Chicken by Chicken Utilizing Finite Automata | by Sofya Lipnitskaya | Might, 2024
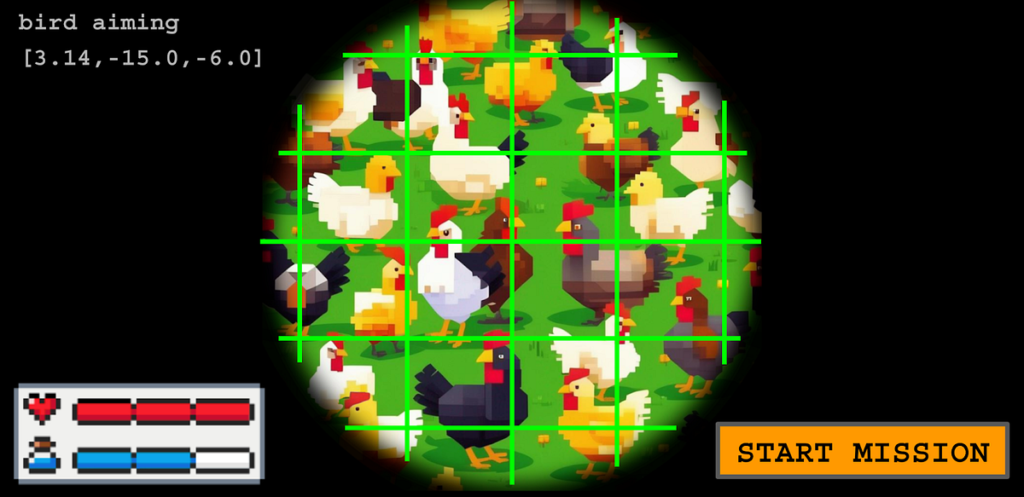
Finite-state machine modelling and simulation for real-world AI methods on object detection utilizing Python
“When life offers you chickens, let AI deal with the fowl play.” — Unknown Engineer.
Why on earth do we’d like simulations? What’s the benefit we will get by sampling one thing and getting a median? However that’s by no means solely this. Actual life is often way more complicated in comparison with simplistic duties we encounter in laptop science courses. Typically we will’t discover an analytical answer, we will’t discover inhabitants parameters. Typically we have now to construct a mannequin to replicate specifics of the system’s dynamics, we have now to run simulations to check the underlying processes in order to achieve a greater understanding of real-world conditions. Simulation modelling gives a useful software for methods design and engineering throughout a variety of industries and functions. It helps to analyse system efficiency, determine potential bottlenecks and inefficiencies, thus permitting for iterative refinements and enhancements.
Talking about our very particular problem, right here, we’re going to create an FSM simulation replicating the conduct of an AI-assisted safety system for garden monitoring and cleansing. Specifically, we’ll deal with the duty of simulating processes to intelligently handle the approaching and going of birds by way of object detection and water sprinkling subsystems. Within the previous article, you had been launched to the speculation and design rules on finite state machines (FSM) for coping with the notorious Hen-and-Turkey (CaT) drawback, ensuing within the creation of a mannequin that describes complicated garden eventualities at a excessive stage of abstraction. Via this text, we’ll additional examine the subject of sensible features of an FSM-based simulation for leveraging the real-life system operation. As well as, we’re going to implement the FSM simulation in Python in order that we will later enhance it through optimization and XAI methods. By the top of the tutorial, you’ll have a completely purposeful FSM answer together with a greater understanding of simulation modelling for fixing engineering issues.
Disclaimer: This work is part of the “Bird by Bird using Deep Learning” collection and is dedicated to modelling and simulation of real-life methods for laptop imaginative and prescient functions utilizing finite automata. All actors, states, occasions and outputs are the merchandise of the FSM design course of for academic functions solely. Any resemblance to precise individuals, birds, or actual occasions is solely coincidental.
“When requested about methods design sans abstractions, simply describe if-then loops for real-life eventualities, ensuring to stutter whereas juggling a number of situations. Then, gracefully retreat, leaving these minutiae behind.” — Unknown Engineer.
