Implement a {custom} AutoML job utilizing pre-selected algorithms in Amazon SageMaker Computerized Mannequin Tuning
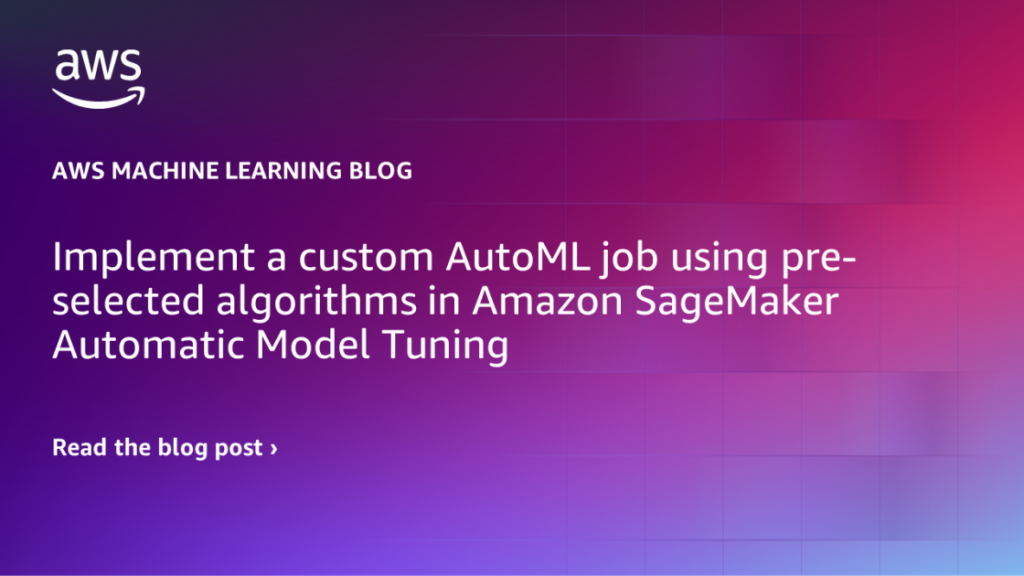
AutoML means that you can derive speedy, basic insights out of your knowledge proper at first of a machine studying (ML) venture lifecycle. Understanding up entrance which preprocessing strategies and algorithm varieties present greatest outcomes reduces the time to develop, prepare, and deploy the precise mannequin. It performs an important function in each mannequin’s growth course of and permits knowledge scientists to concentrate on probably the most promising ML strategies. Moreover, AutoML supplies a baseline mannequin efficiency that may function a reference level for the info science group.
An AutoML instrument applies a mixture of various algorithms and numerous preprocessing strategies to your knowledge. For instance, it may scale the info, carry out univariate function choice, conduct PCA at totally different variance threshold ranges, and apply clustering. Such preprocessing strategies might be utilized individually or be mixed in a pipeline. Subsequently, an AutoML instrument would prepare totally different mannequin varieties, corresponding to Linear Regression, Elastic-Web, or Random Forest, on totally different variations of your preprocessed dataset and carry out hyperparameter optimization (HPO). Amazon SageMaker Autopilot eliminates the heavy lifting of constructing ML fashions. After offering the dataset, SageMaker Autopilot mechanically explores totally different options to search out the perfect mannequin. However what if you wish to deploy your tailor-made model of an AutoML workflow?
This publish exhibits the best way to create a custom-made AutoML workflow on Amazon SageMaker utilizing Amazon SageMaker Automatic Model Tuning with pattern code out there in a GitHub repo.
Answer overview
For this use case, let’s assume you’re a part of an information science group that develops fashions in a specialised area. You might have developed a set of {custom} preprocessing strategies and chosen a variety of algorithms that you simply usually anticipate to work effectively along with your ML drawback. When engaged on new ML use circumstances, you want to first to carry out an AutoML run utilizing your preprocessing strategies and algorithms to slim down the scope of potential options.
For this instance, you don’t use a specialised dataset; as an alternative, you’re employed with the California Housing dataset that you’ll import from Amazon Simple Storage Service (Amazon S3). The main focus is to reveal the technical implementation of the answer utilizing SageMaker HPO, which later will be utilized to any dataset and area.
The next diagram presents the general answer workflow.
Stipulations
The next are conditions for finishing the walkthrough on this publish:
Implement the answer
The complete code is obtainable within the GitHub repo.
