What I Realized Pushing Immediate Engineering to the Restrict | by Jacob Marks, Ph.D. | Jun, 2023
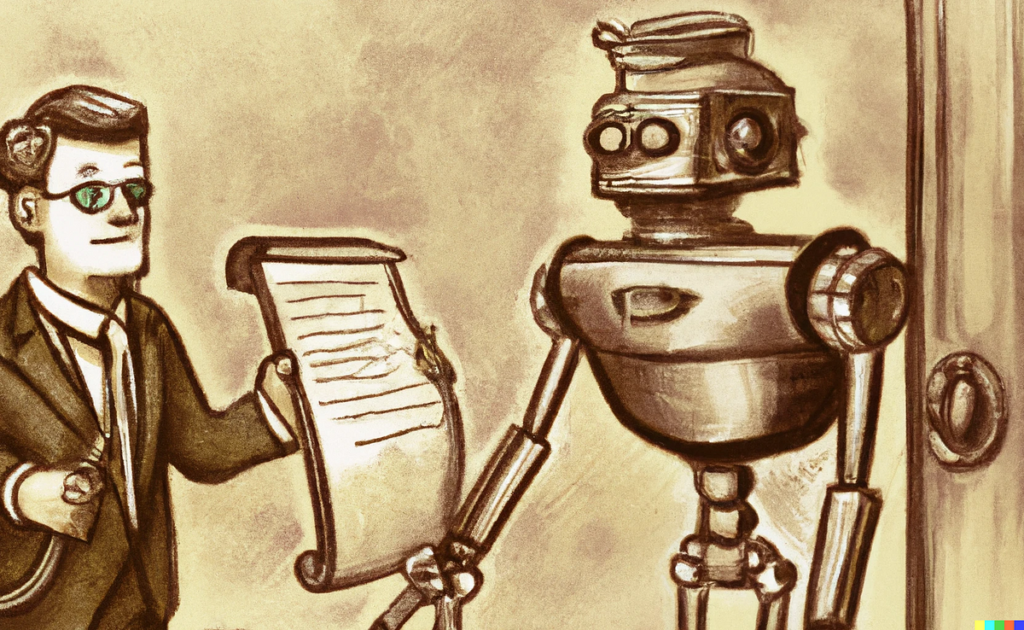
I spent the previous two months constructing a large-language-model (LLM) powered utility. It was an thrilling, intellectually stimulating, and at occasions irritating expertise. My whole conception of immediate engineering — and of what’s potential with LLMs — modified over the course of the venture.
I’d like to share with you a few of my largest takeaways with the objective of shedding gentle on a number of the typically unstated elements of immediate engineering. I hope that after studying about my trials and tribulations, it is possible for you to to make extra knowledgeable immediate engineering selections. If you happen to’d already dabbled in immediate engineering, I hope that this helps you push ahead in your individual journey!
For context, right here is the TL;DR on the venture we’ll be studying from:
- My staff and I constructed VoxelGPT, an utility that mixes LLMs with the FiftyOne pc imaginative and prescient question language to allow looking by way of picture and video datasets by way of pure language. VoxelGPT additionally solutions questions on FiftyOne itself.
- VoxelGPT is open supply (so is FiftyOne!). All the code is available on GitHub.
- You may strive VoxelGPT at no cost at gpt.fiftyone.ai.
- If you happen to’re curious how we constructed VoxelGPT, you possibly can read more about it on TDS here.
Now, I’ve break up the immediate engineering classes into 4 classes:
Science? Engineering? Black Magic?
Immediate engineering is as a lot experimentation as it’s engineering. There are an infinite variety of methods to write down a immediate, from the particular wording of your query, to the content material and formatting of the context you feed in. It may be overwhelming. I discovered it best to begin easy and construct up an instinct — after which check out hypotheses.
In pc imaginative and prescient, every dataset has its personal schema, label varieties, and sophistication names. The objective for VoxelGPT was to have the ability to work with any pc imaginative and prescient dataset, however we began with only a single dataset: MS COCO. Holding the entire extra levels of freedom mounted allowed us to nail down into the LLM’s skill to write down syntactically appropriate queries within the first place.
When you’ve decided a formulation that’s profitable in a restricted context, then work out the way to generalize and construct upon this.
Which Mannequin(s) to Use?
Folks say that one of the vital essential traits of huge language fashions is that they’re comparatively interchangeable. In idea, you must have the ability to swap one LLM out for an additional with out considerably altering the connective tissue.
Whereas it’s true that altering the LLM you utilize is usually so simple as swapping out an API name, there are undoubtedly some difficulties that come up in observe.
- Some fashions have a lot shorter context lengths than others. Switching to a mannequin with a shorter context can require main refactoring.
- Open supply is nice, however open supply LLMs should not as performant (but) as GPT fashions. Plus, in case you are deploying an utility with an open supply LLM, you will want to ensure the container working the mannequin has sufficient reminiscence and storage. This will find yourself being extra troublesome (and costlier) than simply utilizing API endpoints.