Bringing the speculation alive
Simulation, a particular case of mathematical modelling, includes creating simplified representations of real-world methods to grasp their conduct below varied situations. At its core, a mannequin is to seize intrinsic patterns of a real-life system by way of equations, whereas simulation pertains to the algorithmic approximation of those equations by working a program. This course of allows technology of simulation outcomes, facilitating comparability with theoretical assumptions and driving enhancements within the precise system. Simulation modelling permits to supply insights on the system conduct and predict outcomes when it’s too costly and/or difficult to run actual experiments. It may be particularly helpful when an analytical answer is just not possible (e.g., warehouse administration processes).
When coping with the CaT-problem, the target is evident: we need to keep a pristine garden and save assets. Moderately than counting on conventional experimentation, we go for a simulation-based strategy to discover a setup that enables us to reduce water utilization and payments. To attain this, we’ll develop an FSM-based mannequin that displays the important thing system processes, together with chicken intrusion, chicken detection, and water sprinkling. All through the simulation, we’ll then assess the system efficiency to information additional optimization efforts in direction of improved effectivity on chicken detection.
Why not if-else directions
Utilizing if-else conditional branching for system modelling is a naïve answer that can in the end result in elevated complexity and error-proneness by design, making additional improvement and upkeep harder. Beneath you discover the right way to (not) describe a easy chicken-on-the-lawn system, contemplating an instance of the easy FSM we mentioned earlier (see Figure 1 for FSM state transition diagram with simplified CaT- system eventualities).
# import features with enter occasions and actions
from occasions import (
simulate_chicken_intrusion,
initiate_shooing_chicken,
)
from actions import (
spoil_the_lawn,
start_lawn_cleaning,
one_more_juice
)# outline states
START = 0
CHICKEN_PRESENT = 1
NO_CHICKEN = 2
LAWN_SPOILING = 3
ENGINER_REST = 4
END = 5
# initialise simulation step and length
sim_step = 0
max_sim_steps = 8
# initialise states
prev_state = None
current_state = START
# monitor for occasions
whereas current_state != END:
# replace state transitions
if current_state == START:
current_state = NO_CHICKEN
prev_state = START
elif current_state == NO_CHICKEN:
if prev_state == CHICKEN_PRESENT:
start_lawn_cleaning()
if simulate_chicken_intrusion():
current_state = CHICKEN_PRESENT
else:
current_state = ENGINER_REST
prev_state = NO_CHICKEN
elif current_state == CHICKEN_PRESENT:
if initiate_shooing_chicken():
current_state = NO_CHICKEN
else:
current_state = LAWN_SPOILING
prev_state = CHICKEN_PRESENT
elif current_state == LAWN_SPOILING:
spoil_the_lawn()
current_state = CHICKEN_PRESENT
prev_state = LAWN_SPOILING
elif current_state == ENGINER_REST:
one_more_juice()
current_state = NO_CHICKEN
prev_state = ENGINER_REST
sim_step += 1
if sim_step >= max_sim_steps:
current_state = END
On this code snippet, we outline constants to characterize every state of the FSM (e.g., CHICKEN_PRESENT). Then, we initialize the present state to START and constantly monitor for occasions inside some time loop, simulating the conduct of the simplified system. Primarily based on the present state and related occasions, we use if-else conditional branching directions to change between states and invoke corresponding actions. A state transition can have unwanted side effects, reminiscent of initiating the method of the garden spoiling for chickens and beginning the garden cleansing for the engineer. Right here, performance associated to enter occasions and actions signifies processes that may be automated, so we mock importing the related features for simplicity. Notice, that while chickens can spoil a garden practically endlessly, extreme portions of juice are fraught with the chance of hyperhydration. Watch out with this and don’t overlook so as to add constraints on the length of your simulation. In our case, this would be the finish of the day, as outlined by the `max_sim_steps` variable. Seems ugly, proper?
This could work, however think about how a lot time it might take to replace if-else directions if we wished to increase the logic, repeating the identical branching and switching between states time and again. As you’ll be able to think about, because the variety of states and occasions will increase, the scale of the system state house grows quickly. In contrast to if-else branching, FSMs are actually good at dealing with complicated duties, permitting complicated methods to be decomposed into manageable states and transitions, therefore enhancing code modularity and scalability. Right here, we’re about to embark on a journey in implementing the system conduct utilizing finite automata to scale back water utilization for AI-system operation with out compromising accuracy on chicken detection.
