No Extra Tableau Downtime: Metadata API for Proactive Knowledge Well being
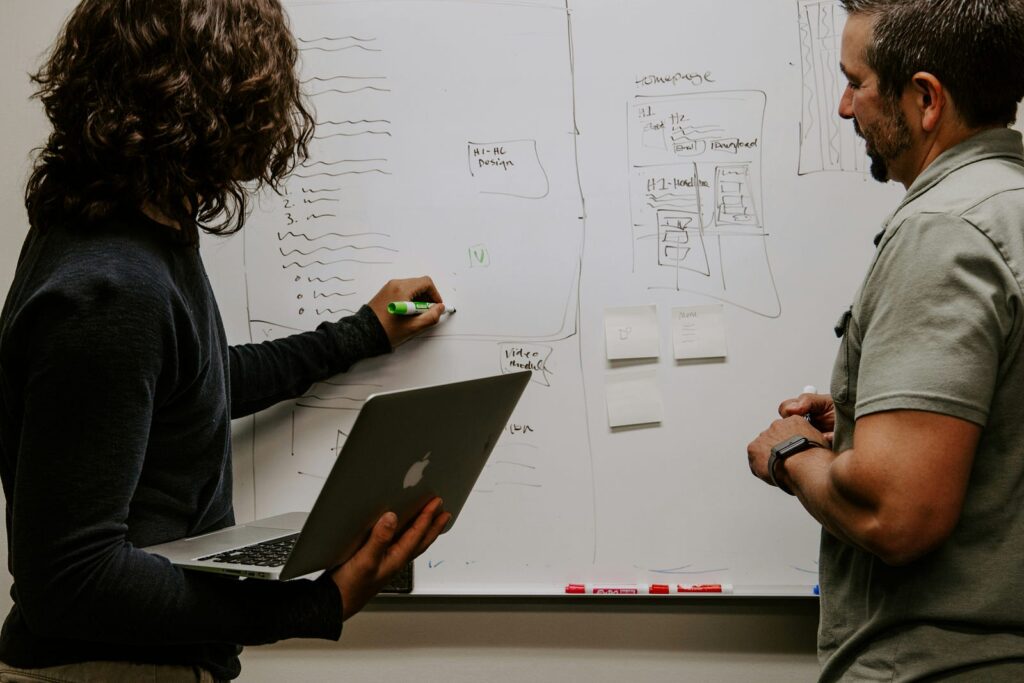
In at this time’s world, the reliability of information options is every thing. Once we construct dashboards and reviews, one expects that the numbers mirrored there are appropriate and up-to-date. Primarily based on these numbers, insights are drawn and actions are taken. For any unexpected motive, if the dashboards are damaged or if the numbers are incorrect — then it turns into a fire-fight to repair every thing. If the problems are usually not mounted in time, then it damages the belief positioned on the information group and their options.
However why would dashboards be damaged or have improper numbers? If the dashboard was constructed appropriately the primary time, then 99% of the time the difficulty comes from the information that feeds the dashboards — from the information warehouse. Some attainable eventualities are:
- Few ETL pipelines failed, so the brand new knowledge shouldn’t be but in
- A desk is changed with one other new one
- Some columns within the desk are dropped or renamed
- Schemas in knowledge warehouse have modified
- And lots of extra.
There may be nonetheless an opportunity that the difficulty is on the Tableau web site, however in my expertise, many of the occasions, it’s all the time as a consequence of some modifications in knowledge warehouse. Despite the fact that we all know the basis trigger, it’s not all the time easy to start out engaged on a repair. There may be no central place the place you’ll be able to test which Tableau knowledge sources depend on particular tables. If in case you have the Tableau Data Management add-on, it might assist, however from what I do know, its onerous to seek out dependencies of customized sql queries utilized in knowledge sources.
However, the add-on is just too costly and most corporations don’t have it. The actual ache begins when it’s important to undergo all the information sources manually to start out fixing it. On prime of it, you may have a string of customers in your head impatiently ready for a quick-fix. The repair itself won’t be tough, it might simply be a time-consuming one.
What if we might anticipate these points and establish impacted knowledge sources earlier than anybody notices an issue? Wouldn’t that simply be nice? Effectively, there’s a method now with the Tableau Metadata API. The Metadata API makes use of GraphQL, a question language for APIs that returns solely the information that you just’re all in favour of. For more information on what’s attainable with GraphQL, do try GraphQL.org.
