A Full Information to Matrices for Machine Studying with Python

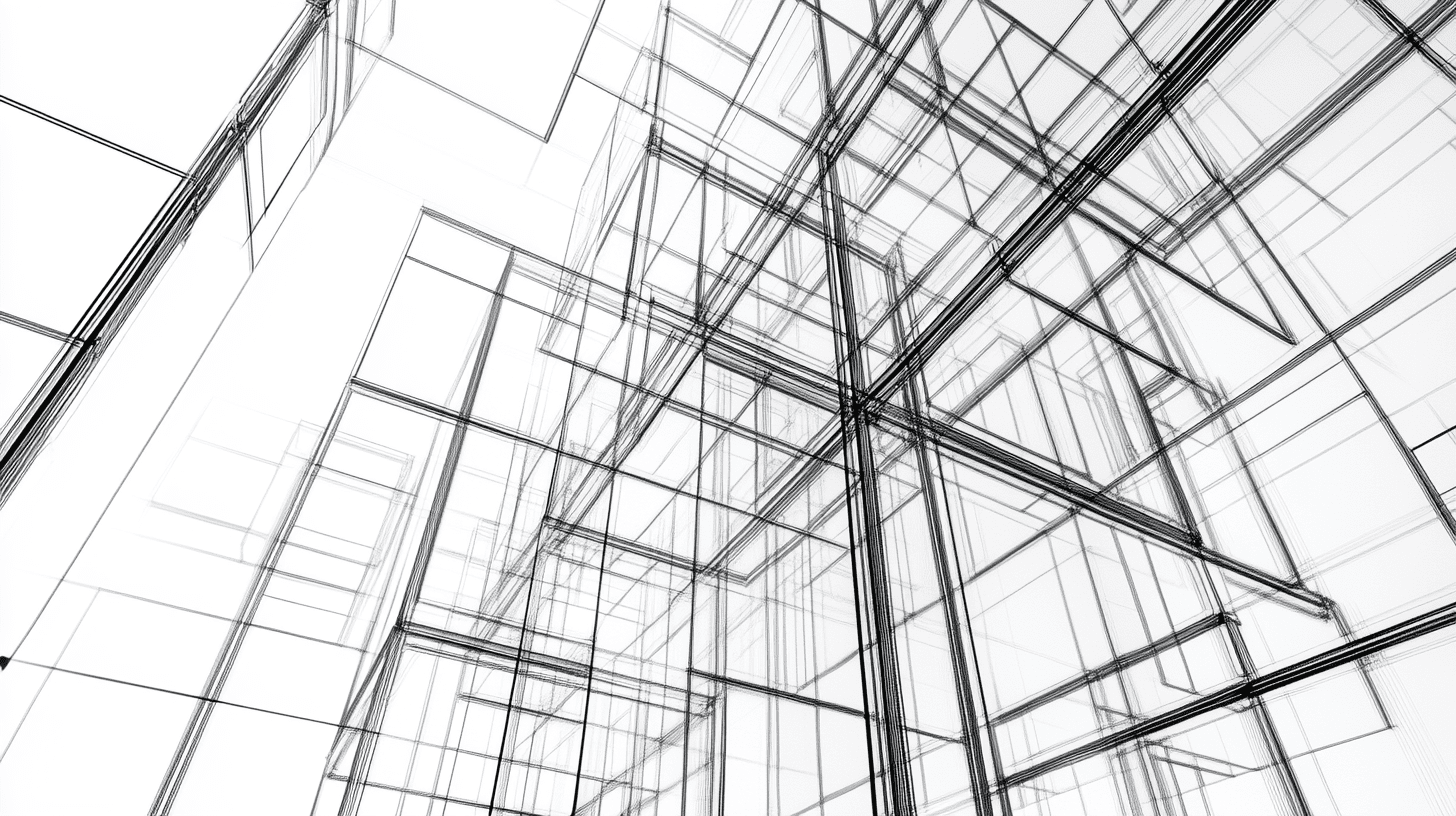
A Full Information to Matrices for Machine Studying with Python
Picture by Writer | Ideogram
Introduction
Matrices are a key idea not solely in linear algebra but additionally with regard to their distinguished utility and use in machine studying (ML) and information science. In essence, a matrix is a structured method to characterize and manipulate information, however it additionally represents a cornerstone ingredient in ML algorithms to coach fashions like linear regression to neural community fashions.
