ames[numeric_features.tolist() + categorical_features.tolist() + electrical_feature]
# Outline the mannequin pipelines with preprocessor and regressor fashions = { ‘Lasso’: Lasso(max_iter=20000), ‘Ridge’: Ridge(), ‘ElasticNet’: ElasticNet() }
outcomes = {} for identify, mannequin in fashions.gadgets(): pipeline = Pipeline(steps=[ (‘preprocessor’, preprocessor), (‘regressor’, model) ]) # Carry out cross-validation scores = cross_val_score(pipeline, X, y) outcomes[name] = spherical(scores.imply(), 4)
# Output the cross-validation scores print(“Cross-validation scores with Easy Imputer:”, outcomes) |
The outcomes from this implementation are displayed, exhibiting how easy imputation impacts mannequin accuracy and establishes a benchmark for extra refined strategies mentioned later:
Cross-validation scores with Easy Imputer: {‘Lasso’: 0.9138, ‘Ridge’: 0.9134, ‘ElasticNet’: 0.8752} |
Transitioning from guide steps to a pipeline method utilizing scikit-learn enhances a number of points of knowledge processing:
- Effectivity and Error Discount: Manually imputing values is time-consuming and vulnerable to errors, particularly as knowledge complexity will increase. The pipeline automates these steps, guaranteeing constant transformations and decreasing errors.
- Reusability and Integration: Handbook strategies are much less reusable. In distinction, pipelines encapsulate all the preprocessing and modeling steps, making them simply reusable and seamlessly built-in into the mannequin coaching course of.
- Information Leakage Prevention: There’s a threat of knowledge leakage with guide imputation, as it might embrace take a look at knowledge when computing values. Pipelines stop this threat with the match/rework methodology, guaranteeing calculations are derived solely from the coaching set.
This framework, demonstrated with SimpleImputer
, reveals a versatile method to knowledge preprocessing that may be simply tailored to incorporate varied imputation methods. In upcoming sections, we’ll discover further strategies, assessing their influence on mannequin efficiency.
Advancing Imputation Strategies with IterativeImputer
Partially two, we experiment with IterativeImputer
, a extra superior imputation approach that fashions every function with lacking values as a perform of different options in a round-robin vogue. Not like easy strategies that may use a normal statistic such because the imply or median, Iterative Imputer fashions every function with lacking values as a dependent variable in a regression, knowledgeable by the opposite options within the dataset. This course of iterates, refining estimates for lacking values utilizing all the set of accessible function interactions. This method can unveil refined knowledge patterns and dependencies not captured by easier imputation strategies:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
# Import the required libraries import pandas as pd from sklearn.pipeline import Pipeline from sklearn.experimental import enable_iterative_imputer # This line is required for IterativeImputer from sklearn.impute import SimpleImputer, IterativeImputer from sklearn.compose import ColumnTransformer from sklearn.preprocessing import StandardScaler, OneHotEncoder, FunctionTransformer from sklearn.linear_model import Lasso, Ridge, ElasticNet from sklearn.model_selection import cross_val_rating
# Load the dataset Ames = pd.read_csv(‘Ames.csv’)
# Exclude ‘PID’ and ‘SalePrice’ from options and particularly deal with the ‘Electrical’ column numeric_features = Ames.select_dtypes(embrace=[‘int64’, ‘float64’]).drop(columns=[‘PID’, ‘SalePrice’]).columns categorical_features = Ames.select_dtypes(embrace=[‘object’]).columns.distinction([‘Electrical’]) electrical_feature = [‘Electrical’] # Particularly deal with the ‘Electrical’ column
# Helper perform to fill ‘None’ for lacking categorical knowledge def fill_none(X): return X.fillna(“None”)
# Pipeline for numeric options: Iterative