On this weblog submit, I’ll present you the way to hook up with the Tableau Metadata API utilizing Python’s Tableau Server Consumer (TSC) library to proactively establish knowledge sources utilizing particular tables, so to act quick earlier than any points come up. As soon as which Tableau knowledge sources are affected by a particular desk, you can also make some updates your self or alert the house owners of these knowledge sources in regards to the upcoming modifications to allow them to be ready for it.
Connecting to the Tableau Metadata API
Lets hook up with the Tableau Server utilizing TSC. We have to import in all of the libraries we would wish for the train!
### Import all required libraries
import tableauserverclient as t
import pandas as pd
import json
import ast
import re
To be able to hook up with the Metadata API, you’ll have to first create a private entry token in your Tableau Account settings. Then replace the <API_TOKEN_NAME>
& <TOKEN_KEY>
with the token you simply created. Additionally replace <YOUR_SITE>
along with your Tableau web site. If the connection is established efficiently, then “Related” shall be printed within the output window.
### Connect with Tableau server utilizing private entry token
tableau_auth = t.PersonalAccessTokenAuth("<API_TOKEN_NAME>", "<TOKEN_KEY>",
site_id="<YOUR_SITE>")
server = t.Server("https://dub01.on-line.tableau.com/", use_server_version=True)
with server.auth.sign_in(tableau_auth):
print("Related")
Lets now get an inventory of all knowledge sources which can be revealed in your web site. There are various attributes you’ll be able to fetch, however for the present use case, lets preserve it easy and solely get the id, title and proprietor contact data for each knowledge supply. This shall be our grasp checklist to which we’ll add in all different data.
############### Get all of the checklist of information sources in your Web site
all_datasources_query = """ {
publishedDatasources {
title
id
proprietor {
title
e mail
}
}
}"""
with server.auth.sign_in(tableau_auth):
end result = server.metadata.question(
all_datasources_query
)
Since I need this weblog to be focussed on the right way to proactively establish which knowledge sources are affected by a particular desk, I’ll not be going into the nuances of Metadata API. To raised perceive how the question works, you’ll be able to consult with a really detailed Tableau’s personal Metadata API documentation.
One factor to notice is that the Metadata API returns knowledge in a JSON format. Relying on what you’re querying, you’ll find yourself with a number of nested json lists and it may well get very difficult to transform this right into a pandas dataframe. For the above metadata question, you’ll find yourself with a end result which would really like under (that is mock knowledge simply to provide you an concept of what the output appears like):
{
"knowledge": {
"publishedDatasources": [
{
"name": "Sales Performance DataSource",
"id": "f3b1a2c4-1234-5678-9abc-1234567890ab",
"owner": {
"name": "Alice Johnson",
"email": "[email protected]"
}
},
{
"title": "Buyer Orders DataSource",
"id": "a4d2b3c5-2345-6789-abcd-2345678901bc",
"proprietor": {
"title": "Bob Smith",
"e mail": "[email protected]"
}
},
{
"title": "Product Returns and Profitability",
"id": "c5e3d4f6-3456-789a-bcde-3456789012cd",
"proprietor": {
"title": "Alice Johnson",
"e mail": "[email protected]"
}
},
{
"title": "Buyer Segmentation Evaluation",
"id": "d6f4e5a7-4567-89ab-cdef-4567890123de",
"proprietor": {
"title": "Charlie Lee",
"e mail": "[email protected]"
}
},
{
"title": "Regional Gross sales Developments (Customized SQL)",
"id": "e7a5f6b8-5678-9abc-def0-5678901234ef",
"proprietor": {
"title": "Bob Smith",
"e mail": "[email protected]"
}
}
]
}
}
We have to convert this JSON response right into a dataframe in order that its straightforward to work with. Discover that we have to extract the title and e mail of the proprietor from contained in the proprietor object.
