Effectively prepare, tune, and deploy customized ensembles utilizing Amazon SageMaker
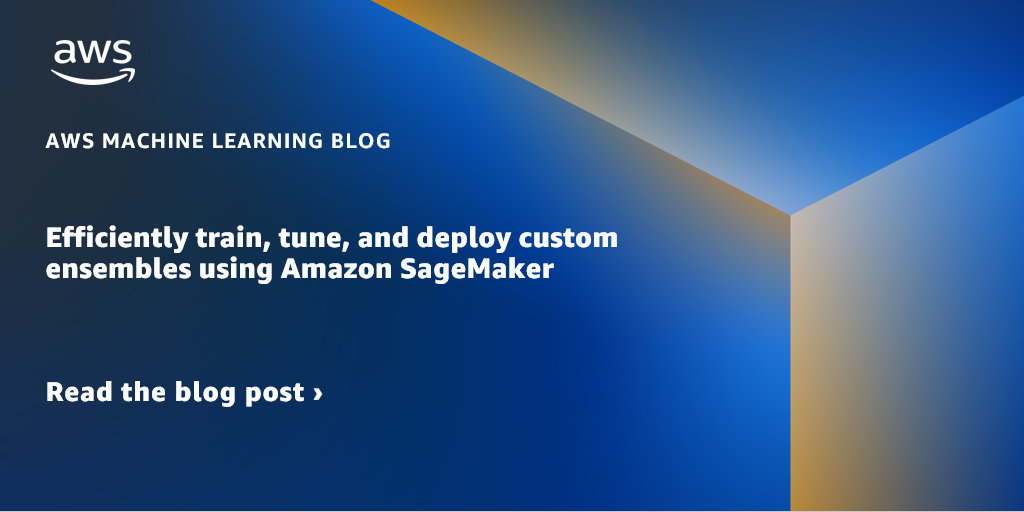
Synthetic intelligence (AI) has turn into an necessary and well-liked matter within the expertise group. As AI has advanced, we’ve seen several types of machine studying (ML) fashions emerge. One strategy, referred to as ensemble modeling, has been quickly gaining traction amongst information scientists and practitioners. On this submit, we talk about what ensemble fashions are and why their utilization may be useful. We then present an instance of how one can prepare, optimize, and deploy your customized ensembles utilizing Amazon SageMaker.
Ensemble studying refers to using a number of studying fashions and algorithms to achieve extra correct predictions than any single, particular person studying algorithm. They’ve been confirmed to be environment friendly in various purposes and studying settings comparable to cybersecurity [1] and fraud detection, distant sensing, predicting finest subsequent steps in monetary decision-making, medical analysis, and even pc imaginative and prescient and pure language processing (NLP) duties. We are likely to categorize ensembles by the methods used to coach them, their composition, and the way in which they merge the totally different predictions right into a single inference. These classes embody:
- Boosting – Coaching sequentially a number of weak learners, the place every incorrect prediction from earlier learners within the sequence is given the next weight and enter to the subsequent learner, thereby making a stronger learner. Examples embody AdaBoost, Gradient Boosting, and XGBoost.
- Bagging – Makes use of a number of fashions to scale back the variance of a single mannequin. Examples embody Random Forest and Further Timber.
- Stacking (mixing) – Usually makes use of heterogenous fashions, the place predictions of every particular person estimator are stacked collectively and used as enter to a remaining estimator that handles the prediction. This remaining estimator’s coaching course of usually makes use of cross-validation.
There are a number of strategies of mixing the predictions into the one one which the mannequin lastly produce, for instance, utilizing a meta-estimator comparable to linear learner, a voting methodology that makes use of a number of fashions to make a prediction based mostly on majority voting for classification duties, or an ensemble averaging for regression.
Though a number of libraries and frameworks present implementations of ensemble fashions, comparable to XGBoost, CatBoost, or scikit-learn’s random forest, on this submit we deal with bringing your personal fashions and utilizing them as a stacking ensemble. Nevertheless, as an alternative of utilizing devoted sources for every mannequin (devoted coaching and tuning jobs and internet hosting endpoints per mannequin), we prepare, tune, and deploy a customized ensemble (a number of fashions) utilizing a single SageMaker coaching job and a single tuning job, and deploy to a single endpoint, thereby decreasing doable price and operational overhead.
