NumPy and OpenCV Tutorial for Pc Imaginative and prescient
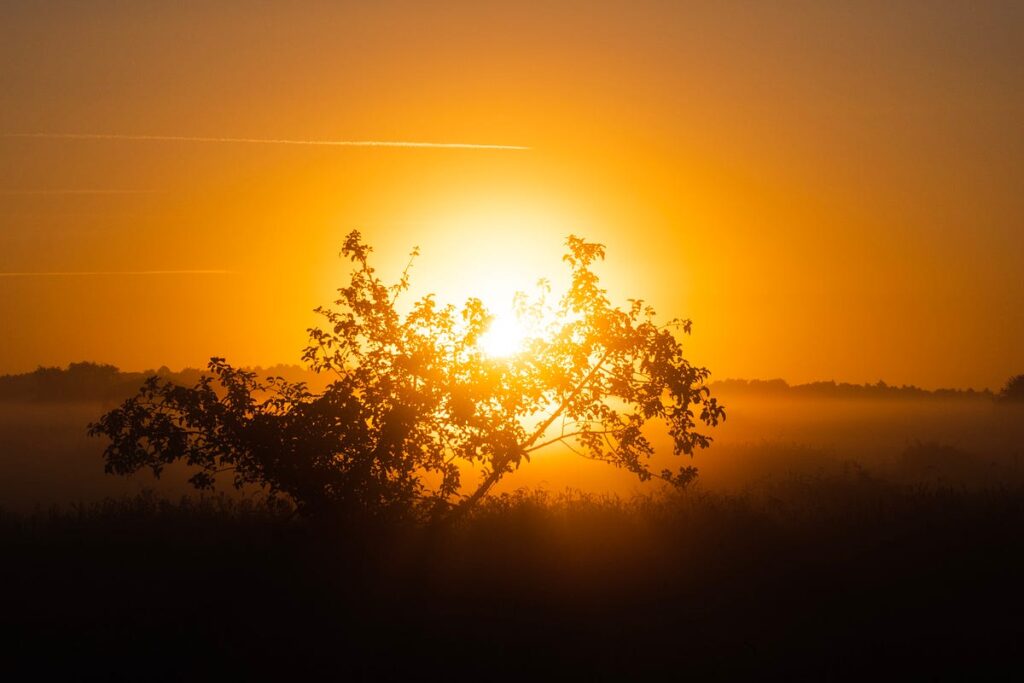
Begin Your Coding for Pc imaginative and prescient with Python
Motivation
We, human beings, understand the setting and environment with our imaginative and prescient system. The human eye, mind, and limbs work collectively to understand the setting and act accordingly. An clever system can carry out these duties which require some stage of intelligence if completed by a human. So, for performing clever duties, synthetic imaginative and prescient system is without doubt one of the essential issues for a pc. Usually, the digicam and picture are used to assemble info wanted to do the job. Pc imaginative and prescient and Picture processing strategies assist us to carry out related duties completed by people, like picture recognition, object monitoring, and many others.
In pc imaginative and prescient, the digicam works as a human eye to seize the picture, and the processor works as a mind to course of the captured picture and generate vital outcomes. However there’s a primary distinction between people and computer systems. The human mind works routinely, and intelligence is a by-born acquisition. Quite the opposite, the pc has no intelligence with out human instruction (program). Pc imaginative and prescient is the best way to offer the suitable instruction in order that it may work suitable with the human imaginative and prescient system. However the capability is proscribed.
Within the upcoming sections, we are going to talk about the essential thought of how the picture is shaped and will be manipulated utilizing python.
How Picture is Shaped and Displayed
The picture is nothing however a mixture of pixels with completely different shade intensities. The jargon ‘pixels’ and ‘shade depth’ could also be unknown to you. Don’t fear. It is going to be crystal clear, simply learn the article until the tip.
Pixel is the smallest unit/aspect of the digital picture. Particulars are within the picture under.
The show is shaped with pixels. Within the above determine, there are 25 columns and 25 rows. Every small sq. is taken into account a pixel. The setup can home 625 pixels. It represents a show with 625 pixels. If we shine the pixels with completely different shade depth (brightness), it is going to type a digital picture.
How does the pc retailer the picture within the reminiscence?
If we take a look at the picture rigorously, we are able to evaluate it with a 2D matrix. A matrix has rows and columns, and its components will be addressed with its index. The matrix construction is just like an array. And pc retailer the picture in an array of pc reminiscence.
Every array aspect holds the depth worth of a shade. Typically, the depth worth ranges from 0 to 255
. For demonstration functions, I’ve included an array illustration of a picture.
Grayscale and Coloured Picture
The grayscale picture is a black-and-white picture. It’s shaped with just one shade. A pixel worth near 0 represents darkness and turns into brighter with increased depth values. The best worth is 255, which represents the white shade. A 2D array is ample to carry the grayscale picture, because the final determine exhibits.
Coloured pictures can’t be shaped with just one shade; there is likely to be tons of of 1000’s of shade combos. Primarily, there are three major shade channels RED (R), GREEN(G), and Blue(B)
. And every shade channel is saved in a 2D array and holds its depth values, and the ultimate picture is the mix of those three shade channels.
This shade mannequin has (256 x 256 x 256) = 16,777,216 potential shade combos. You may visualize the combination here.