The steps to implement the answer (as famous within the workflow diagram) are as follows:
- Create a notebook instance and specify the next:
- For Pocket book occasion kind, select ml.t3.medium.
- For Elastic Inference, select none.
- For Platform identifier, select Amazon Linux 2, Jupyter Lab 3.
- For IAM function, select the default
AmazonSageMaker-ExecutionRole
. If it doesn’t exist, create a brand new AWS Identity and Access Management (IAM) function and connect the AmazonSageMakerFullAccess IAM policy.
Notice that it is best to create a minimally scoped execution function and coverage in manufacturing.
- Open the JupyterLab interface in your pocket book occasion and clone the GitHub repo.
You are able to do that by beginning a brand new terminal session and working the git clone <REPO>
command or through the use of the UI performance, as proven within the following screenshot.
- Open the
automl.ipynb
pocket book file, choose theconda_python3
kernel, and comply with the directions to set off a set of HPO jobs.
To run the code with none adjustments, you have to improve the service quota for ml.m5.giant for coaching job utilization and Variety of cases throughout all coaching jobs. AWS permits by default solely 20 parallel SageMaker coaching jobs for each quotas. You should request a quota improve to 30 for each. Each quota adjustments ought to usually be permitted inside a couple of minutes. Seek advice from Requesting a quota increase for extra data.
When you don’t wish to change the quota, you possibly can merely modify the worth of the MAX_PARALLEL_JOBS
variable within the script (for instance, to five).
- Every HPO job will full a set of training job trials and point out the mannequin with optimum hyperparameters.
- Analyze the outcomes and deploy the best-performing model.
This answer will incur prices in your AWS account. The price of this answer will depend upon the quantity and period of HPO coaching jobs. As these improve, so will the price. You may scale back prices by limiting coaching time and configuring TuningJobCompletionCriteriaConfig
in keeping with the directions mentioned later on this publish. For pricing data, check with Amazon SageMaker Pricing.
Within the following sections, we focus on the pocket book in additional element with code examples and the steps to investigate the outcomes and choose the perfect mannequin.
Preliminary setup
Let’s begin with working the Imports & Setup part within the custom-automl.ipynb
pocket book. It installs and imports all of the required dependencies, instantiates a SageMaker session and shopper, and units the default Area and S3 bucket for storing knowledge.
Information preparation
Obtain the California Housing dataset and put together it by working the Obtain Information part of the pocket book. The dataset is break up into coaching and testing knowledge frames and uploaded to the SageMaker session default S3 bucket.
The whole dataset has 20,640 information and 9 columns in whole, together with the goal. The purpose is to foretell the median worth of a home (medianHouseValue
column). The next screenshot exhibits the highest rows of the dataset.
Coaching script template
The AutoML workflow on this publish relies on scikit-learn preprocessing pipelines and algorithms. The intention is to generate a big mixture of various preprocessing pipelines and algorithms to search out the best-performing setup. Let’s begin with making a generic coaching script, which is persevered regionally on the pocket book occasion. On this script, there are two empty remark blocks: one for injecting hyperparameters and the opposite for the preprocessing-model pipeline object. They are going to be injected dynamically for every preprocessing mannequin candidate. The aim of getting one generic script is to maintain the implementation DRY (don’t repeat your self).
Create preprocessing and mannequin mixtures
The preprocessors
dictionary comprises a specification of preprocessing strategies utilized to all enter options of the mannequin. Every recipe is outlined utilizing a Pipeline
or a FeatureUnion
object from scikit-learn, which chains collectively particular person knowledge transformations and stack them collectively. For instance, mean-imp-scale
is a straightforward recipe that ensures that lacking values are imputed utilizing imply values of respective columns and that every one options are scaled utilizing the StandardScaler. In distinction, the mean-imp-scale-pca
recipe chains collectively a couple of extra operations:
- Impute lacking values in columns with its imply.
- Apply function scaling utilizing imply and commonplace deviation.
- Calculate PCA on prime of the enter knowledge at a specified variance threshold worth and merge it along with the imputed and scaled enter options.
On this publish, all enter options are numeric. When you’ve got extra knowledge varieties in your enter dataset, it is best to specify a extra sophisticated pipeline the place totally different preprocessing branches are utilized to totally different function kind units.