### We have to convert the response into dataframe for straightforward knowledge manipulation
col_names = end result['data']['publishedDatasources'][0].keys()
master_df = pd.DataFrame(columns=col_names)
for i in end result['data']['publishedDatasources']:
tmp_dt = {ok:v for ok,v in i.objects()}
master_df = pd.concat([master_df, pd.DataFrame.from_dict(tmp_dt, orient='index').T])
# Extract the proprietor title and e mail from the proprietor object
master_df['owner_name'] = master_df['owner'].apply(lambda x: x.get('title') if isinstance(x, dict) else None)
master_df['owner_email'] = master_df['owner'].apply(lambda x: x.get('e mail') if isinstance(x, dict) else None)
master_df.reset_index(inplace=True)
master_df.drop(['index','owner'], axis=1, inplace=True)
print('There are ', master_df.form[0] , ' datasources in your web site')
That is how the construction of master_df
would appear like:

As soon as we’ve got the principle checklist prepared, we are able to go forward and begin getting the names of the tables embedded within the knowledge sources. If you’re an avid Tableau consumer, that there are two methods to deciding on tables in a Tableau knowledge supply — one is to instantly select the tables and set up a relation between them and the opposite is to make use of a customized sql question with a number of tables to attain a brand new resultant desk. Due to this fact, we have to handle each the circumstances.
Processing of Customized SQL question tables
Beneath is the question to get the checklist of all customized SQLs used within the web site together with their knowledge sources. Discover that I’ve filtered the checklist to get solely first 500 customized sql queries. In case there are extra in your org, you’ll have to use an offset to get the subsequent set of customized sql queries. There may be additionally an possibility of utilizing cursor methodology in Pagination whenever you wish to fetch massive checklist of outcomes (refer here). For the sake of simplicity, I simply use the offset methodology as I do know, as there are lower than 500 customized sql queries used on the location.
# Get the information sources and the desk names from all of the customized sql queries used in your Web site
custom_table_query = """ {
customSQLTablesConnection(first: 500){
nodes {
id
title
downstreamDatasources {
title
}
question
}
}
}
"""
with server.auth.sign_in(tableau_auth):
custom_table_query_result = server.metadata.question(
custom_table_query
)
Primarily based on our mock knowledge, that is how our output would appear like:
{
"knowledge": {
"customSQLTablesConnection": {
"nodes": [
{
"id": "csql-1234",
"name": "RegionalSales_CustomSQL",
"downstreamDatasources": [
{
"name": "Regional Sales Trends (Custom SQL)"
}
],
"question": "SELECT r.region_name, SUM(s.sales_amount) AS total_sales FROM ecommerce.sales_data.Gross sales s JOIN ecommerce.sales_data.Areas r ON s.region_id = r.region_id GROUP BY r.region_name"
},
{
"id": "csql-5678",
"title": "ProfitabilityAnalysis_CustomSQL",
"downstreamDatasources": [
{
"name": "Product Returns and Profitability"
}
],
"question": "SELECT p.product_category, SUM(s.revenue) AS total_profit FROM ecommerce.sales_data.Gross sales s JOIN ecommerce.sales_data.Merchandise p ON s.product_id = p.product_id GROUP BY p.product_category"
},
{
"id": "csql-9101",
"title": "CustomerSegmentation_CustomSQL",
"downstreamDatasources": [
{
"name": "Customer Segmentation Analysis"
}
],
"question": "SELECT c.customer_id, c.location, COUNT(o.order_id) AS total_orders FROM ecommerce.sales_data.Prospects c JOIN ecommerce.sales_data.Orders o ON c.customer_id = o.customer_id GROUP BY c.customer_id, c.location"
},
{
"id": "csql-3141",
"title": "CustomerOrders_CustomSQL",
"downstreamDatasources": [
{
"name": "Customer Orders DataSource"
}
],
"question": "SELECT o.order_id, o.customer_id, o.order_date, o.sales_amount FROM ecommerce.sales_data.Orders o WHERE o.order_status = 'Accomplished'"
},
{
"id": "csql-3142",
"title": "CustomerProfiles_CustomSQL",
"downstreamDatasources": [
{
"name": "Customer Orders DataSource"
}
],
"question": "SELECT c.customer_id, c.customer_name, c.section, c.location FROM ecommerce.sales_data.Prospects c WHERE c.active_flag = 1"
},
{
"id": "csql-3143",
"title": "CustomerReturns_CustomSQL",
"downstreamDatasources": [
{
"name": "Customer Orders DataSource"
}
],
"question": "SELECT r.return_id, r.order_id, r.return_reason FROM ecommerce.sales_data.Returns r"
}
]
}
}
}
Identical to earlier than after we have been creating the grasp checklist of information sources, right here additionally we’ve got nested json for the downstream knowledge sources the place we would wish to extract solely the “title” a part of it. Within the “question” column, all the customized sql is dumped. If we use regex sample, we are able to simply seek for the names of the desk used within the question.
