Posit AI Weblog: Optimizers in torch
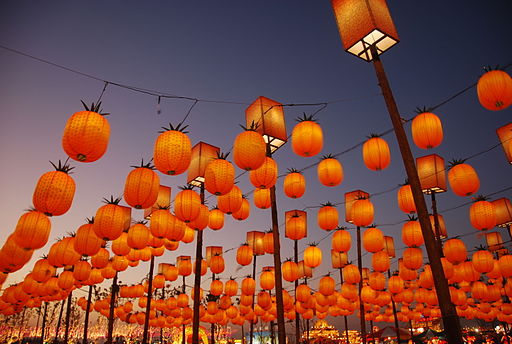
That is the fourth and final installment in a sequence introducing torch
fundamentals. Initially, we focused on tensors. For instance their energy, we coded a whole (if toy-size) neural community from scratch. We didn’t make use of any of torch
’s higher-level capabilities – not even autograd, its automatic-differentiation function.
This modified within the follow-up post. No extra fascinated with derivatives and the chain rule; a single name to backward()
did all of it.
In the third post, the code once more noticed a significant simplification. As an alternative of tediously assembling a DAG by hand, we let modules care for the logic.
Based mostly on that final state, there are simply two extra issues to do. For one, we nonetheless compute the loss by hand. And secondly, despite the fact that we get the gradients all properly computed from autograd, we nonetheless loop over the mannequin’s parameters, updating all of them ourselves. You received’t be shocked to listen to that none of that is mandatory.
Losses and loss capabilities
torch
comes with all the same old loss capabilities, reminiscent of imply squared error, cross entropy, Kullback-Leibler divergence, and the like. Typically, there are two utilization modes.
Take the instance of calculating imply squared error. A method is to name nnf_mse_loss()
immediately on the prediction and floor reality tensors. For instance:
torch_tensor
0.682362
[ CPUFloatType{} ]
Different loss capabilities designed to be known as immediately begin with nnf_
as properly: nnf_binary_cross_entropy()
, nnf_nll_loss()
, nnf_kl_div()
… and so forth.
The second means is to outline the algorithm prematurely and name it at some later time. Right here, respective constructors all begin with nn_
and finish in _loss
. For instance: nn_bce_loss()
, nn_nll_loss(),
nn_kl_div_loss()
…
loss <- nn_mse_loss()
loss(x, y)
torch_tensor
0.682362
[ CPUFloatType{} ]
This methodology could also be preferable when one and the identical algorithm must be utilized to multiple pair of tensors.
Optimizers
To date, we’ve been updating mannequin parameters following a easy technique: The gradients informed us which route on the loss curve was downward; the training charge informed us how huge of a step to take. What we did was an easy implementation of gradient descent.
Nevertheless, optimization algorithms utilized in deep studying get much more subtle than that. Beneath, we’ll see substitute our handbook updates utilizing optim_adam()
, torch
’s implementation of the Adam algorithm (Kingma and Ba 2017). First although, let’s take a fast have a look at how torch
optimizers work.
Here’s a quite simple community, consisting of only one linear layer, to be known as on a single information level.
information <- torch_randn(1, 3)
mannequin <- nn_linear(3, 1)
mannequin$parameters
$weight
torch_tensor
-0.0385 0.1412 -0.5436
[ CPUFloatType{1,3} ]
$bias
torch_tensor
-0.1950
[ CPUFloatType{1} ]
Once we create an optimizer, we inform it what parameters it’s presupposed to work on.
optimizer <- optim_adam(mannequin$parameters, lr = 0.01)
optimizer
<optim_adam>
Inherits from: <torch_Optimizer>
Public:
add_param_group: operate (param_group)
clone: operate (deep = FALSE)
defaults: listing
initialize: operate (params, lr = 0.001, betas = c(0.9, 0.999), eps = 1e-08,
param_groups: listing
state: listing
step: operate (closure = NULL)
zero_grad: operate ()
At any time, we will examine these parameters:
optimizer$param_groups[[1]]$params
$weight
torch_tensor
-0.0385 0.1412 -0.5436
[ CPUFloatType{1,3} ]
$bias
torch_tensor
-0.1950
[ CPUFloatType{1} ]
Now we carry out the ahead and backward passes. The backward cross calculates the gradients, however does not replace the parameters, as we will see each from the mannequin and the optimizer objects:
out <- mannequin(information)
out$backward()
optimizer$param_groups[[1]]$params
mannequin$parameters
$weight
torch_tensor
-0.0385 0.1412 -0.5436
[ CPUFloatType{1,3} ]
$bias
torch_tensor
-0.1950
[ CPUFloatType{1} ]
$weight
torch_tensor
-0.0385 0.1412 -0.5436
[ CPUFloatType{1,3} ]
$bias
torch_tensor
-0.1950
[ CPUFloatType{1} ]
Calling step()
on the optimizer truly performs the updates. Once more, let’s test that each mannequin and optimizer now maintain the up to date values:
optimizer$step()
optimizer$param_groups[[1]]$params
mannequin$parameters
NULL
$weight
torch_tensor
-0.0285 0.1312 -0.5536
[ CPUFloatType{1,3} ]
$bias
torch_tensor
-0.2050
[ CPUFloatType{1} ]
$weight
torch_tensor
-0.0285 0.1312 -0.5536
[ CPUFloatType{1,3} ]
$bias
torch_tensor
-0.2050
[ CPUFloatType{1} ]
If we carry out optimization in a loop, we’d like to verify to name optimizer$zero_grad()
on each step, as in any other case gradients could be collected. You’ll be able to see this in our ultimate model of the community.
Easy community: ultimate model
library(torch)
### generate coaching information -----------------------------------------------------
# enter dimensionality (variety of enter options)
d_in <- 3
# output dimensionality (variety of predicted options)
d_out <- 1
# variety of observations in coaching set
n <- 100
# create random information
x <- torch_randn(n, d_in)
y <- x[, 1, NULL] * 0.2 - x[, 2, NULL] * 1.3 - x[, 3, NULL] * 0.5 + torch_randn(n, 1)
### outline the community ---------------------------------------------------------
# dimensionality of hidden layer
d_hidden <- 32
mannequin <- nn_sequential(
nn_linear(d_in, d_hidden),
nn_relu(),
nn_linear(d_hidden, d_out)
)
### community parameters ---------------------------------------------------------
# for adam, want to decide on a a lot greater studying charge on this drawback
learning_rate <- 0.08
optimizer <- optim_adam(mannequin$parameters, lr = learning_rate)
### coaching loop --------------------------------------------------------------
for (t in 1:200) {
### -------- Ahead cross --------
y_pred <- mannequin(x)
### -------- compute loss --------
loss <- nnf_mse_loss(y_pred, y, discount = "sum")
if (t %% 10 == 0)
cat("Epoch: ", t, " Loss: ", loss$merchandise(), "n")
### -------- Backpropagation --------
# Nonetheless have to zero out the gradients earlier than the backward cross, solely this time,
# on the optimizer object
optimizer$zero_grad()
# gradients are nonetheless computed on the loss tensor (no change right here)
loss$backward()
### -------- Replace weights --------
# use the optimizer to replace mannequin parameters
optimizer$step()
}
And that’s it! We’ve seen all the foremost actors on stage: tensors, autograd, modules, loss capabilities, and optimizers. In future posts, we’ll discover use torch for traditional deep studying duties involving pictures, textual content, tabular information, and extra. Thanks for studying!