Create SageMaker Pipelines for coaching, consuming and monitoring your batch use instances
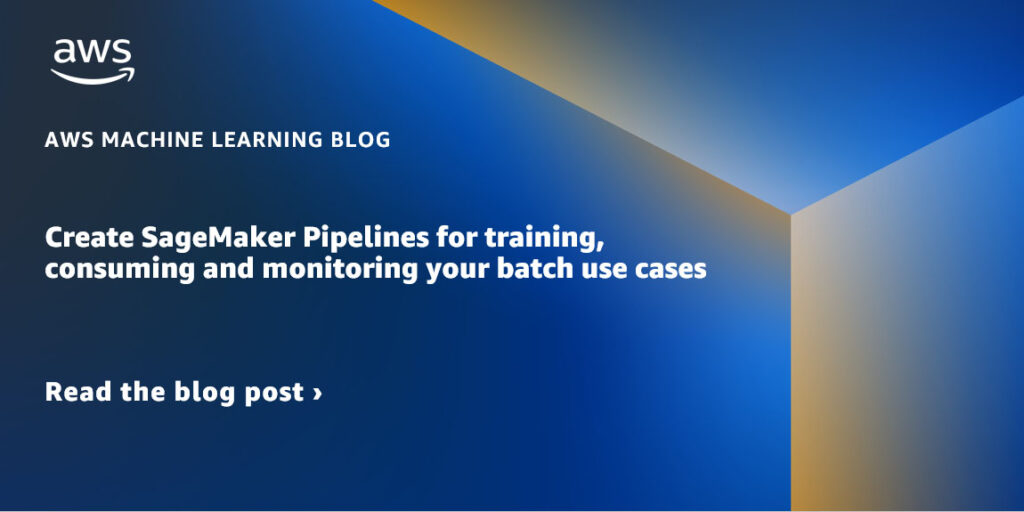
Batch inference is a standard sample the place prediction requests are batched collectively on enter, a job runs to course of these requests in opposition to a skilled mannequin, and the output contains batch prediction responses that may then be consumed by different functions or enterprise capabilities. Working batch use instances in manufacturing environments requires a repeatable course of for mannequin retraining in addition to batch inference. That course of must also embrace monitoring that mannequin to measure efficiency over time.
On this publish, we present the way to create repeatable pipelines to your batch use instances utilizing Amazon SageMaker Pipelines, Amazon SageMaker model registry, SageMaker batch transform jobs, and Amazon SageMaker Model Monitor. This resolution highlights the power to make use of the totally managed options inside SageMaker MLOps to scale back operational overhead by totally managed and built-in capabilities.
Resolution overview
There are a number of eventualities for performing batch inference. In some instances, you might be retraining your mannequin each time you run batch inference. Alternatively, you might be coaching your mannequin much less steadily than you might be performing batch inference. On this publish, we concentrate on the second situation. For this instance, let’s assume you will have a mannequin that’s skilled periodically, roughly one time per 30 days. Nonetheless, batch inference is carried out in opposition to the most recent mannequin model every day. This can be a frequent situation, through which the mannequin coaching lifecycle is completely different than the batch inference lifecycle.
The structure supporting the launched batch situation incorporates two separate SageMaker pipelines, as proven within the following diagram.
We use the primary pipeline to coach the mannequin and baseline the coaching information. We use the generated baseline for ongoing monitoring within the second pipeline. The primary pipeline contains the steps wanted to organize information, prepare the mannequin, and consider the efficiency of the mannequin. If the mannequin performs acceptably in keeping with the analysis standards, the pipeline continues with a step to baseline the info utilizing a built-in SageMaker Pipelines step. For the data drift Model Monitor kind, the baselining step makes use of a SageMaker managed container picture to generate statistics and constraints based mostly in your coaching information. This baseline is then used to watch for alerts of knowledge drift throughout batch inference. Lastly, the primary pipeline completes when a brand new mannequin model is registered into the SageMaker mannequin registry. At this level, the mannequin will be permitted mechanically, or a secondary guide approval will be required based mostly on a peer evaluate of mannequin efficiency and every other recognized standards.
