Construct highly effective RAG pipelines with LlamaIndex and Amazon Bedrock
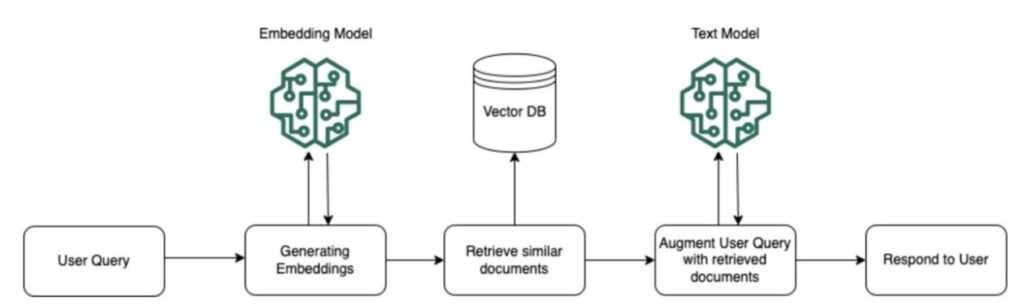
This put up was co-written with Jerry Liu from LlamaIndex.
Retrieval Augmented Technology (RAG) has emerged as a robust method for enhancing the capabilities of enormous language fashions (LLMs). By combining the huge data saved in exterior knowledge sources with the generative energy of LLMs, RAG allows you to sort out advanced duties that require each data and creativity. At the moment, RAG strategies are utilized in each enterprise, small and enormous, the place generative artificial intelligence (AI) is used as an enabler for fixing document-based query answering and different sorts of evaluation.
Though constructing a easy RAG system is easy, constructing manufacturing RAG methods utilizing superior patterns is difficult. A manufacturing RAG pipeline usually operates over a bigger knowledge quantity and bigger knowledge complexity, and should meet the next high quality bar in comparison with constructing a proof of idea. A normal broad problem that builders face is low response high quality; the RAG pipeline shouldn’t be in a position to sufficiently reply numerous questions. This may be as a consequence of a wide range of causes; the next are among the most typical:
- Dangerous retrievals – The related context wanted to reply the query is lacking.
- Incomplete responses – The related context is partially there however not utterly. The generated output doesn’t totally reply the enter query.
- Hallucinations – The related context is there however the mannequin shouldn’t be in a position to extract the related info so as to reply the query.
This necessitates extra superior RAG strategies on the question understanding, retrieval, and technology parts so as to deal with these failure modes.
That is the place LlamaIndex is available in. LlamaIndex is an open supply library with each easy and superior strategies that permits builders to construct manufacturing RAG pipelines. It supplies a versatile and modular framework for constructing and querying doc indexes, integrating with varied LLMs, and implementing superior RAG patterns.
Amazon Bedrock is a managed service offering entry to high-performing basis fashions (FMs) from main AI suppliers by means of a unified API. It affords a variety of enormous fashions to select from, together with capabilities to securely construct and customise generative AI purposes. Key superior options embrace mannequin customization with fine-tuning and continued pre-training utilizing your personal knowledge, in addition to RAG to enhance mannequin outputs by retrieving context from configured data bases containing your non-public knowledge sources. You may also create clever brokers that orchestrate FMs with enterprise methods and knowledge. Different enterprise capabilities embrace provisioned throughput for assured low-latency inference at scale, mannequin analysis to check efficiency, and AI guardrails to implement safeguards. Amazon Bedrock abstracts away infrastructure administration by means of a totally managed, serverless expertise.
On this put up, we discover tips on how to use LlamaIndex to construct superior RAG pipelines with Amazon Bedrock. We talk about tips on how to arrange the next:
- Easy RAG pipeline – Arrange a RAG pipeline in LlamaIndex with Amazon Bedrock fashions and top-k vector search
- Router question – Add an automatic router that may dynamically do semantic search (top-k) or summarization over knowledge
- Sub-question question – Add a question decomposition layer that may decompose advanced queries into a number of easier ones, and run them with the related instruments
- Agentic RAG – Construct a stateful agent that may do the previous parts (software use, question decomposition), but in addition keep state-like dialog historical past and reasoning over time
Easy RAG pipeline
At its core, RAG entails retrieving related info from exterior knowledge sources and utilizing it to enhance the prompts fed to an LLM. This permits the LLM to generate responses which are grounded in factual data and tailor-made to the particular question.
