Geocoding for Knowledge Scientists – KDnuggets
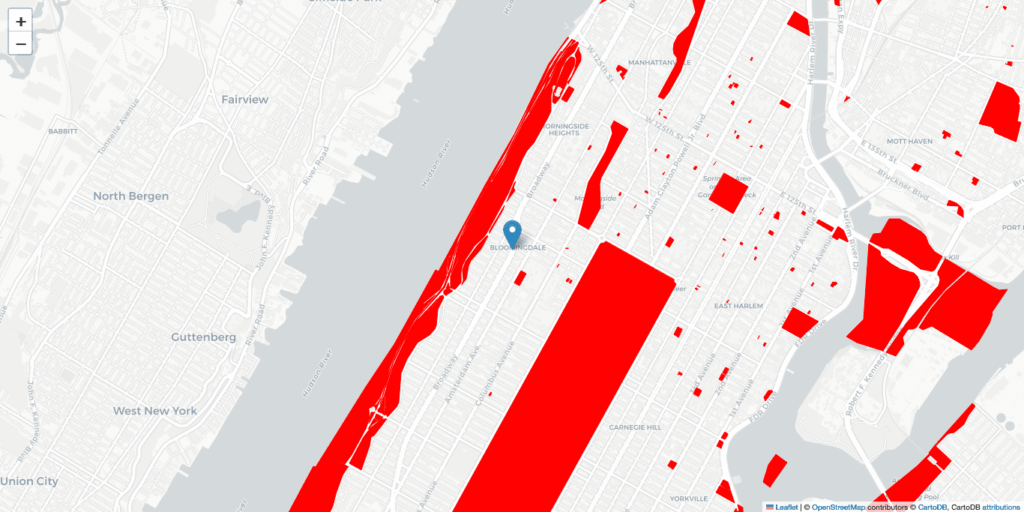
When knowledge scientists must know the whole lot there’s to know in regards to the “the place” of their knowledge, they typically flip to Geographic Info Programs (GIS). GIS is a sophisticated set of applied sciences and applications that serve all kinds of functions, however the College of Washington gives a reasonably complete definition, saying “a geographic data system is a fancy association of related or related issues or objects, whose goal is to speak data about options on the floor of the earth” (Lawler et al). GIS encompasses a broad vary of methods for processing spatial knowledge from acquisition to visualization, lots of that are priceless instruments even in case you are not a GIS specialist. This text gives a complete overview of geocoding with demonstrations in Python of a number of sensible functions. Particularly, you’ll decide the precise location of a pizza parlor in New York Metropolis, New York utilizing its handle and join it to knowledge about close by parks. Whereas the demonstrations use Python code, the core ideas might be utilized to many programming environments to combine geocoding into your workflow. These instruments present the idea for reworking knowledge into spatial knowledge and open the door for extra advanced geographic evaluation.

Geocoding is mostly outlined because the transformation of handle knowledge into mapping coordinates. Often, this entails detecting a road title in an handle, matching that road to the boundaries of its real-world counterpart in a database, then estimating the place on the road to put the handle utilizing the road quantity. For instance, let’s undergo the method of a easy guide geocode for the handle of a pizza parlor in New York on Broadway: 2709 Broadway, New York, NY 10025. The primary job is discovering applicable shapefiles for the highway system of the placement of your handle. Observe that on this case town and state of the handle are “New York, NY.” Fortuitously, town of New York publishes detailed highway data on the NYC Open Data web page (CSCL PUB). Second, look at the road title “Broadway.” You now know that the handle can lie on any road referred to as “Broadway” in NY city, so you possibly can execute the next Python code to question the NYC Open Knowledge SODA API for all streets named “Broadway.”
import geopandas as gpd
import requests
from io import BytesIO
# Request the info from the SODA API
req = requests.get(
"https://knowledge.cityofnewyork.us/useful resource/gdww-crzy.geojson?stname_lab=BROADWAY"
)
# Convert to a stream of bytes
reqstrm = BytesIO(req.content material)
# Learn the stream as a GeoDataFrame
ny_streets = gpd.read_file(reqstrm)
There are over 700 outcomes of this question, however that doesn’t imply it’s a must to examine 700 streets to seek out your pizza. Visualizing the info, you possibly can see that there are 3 foremost Broadway streets and some smaller ones.

The rationale for that is that every road is damaged up into sections that correspond roughly to a block, permitting for a extra granular have a look at the info. The following step of the method is figuring out precisely which of those sections the handle is on utilizing the ZIP code and road quantity. Every road section within the dataset incorporates handle ranges for the addresses of buildings on each the left and proper sides of the road. Equally, every section incorporates the ZIP code for each the left and proper sides of the road. To find the proper section, the next code applies filters to seek out the road section whose ZIP code matches the handle’ ZIP code and whose handle vary incorporates the road variety of the handle.