BYOE: Carry your personal ensemble
There are a number of methods to coach and deploy heterogenous ensemble fashions with SageMaker: you possibly can prepare every mannequin in a separate training job and optimize every mannequin individually utilizing Amazon SageMaker Automatic Model Tuning. When internet hosting these fashions, SageMaker supplies numerous cost-effective methods to host a number of fashions on the identical tenant infrastructure. Detailed deployment patterns for this sort of settings may be present in Model hosting patterns in Amazon SageMaker, Part 1: Common design patterns for building ML applications on Amazon SageMaker. These patterns embody utilizing a number of endpoints (for every skilled mannequin) or a single multi-model endpoint, or perhaps a single multi-container endpoint the place the containers may be invoked individually or chained in a pipeline. All these options embody a meta-estimator (for instance in an AWS Lambda perform) that invokes every mannequin and implements the mixing or voting perform.
Nevertheless, working a number of coaching jobs would possibly introduce operational and price overhead, particularly in case your ensemble requires coaching on the identical information. Equally, internet hosting totally different fashions on separate endpoints or containers and mixing their prediction outcomes for higher accuracy requires a number of invocations, and subsequently introduces extra administration, price, and monitoring efforts. For instance, SageMaker helps ensemble ML models using Triton Inference Server, however this answer requires the fashions or mannequin ensembles to be supported by the Triton backend. Moreover, extra efforts are required from the client to arrange the Triton server and extra studying to grasp how totally different Triton backends work. Subsequently, clients desire a extra simple approach to implement options the place they solely have to ship the invocation as soon as to the endpoint and have the flexibleness to manage how the outcomes are aggregated to generate the ultimate output.
Answer overview
To handle these considerations, we stroll by way of an instance of ensemble coaching utilizing a single coaching job, optimizing the mannequin’s hyperparameters and deploying it utilizing a single container to a serverless endpoint. We use two fashions for our ensemble stack: CatBoost and XGBoost (each of that are boosting ensembles). For our information, we use the diabetes dataset [2] from the scikit-learn library: It consists of 10 options (age, intercourse, physique mass, blood stress, and 6 blood serum measurements), and our mannequin predicts the illness development 1 yr after baseline options have been collected (a regression mannequin).
The complete code repository may be discovered on GitHub.
Practice a number of fashions in a single SageMaker job
For coaching our fashions, we use SageMaker coaching jobs in Script mode. With Script mode, you possibly can write customized coaching (and later inference code) whereas utilizing SageMaker framework containers. Framework containers allow you to make use of ready-made environments managed by AWS that embody all vital configuration and modules. To show how one can customise a framework container, for instance, we use the pre-built SKLearn container, which doesn’t embody the XGBoost and CatBoost packages. There are two choices so as to add these packages: both extend the built-in container to put in CatBoost and XGBoost (after which deploy as a customized container), or use the SageMaker coaching job script mode function, which lets you present a necessities.txt
file when creating the coaching estimator. The SageMaker coaching job installs the listed libraries within the necessities.txt
file throughout run time. This manner, you don’t have to handle your personal Docker picture repository and it supplies extra flexibility to working coaching scripts that want extra Python packages.
The next code block reveals the code we use to start out the coaching. The entry_point
parameter factors to our coaching script. We additionally use two of the SageMaker SDK API’s compelling options:
- First, we specify the native path to our supply listing and dependencies within the
source_dir
anddependencies
parameters, respectively. The SDK will compress and add these directories to Amazon Simple Storage Service (Amazon S3) and SageMaker will make them obtainable on the coaching occasion underneath the working listing/decide/ml/code
. - Second, we use the SDK
SKLearn
estimator object with our most popular Python and framework model, in order that SageMaker will pull the corresponding container. We have now additionally outlined a customized coaching metric ‘validation:rmse
‘, which shall be emitted within the coaching logs and captured by SageMaker. Later, we use this metric as the target metric within the tuning job.