For RAG workflows in Amazon Bedrock, paperwork from configured data bases undergo preprocessing, the place they’re cut up into chunks, embedded into vectors, and listed in a vector database. This permits environment friendly retrieval of related info at runtime. When a consumer question is available in, the identical embedding mannequin is used to transform the question textual content right into a vector illustration. This question vector is in contrast in opposition to the listed doc vectors to determine essentially the most semantically related chunks from the data base. The retrieved chunks present further context associated to the consumer’s question. This contextual info is appended to the unique consumer immediate earlier than being handed to the FM to generate a response. By augmenting the immediate with related knowledge pulled from the data base, the mannequin’s output is ready to use and learn by a corporation’s proprietary info sources. This RAG course of can be orchestrated by brokers, which use the FM to find out when to question the data base and tips on how to incorporate the retrieved context into the workflow.
The next diagram illustrates this workflow.
The next is a simplified instance of a RAG pipeline utilizing LlamaIndex:
The pipeline contains the next steps:
- Use the
SimpleDirectoryReader
to load paperwork from the “knowledge/” - Create a
VectorStoreIndex
from the loaded paperwork. Such a index converts paperwork into numerical representations (vectors) that seize their semantic that means. - Question the index with the query “What’s the capital of France?” The index makes use of similarity measures to determine the paperwork most related to the question.
- The retrieved paperwork are then used to enhance the immediate for the LLM, which generates a response primarily based on the mixed info.
LlamaIndex goes past easy RAG and allows the implementation of extra refined patterns, which we talk about within the following sections.
Router question
RouterQueryEngine
lets you route queries to completely different indexes or question engines primarily based on the character of the question. For instance, you can route summarization inquiries to a abstract index and factual inquiries to a vector retailer index.
The next is a code snippet from the instance notebooks demonstrating RouterQueryEngine:
Sub-question question
SubQuestionQueryEngine
breaks down advanced queries into easier sub-queries after which combines the solutions from every sub-query to generate a complete response. That is notably helpful for queries that span throughout a number of paperwork. It first breaks down the advanced question into sub-questions for every related knowledge supply, then gathers the intermediate responses and synthesizes a last response that integrates the related info from every sub-query. For instance, if the unique question was “What’s the inhabitants of the capital metropolis of the nation with the best GDP in Europe,” the engine would first break it down into sub-queries like “What’s the highest GDP nation in Europe,” “What’s the capital metropolis of that nation,” and “What’s the inhabitants of that capital metropolis,” after which mix the solutions to these sub-queries right into a last complete response.
The next is an instance of utilizing SubQuestionQueryEngine
:
Agentic RAG
An agentic method to RAG makes use of an LLM to cause in regards to the question and decide which instruments (akin to indexes or question engines) to make use of and in what sequence. This permits for a extra dynamic and adaptive RAG pipeline. The next structure diagram exhibits how agentic RAG works on Amazon Bedrock.
Agentic RAG in Amazon Bedrock combines the capabilities of brokers and data bases to allow RAG workflows. Brokers act as clever orchestrators that may question data bases throughout their workflow to retrieve related info and context to enhance the responses generated by the FM.
After the preliminary preprocessing of the consumer enter, the agent enters an orchestration loop. On this loop, the agent invokes the FM, which generates a rationale outlining the subsequent step the agent ought to take. One potential step is to question an connected data base to retrieve supplemental context from the listed paperwork and knowledge sources.
If a data base question is deemed helpful, the agent invokes an InvokeModel
name particularly for data base response technology. This fetches related doc chunks from the data base primarily based on semantic similarity to the present context. These retrieved chunks present further info that’s included within the immediate despatched again to the FM. The mannequin then generates an remark response that’s parsed and might invoke additional orchestration steps, like invoking exterior APIs (by means of motion group AWS Lambda features) or present a last response to the consumer. This agentic orchestration augmented by data base retrieval continues till the request is totally dealt with.
One instance of an agent orchestration loop is the ReAct agent, which was initially launched by Yao et al. ReAct interleaves chain-of-thought and gear use. At each stage, the agent takes within the enter job together with the earlier dialog historical past and decides whether or not to invoke a software (akin to querying a data base) with the suitable enter or not.
The next is an instance of utilizing the ReAct agent with the LlamaIndex SDK:
The ReAct agent will analyze the question and resolve whether or not to make use of the Lyft 10K software or one other software to reply the query. To check out agentic RAG, consult with the GitHub repo.
LlamaCloud and LlamaParse
LlamaCloud represents a major development within the LlamaIndex panorama, providing a complete suite of managed providers tailor-made for enterprise-grade context augmentation inside LLM and RAG purposes. This service empowers AI engineers to focus on growing core enterprise logic by streamlining the intricate course of of information wrangling.