The fashions
dictionary comprises specs of various algorithms that you simply match the dataset to. Each mannequin kind comes with the next specification within the dictionary:
- script_output – Factors to the situation of the coaching script utilized by the estimator. This area is stuffed dynamically when the
fashions
dictionary is mixed with thepreprocessors
dictionary. - insertions – Defines code that will likely be inserted into the
script_draft.py
and subsequently saved beneathscript_output
. The important thing“preprocessor”
is deliberately left clean as a result of this location is full of one of many preprocessors with a view to create a number of model-preprocessor mixtures. - hyperparameters – A set of hyperparameters which might be optimized by the HPO job.
- include_cls_metadata – Extra configuration particulars required by the SageMaker
Tuner
class.
A full instance of the fashions
dictionary is obtainable within the GitHub repository.
Subsequent, let’s iterate by the preprocessors
and fashions
dictionaries and create all attainable mixtures. For instance, in case your preprocessors
dictionary comprises 10 recipes and you’ve got 5 mannequin definitions within the fashions
dictionary, the newly created pipelines dictionary comprises 50 preprocessor-model pipelines which might be evaluated throughout HPO. Notice that particular person pipeline scripts should not created but at this level. The subsequent code block (cell 9) of the Jupyter pocket book iterates by all preprocessor-model objects within the pipelines
dictionary, inserts all related code items, and persists a pipeline-specific model of the script regionally within the pocket book. These scripts are used within the subsequent steps when creating particular person estimators that you simply plug into the HPO job.
Outline estimators
Now you can work on defining SageMaker Estimators that the HPO job makes use of after scripts are prepared. Let’s begin with making a wrapper class that defines some widespread properties for all estimators. It inherits from the SKLearn
class and specifies the function, occasion rely, and sort, in addition to which columns are utilized by the script as options and the goal.
Let’s construct the estimators
dictionary by iterating by all scripts generated earlier than and positioned within the scripts
listing. You instantiate a brand new estimator utilizing the SKLearnBase
class, with a novel estimator identify, and one of many scripts. Notice that the estimators
dictionary has two ranges: the highest stage defines a pipeline_family
. It is a logical grouping based mostly on the kind of fashions to guage and is the same as the size of the fashions
dictionary. The second stage comprises particular person preprocessor varieties mixed with the given pipeline_family
. This logical grouping is required when creating the HPO job.
Outline HPO tuner arguments
To optimize passing arguments into the HPO Tuner
class, the HyperparameterTunerArgs
knowledge class is initialized with arguments required by the HPO class. It comes with a set of capabilities, which guarantee HPO arguments are returned in a format anticipated when deploying a number of mannequin definitions without delay.
The subsequent code block makes use of the beforehand launched HyperparameterTunerArgs
knowledge class. You create one other dictionary referred to as hp_args
and generate a set of enter parameters particular to every estimator_family
from the estimators
dictionary. These arguments are used within the subsequent step when initializing HPO jobs for every mannequin household.
Create HPO tuner objects
On this step, you create particular person tuners for each estimator_family
. Why do you create three separate HPO jobs as an alternative of launching only one throughout all estimators? The HyperparameterTuner
class is restricted to 10 mannequin definitions connected to it. Due to this fact, every HPO is answerable for discovering the best-performing preprocessor for a given mannequin household and tuning that mannequin household’s hyperparameters.
The next are a couple of extra factors concerning the setup:
- The optimization technique is Bayesian, which implies that the HPO actively displays the efficiency of all trials and navigates the optimization in direction of extra promising hyperparameter mixtures. Early stopping needs to be set to Off or Auto when working with a Bayesian technique, which handles that logic itself.
- Every HPO job runs for a most of 100 jobs and runs 10 jobs in parallel. When you’re coping with bigger datasets, you would possibly wish to improve the entire variety of jobs.
- Moreover, you could wish to use settings that management how lengthy a job runs and what number of jobs your HPO is triggering. A technique to do this is to set the utmost runtime in seconds (for this publish, we set it to 1 hour). One other is to make use of the lately launched
TuningJobCompletionCriteriaConfig
. It affords a set of settings that monitor the progress of your jobs and resolve whether or not it’s probably that extra jobs will enhance the outcome. On this publish, we set the utmost variety of coaching jobs not enhancing to twenty. That means, if the rating isn’t enhancing (for instance, from the fortieth trial), you received’t must pay for the remaining trials tillmax_jobs
is reached.