hyperparameters = {"num_round": 6, "max_depth": 5}
estimator_parameters = {
"entry_point": "multi_model_hpo.py",
"source_dir": "code",
"dependencies": ["my_custom_library"],
"instance_type": training_instance_type,
"instance_count": 1,
"hyperparameters": hyperparameters,
"position": position,
"base_job_name": "xgboost-model",
"framework_version": "1.0-1",
"keep_alive_period_in_seconds": 60,
"metric_definitions":[
{'Name': 'validation:rmse', 'Regex': 'validation-rmse:(.*?);'}
]
}
estimator = SKLearn(**estimator_parameters)
Subsequent, we write our coaching script (multi_model_hpo.py). Our script follows a easy circulate: capture hyperparameters with which the job was configured and train the CatBoost model and XGBoost model. We additionally implement a k-fold cross validation perform. See the next code:
if __name__ == "__main__":
parser = argparse.ArgumentParser()
# Sagemaker particular arguments. Defaults are set within the atmosphere variables.
parser.add_argument("--output-data-dir", sort=str, default=os.environ["SM_OUTPUT_DATA_DIR"])
parser.add_argument("--model-dir", sort=str, default=os.environ["SM_MODEL_DIR"])
parser.add_argument("--train", sort=str, default=os.environ["SM_CHANNEL_TRAIN"])
parser.add_argument("--validation", sort=str, default=os.environ["SM_CHANNEL_VALIDATION"])
.
.
.
"""
Practice catboost
"""
Ok = args.k_fold
catboost_hyperparameters = {
"max_depth": args.max_depth,
"eta": args.eta,
}
rmse_list, model_catboost = cross_validation_catboost(train_df, Ok, catboost_hyperparameters)
.
.
.
"""
Practice the XGBoost mannequin
"""
hyperparameters = {
"max_depth": args.max_depth,
"eta": args.eta,
"goal": args.goal,
"num_round": args.num_round,
}
rmse_list, model_xgb = cross_validation(train_df, Ok, hyperparameters)
After the fashions are skilled, we calculate the imply of each the CatBoost and XGBoost predictions. The consequence, pred_mean
, is our ensemble’s remaining prediction. Then, we decide the mean_squared_error
towards the validation set. val_rmse
is used for the analysis of the entire ensemble throughout coaching. Discover that we additionally print the RMSE worth in a sample that matches the regex we used within the metric_definitions
. Later, SageMaker Computerized Mannequin Tuning will use that to seize the target metric. See the next code:
pred_mean = np.imply(np.array([pred_catboost, pred_xgb]), axis=0)
val_rmse = mean_squared_error(y_validation, pred_mean, squared=False)
print(f"Closing analysis consequence: validation-rmse:{val_rmse}")
Lastly, our script saves each mannequin artifacts to the output folder situated at /decide/ml/mannequin
.
When a coaching job is full, SageMaker packages and copies the content material of the /decide/ml/mannequin
listing as a single object in compressed TAR format to the S3 location that you just specified within the job configuration. In our case, SageMaker bundles the 2 fashions in a TAR file and uploads it to Amazon S3 on the finish of the coaching job. See the next code:
model_file_name="catboost-regressor-model.dump"
# Save CatBoost mannequin
path = os.path.be a part of(args.model_dir, model_file_name)
print('saving mannequin file to {}'.format(path))
mannequin.save_model(path)
.
.
.
# Save XGBoost mannequin
model_location = args.model_dir + "/xgboost-model"
pickle.dump(mannequin, open(model_location, "wb"))
logging.information("Saved skilled mannequin at {}".format(model_location))
In abstract, it is best to discover that on this process we downloaded the information one time and skilled two fashions utilizing a single coaching job.
Computerized ensemble mannequin tuning
As a result of we’re constructing a set of ML fashions, exploring the entire doable hyperparameter permutations is impractical. SageMaker presents Automatic Model Tuning (AMT), which appears for one of the best mannequin hyperparameters by specializing in essentially the most promising combos of values inside ranges that you just specify (it’s as much as you to outline the appropriate ranges to discover). SageMaker supports multiple optimization methods so that you can select from.