imputation then scale numeric_transformer_advanced = Pipeline(steps=[ (‘impute_iterative’, IterativeImputer(random_state=42)), (‘scaler’, StandardScaler()) ])
# Pipeline for normal categorical options: Fill lacking values with ‘None’ then apply one-hot encoding categorical_transformer = Pipeline(steps=[ (‘fill_none’, FunctionTransformer(fill_none, validate=False)), (‘onehot’, OneHotEncoder(handle_unknown=‘ignore’)) ])
# Particular transformer for ‘Electrical’ utilizing the mode for imputation electrical_transformer = Pipeline(steps=[ (‘impute_electrical’, SimpleImputer(strategy=‘most_frequent’)), (‘onehot_electrical’, OneHotEncoder(handle_unknown=‘ignore’)) ])
# Mixed preprocessor for numeric, normal categorical, and electrical knowledge preprocessor_advanced = ColumnTransformer( transformers=[ (‘num’, numeric_transformer_advanced, numeric_features), (‘cat’, categorical_transformer, categorical_features), (‘electrical’, electrical_transformer, electrical_feature) ])
# Goal variable y = Ames[‘SalePrice’]
# All options X = Ames[numeric_features.tolist() + categorical_features.tolist() + electrical_feature]
# Outline the mannequin pipelines with preprocessor and regressor fashions = { ‘Lasso’: Lasso(max_iter=20000), ‘Ridge’: Ridge(), ‘ElasticNet’: ElasticNet() }
results_advanced = {} for identify, mannequin in fashions.gadgets(): pipeline = Pipeline(steps=[ (‘preprocessor’, preprocessor_advanced), (‘regressor’, model) ]) # Carry out cross-validation scores = cross_val_score(pipeline, X, y) results_advanced[name] = spherical(scores.imply(), 4)
# Output the cross-validation scores for superior imputation print(“Cross-validation scores with Iterative Imputer:”, results_advanced) |
Whereas the enhancements in accuracy from IterativeImputer
over SimpleImputer
are modest, they spotlight an essential facet of knowledge imputation: the complexity and interdependencies in a dataset could not at all times result in dramatically greater scores with extra refined strategies:
Cross-validation scores with Iterative Imputer: {‘Lasso’: 0.9142, ‘Ridge’: 0.9135, ‘ElasticNet’: 0.8746} |
These modest enhancements reveal that whereas IterativeImputer
can refine the precision of our fashions, the extent of its influence can differ relying on the dataset’s traits. As we transfer into the third and closing a part of this put up, we’ll discover KNNImputer
, another superior approach that leverages the closest neighbors method, probably providing completely different insights and benefits for dealing with lacking knowledge in varied kinds of datasets.
Leveraging Neighborhood Insights with KNN Imputation
Within the closing a part of this put up, we discover KNNImputer
, which imputes lacking values utilizing the imply of the k-nearest neighbors discovered within the coaching set. This technique assumes that comparable knowledge factors might be discovered shut in function area, making it extremely efficient for datasets the place such assumptions maintain true. KNN imputation is especially highly effective in situations the place knowledge factors with comparable traits are more likely to have comparable responses or options. We look at its influence on the identical predictive fashions, offering a full spectrum of how completely different imputation strategies may affect the outcomes of regression analyses:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 |