We all know that the desk names all the time come after FROM or a JOIN clause and so they usually observe the format <database_name>.<schema>.<table_name>
. The <database_name>
is optionally available and many of the occasions not used. There have been some queries I discovered which used this format and I ended up solely getting the database and schema names, and never the entire desk title. As soon as we’ve got extracted the names of the information sources and the names of the tables, we have to merge the rows per knowledge supply as there will be a number of customized sql queries utilized in a single knowledge supply.
### Convert the customized sql response into dataframe
col_names = custom_table_query_result['data']['customSQLTablesConnection']['nodes'][0].keys()
cs_df = pd.DataFrame(columns=col_names)
for i in custom_table_query_result['data']['customSQLTablesConnection']['nodes']:
tmp_dt = {ok:v for ok,v in i.objects()}
cs_df = pd.concat([cs_df, pd.DataFrame.from_dict(tmp_dt, orient='index').T])
# Extract the information supply title the place the customized sql question was used
cs_df['data_source'] = cs_df.downstreamDatasources.apply(lambda x: x[0]['name'] if x and 'title' in x[0] else None)
cs_df.reset_index(inplace=True)
cs_df.drop(['index','downstreamDatasources'], axis=1,inplace=True)
### We have to extract the desk names from the sql question. We all know the desk title comes after FROM or JOIN clause
# Be aware that the title of desk will be of the format <data_warehouse>.<schema>.<table_name>
# Relying on the format of how desk is known as, you'll have to modify the regex expression
def extract_tables(sql):
# Regex to match database.schema.desk or schema.desk, keep away from alias
sample = r'(?:FROM|JOIN)s+((?:[w+]|w+).(?:[w+]|w+)(?:.(?:[w+]|w+))?)b'
matches = re.findall(sample, sql, re.IGNORECASE)
return checklist(set(matches)) # Distinctive desk names
cs_df['customSQLTables'] = cs_df['query'].apply(extract_tables)
cs_df = cs_df[['data_source','customSQLTables']]
# We have to merge datasources as there will be a number of customized sqls utilized in the identical knowledge supply
cs_df = cs_df.groupby('data_source', as_index=False).agg({
'customSQLTables': lambda x: checklist(set(merchandise for sublist in x for merchandise in sublist)) # Flatten & make distinctive
})
print('There are ', cs_df.form[0], 'datasources with customized sqls utilized in it')
After we carry out all of the above operations, that is how the construction of cs_df
would appear like:

Processing of normal Tables in Knowledge Sources
Now we have to get the checklist of all of the common tables utilized in a datasource which aren’t part of customized SQL. There are two methods to go about it. Both use the publishedDatasources
object and test for upstreamTables
or use DatabaseTable
and test for upstreamDatasources
. I’ll go by the primary methodology as a result of I need the outcomes at an information supply stage (principally, I need some code able to reuse after I wish to test a particular knowledge supply in additional element). Right here once more, for the sake of simplicity, as an alternative of going for pagination, I’m looping by means of every datasource to make sure I’ve every thing. We get the upstreamTables
within the sphere object in order that must be cleaned out.
############### Get the information sources with the common desk names utilized in your web site
### Its greatest to extract the tables data for each knowledge supply after which merge the outcomes.
# Since we solely get the desk data nested beneath fields, in case there are a whole lot of fields
# utilized in a single knowledge supply, we'll hit the response limits and won't be able to retrieve all the information.
data_source_list = master_df.title.tolist()
col_names = ['name', 'id', 'extractLastUpdateTime', 'fields']
ds_df = pd.DataFrame(columns=col_names)
with server.auth.sign_in(tableau_auth):
for ds_name in data_source_list:
question = """ {
publishedDatasources (filter: { title: """"+ ds_name + """" }) {
title
id
extractLastUpdateTime
fields {
title
upstreamTables {
title
}
}
}
} """
ds_name_result = server.metadata.question(
question
)
for i in ds_name_result['data']['publishedDatasources']:
tmp_dt = {ok:v for ok,v in i.objects() if ok != 'fields'}
tmp_dt['fields'] = json.dumps(i['fields'])
ds_df = pd.concat([ds_df, pd.DataFrame.from_dict(tmp_dt, orient='index').T])
ds_df.reset_index(inplace=True)
That is how the construction of ds_df
would look:

We will have to flatten out the fields
object and extract the sphere names in addition to the desk names. Because the desk names shall be repeating a number of occasions, we must deduplicate to maintain solely the distinctive ones.