Within the second pipeline, step one queries the mannequin registry for the most recent permitted mannequin model and runs the info monitoring job, which compares the info baseline generated from the primary pipeline with the present enter information. The ultimate step within the pipeline is performing batch inference in opposition to the most recent permitted mannequin.
The next diagram illustrates the answer structure for every pipeline.
For our dataset, we use a synthetic dataset from a telecommunications cell phone service. This pattern dataset incorporates 5,000 data, the place every document makes use of 21 attributes to explain the client profile. The final attribute, Churn
, is the attribute that we wish the ML mannequin to foretell. The goal attribute is binary, that means the mannequin predicts the output as certainly one of two classes (True
or False
).
The next GitHub repo incorporates the code for demonstrating the steps carried out in every pipeline. It incorporates three notebooks: to carry out the preliminary setup, to create the mannequin prepare and baseline pipeline, and create the batch inference and Mannequin Monitor pipeline. The repository additionally contains further Python supply code with helper capabilities, used within the setup pocket book, to arrange required permissions.
Stipulations
The next screenshot lists some permission insurance policies which might be required by the SageMaker execution position for the workflow. You’ll be able to allow these permission insurance policies by AWS Identity and Access Management (IAM) position permissions.
AmazonSageMaker-ExecutionPolicy-<...>
is the execution position related to the SageMaker person and has the mandatory Amazon Simple Storage Service (Amazon S3) bucket insurance policies. Custom_IAM_roles_policy and Custom_Lambda_policy are two customized insurance policies created to help the required actions for the AWS Lambda perform. So as to add the 2 customized insurance policies, go to the suitable position (related along with your SageMaker person) in IAM, click on on Add permissions after which Create inline coverage. Then, select JSON inside Create coverage, add the coverage code for first customized coverage and save the coverage. Repeat the identical for the second customized coverage.
0.Setup.ipynb is a prerequisite pocket book required earlier than operating notebooks 1 and a couple of. The code units up the S3 paths for pipeline inputs, outputs, and mannequin artifacts, and uploads scripts used inside the pipeline steps. This pocket book additionally makes use of one of many offered helper capabilities, create_lambda_role
, to create a Lambda position that’s utilized in pocket book 2, 2.SageMakerPipeline-ModelMonitoring-DataQuality-BatchTransform.ipynb
. See the next code:
After you’ve efficiently accomplished all the duties within the setup pocket book, you’re able to construct the primary pipeline to coach and baseline the mannequin.
Pipeline 1: Prepare and baseline pipeline
On this part, we take a deep dive into the SageMaker pipeline used to coach and baseline the mannequin. The required steps and code are within the 1.SageMakerPipeline-BaselineData-Train.ipynb pocket book. This pipeline takes the uncooked buyer churn information as enter, after which performs the steps required to organize the info, prepare the mannequin, consider the mannequin, baseline the mannequin, and register the mannequin within the mannequin registry.
To construct a SageMaker pipeline, you configure the underlying job (equivalent to SageMaker Processing), configure the pipeline steps to run the job, after which configure and run the pipeline. We full the next steps:
- Configure the mannequin construct pipeline to organize the info, prepare the mannequin, and consider the mannequin.
- Configure the baseline step for the info drift with Mannequin Monitor.
- Configure steps to bundle the mannequin and register the mannequin model.
- Configure a conditional step to judge mannequin efficiency.
Configure the mannequin construct pipeline
The mannequin construct pipeline is a three-step course of:
- Put together the info.
- Prepare the mannequin.
- Consider the mannequin.
To arrange the info, we configure an information processing step. This step runs a SageMaker Processing job, utilizing the built-in ProcessingStep, to organize the uncooked information on enter for coaching and analysis.
To coach the mannequin, we configure a coaching job step. This step runs a SageMaker Coaching job, utilizing the built-in TrainingStep. For this use case, we carry out binary classification utilizing XGBoost. The output of this step is a mannequin artifact, mannequin.tar.gz
, saved in Amazon S3.