This information introduces the way to outline and use matrices in Python, their operations, and a top level view of their makes use of in ML processes.
What’s a Matrix?
A matrix is a two-dimensional array (assortment, in day by day phrases) of numbers organized in a row-column style. Given this structural similarity to tabular datasets consisting of situations and options, they’re extremely helpful for representing datasets and performing transformations on information, in addition to endeavor mathematical operations effectively.
Python offers two fundamental approaches to implement matrices: both utilizing nested lists or resorting to the NumPy
library, the latter of which helps optimized matrix operations.
Right here’s an instance 3×3 matrix outlined utilizing an inventory of lists, with every nested listing representing a row of the matrix:
matrix = [[1][2],, ] print(matrix) |
A matrix like this might nicely characterize a tiny dataset of three situations, described by three options every.
Right here’s the choice technique to outline a matrix utilizing NumPy:
import numpy as np
matrix_np = np.array([[1][2],, ]) print(matrix_np) |
Primary Operations with Matrices
In addition to defining matrices to comprise information, matrices operations are important in ML to conduct transformations, optimizations, and fixing linear equations. The underlying processes behind coaching a neural community, as an example, depend on these sorts of matrix operations mixed with differential calculus at a big scale.
Let’s define the fundamental matrix operations, beginning with addition and subtraction:
A = np.array([[1][2],]) B = np.array([,])
sum_matrix = A + B sub_matrix = A – B
print(“Sum:n”, sum_matrix) print(“Distinction:n”, sub_matrix) |
Matrix multiplication is especially frequent in ML processes, each to use information transformations like scaling attributes and in superior neural community architectures like convolutional neural networks (CNN), the place matrix multiplications are utilized to carry out filters on picture information, thereby serving to acknowledge patterns like edges, colours, and so forth., in visible information. Matrix multiplication in Python is carried out as follows:
C = np.dot(A, B) print(“Matrix Multiplication:n”, C) |
However there’s a less complicated means, utilizing the ‘@’ operator:
C = A @ B print(“Matrix Multiplication made easy:n”, C) |
Transposing a matrix is one other frequent operation in ML to effectively reshape information or to compute covariance matrices in PCA, a widely known technique for lowering the dimensionality of information.
transpose_A = A.T print(“Transpose of A:n”, transpose_A) |
Particular Matrices and Their Position in Machine Studying with Python
Time to establish some significantly necessary matrices on account of their particular properties, whose use is especially widespread in ML approaches.
First, now we have the id matrix, which is utilized in a number of ML algorithms for information normalization and transformations. This matrix accommodates zeros in all its parts apart from the primary diagonal, made up of ones.
identity_matrix = np.eye(3) print(“Identification Matrix:n”, identity_matrix) |
As necessary because the establish matrix, if no more, is the inverse of a matrix, key in optimization processes that information the coaching strategy of most ML fashions. The inverse of a matrix will be simply calculated in Python by:
A_inv = np.linalg.inv(A) print(“Inverse of A:n”, A_inv) |
Subsequent, the determinant of a matrix is a worth related to a matrix used to examine if a matrix is singular, wherein case it has no inverse. The determinant have to be nonzero for the matrix to be invertible.
det_A = np.linalg.det(A) print(“Determinant of A:”, det_A) |
Instance: Matrices in Linear Regression
To finalize this introductory information, let’s see the way to apply a few of the concepts realized in an ML workflow, significantly in linear regression. This quite simple instance solves a linear regression downside utilizing the regular equation, which blends a number of of the matrix operations seen beforehand, specifically matrix merchandise and inverses: W = (X^T X)^{-1} X^T Y
.
X = np.array([[1][1],[1][2],[1]]) Y = np.array([[2], [2.5], [3.5]]) W = np.linalg.inv(X.T @ X) @ X.T @ Y
print(“Computed Weights:n”, W) |
Abstract and Conclusion
Matrices have varied makes use of in machine studying, together with information illustration, becoming fashions like linear regression, coaching neural networks by storing and making use of operations in connection weights between neurons and in dimensionality discount strategies like Principal Part Evaluation (PCA).
Since matrices are a central part of machine studying, they’re nicely supported by Python libraries like NumPy. This text offered an preliminary basis and understanding of matrices for machine studying in Python. Understanding these fundamentals is vital to mastering extra superior machine studying approaches and strategies.