# Handle to be geocoded
handle = "2709 Broadway, New York, NY 10025"
zipcode = handle.break up(" ")[-1]
street_num = handle.break up(" ")[0]
# Discover road segments whose left aspect handle ranges comprise the road quantity
potentials = ny_streets.loc[ny_streets["l_low_hn"] < street_num]
potentials = potentials.loc[potentials["l_high_hn"] > street_num]
# Discover road segments whose zipcode matches the handle'
potentials = potentials.loc[potentials["l_zip"] == zipcode]
This narrows the checklist to the one road section seen under.

The ultimate job is to find out the place the handle lies on this line. That is performed by putting the road quantity contained in the handle vary for the section, normalizing to find out how far alongside the road the handle needs to be, and making use of that fixed to the coordinates of the endpoints of the road to get the coordinates of the handle. The next code outlines this course of.
import numpy as np
from shapely.geometry import Level
# Calculate how far alongside the road to put the purpose
denom = (
potentials["l_high_hn"].astype(float) - potentials["l_low_hn"].astype(float)
).values[0]
normalized_street_num = (
float(street_num) - potentials["l_low_hn"].astype(float).values[0]
) / denom
# Outline some extent that far alongside the road
# Transfer the road to start out at (0,0)
pizza = np.array(potentials["geometry"].values[0].coords[1]) - np.array(
potentials["geometry"].values[0].coords[0]
)
# Multiply by normalized road quantity to get coordinates on line
pizza = pizza * normalized_street_num
# Add beginning section to put line again on the map
pizza = pizza + np.array(potentials["geometry"].values[0].coords[0])
# Convert to geometry array for geopandas
pizza = gpd.GeoDataFrame(
{"handle": [address], "geometry": [Point(pizza[0], pizza[1])]},
crs=ny_streets.crs,
geometry="geometry",
)
Having completed geocoding the handle, it’s now doable to plot the placement of this pizza parlor on a map to grasp its location. Because the code above checked out data pertaining to the left aspect of a road section, the precise location shall be barely left of the plotted level in a constructing on the left aspect of the highway. You lastly know the place you will get some pizza.
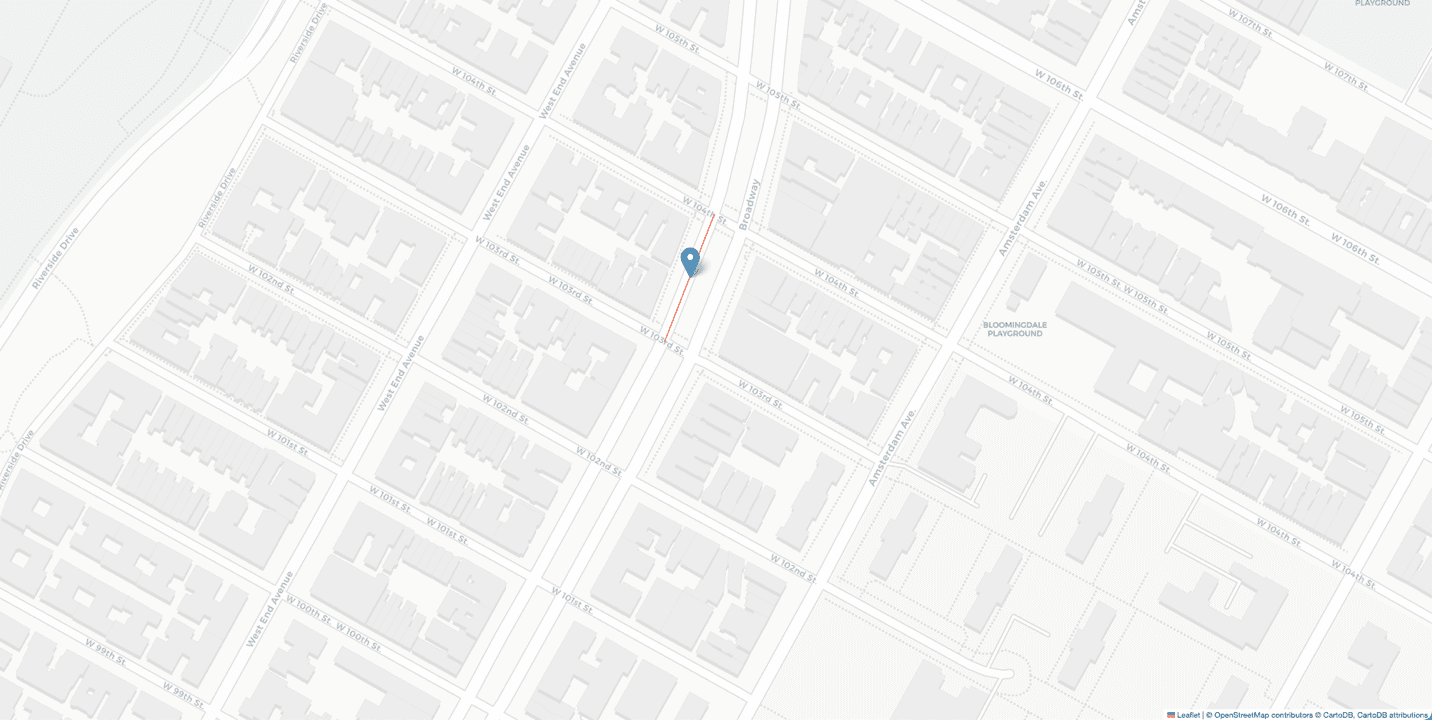
This course of covers what’s mostly known as geocoding, however it’s not the one approach the time period is used. You might also see geocoding consult with the method of transferring landmark names to coordinates, ZIP codes to coordinates, or coordinates to GIS vectors. It’s possible you’ll even hear reverse geocoding (which shall be lined later) known as geocoding. A extra lenient definition for geocoding that encompasses these can be “the switch between approximate, pure language descriptions of areas and geographic coordinates.” So, any time it’s worthwhile to transfer between these two sorts of information, take into account geocoding as an answer.