We begin by defining the 2 components of the optimization course of: the target metric and hyperparameters we wish to tune. In our instance, we use the validation RMSE because the goal metric and we tune eta
and max_depth
(for different hyperparameters, seek advice from XGBoost Hyperparameters and CatBoost hyperparameters):
from sagemaker.tuner import (
IntegerParameter,
ContinuousParameter,
HyperparameterTuner,
)
hyperparameter_ranges = {
"eta": ContinuousParameter(0.2, 0.3),
"max_depth": IntegerParameter(3, 4)
}
metric_definitions = [{"Name": "validation:rmse", "Regex": "validation-rmse:([0-9.]+)"}]
objective_metric_name = "validation:rmse"
We additionally want to make sure within the training script that our hyperparameters aren’t hardcoded and are pulled from the SageMaker runtime arguments:
catboost_hyperparameters = {
"max_depth": args.max_depth,
"eta": args.eta,
}
SageMaker additionally writes the hyperparameters to a JSON file and may be learn from /decide/ml/enter/config/hyperparameters.json
on the coaching occasion.
Like CatBoost, we additionally seize the hyperparameters for the XGBoost mannequin (discover that goal
and num_round
aren’t tuned):
catboost_hyperparameters = {
"max_depth": args.max_depth,
"eta": args.eta,
}
Lastly, we launch the hyperparameter tuning job utilizing these configurations:
tuner = HyperparameterTuner(
estimator,
objective_metric_name,
hyperparameter_ranges,
max_jobs=4,
max_parallel_jobs=2,
objective_type="Decrease"
)
tuner.match({"prepare": train_location, "validation": validation_location}, include_cls_metadata=False)
When the job is full, you possibly can retrieve the values for one of the best coaching job (with minimal RMSE):
job_name=tuner.latest_tuning_job.title
attached_tuner = HyperparameterTuner.connect(job_name)
attached_tuner.describe()["BestTrainingJob"]
For extra data on AMT, seek advice from Perform Automatic Model Tuning with SageMaker.
Deployment
To deploy our customized ensemble, we have to present a script to deal with the inference request and configure SageMaker internet hosting. On this instance, we used a single file that features each the coaching and inference code (multi_model_hpo.py). SageMaker makes use of the code underneath if _ title _ == "_ essential _"
for the coaching and the capabilities model_fn
, input_fn
, and predict_fn
when deploying and serving the mannequin.
Inference script
As with coaching, we use the SageMaker SKLearn framework container with our personal inference script. The script will implement three strategies required by SageMaker.
First, the model_fn
methodology reads our saved mannequin artifact recordsdata and masses them into reminiscence. In our case, the tactic returns our ensemble as all_model
, which is a Python record, however you can too use a dictionary with mannequin names as keys.
def model_fn(model_dir):
catboost_model = CatBoostRegressor()
catboost_model.load_model(os.path.be a part of(model_dir, model_file_name))
model_file = "xgboost-model"
mannequin = pickle.load(open(os.path.be a part of(model_dir, model_file), "rb"))
all_model = [catboost_model, model]
return all_model
Second, the input_fn
methodology deserializes the request enter information to be handed to our inference handler. For extra details about enter handlers, seek advice from Adapting Your Own Inference Container.
def input_fn(input_data, content_type):
dtype=None
payload = StringIO(input_data)
return np.genfromtxt(payload, dtype=dtype, delimiter=",")
Third, the predict_fn
methodology is answerable for getting predictions from the fashions. The strategy takes the mannequin and the information returned from input_fn
as parameters and returns the ultimate prediction. In our instance, we get the CatBoost consequence from the mannequin record first member (mannequin[0]
) and the XGBoost from the second member (mannequin[1]
), and we use a mixing perform that returns the imply of each predictions:
def predict_fn(input_data, mannequin):
predictions_catb = mannequin[0].predict(input_data)
dtest = xgb.DMatrix(input_data)
predictions_xgb = mannequin[1].predict(dtest,
ntree_limit=getattr(mannequin, "best_ntree_limit", 0),
validate_features=False)
return np.imply(np.array([predictions_catb, predictions_xgb]), axis=0)
Now that we’ve our skilled fashions and inference script, we are able to configure the atmosphere to deploy our ensemble.