“Okay, kiddo, we’re about to create a rooster now.” — Unknown Engineer.
FSM all the best way down
On this part, we delve into the design selections underlying FSM implementation, elucidating methods to streamline the simulation course of and maximize its utility in real-world system optimization. To construct the simulation, we first must create a mannequin representing the system based mostly on our assumptions concerning the underlying processes. A technique to do that is to begin with encapsulating functionally for particular person states and transitions. Then we will mix them to create a sequence of occasions by replicating an actual system conduct. We additionally need to monitor output statistics for every simulation run to supply an thought of its efficiency. What we need to do is watch how the system evolves over time given variation in situations (e.g., stochastic processes of birds spawning and spoiling the garden given a chance). For this, let’s begin with defining and arranging constructing blocks we’re going to implement in a while. Right here is the plan:
- Outline class contracts.
- Construct class hierarchy for targets, describe particular person targets.
- Implement transition logic between states.
- Implement a single simulation step together with the complete run.
- Monitor output statistics of the simulation run.
The supply code used for this tutorial will be discovered on this GitHub repository: https://github.com/slipnitskaya/Bird-by-Bird-AI-Tutorials.
Let’s speak summary
First, we have to create a category hierarchy for our simulation, spanning from base courses for states to a extra area particular yard simulation subclass. We are going to use `@abc.abstractmethod` and `@property` decorators to mark summary strategies and properties, respectively. Within the AbstractSimulation class, we’ll outline `step()` and `run()` summary strategies to be sure that youngster courses implement them.
class AbstractSimulation(abc.ABC):
@abc.abstractmethod
def step(self) -> Tuple[int, List['AbstractState']]:
move@abc.abstractmethod
def run(self) -> Iterator[Tuple[int, List['AbstractState']]]:
move
Related applies to AbstractState, which defines an summary methodology `transit()` to be carried out by subclasses:
class AbstractState(abc.ABC):
def __init__(self, state_machine: AbstractSimulation):
tremendous().__init__()
self.state_machine = state_machinedef __eq__(self, different):
return self.__class__ is different.__class__
@abc.abstractmethod
def transit(self) -> 'AbstractState':
move
For our FSM, extra particular features of the system simulation will likely be encapsulated within the AbstractYardSimulation class, which inherits from AbstractSimulation. As you’ll be able to see in its identify, AbstractYardSimulation outlines the area of simulation extra exactly, so we will outline some additional strategies and properties which can be particular to the yard simulation within the context of the CaT drawback, together with `simulate_intrusion()`, `simulate_detection()`, `simulate_sprinkling()`, `simulate_spoiling()`.
We may also create an intermediate summary class named AbstractYardState to implement typing consistency within the hierarchy of courses:
class AbstractYardState(AbstractState, abc.ABC):
state_machine: AbstractYardSimulation
Now, let’s check out the inheritance tree reflecting an entity named Goal and its descendants.
Hen and Turkey creation
Goal conduct is a cornerstone of our simulation, because it impacts all of the features in direction of constructing an efficient mannequin together with its optimization downstream. Determine 1 reveals a category diagram for the goal courses we’re going to implement.
For our system, it’s essential to notice {that a} goal seems with a sure frequency, it could trigger some injury to the garden, and it additionally has a well being property. The latter is said to the scale of the goal, which can differ, thus a water gun can goal for both smaller or bigger targets (which, in flip, impacts the water consumption). Consequently, a big goal has loads of well being factors, so a small water stream will be unable to successfully handle it.
To mannequin targets trespassing the garden with totally different frequencies we additionally create the related property. Right here we go:
class AbstractTarget(int, abc.ABC):
@property
@abc.abstractmethod
def well being(self) -> float:
move@property
@abc.abstractmethod
def injury(self) -> float:
move
@property
@abc.abstractmethod
def frequency(self) -> float:
move
Notice that in our implementation we would like the goal objects to be legitimate integers, which will likely be of use for modelling randomness within the simulation.