As a substitute for repeating this course of at any time when it’s worthwhile to geocode addresses, quite a lot of API endpoints, such because the U.S. Census Bureau Geocoder and the Google Geocoding API, present an correct geocoding service totally free. Some paid choices, comparable to Esri’s ArcGIS, Geocodio, and Smarty even supply rooftop accuracy for choose addresses, which implies that the returned coordinate lands precisely on the roof of the constructing as a substitute of on a close-by road. The next sections define how you can use these providers to suit geocoding into your knowledge pipeline utilizing the U.S. Census Bureau Geocoder for instance.
To be able to get the very best doable accuracy when geocoding, it’s best to all the time start by guaranteeing that your addresses are formatted to suit the requirements of your chosen service. This may differ barely between every service, however a typical format is the USPS format of “PRIMARY# STREET, CITY, STATE, ZIP” the place STATE is an abbreviation code, PRIMARY# is the road quantity, and all mentions of suite numbers, constructing numbers, and PO containers are eliminated.
As soon as your handle is formatted, it’s worthwhile to submit it to the API for geocoding. Within the case of the U.S. Census Bureau Geocoder, you possibly can both manually submit the handle by the One Line Handle Processing tab or use the provided REST API to submit the handle programmatically. The U.S. Census Bureau Geocoder additionally means that you can geocode complete recordsdata utilizing the batch geocoder and specify the info supply utilizing the benchmark parameter. To geocode the pizza parlor from earlier, this link can be utilized to cross the handle to the REST API, which might be performed in Python with the next code.
# Submit the handle to the U.S. Census Bureau Geocoder REST API for processing
response = requests.get(
"https://geocoding.geo.census.gov/geocoder/areas/onelineaddress?handle=2709+Broadwaypercent2C+New+Yorkpercent2C+NY+10025&benchmark=Public_AR_Current&format=json"
).json()
The returned knowledge is a JSON file, which is decoded simply right into a Python dictionary. It incorporates a “tigerLineId” discipline which can be utilized to match the shapefile for the closest road, a “aspect” discipline which can be utilized to find out which aspect of that road the handle is on, and “fromAddress” and “toAddress” fields which comprise the handle vary for the road section. Most significantly, it incorporates a “coordinates” discipline that can be utilized to find the handle on a map. The next code extracts the coordinates from the JSON file and processes it right into a GeoDataFrame to arrange it for spatial evaluation.
# Extract coordinates from the JSON file
coords = response["result"]["addressMatches"][0]["coordinates"]
# Convert coordinates to a Shapely Level
coords = Level(coords["x"], coords["y"])
# Extract matched handle
matched_address = response["result"]["addressMatches"][0]["matchedAddress"]
# Create a GeoDataFrame containing the outcomes
pizza_point = gpd.GeoDataFrame(
{"handle": [matched_address], "geometry": coords},
crs=ny_streets.crs,
geometry="geometry",
)
Visualizing this level exhibits that it’s barely off the highway to the left of the purpose that was geocoded manually.