The final step is liable for evaluating mannequin efficiency utilizing the check holdout dataset. This step makes use of the built-in ProcessingStep
with the offered code, analysis.py
, to judge efficiency metrics (accuracy, space beneath curve).
Configure the baseline step
To observe the mannequin and information, a baseline is required.
Monitoring for information drift requires a baseline of coaching information. The baseline step makes use of Pipelines’ built-in QualityCheckStep. This step mechanically runs a SageMaker Processing job that makes use of the Mannequin Monitor pre-built container picture. We use this similar container picture for the baselining in addition to the mannequin monitoring; nonetheless, the parameters used throughout configuration of this step direct the suitable conduct. On this case, we’re baselining the info, so we have to be certain that the quality_check_config
parameter is utilizing DataQualityCheckConfig
, which identifies the S3 enter and output paths. We’re additionally setting register_new_baseline
and skip_check
to true
. When these values are each set to true
, it tells SageMaker to run this step as a baseline job and create a brand new baseline. To get a greater understanding of the parameters that management the conduct of the SageMaker pre-built container picture, check with Baseline calculation, drift detection and lifecycle with ClarifyCheck and QualityCheck steps in Amazon SageMaker Model Building Pipelines.
See the next code:
This step generates two JSON recordsdata as output:
- statistics.json – Accommodates calculated statistics for every function of the coaching dataset
- constraints.json – Suggests information constraints based mostly on the statistics collected
These constraints can be modified and are used to detect alerts of drift throughout mannequin monitoring.
Configure steps to bundle and register the mannequin model
Subsequent, we configure the steps to bundle for deployment and register the mannequin within the mannequin registry utilizing two further pipeline steps.
The bundle mannequin step packages the mannequin to be used with the SageMaker batch remodel deployment possibility. mannequin.create()
creates a mannequin entity, which can be included within the customized metadata registered for this mannequin model and later used within the second pipeline for batch inference and mannequin monitoring. See the next code:
The register mannequin step registers the mannequin model and related metadata to the SageMaker mannequin registry. This contains mannequin efficiency metrics in addition to metadata for the info drift baseline, together with the Amazon S3 places of the statistics and constraints recordsdata produced by the baselining step. You’ll additionally discover the extra customized metadata famous customer_metadata_properties
pulling the mannequin entity info that can be used later within the inference pipeline. The flexibility to offer customized metadata inside the mannequin registry is a good way to include further metadata that needs to be collected that isn’t explicitly outlined in native SageMaker parameters. See the next code:
Configure a conditional step to judge mannequin efficiency
The conditional step, ConditionStep, compares mannequin accuracy in opposition to an recognized threshold and checks the standard of the skilled mannequin.
It reads the analysis.json
file and checks if the mannequin accuracy, or no matter goal metric you might be optimizing for, meets the standards you’ve outlined. On this case, the standards is outlined utilizing one of many built-in conditions, ConditionGreaterThanOrEqualTo
. If the situation is happy, the pipeline continues to baseline the info and carry out subsequent steps within the pipeline. The pipeline stops if the situation is just not met. As a result of the situation explicitly calls out the subsequent steps within the pipeline, we’ve to make sure these steps are configured previous to configuring our conditional step. See the next code:
Outline, create, and begin the SageMaker pipeline
At this level, all of the steps of the prepare and baseline pipeline are outlined and configured. Now it’s time to outline, create, and begin the pipeline.
First, we outline the pipeline, Pipeline(), offering a pipeline title and a listing of steps beforehand configured to incorporate within the pipeline. Subsequent, we create the pipeline utilizing training_pipeline.upsert()
. Lastly, we begin the pipeline utilizing training_pipeline.begin()
. See the next code:
When the pipeline begins operating, you’ll be able to visualize its standing on Studio. The next diagram exhibits which steps from the pipeline course of relate to the steps of the pipeline directed acyclic graph (DAG). After the prepare and baseline pipeline run efficiently, it registers the skilled mannequin as a part of the mannequin group within the mannequin registry. The pipeline is at the moment set as much as register the mannequin in a Pending state, which requires a guide approval. Optionally, you’ll be able to configure the mannequin registration step to mechanically approve the mannequin within the mannequin registry. The second pipeline will pull the most recent permitted mannequin from the registry for inference.