Subsequent, we create youngster courses to implement totally different sorts of targets. Beneath is the code of the category Hen, the place we override summary strategies inherited from the guardian:
class Hen(AbstractTarget):
@property
def well being(self) -> float:
return 4@property
def injury(self) -> float:
return 10
@property
def frequency(self) -> float:
return 9
We repeat the same process for remaining Turkey and Empty courses. Within the case of Turkey, well being and injury parameters will likely be set to 7 and 17, respectively (let’s see how we will deal with these cumbersome ones with our AI-assisted system). Empty is a particular kind of Goal that refers back to the absence of both chicken species on the garden. Though we will’t assign to its well being and injury properties different values than 0, an unconditional (i.e. not brought on by the engineer) birdlessness on the garden has a non-zero chance mirrored by the frequency worth of 9.
From Intrusion to Enemy Noticed with ease
Now think about a chicken in its pure habitat. It may exhibit all kinds of agonistic behaviors and shows. Within the face of problem, animals could make use of a set of adaptive methods relying on the circumstances, together with battle, or flight responses and different intermediate actions. Following up on the earlier article on the FSM design and modelling, you could do not forget that we already described the important thing elements of the CaT system, which we’ll use for the precise implementation (see Table 2 for FSM inputs describing the occasions triggering state adjustments).
Within the realm of the FSM simulation, a chicken will be considered as an unbiased actor triggering a set of occasions: trespassing the yard, spoiling the grass, and so forth. Specifically, we anticipate the next sequential patterns in case of an optimistic state of affairs (success in chicken detection and identification, protection actions): a chicken invades the yard earlier than presumably being acknowledged by the CV-based chicken detector with a view to transfer forward with water sprinkling module, these configuration relies on the invader class predicted upstream. This manner, the chicken will be chased away efficiently (hit) or not (miss). For this state of affairs (success in chicken detection, class prediction, protection actions), the chicken, ultimately, escapes from the garden. Mission full. Tadaa!
You might do not forget that the FSM will be represented graphically as a state transition diagram, which we lined within the earlier tutorial (see Table 3 for FSM state transition desk with next-stage transition logic). Contemplating that, now we’ll create subclasses of AbstractYardState and override the `transit()` methodology to specify transitions between states based mostly on the present state and occasions.
Begin is the preliminary state from which the state machine transits to Spawn.
class Begin(AbstractYardState):
def transit(self) -> 'Spawn':
return Spawn(self.state_machine)
From Spawn, the system can transit to one of many following states: Intrusion, Empty, or Finish.
class Spawn(AbstractYardState):
def transit(self) -> Union['Intrusion', 'Empty', 'End']:
self.state_machine.stayed_steps += 1self.state_machine.simulate_intrusion()
next_state: Union['Intrusion', 'Empty', 'End']
if self.state_machine.max_steps_reached:
next_state = Finish(self.state_machine)
elif self.state_machine.bird_present:
next_state = Intrusion(self.state_machine)
else:
next_state = Empty(self.state_machine)
return next_state
Transition to the Finish state occurs if we attain the restrict on the variety of simulation time steps. The state machine switches to Intrusion if a chicken invades or is already current on the garden, whereas Empty is the subsequent state in any other case.
Each Intrusion and Empty states are adopted by a detection try, so that they share a transition logic. Thus, we will cut back code duplication by making a guardian class, specifically IntrusionStatus, to encapsulate this logic, whereas aiming the subclasses at making the precise states of the simulation Intrusion and Empty distinguishable on the kind stage.
class IntrusionStatus(AbstractYardState):
intruder_class: Goaldef transit(self) -> Union['Detected', 'NotDetected']:
self.state_machine.simulate_detection()
self.intruder_class = self.state_machine.intruder_class
next_state: Union['Detected', 'NotDetected']
if self.state_machine.predicted_bird:
next_state = Detected(self.state_machine)
else:
next_state = NotDetected(self.state_machine)
return next_state
We apply an identical strategy to the Detected and NotDetected courses, these superclass DetectionStatus handles goal prediction.
class DetectionStatus(AbstractYardState):
detected_class: Goaldef transit(self) -> 'DetectionStatus':
self.detected_class = self.state_machine.detected_class
return self
Nonetheless, in distinction to the Intrusion/Empty pair, the NotDetected class introduces an additional transition logic steering the simulation movement with respect to the garden contamination/spoiling.