However in pc reminiscence, the picture is saved otherwise.
The pc doesn’t know the RGB channels. It is aware of the depth worth. The crimson channel is saved with excessive depth, and the inexperienced and blue channels are saved with medium and low-intensity values, respectively.
NumPy Fundamentals to Work with Python
NumPy is a elementary python bundle for scientific computation. It really works primarily as an array object, however its operation isn’t restricted to the array. Nonetheless, the library can deal with numerous numeric and logical operations on numbers [1]. You'll get NumPy official documentation here.
Let’s begin our journey. Very first thing first.
- Importing the NumPy library.
It’s time to work with NumPy. As we all know, NumPy works with an array. So, let’s attempt to create our first 2D array of all zeros.
It’s so simple as that. We will additionally create a NumPy array with all ones simply as follows.
Curiously, NumPy additionally offers a technique to fill the array with any values. The straightforward syntax array.fill(worth)
can do the job.
The array ‘b’
with all ones is now stuffed with 3
.
- The Operate of Seed in case of Random Quantity Technology
Simply take a look on the following coding examples.
Within the first code cell, we have now used np.random.seed(seed_value)
, however we haven’t used any seeding for the opposite two code cells. There’s a main distinction between random quantity era with and with out seeding. Within the case of random seeding, the generated random quantity stays the identical for a particular seed worth. Then again, with no seed worth, random quantity modifications for every execution.
- Fundamental operations (max, min, imply, reshape, and many others.) with NumPy
NumPy has made our life simpler by offering quite a few features to do mathematical operations. array_name.min(), array_name.max(), array_name.imply()
syntaxes assist us discover an array’s minimal, most, and imply values. Coding instance —
Indeies of the minimal and most values will be extracted with the syntaxes array_name.argmax(), array_name.argmin()
. Instance —
Array reshaping is without doubt one of the essential operations of NumPy. array_name.reshape(row_no, column_no)
is the syntax for reshaping an array. Whereas reshaping the array, we have to be cautious concerning the variety of array components earlier than and after reshaping. In each instances, the whole variety of components have to be the identical.
- Array Indexing and Slicing
Every array aspect will be addressed with its column and row
quantity. Let’s generate one other array with 10 rows and columns.
Suppose we wish to discover the worth of the primary worth of the array. It may be extracted by passing the row and column index (0 , 0).
Particular row and column values will be sliced with the syntax array_name[row_no,:], array_name[:,column_no].
Let’s attempt to slice the central components of the array.
OpenCV Fundamentals
OpenCV is an open-source python library for Pc Imaginative and prescient developed by Intel [2]. I’ll talk about just a few usages of OpvenCv although its scope is huge. You will see the official documentation here.
I've used the next picture for demonstration functions.
- Importing OpenCV and Matplotlib library
Matplotlib is a visualization library. It helps to visualise the picture.
- Loading the picture with OpenCV and visualize with matplotlib
We now have learn the picture with OpenCV and visualized it with the matplotlib library. The colour has been modified as a result of OpenCV reads the picture in BGR format as an alternative of RGB, however matplotlib expects the picture in RGB format. So, we have to convert the picture from BGR to RGB.
- Changing the picture from BGR to RGB format
Now, the picture appears okay.
- Changing picture to grayscale
We will simply convert the picture from BGR to grayscale with cv2.COLOR_BGR2GRAY
is as follows.
The above picture just isn’t correctly grey although it has been transformed to grayscale. It has been visualized with matplotlib. By default, matplotlib makes use of shade mapping apart from grayscale. To correctly visualize it, we have to specify the grayscale shade mapping in matplotlib. Let’s do this.
Rotating can be a simple job with OpenCV
. cv2.rotate()
operate helps us to do this. Clockwise and anticlockwise 90-degree and 180-degree
rotation have proven under.
We will resize the picture by passing the width and peak pixel values to the cv2.resize()
operate.
Typically we have to draw on an present picture. For instance, we have to draw a bounding field on a picture object to establish it. Let’s draw a rectangle on the flower. cv2.rectangle()
operate helps to attract on it. It takes some parameters just like the picture on which we draw the rectangle, the coordinate level of the higher left nook (pt1)
and the decrease proper nook (pt2)
, and the thickness of the boundary line. A coding instance is given under.
There are different drawing features cv.line(), cv.circle() , cv.ellipse(), cv.putText(), and many others
. The complete official documentation is offered here
[3].
Play with NumPy
We are going to change the depth worth of a picture. I’ll attempt to hold it easy. So, take into account the grayscale picture proven beforehand. Discover the form of the picture.
It exhibits it’s a 2D array with a measurement of 1200 x 1920
. Within the primary NumPy operation, we realized how you can slice an array.
Utilizing the idea, we have now taken the grayscale picture array slice [400:800, 750:1350]
and changed the depth values with 255
. Lastly, we visualize it and discover the above picture.
Conclusion
Pc imaginative and prescient is without doubt one of the promising fields in trendy pc science expertise. I at all times emphasize the essential information of any area. I’ve mentioned simply the first information of pc imaginative and prescient and proven some hands-on coding. The ideas are quite simple however could play a major function for the newbie of pc imaginative and prescient.
That is the primary article of the pc imaginative and prescient sequence. Get related to learn the upcoming articles.
[N.B. Instructor Jose Portilla’s course helps me to gather knowledge.]