Reverse geocoding is the method of taking geographic coordinates and matching them to pure language descriptions of a geographic area. When utilized appropriately, it is among the strongest methods for attaching exterior knowledge within the knowledge science toolkit. Step one of reverse geocoding is figuring out your goal geographies. That is the area that can comprise your coordinate knowledge. Some widespread examples are census tracts, ZIP codes, and cities. The second step is figuring out which, if any, of these areas the purpose is in. When utilizing widespread areas, the U.S. Census Geocoder can be utilized to reverse geocode by making small adjustments to the REST API request. A request for figuring out which Census geographies comprise the pizza parlor from earlier than is linked here. The results of this question might be processed utilizing the identical strategies as earlier than. Nonetheless, creatively defining the area to suit an evaluation want and manually reverse geocoding to it opens up many prospects.
To manually reverse geocode, it’s worthwhile to decide the placement and form of a area, then decide if the purpose is on the inside of that area. Figuring out if some extent is inside a polygon is definitely a reasonably troublesome downside, however the ray casting algorithm, the place a ray beginning on the level and travelling infinitely in a route intersects the boundary of the area an odd variety of occasions whether it is contained in the area and an excellent variety of occasions in any other case (Shimrat), can be utilized to resolve it typically. For the mathematically inclined, that is really a direct utility of the Jordan curve theorem (Hosch). As a notice, in case you are utilizing knowledge from world wide, the ray casting algorithm can really fail since a ray will ultimately wrap across the Earth’s floor and grow to be a circle. On this case, you’ll as a substitute have to seek out the winding quantity (Weisstein) for the area and the purpose. The purpose is contained in the area if the winding quantity just isn’t zero. Fortuitously, Python’s geopandas library gives the performance essential to each outline the inside of a polygonal area and check if some extent is within it with out all of the advanced arithmetic.
Whereas guide geocoding might be too advanced for a lot of functions, guide reverse geocoding could be a sensible addition to your talent set because it means that you can simply match your factors to extremely personalized areas. For instance, assume you need to take your slice of pizza to a park and have a picnic. It’s possible you’ll need to know if the pizza parlor is inside a brief distance of a park. New York Metropolis gives shapefiles for his or her parks as a part of the Parks Properties dataset (NYC Parks Open Knowledge Staff), and so they may also be accessed by way of their SODA API utilizing the next code.
# Pull NYC park shapefiles
parks = gpd.read_file(
BytesIO(
requests.get(
"https://knowledge.cityofnewyork.us/useful resource/enfh-gkve.geojson?$restrict=5000"
).content material
)
)
# Restrict to parks with inexperienced space for a picnic
parks = parks.loc[
parks["typecategory"].isin(
[
"Garden",