class Detected(DetectionStatus):
def transit(self) -> 'Sprinkling':
tremendous().transit()return Sprinkling(self.state_machine)
class NotDetected(DetectionStatus):
def transit(self) -> Union['Attacking', 'NotAttacked']:
tremendous().transit()
next_state: Union['Attacking', 'NotAttacked']
if self.state_machine.bird_present:
next_state = Attacking(self.state_machine)
else:
next_state = NotAttacked(self.state_machine)
return next_state
The Detected class performs an unconditional transition to Sprinkling. For its antagonist, there are two attainable subsequent states, relying on whether or not a chicken is definitely on the garden. If the chicken is just not there, no poops are anticipated for apparent causes, whereas there could doubtlessly be some grass cleansing wanted in any other case (or not, the CaT universe is filled with randomness).
Getting again to Sprinkling, it has two attainable outcomes (Hit or Miss), relying on whether or not the system was profitable in chasing the chicken away (this time, not less than).
class Sprinkling(AbstractYardState):
def transit(self) -> Union['Hit', 'Miss']:
self.state_machine.simulate_sprinkling()next_state: Union['Hit', 'Miss']
if self.state_machine.hit_successfully:
next_state = Hit(self.state_machine)
else:
next_state = Miss(self.state_machine)
return next_state
Notice: The Hit state doesn’t deliver a devoted transition logic and is included to comply with semantics of the area of wing-aided shitting on the grass. Omitting it would trigger the Taking pictures state transition to Leaving straight.
class Hit(AbstractYardState):
def transit(self) -> 'Leaving':
return Leaving(self.state_machine)
If the water sprinkler was activated and there was no chicken on the garden (detector mis-predicted the chicken), the state machine will return to Spawn. In case the chicken was really current and we missed it, there’s a chance of chicken spoils on the grass.
class Miss(AbstractYardState):
def transit(self) -> Union['Attacking', 'Spawn']:
next_state: Union['Attacking', 'Spawn']
if self.state_machine.bird_present:
next_state = Attacking(self.state_machine)
else:
next_state = Spawn(self.state_machine)return next_state
Ultimately, the attacking try can lead to an actual injury to the grass, as mirrored by the Attacking class and its descendants:
class Attacking(AbstractYardState):
def transit(self) -> Union['Attacked', 'NotAttacked']:
self.state_machine.simulate_spoiling()next_state: Union['Attacked', 'NotAttacked']
if self.state_machine.spoiled:
next_state = Attacked(self.state_machine)
else:
next_state = NotAttacked(self.state_machine)
return next_state
class Attacked(AfterAttacking):
def transit(self) -> Union['Leaving', 'Spawn']:
return tremendous().transit()
class NotAttacked(AfterAttacking):
def transit(self) -> Union['Leaving', 'Spawn']:
return tremendous().transit()
We are able to make use of the identical thought as for the Intrusion standing and encapsulate the shared transition logic right into a superclass AfterAttacking, leading to both Leaving or returning to the Spawn state:
class AfterAttacking(AbstractYardState):
def transit(self) -> Union['Leaving', 'Spawn']:
next_state: Union['Leaving', 'Spawn']
if self.state_machine.max_stay_reached:
next_state = Leaving(self.state_machine)
else:
next_state = Spawn(self.state_machine)return next_state
What occurs subsequent? When the simulation reaches the restrict of steps, it stucks within the Finish state:
class Finish(AbstractYardState):
def transit(self) -> 'Finish':
return self
In observe, we don’t need this system to execute endlessly. So, subsequently, as soon as the simulation detects a transition into the Finish state, it shuts down.
“Within the refined world of chicken detection, bear in mind: whereas a mannequin says “no chickens detected,” a sneaky chicken might be on the garden unnoticed. This discrepancy stands as a name to refine and improve our AI methods.” — Unknown Engineer.
Now, we’d prefer to simulate a strategy of birds trespassing the garden, spoiling it and leaving. To take action, we’ll flip to a sort of simulation modelling known as discrete-event simulation. We are going to reproduce the system conduct by analyzing probably the most important relationships between its components and creating a simulation based mostly on finite automata mechanics. For this, we have now to contemplate the next features:
- Birds can trespass within the yard of the property.