One key element is LlamaParse, a proprietary parsing engine adept at dealing with advanced, semi-structured paperwork replete with embedded objects like tables and figures, seamlessly integrating with LlamaIndex’s ingestion and retrieval pipelines. One other key element is the Managed Ingestion and Retrieval API, which facilitates easy loading, processing, and storage of information from numerous sources, together with LlamaParse outputs and LlamaHub’s centralized knowledge repository, whereas accommodating varied knowledge storage integrations.
Collectively, these options allow the processing of huge manufacturing knowledge volumes, culminating in enhanced response high quality and unlocking unprecedented capabilities in context-aware query answering for RAG purposes. To study extra about these options, consult with Introducing LlamaCloud and LlamaParse.
For this put up, we use LlamaParse to showcase the mixing with Amazon Bedrock. LlamaParse is an API created by LlamaIndex to effectively parse and signify information for environment friendly retrieval and context augmentation utilizing LlamaIndex frameworks. What is exclusive about LlamaParse is that it’s the world’s first generative AI native doc parsing service, which permits customers to submit paperwork together with parsing directions. The important thing perception behind parsing directions is that you understand what sort of paperwork you may have, so that you already know what sort of output you need. The next determine exhibits a comparability of parsing a fancy PDF with LlamaParse vs. two in style open supply PDF parsers.
A inexperienced spotlight in a cell implies that the RAG pipeline appropriately returned the cell worth as the reply to a query over that cell. A crimson spotlight implies that the query was answered incorrectly.
Combine Amazon Bedrock and LlamaIndex to construct an Superior RAG Pipeline
On this part, we present you tips on how to construct a sophisticated RAG stack combining LlamaParse and LlamaIndex with Amazon Bedrock providers – LLMs, embedding fashions, and Bedrock Information Base.
To make use of LlamaParse with Amazon Bedrock, you may comply with these high-level steps:
- Obtain your supply paperwork.
- Ship the paperwork to LlamaParse utilizing the Python SDK:
- Look ahead to the parsing job to complete and add the ensuing Markdown paperwork to Amazon Simple Storage Service (Amazon S3).
- Create an Amazon Bedrock data base utilizing the supply paperwork.
- Select your most popular embedding and technology mannequin from Amazon Bedrock utilizing the LlamaIndex SDK:
- Implement a sophisticated RAG sample utilizing LlamaIndex. Within the following instance, we use
SubQuestionQueryEngine
and a retriever specifically created for Amazon Bedrock data bases: - Lastly, question the index along with your query:
We examined Llamaparse on a real-world, difficult instance of asking questions on a doc containing Financial institution of America Q3 2023 monetary outcomes. An instance slide from the full slide deck (48 advanced slides!) is proven under.
Utilizing the process outlined above, we requested “What’s the development in digital households/relationships from 3Q20 to 3Q23?”; check out the reply generated utilizing Llamaindex instruments vs. the reference reply from human annotation.
LlamaIndex + LlamaParse reply | Reference reply |
The development in digital households/relationships exhibits a gradual enhance from 3Q20 to 3Q23. In 3Q20, the variety of digital households/relationships was 550K, which elevated to 645K in 3Q21, then to 672K in 3Q22, and additional to 716K in 3Q23. This means constant progress within the adoption of digital providers amongst households and relationships over the reported quarters. | The development exhibits a gradual enhance in digital households/relationships from 645,000 in 3Q20 to 716,000 in 3Q23. The digital adoption proportion additionally elevated from 76% to 83% over the identical interval. |
The next are instance notebooks to check out these steps by yourself examples. Word the prerequisite steps and cleanup assets after testing them.
Conclusion
On this put up, we explored varied superior RAG patterns with LlamaIndex and Amazon Bedrock. To delve deeper into the capabilities of LlamaIndex and its integration with Amazon Bedrock, try the next assets:
By combining the ability of LlamaIndex and Amazon Bedrock, you may construct strong and complex RAG pipelines that unlock the complete potential of LLMs for knowledge-intensive duties.
Concerning the Creator
Shreyas Subramanian is a Principal knowledge scientist and helps prospects by utilizing Machine Studying to resolve their enterprise challenges utilizing the AWS platform. Shreyas has a background in giant scale optimization and Machine Studying, and in use of Machine Studying and Reinforcement Studying for accelerating optimization duties.
Jerry Liu is the co-founder/CEO of LlamaIndex, an information framework for constructing LLM purposes. Earlier than this, he has spent his profession on the intersection of ML, analysis, and startups. He led the ML monitoring staff at Strong Intelligence, did self-driving AI analysis at Uber ATG, and labored on suggestion methods at Quora.