# Import the required libraries import pandas as pd from sklearn.pipeline import Pipeline from sklearn.impute import SimpleImputer, KNNImputer from sklearn.compose import ColumnTransformer from sklearn.preprocessing import StandardScaler, OneHotEncoder, FunctionTransformer from sklearn.linear_model import Lasso, Ridge, ElasticNet from sklearn.model_selection import cross_val_rating
# Load the dataset Ames = pd.read_csv(‘Ames.csv’)
# Exclude ‘PID’ and ‘SalePrice’ from options and particularly deal with the ‘Electrical’ column numeric_features = Ames.select_dtypes(embrace=[‘int64’, ‘float64’]).drop(columns=[‘PID’, ‘SalePrice’]).columns categorical_features = Ames.select_dtypes(embrace=[‘object’]).columns.distinction([‘Electrical’]) electrical_feature = [‘Electrical’] # Particularly deal with the ‘Electrical’ column
# Helper perform to fill ‘None’ for lacking categorical knowledge def fill_none(X): return X.fillna(“None”)
# Pipeline for numeric options: Ok-Nearest Neighbors Imputation then scale numeric_transformer_knn = Pipeline(steps=[ (‘impute_knn’, KNNImputer(n_neighbors=5)), (‘scaler’, StandardScaler()) ])
# Pipeline for normal categorical options: Fill lacking values with ‘None’ then apply one-hot encoding categorical_transformer = Pipeline(steps=[ (‘fill_none’, FunctionTransformer(fill_none, validate=False)), (‘onehot’, OneHotEncoder(handle_unknown=‘ignore’)) ])
# Particular transformer for ‘Electrical’ utilizing the mode for imputation electrical_transformer = Pipeline(steps=[ (‘impute_electrical’, SimpleImputer(strategy=‘most_frequent’)), (‘onehot_electrical’, OneHotEncoder(handle_unknown=‘ignore’)) ])
# Mixed preprocessor for numeric, normal categorical, and electrical knowledge preprocessor_knn = ColumnTransformer( transformers=[ (‘num’, numeric_transformer_knn, numeric_features), (‘cat’, categorical_transformer, categorical_features), (‘electrical’, electrical_transformer, electrical_feature) ])
# Goal variable y = Ames[‘SalePrice’]
# All options X = Ames[numeric_features.tolist() + categorical_features.tolist() + electrical_feature]
# Outline the mannequin pipelines with preprocessor and regressor fashions = { ‘Lasso’: Lasso(max_iter=20000), ‘Ridge’: Ridge(), ‘ElasticNet’: ElasticNet() }
results_knn = {} for identify, mannequin in fashions.gadgets(): pipeline = Pipeline(steps=[ (‘preprocessor’, preprocessor_knn), (‘regressor’, model) ]) # Carry out cross-validation scores = cross_val_score(pipeline, X, y) results_knn[name] = spherical(scores.imply(), 4)
# Output the cross-validation scores for KNN imputation print(“Cross-validation scores with KNN Imputer:”, results_knn) |
The cross-validation outcomes utilizing KNNImputer
present a really slight enchancment in comparison with these achieved with SimpleImputer
and IterativeImputer:
Cross–validation scores with KNN Imputer: {‘Lasso’: 0.9146, ‘Ridge’: 0.9138, ‘ElasticNet’: 0.8748} |
This refined enhancement means that for sure datasets, the proximity-based method of KNNImputer
—which components within the similarity between knowledge factors—might be more practical in capturing and preserving the underlying construction of the info, probably resulting in extra correct predictions.
Additional Studying
APIs
Tutorials
Assets
Abstract
This put up has guided you thru the development from guide to automated imputation strategies, beginning with a replication of fundamental guide imputation utilizing SimpleImputer
to ascertain a benchmark. We then explored extra refined methods with IterativeImputer
, which fashions every function with lacking values as depending on different options, and concluded with KNNImputer
, leveraging the proximity of knowledge factors to fill in lacking values. Curiously, in our case, these refined strategies didn’t present a big enchancment over the essential technique. This demonstrates that whereas superior imputation strategies might be utilized to deal with lacking knowledge, their effectiveness can differ relying on the particular traits and construction of the dataset concerned.
Particularly, you realized:
- How you can replicate and automate guide imputation processing utilizing
SimpleImputer
. - How enhancements in predictive efficiency could not at all times justify the complexity of
IterativeImputer
. - How
KNNImputer
demonstrates the potential for leveraging knowledge construction in imputation, although it equally confirmed solely modest enhancements in our dataset.
Do you’ve any questions? Please ask your questions within the feedback beneath, and I’ll do my finest to reply.