- CV-based system makes an attempt to detect and classify the intruding object.
- Primarily based on the above, in case the thing was recognized as a specific chicken selection, we mannequin the water sprinkling course of to drive it away.
- It needs to be talked about that there’s additionally a probabilistic course of that leads to a chicken spoiling the garden (once more, nothing private, feathery).
Yard simulation processes
Now, it’s time to discover the magic of chance to simulate these processes utilizing the carried out FSM. For that, we have to create a YardSimulation class that encapsulates the simulation logic. As mentioned, the simulation is greater than an FSM. The identical applies to the correspondences between simulation steps and state machine transitions. That’s, the system must carry out a number of state transitions to change to the subsequent time step.
Right here, the `step()` methodology handles transitions from the present to the subsequent state and invokes the FSM’s methodology `transit()` till the state machine returns into the Spawn state or reaches Finish.
def step(self) -> Tuple[int, List[AbstractYardState]]:
self.step_idx += 1transitions = record()
whereas True:
next_state = self.current_state.transit()
transitions.append(next_state)
self.current_state = next_state
if self.current_state in (Spawn(self), Finish(self)):
break
return self.step_idx, transitions
Within the `run()` methodology, we name `step()` within the loop and yield its outputs till the system transits to the Finish step:
def run(self) -> Iterator[Tuple[int, List[AbstractYardState]]]:
whereas self.current_state != Finish(self):
yield self.step()
The `reset()` methodology resets the FSM reminiscence after the chicken leaves.
def reset(self) -> 'YardSimulation':
self.current_state = Begin(self)
self.intruder_class = Goal.EMPTY
self.detected_class = Goal.EMPTY
self.hit_successfully = False
self.spoiled = False
self.stayed_steps = 0return self
A chicken is leaving when both it’s efficiently hit by the water sprinkler or it stays too lengthy on the garden (e.g., assuming it obtained bored). The latter is equal to having a chicken current on the garden throughout 5 simulation steps (= minutes). Not that lengthy, who is aware of, perhaps the neighbor’s garden seems to be extra engaging.
Subsequent, let’s implement some important items of our system’s conduct. For (1), if no chicken is current on the garden (true intruder class), we attempt to spawn the one.
def simulate_intrusion(self) -> Goal:
if not self.bird_present:
self.intruder_class = self.spawn_target()return self.intruder_class
Right here, spawning pertains to the stay creation of the trespassing entity (chicken or nothing).
@property
def bird_present(self) -> bool:
return self.intruder_class != Goal.EMPTY
Then (2), the CV-based system — that’s described by a category confusion matrix — tries to detect and classify the intruding object. For this course of, we simulate a prediction technology, whereas conserving in thoughts the precise intruder class (floor reality).
def simulate_detection(self) -> Goal:
self.detected_class = self.get_random_target(self.intruder_class)return self.detected_class
Detector works on each timestep of the simulation, because the simulated system doesn’t know the bottom reality (in any other case, why would we’d like the detector?). If the detector identifies a chicken (level 3), we attempt to chase it away with the water sprinkler tuned to a particular water movement fee that depends upon the detected goal class:
def simulate_sprinkling(self) -> bool:
self.hit_successfully = self.bird_present and (self.rng.uniform() <= self.hit_proba) and self.target_vulnerablereturn self.hit_successfully
Whatever the success of the sprinkling, the system consumes water anyway. Hit success standards contains the next situations: a chicken was current on the garden (a), water sprinkler hit the chicken (b), the shot was enough/ample to deal with the chicken of a given measurement ©. Notice, that © the rooster “shot” received’t deal with the turkey, however applies in any other case.
Spoiling half (4) — a chicken can doubtlessly mess up with the grass. If this occurs, the garden injury fee will increase (clearly).
def simulate_spoiling(self) -> bool:
self.spoiled = self.bird_present and (self.rng.uniform() <= self.shit_proba)
if self.spoiled:
self.lawn_damage[self.intruder_class] += self.intruder_class.injuryreturn self.spoiled
Now we have now all of the necessities to simulate a single time step for the CaT drawback we’re going to deal with. Simulation time!