In Studio, you’ll be able to select any step to see its key metadata. For instance, the info high quality verify step (baseline step) inside the pipeline DAG exhibits the S3 output places of statistics.json
and constraints.json
within the Studies part. These are key recordsdata calculated from uncooked information used as a baseline.
After the pipeline has run, the baseline (statistics and constraints) for information high quality monitoring will be inspected, as proven within the following screenshots.
Pipeline 2: Batch inference and Mannequin Monitor pipeline
On this part, we dive into the second pipeline used for monitoring the brand new batch enter information for alerts of knowledge drift and operating batch inference utilizing SageMaker Pipelines. The required steps and code are inside 2.SageMakerPipeline-ModelMonitoring-DataQuality-BatchTransform.ipynb. This pipeline contains the next steps:
- A Lambda step to retrieve the most recent permitted mannequin model and related metadata from the mannequin registry.
- A Mannequin Monitor step to detect alerts of knowledge drift utilizing the brand new enter information and the baseline from Pipeline 1.
- A batch remodel step to course of the batch enter information in opposition to the most recent permitted mannequin.
Configure a Lambda Step
Earlier than we begin the mannequin monitoring and batch remodel job, we have to question the mannequin registry to get the most recent permitted mannequin that we’ll use for batch inference.
To do that, we use a Lambda step, which permits us to incorporate customized logic inside our pipeline. The lambda_getapproved_model.py
Lambda perform queries the SageMaker mannequin registry for a selected mannequin bundle group offered on enter to establish the most recent permitted mannequin model and return associated metadata. The output contains metadata created from our first pipeline:
- Mannequin bundle ARN
- Packaged mannequin title
- S3 URI for statistics baseline
- S3 URI for constraints baseline
The output is then used as enter within the subsequent step within the pipeline, which performs batch monitoring and scoring utilizing the most recent permitted mannequin.
To create and run the Lambda perform as a part of the SageMaker pipeline, we have to add the perform as a LambdaStep within the pipeline:
Configure the info monitor and batch remodel steps
After we create the Lambda step to get the most recent permitted mannequin, we are able to create the MonitorBatchTransformStep. This native step orchestrates and manages two baby duties which might be run in succession. The primary job contains the Mannequin Monitor job that runs a Processing job utilizing a built-in container picture used to watch the batch enter information and examine it in opposition to the constraints from the beforehand generated baseline from Pipeline 1. As well as, this step kicks off the batch remodel job, which processes the enter information in opposition to the most recent permitted mannequin within the mannequin registry.
This batch deployment and information high quality monitoring step takes the S3 URI of the batch prediction enter information on enter. That is parameterized to permit for every run of the pipeline to incorporate a brand new enter dataset. See the next code:
Subsequent, we have to configure the transformer for the batch remodel job that may course of the batch prediction requests. Within the following code, we go within the mannequin title that was pulled from the customized metadata of the mannequin registry, together with different required parameters:
The information high quality monitor accepts the S3 URI of the baseline statistics and constraints for the most recent permitted mannequin model from the mannequin registry to run the info high quality monitoring job through the pipeline run. This job compares the batch prediction enter information with the baseline information to establish any violations signaling potential information drift. See the next code:
Subsequent, we use MonitorBatchTransformStep to run and monitor the remodel job. This step runs a batch remodel job utilizing the transformer object we configured and displays the info handed to the transformer earlier than operating the job.
Optionally, you’ll be able to configure the step to fail if a violation to information high quality is discovered by setting the fail_on_violation
flag to False
.