- If you happen to begin utilizing GPT-4 after which change to GPT-3.5 due to value, chances are you’ll be shocked by the drop-off in efficiency. For sophisticated code era and inference duties, GPT-4 is MUCH higher.
The place to Use LLMs?
Massive language fashions are highly effective. However simply because they could be able to sure duties doesn’t imply it is advisable to — and even ought to — use them for these duties. One of the best ways to consider LLMs is as enablers. LLMs should not the WHOLE answer: they’re simply part of it. Don’t count on massive language fashions to do all the things.
For example, it could be the case that the LLM you’re utilizing can (underneath excellent circumstances) generate correctly formatted API calls. But when you recognize what the construction of the API name ought to appear to be, and you’re really serious about filling in sections of the API name (variable names, situations, and so forth.), then simply use the LLM to do these duties, and use the (correctly post-processed) LLM outputs to generate structured API calls your self. This shall be cheaper, extra environment friendly, and extra dependable.
A whole system with LLMs will certainly have a variety of connective tissue and classical logic, plus a slew of conventional software program engineering and ML engineering parts. Discover what works greatest to your utility.
LLMs Are Biased
Language fashions are each inference engines and data shops. Oftentimes, the data retailer facet of an LLM might be of nice curiosity to customers — many individuals use LLMs as search engine replacements! By now, anybody who has used an LLM is aware of that they’re inclined to creating up pretend “information” — a phenomenon known as hallucination.
Generally, nevertheless, LLMs endure from the alternative drawback: they’re too firmly fixated on information from their coaching knowledge.
In our case, we have been making an attempt to immediate GPT-3.5 to find out the suitable ViewStages (pipelines of logical operations) required in changing a consumer’s pure language question into a sound FiftyOne Python question. The issue was that GPT-3.5 knew concerning the `Match` and `FilterLabels` ViewStages, which have existed in FiftyOne for a while, however its coaching knowledge did not embrace lately added performance whereby a `SortBySimilarity` ViewStage can be utilized to search out pictures the resemble a textual content immediate.
We tried passing in a definition of `SortBySimilarity`, particulars about its utilization, and examples. We even tried instructing GPT-3.5 that it MUST NOT use the `Match` or `FilterLabels` ViewStages, or else it is going to be penalized. It doesn’t matter what we tried, the LLM nonetheless oriented itself in direction of what it knew, whether or not it was the proper alternative or not. We have been preventing towards the LLM’s instincts!
We ended up having to cope with this difficulty in post-processing.
Painful Publish-Processing Is Inevitable
Regardless of how good your examples are; irrespective of how strict your prompts are — massive language fashions will invariably hallucinate, offer you improperly formatted responses, and throw a tantrum after they don’t perceive enter info. Probably the most predictable property of LLMs is the unpredictability of their outputs.
I spent an ungodly period of time writing routines to sample match for and proper hallucinated syntax. The post-processing file ended up containing nearly 1600 strains of Python code!
A few of these subroutines have been as easy as including parenthesis, or altering “and” and “or” to “&” and “|” in logical expressions. Some subroutines have been way more concerned, like validating the names of the entities within the LLM’s responses, changing one ViewStage to a different if sure situations have been met, guaranteeing that the numbers and sorts of arguments to strategies have been legitimate.
In case you are utilizing immediate engineering in a considerably confined code era context, I’d suggest the next strategy:
- Write your individual customized error parser utilizing Summary Syntax Bushes (Python’s ast module).
- If the outcomes are syntactically invalid, feed the generated error message into your LLM and have it strive once more.
This strategy fails to handle the extra insidious case the place syntax is legitimate however the outcomes should not proper. If anybody has a very good suggestion for this (past AutoGPT and “present your work” fashion approaches), please let me know!
The Extra the Merrier
To construct VoxelGPT, I used what appeared like each prompting method underneath the solar:
- “You might be an skilled”
- “Your job is”
- “You MUST”
- “You’ll be penalized”
- “Listed here are the foundations”
No mixture of such phrases will guarantee a sure kind of conduct. Intelligent prompting simply isn’t sufficient.
That being stated, the extra of those methods you use in a immediate, the extra you nudge the LLM in the proper path!
Examples > Documentation
It’s common data by now (and customary sense!) that each examples and different contextual info like documentation may also help elicit higher responses from a big language mannequin. I discovered this to be the case for VoxelGPT.
When you add the entire instantly pertinent examples and documentation although, what do you have to do when you’ve got additional room within the context window? In my expertise, I discovered that tangentially associated examples mattered greater than tangentially associated documentation.
Modularity >> Monolith
The extra you possibly can break down an overarching drawback into smaller subproblems, the higher. Quite than feeding the dataset schema and a listing of end-to-end examples, it’s far more efficient to determine particular person choice and inference steps (selection-inference prompting), and feed in solely the related info at every step.
That is preferable for 3 causes:
- LLMs are higher at doing one job at a time than a number of duties directly.