Chicken on the run
Now, we’re all set to make use of our FSM simulation to emulate an AI-assisted garden safety system throughout totally different settings. Whereas working a yard simulation, the `YardSimulation.run()` methodology iterates over a sequence of state transitions till the system reaches the restrict of steps. For this, we instantiate a simulation object (a.okay.a. state machine), setting the `num_steps` argument that displays the full quantity of the simulation timesteps (let’s say 12 hours or daytime) and `detector_matrix` that pertains to the confusion matrix of the CV-based chicken detector subsystem educated to foretell chickens and turkeys:
sim = YardSimulation(detector_matrix=detector_matrix, num_steps=num_steps)
Now we will run the FSM simulation and print state transitions that the FSM undergoes at each timestep:
for step_idx, states in sim.run():
print(f't{step_idx:0>3}: {" -> ".be part of(map(str, states))}')
As well as, we accumulate simulation statistics associated to the water utilization for chicken sprinkling (`simulate_sprinkling`) and grass cleansing after birds arrive (`simulate_spoiling`).
def simulate_sprinkling(self) -> bool:
...
self.water_consumption[self.detected_class] += self.detected_class.well being
...def simulate_spoiling(self) -> bool:
...
if self.spoiled:
self.lawn_damage[self.intruder_class] += self.intruder_class.injury
...
When the simulation reaches its restrict, we will then compute the full water consumption by the top of the day for every of the classes. What we wish to see is what occurs after every run of the simulation.
water_sprinkling_total = sum(sim.water_consumption.values())
lawn_damage_total = sum(sim.lawn_damage.values())
Lastly, let’s conduct experiments to evaluate how the system can carry out given adjustments within the laptop vision-based subsystem. To that finish, we’ll run simulations utilizing YardSimulation.run()` methodology for 100 trials for a non-trained (baseline) and ideal detection matrices:
detector_matrix_baseline = np.full(
(len(Goal),) * 2, # measurement of the confusion matrix (3 x 3)
len(Goal) ** -1 # prediction chance for every class is similar and equals to 1/3
)
detector_matrix_perfect = np.eye(len(Goal))
Thereafter, we will combination and evaluate output statistics associated to the full water utilization for goal sprinkling and garden cleansing for various experimental settings:
A comparability of abstract outcomes throughout experiments reveals that having a greater CV mannequin would contribute to elevated effectivity in minimizing water utilization by 37.8% (70.9 vs. 44.1), in comparison with the non-trained baseline detector for birds below the given enter parameters and simulation situations — an idea each intuitive and anticipated. However what does “higher” imply quantitatively? Is it value fiddling round with refining the mannequin? The numerical outcomes display the worth of bettering the mannequin, motivating additional refinement efforts. Going ahead, we’ll use the ensuing statistics as an goal for world optimization to enhance effectivity of the chicken detection subsystem and reduce down on water consumption for system operation and upkeep, making the engineer just a little happier.
To sum up, simulation modelling is a useful gizmo that can be utilized to estimate effectivity of processes, allow fast testing of anticipated adjustments, and perceive the right way to enhance processes by way of operation and upkeep. Via this text, you could have gained a greater understanding on sensible functions of simulation modelling for fixing engineering issues. Specifically, we’ve lined the next:
- design a mannequin to approximate a posh system to enhance its operation on chicken detection and water sprinkling.
- create a simulation of real-world processes to grasp the CaT-system conduct below varied situations.
- implement an FSM-based answer in Python for the system to trace related statistics of the simulation.
Specializing in bettering useful resource effectivity, within the follow-up articles, you’ll uncover the right way to tackle a non-analytic optimization drawback of the water value discount by making use of Monte-Carlo and eXplainable AI (XAI) strategies to reinforce the pc vision-based chicken detection subsystem, thus advancing our simulated AI-assisted garden safety system.
What are different essential ideas in simulation modelling and optimization for imaginative and prescient tasks? Discover out extra on Bird by Bird Tech.
- Forsyth, David. Chance and statistics for laptop science. Vol. 13. Cham: Springer Worldwide Publishing, 2018.
- Knuth, Donald Ervin. The artwork of laptop programming. Vol. 3. Studying, MA: Addison-Wesley, 1973.
- Wagner, Ferdinand, et al. Modeling software program with finite state machines: a sensible strategy. Auerbach Publications, 2006.