See the next code:
Outline, create, and begin the pipeline
After we outline the LambdaStep
and MonitorBatchTransformStep
, we are able to create the SageMaker pipeline.
See the next code:
We will now use the upsert()
technique, which can create or replace the SageMaker pipeline with the configuration we specified:
Though there are a number of methods to start out a SageMaker pipeline, when the pipeline has been created, we are able to run the pipeline utilizing the begin()
technique.
Word that to ensure that the LambdaStep
to efficiently retrieve an permitted mannequin, the mannequin that was registered as a part of Pipeline 1 must have an Accepted standing. This may be executed in Studio or utilizing Boto3. Consult with Update the Approval Status of a Model for extra info.
To run the SageMaker pipeline on a schedule or based mostly on an occasion, check with Schedule a Pipeline with Amazon EventBridge.
Assessment the Mannequin Monitor reviews
Mannequin Monitor makes use of a SageMaker Processing job that runs the DataQuality
verify utilizing the baseline statistics and constraints. The DataQuality
Processing job emits a violations report back to Amazon S3 and likewise emits log information to Amazon CloudWatch Logs beneath the log group for the corresponding Processing job. Pattern code for querying Amazon CloudWatch logs is offered within the pocket book.
We’ve now walked you thru the way to create the primary pipeline for mannequin coaching and baselining, in addition to the second pipeline for performing batch inference and mannequin monitoring. This lets you automate each pipelines whereas incorporating the completely different lifecycles between coaching and inference.
To additional mature this reference sample, you’ll be able to establish a method for suggestions loops, offering consciousness and visibility of potential alerts of drift throughout key stakeholders. At a minimal, it’s really useful to automate exception dealing with by filtering logs and creating alarms. These alarms may have further evaluation by an information scientist, or you’ll be able to implement further automation supporting an automated retraining technique utilizing new floor reality information by integrating the mannequin coaching and baselining pipeline with Amazon EventBridge. For extra info, check with Amazon EventBridge Integration.
Clear up
After you run the baseline and batch monitoring pipelines, ensure to wash up any assets that gained’t be utilized, both programmatically by way of the SageMaker console, or by Studio. As well as, delete the info in Amazon S3, and ensure to cease any Studio pocket book situations to not incur any additional prices.
Conclusion
On this publish, you discovered the way to create an answer for a batch mannequin that’s skilled much less steadily than batch inference is carried out in opposition to that skilled mannequin utilizing SageMaker MLOps options, together with Pipelines, the mannequin registry, and Mannequin Monitor. To broaden this resolution, you might incorporate this right into a customized SageMaker mission that additionally incorporates CI/CD and automatic triggers utilizing standardized MLOps templates. To dive deeper into the answer and code proven on this demo, try the GitHub repo. Additionally, check with Amazon SageMaker for MLOps for examples associated to implementing MLOps practices with SageMaker.
In regards to the Authors
Shelbee Eigenbrode is a Principal AI and Machine Studying Specialist Options Architect at Amazon Internet Companies (AWS). She has been in expertise for twenty-four years spanning a number of industries, applied sciences, and roles. She is at the moment specializing in combining her DevOps and ML background into the area of MLOps to assist prospects ship and handle ML workloads at scale. With over 35 patents granted throughout numerous expertise domains, she has a ardour for steady innovation and utilizing information to drive enterprise outcomes. Shelbee is a co-creator and teacher of the Sensible Information Science specialization on Coursera. She can be the Co-Director of Girls In Large Information (WiBD), Denver chapter. In her spare time, she likes to spend time together with her household, buddies, and overactive canines.
Sovik Kumar Nath is an AI/ML resolution architect with AWS. He has expertise in designs and options for machine studying, enterprise analytics inside monetary, operational, and advertising and marketing analytics; healthcare; provide chain; and IoT. Outdoors work, Sovik enjoys touring and watching films.
Marc Karp is a ML Architect with the Amazon SageMaker Service group. He focuses on serving to prospects design, deploy, and handle ML workloads at scale. In his spare time, he enjoys touring and exploring new locations.