# Perform to extract the values of fields and upstream tables in json lists
def extract_values(json_list, key):
values = []
for merchandise in json_list:
values.append(merchandise[key])
return values
ds_df["fields"] = ds_df["fields"].apply(ast.literal_eval)
ds_df['field_names'] = ds_df.apply(lambda x: extract_values(x['fields'],'title'), axis=1)
ds_df['upstreamTables'] = ds_df.apply(lambda x: extract_values(x['fields'],'upstreamTables'), axis=1)
# Perform to extract the distinctive desk names
def extract_upstreamTable_values(table_list):
values = set()a
for inner_list in table_list:
for merchandise in inner_list:
if 'title' in merchandise:
values.add(merchandise['name'])
return checklist(values)
ds_df['upstreamTables'] = ds_df.apply(lambda x: extract_upstreamTable_values(x['upstreamTables']), axis=1)
ds_df.drop(["index","fields"], axis=1, inplace=True)
As soon as we do the above operations, the ultimate construction of ds_df
would look one thing like this:

We’ve all of the items and now we simply should merge them collectively:
###### Be a part of all the information collectively
master_data = pd.merge(master_df, ds_df, how="left", on=["name","id"])
master_data = pd.merge(master_data, cs_df, how="left", left_on="title", right_on="data_source")
# Save the outcomes to analyse additional
master_data.to_excel("Tableau Knowledge Sources with Tables.xlsx", index=False)
That is our remaining master_data
:

Desk-level Influence Evaluation
Let’s say there have been some schema modifications on the “Gross sales” desk and also you wish to know which knowledge sources shall be impacted. Then you’ll be able to merely write a small perform which checks if a desk is current in both of the 2 columns — upstreamTables
or customSQLTables
like under.
def filter_rows_with_table(df, col1, col2, target_table):
"""
Filters rows in df the place target_table is a part of any worth in both col1 or col2 (helps partial match).
Returns full rows (all columns retained).
"""
return df[
df.apply(
lambda row:
(isinstance(row[col1], checklist) and any(target_table in merchandise for merchandise in row[col1])) or
(isinstance(row[col2], checklist) and any(target_table in merchandise for merchandise in row[col2])),
axis=1
)
]
# For instance
filter_rows_with_table(master_data, 'upstreamTables', 'customSQLTables', 'Gross sales')
Beneath is the output. You may see that 3 knowledge sources shall be impacted by this alteration. You can even alert the information supply house owners Alice and Bob prematurely about this to allow them to begin engaged on a repair earlier than one thing breaks on the Tableau dashboards.

You may try the entire model of the code in my Github repository here.
That is simply one of many potential use-cases of the Tableau Metadata API. You can even extract the sphere names utilized in customized sql queries and add to the dataset to get a field-level impression evaluation. One can even monitor the stale knowledge sources with the extractLastUpdateTime
to see if these have any points or have to be archived if they don’t seem to be used any extra. We will additionally use the dashboards
object to fetch data at a dashboard stage.
Last Ideas
If in case you have come this far, kudos. This is only one use case of automating Tableau knowledge administration. It’s time to mirror by yourself work and assume which of these different duties you can automate to make your life simpler. I hope this mini-project served as an fulfilling studying expertise to grasp the ability of Tableau Metadata API. For those who favored studying this, you may also like one other one in every of my weblog posts about Tableau, on a number of the challenges I confronted when coping with large .
Additionally do try my earlier weblog the place I explored constructing an interactive, database-powered app with Python, Streamlit, and SQLite.
Earlier than you go…
Observe me so that you don’t miss any new posts I write in future; you will see that extra of my articles on my . You can even join with me on LinkedIn or Twitter!