SageMaker Serverless Inference
Though there are many hosting options in SageMaker, on this instance, we use a serverless endpoint. Serverless endpoints routinely launch compute sources and scale them out and in relying on visitors. This takes away the undifferentiated heavy lifting of managing servers. This feature is right for workloads which have idle durations between visitors spurts and may tolerate chilly begins.
Configuring the serverless endpoint is easy as a result of we don’t want to decide on occasion varieties or handle scaling insurance policies. We solely want to supply two parameters: reminiscence measurement and most concurrency. The serverless endpoint routinely assigns compute sources proportional to the reminiscence you choose. If you happen to select a bigger reminiscence measurement, your container has entry to extra vCPUs. It is best to all the time select your endpoint’s reminiscence measurement based on your mannequin measurement. The second parameter we have to present is most concurrency. For a single endpoint, this parameter may be set as much as 200 (as of this writing, the restrict for whole variety of serverless endpoints in a Area is 50). It is best to word that the utmost concurrency for a person endpoint prevents that endpoint from taking over all of the invocations allowed on your account, as a result of any endpoint invocations past the utmost are throttled (for extra details about the entire concurrency for all serverless endpoints per Area, seek advice from Amazon SageMaker endpoints and quotas).
from sagemaker.serverless.serverless_inference_config import ServerlessInferenceConfig
serverless_config = ServerlessInferenceConfig(
memory_size_in_mb=6144,
max_concurrency=1,
)
Now that we configured the endpoint, we are able to lastly deploy the mannequin that was chosen in our hyperparameter optimization job:
estimator=attached_tuner.best_estimator()
predictor = estimator.deploy(serverless_inference_config=serverless_config)
Clear up
Though serverless endpoints have zero price when not getting used, when you’ve got completed working this instance, it is best to be sure that to delete the endpoint:
predictor.delete_endpoint(predictor.endpoint)
Conclusion
On this submit, we coated one strategy to coach, optimize, and deploy a customized ensemble. We detailed the method of utilizing a single coaching job to coach a number of fashions, use automated mannequin tuning to optimize the ensemble hyperparameters, and deploy a single serverless endpoint that blends the inferences from a number of fashions.
Utilizing this methodology solves potential price and operational points. The price of a coaching job relies on the sources you employ at some stage in utilization. By downloading the information solely as soon as for coaching the 2 fashions, we decreased by half the job’s information obtain part and the used quantity that shops the information, thereby decreasing the coaching job’s general price. Moreover, the AMT job ran 4 coaching jobs, every with the aforementioned decreased time and storage, in order that signify 4 occasions in price saving! With regard to mannequin deployment on a serverless endpoint, since you additionally pay for the quantity of knowledge processed, by invoking the endpoint solely as soon as for 2 fashions, you pay half of the I/O information prices.
Though this submit solely confirmed the advantages with two fashions, you should use this methodology to coach, tune, and deploy quite a few ensemble fashions to see a fair higher impact.
References
[1] Raj Kumar, P. Arun; Selvakumar, S. (2011). “Distributed denial of service assault detection utilizing an ensemble of neural classifier”. Pc Communications. 34 (11): 1328–1341. doi:10.1016/j.comcom.2011.01.012.
[2] Bradley Efron, Trevor Hastie, Iain Johnstone and Robert Tibshirani (2004) “Least Angle Regression,” Annals of Statistics (with dialogue), 407-499. (https://web.stanford.edu/~hastie/Papers/LARS/LeastAngle_2002.pdf)
In regards to the Authors
Melanie Li, PhD, is a Senior AI/ML Specialist TAM at AWS based mostly in Sydney, Australia. She helps enterprise clients to construct options leveraging the state-of-the-art AI/ML instruments on AWS and supplies steerage on architecting and implementing machine studying options with finest practices. In her spare time, she likes to discover nature outdoor and spend time with household and mates.
Uri Rosenberg is the AI & ML Specialist Technical Supervisor for Europe, Center East, and Africa. Based mostly out of Israel, Uri works to empower enterprise clients to design, construct, and function ML workloads at scale. In his spare time, he enjoys biking, climbing, and minimizing RMSEs.