Now let’s iterate by the tuners
and hp_args
dictionaries and set off all HPO jobs in SageMaker. Notice the utilization of the wait argument set to False
, which implies that the kernel received’t wait till the outcomes are full and you may set off all jobs without delay.
It’s probably that not all coaching jobs will full and a few of them could be stopped by the HPO job. The rationale for that is the TuningJobCompletionCriteriaConfig
—the optimization finishes if any of the desired standards is met. On this case, when the optimization standards isn’t enhancing for 20 consecutive jobs.
Analyze outcomes
Cell 15 of the pocket book checks if all HPO jobs are full and combines all leads to the type of a pandas knowledge body for additional evaluation. Earlier than analyzing the leads to element, let’s take a high-level take a look at the SageMaker console.
On the prime of the Hyperparameter tuning jobs web page, you possibly can see your three launched HPO jobs. All of them completed early and didn’t carry out all 100 coaching jobs. Within the following screenshot, you possibly can see that the Elastic-Web mannequin household accomplished the very best variety of trials, whereas others didn’t want so many coaching jobs to search out the perfect outcome.
You may open the HPO job to entry extra particulars, corresponding to particular person coaching jobs, job configuration, and the perfect coaching job’s data and efficiency.
Let’s produce a visualization based mostly on the outcomes to get extra insights of the AutoML workflow efficiency throughout all mannequin households.
From the next graph, you possibly can conclude that the Elastic-Web
mannequin’s efficiency was oscillating between 70,000 and 80,000 RMSE and finally stalled, because the algorithm wasn’t capable of enhance its efficiency regardless of attempting numerous preprocessing strategies and hyperparameter values. It additionally appears that RandomForest
efficiency diversified quite a bit relying on the hyperparameter set explored by HPO, however regardless of many trials it couldn’t go beneath the 50,000 RMSE error. GradientBoosting
achieved the perfect efficiency already from the beginning going beneath 50,000 RMSE. HPO tried to enhance that outcome additional however wasn’t capable of obtain higher efficiency throughout different hyperparameter mixtures. A basic conclusion for all HPO jobs is that not so many roles have been required to search out the perfect performing set of hyperparameters for every algorithm. To additional enhance the outcome, you would wish to experiment with creating extra options and performing extra function engineering.
It’s also possible to look at a extra detailed view of the model-preprocessor mixture to attract conclusions about probably the most promising mixtures.
Choose the perfect mannequin and deploy it
The next code snippet selects the perfect mannequin based mostly on the bottom achieved goal worth. You may then deploy the mannequin as a SageMaker endpoint.
Clear up
To forestall undesirable fees to your AWS account, we suggest deleting the AWS assets that you simply used on this publish:
- On the Amazon S3 console, empty the info from the S3 bucket the place the coaching knowledge was saved.
- On the SageMaker console, cease the pocket book occasion.
- Delete the mannequin endpoint in case you deployed it. Endpoints needs to be deleted when not in use, as a result of they’re billed by time deployed.
Conclusion
On this publish, we showcased the best way to create a {custom} HPO job in SageMaker utilizing a {custom} number of algorithms and preprocessing strategies. Specifically, this instance demonstrates the best way to automate the method of producing many coaching scripts and the best way to use Python programming buildings for environment friendly deployment of a number of parallel optimization jobs. We hope this answer will type the scaffolding of any {custom} mannequin tuning jobs you’ll deploy utilizing SageMaker to realize increased efficiency and pace up of your ML workflows.
Try the next assets to additional deepen your information of the best way to use SageMaker HPO:
Concerning the Authors
Konrad Semsch is a Senior ML Options Architect at Amazon Net Companies Information Lab Workforce. He helps prospects use machine studying to resolve their enterprise challenges with AWS. He enjoys inventing and simplifying to allow prospects with easy and pragmatic options for his or her AI/ML initiatives. He’s most captivated with MlOps and conventional knowledge science. Exterior of labor, he’s an enormous fan of windsurfing and kitesurfing.
Tuna Ersoy is a Senior Options Architect at AWS. Her main focus helps Public Sector prospects undertake cloud applied sciences for his or her workloads. She has a background in utility growth, enterprise structure, and get in touch with heart applied sciences. Her pursuits embrace serverless architectures and AI/ML.