"Nature Area",
"Community Park",
"Neighborhood Park",
"Flagship Park",
]
)
]
These parks might be added to the visualization to see what parks are close by the pizza parlor.
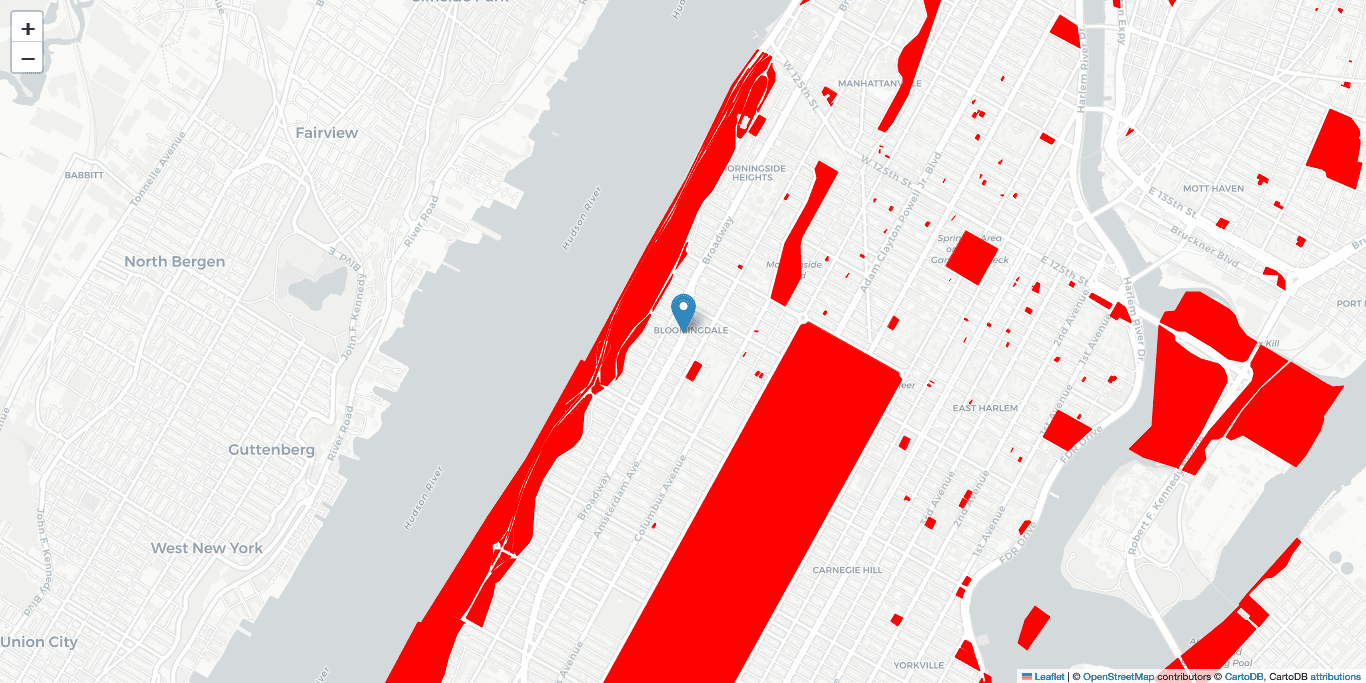
There are clearly some choices close by, however determining the gap utilizing the shapefiles and the purpose might be troublesome and computationally costly. As an alternative, reverse geocoding might be utilized. Step one, as talked about above, is figuring out the area you need to connect the purpose to. On this case, the area is “a 1/2-mile distance from a park in New York Metropolis.” The second step is calculation if the purpose lies inside a area, which might be performed mathematically utilizing the beforehand talked about strategies or by making use of the “incorporates” operate in geopandas. The next code is used so as to add a 1/2-mile buffer to the boundaries of the parks earlier than testing to see which parks’ buffered areas now comprise the purpose.
# Challenge the coordinates from latitude and longitude into meters for distance calculations
buffered_parks = parks.to_crs(epsg=2263)
pizza_point = pizza_point.to_crs(epsg=2263)
# Add a buffer to the areas extending the border by 1/2 mile = 2640 ft
buffered_parks = buffered_parks.buffer(2640)
# Discover all parks whose buffered area incorporates the pizza parlor
pizza_parks = parks.loc[buffered_parks.contains(pizza_point["geometry"].values[0])]
This buffer reveals the close by parks, that are highlighted in blue within the picture under

After profitable reverse geocoding, you’ve realized that there are 8 parks inside a half mile of the pizza parlor during which you can have your picnic. Take pleasure in that slice.

Pizza Slice by j4p4n
Sources
- Lawler, Josh and Schiess, Peter. ESRM 250: Introduction to Geographic Info Programs in Forest Sources. Definitions of GIS, 12 Feb. 2009, College of Washington, Seattle. Class Lecture. https://courses.washington.edu/gis250/lessons/introduction_gis/definitions.html
- CSCL PUB. New York OpenData. https://data.cityofnewyork.us/City-Government/road/svwp-sbcd
- U.S. Census Bureau Geocoder Documentation. August 2022. https://geocoding.geo.census.gov/geocoder/Geocoding_Services_API.pdf
- Shimrat, M., “Algorithm 112: Place of level relative to polygon” 1962, Communications of the ACM Quantity 5 Problem 8, Aug. 1962. https://dl.acm.org/doi/10.1145/368637.368653
- Hosch, William L.. “Jordan curve theorem”. Encyclopedia Britannica, 13 Apr. 2018, https://www.britannica.com/science/Jordan-curve-theorem
- Weisstein, Eric W. “Contour Winding Quantity.” From MathWorld–A Wolfram Internet Useful resource. https://mathworld.wolfram.com/ContourWindingNumber.html
- NYC Parks Open Knowledge Staff. Parks Properties. April 14, 2023. https://nycopendata.socrata.com/Recreation/Parks-Properties/enfh-gkve
- j4p4n, “Pizza Slice.” From OpenClipArt. https://openclipart.org/detail/331718/pizza-slice
Evan Miller is a Knowledge Science Fellow at Tech Impression, the place he makes use of knowledge to assist nonprofit and authorities businesses with a mission of social good. Beforehand, Evan used machine studying to coach autonomous automobiles at Central Michigan College.