- The smaller the steps, the better to sanitize inputs and outputs.
- It is a vital train for you because the engineer to know the logic of your utility. The purpose of LLMs isn’t to make the world a black field. It’s to allow new workflows.
How Many Do I Want?
A giant a part of immediate engineering is determining what number of examples you want for a given job. That is extremely drawback particular.
For some duties (effective query generation and answering questions based on the FiftyOne documentation), we have been capable of get away with out any examples. For others (tag selection, whether or not chat history is relevant, and named entity recognition for label classes) we simply wanted just a few examples to get the job completed. Our principal inference job, nevertheless, has nearly 400 examples (and that’s nonetheless the limiting consider general efficiency), so we solely move in essentially the most related examples at inference time.
When you’re producing examples, attempt to comply with two tips:
- Be as complete as potential. When you’ve got a finite house of potentialities, then attempt to give the LLM at the very least one instance for every case. For VoxelGPT, we tried to have on the very least one instance for every syntactically appropriate approach of utilizing every ViewStage — and sometimes just a few examples for every, so the LLM can do sample matching.
- Be as constant as potential. In case you are breaking the duty down into a number of subtasks, be sure the examples are constant from one job to the subsequent. You may reuse examples!
Artificial Examples
Producing examples is a laborious course of, and handcrafted examples can solely take you to this point. It’s simply not potential to consider each potential state of affairs forward of time. If you deploy your utility, you possibly can log consumer queries and use these to enhance your instance set.
Previous to deployment, nevertheless, your greatest guess is perhaps to generate artificial examples.
Listed here are two approaches to producing artificial examples that you simply would possibly discover useful:
- Use an LLM to generate examples. You may ask the LLM to fluctuate its language, and even imitate the fashion of potential customers! This didn’t work for us, however I’m satisfied it might work for a lot of functions.
- Programmatically generate examples — doubtlessly with randomness — primarily based on components within the enter question itself. For VoxelGPT, this implies producing examples primarily based on the fields within the consumer’s dataset. We’re within the strategy of incorporating this into our pipeline, and the outcomes we’ve seen to this point have been promising.
LangChain
LangChain is widespread for a motive: the library makes it simple to attach LLM inputs and outputs in complicated methods, abstracting away the gory particulars. The Fashions and Prompts modules particularly are high notch.
That being stated, LangChain is unquestionably a piece in progress: their Recollections, Indexes, and Chains modules all have vital limitations. Listed here are only a few of the problems I encountered when making an attempt to make use of LangChain:
- Doc Loaders and Textual content Splitters: In LangChain, Document Loaders are supposed to remodel knowledge from completely different file codecs into textual content, and Text Splitters are supposed to separate textual content into semantically significant chunks. VoxelGPT solutions questions concerning the FiftyOne documentation by retrieving essentially the most related chunks of the docs and piping them right into a immediate. To be able to generate significant solutions to questions concerning the FiftyOne docs, I needed to successfully construct customized loaders and splitters, as a result of LangChain didn’t present the suitable flexibility.
- Vectorstores: LangChain affords Vectorstore integrations and Vectorstore-based Retrievers to assist discover related info to include into LLM prompts. That is nice in idea, however the implementations are missing in flexibility. I needed to write a customized implementation with ChromaDB with the intention to move embedding vectors forward of time and never have them recomputed each time I ran the appliance. I additionally needed to write a customized retriever to implement the customized pre-filtering I wanted.
- Query Answering with Sources: When constructing out query answering over the FiftyOne docs, I arrived at an inexpensive answer using LangChain’s `RetrievalQA` Chain. Once I wished so as to add sources in, I assumed it could be as easy as swapping out that chain for LangChain’s `RetrievalQAWithSourcesChain`. Nevertheless, unhealthy prompting methods meant that this chain exhibited some unlucky conduct, comparable to hallucinating about Michael Jackson. As soon as once more, I needed to take matters into my own hands.
What does all of this imply? It might be simpler to only construct the parts your self!
Vector Databases
Vector search could also be on 🔥🔥🔥, however that doesn’t imply you NEED it to your venture. I initially applied our comparable instance retrieval routine utilizing ChromaDB, however as a result of we solely had a whole bunch of examples, I ended up switching to an actual nearest neighbor search. I did must cope with the entire metadata filtering myself, however the end result was a quicker routine with fewer dependencies.
TikToken
Including TikToken into the equation was extremely simple. In complete, TikToken added <10 strains of code to the venture, however allowed us to be far more exact when counting tokens and making an attempt to suit as a lot info as potential into the context size. That is the one true no-brainer in relation to tooling.
There are tons of LLMs to select from, numerous shiny new instruments, and a bunch of “immediate engineering” methods. All of this may be each thrilling and overwhelming. The important thing to constructing an utility with immediate engineering is to:
- Break the issue down; construct the answer up
- Deal with LLMs as enablers, not as end-to-end options
- Solely use instruments after they make your life simpler
- Embrace experimentation!
Go construct one thing cool!