Enhance RAG accuracy with fine-tuned embedding fashions on Amazon SageMaker
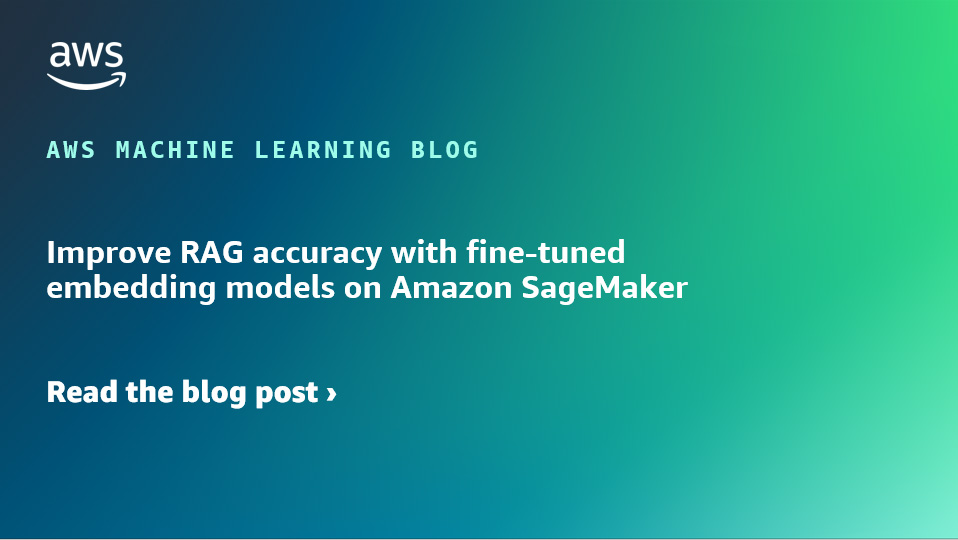
Retrieval Augmented Technology (RAG) is a well-liked paradigm that gives further data to giant language fashions (LLMs) from an exterior supply of knowledge that wasn’t current of their coaching corpus.
RAG supplies further data to the LLM via its enter immediate area and its structure usually consists of the next elements:
- Indexing: Put together a corpus of unstructured textual content, parse and chunk it, after which, embed every chunk and retailer it in a vector database.
- Retrieval: Retrieve context related to answering a query from the vector database utilizing vector similarity. Use immediate engineering to offer this extra context to the LLM together with the unique query. The LLM will then use the unique query and the context from the vector database to generate a solution based mostly on knowledge that wasn’t a part of its coaching corpus.
Challenges in RAG accuracy
Pre-trained embedding fashions are usually educated on giant, general-purpose datasets like Wikipedia or web-crawl knowledge. Whereas these fashions seize a broad vary of semantic relationships and may generalize effectively throughout varied duties, they could battle to precisely signify domain-specific ideas and nuances. This limitation can result in suboptimal efficiency when utilizing these pre-trained embeddings for specialised duties or domains, akin to authorized, medical, or technical domains. Moreover, pre-trained embeddings may not successfully seize the contextual relationships and nuances which are particular to a selected process or area. For instance, within the authorized area, the identical time period can have totally different meanings or implications relying on the context, and these nuances may not be adequately represented in a general-purpose embedding mannequin.
To deal with the restrictions of pre-trained embeddings and enhance the accuracy of RAG methods for particular domains or duties, it’s important to effective tune the embedding mannequin on domain-specific knowledge. By effective tuning the mannequin on knowledge that’s consultant of the goal area or process, the mannequin can be taught to seize the related semantics, jargon, and contextual relationships which are essential for that area.
Area-specific embeddings can considerably enhance the standard of vector representations, resulting in extra correct retrieval of related context from the vector database. This, in flip, enhances the efficiency of the RAG system by way of producing extra correct and related responses.
This put up demonstrates easy methods to use Amazon SageMaker to effective tune a Sentence Transformer embedding mannequin and deploy it with an Amazon SageMaker Endpoint. The code from this put up and extra examples can be found within the GitHub repo. For extra details about effective tuning Sentence Transformer, see Sentence Transformer training overview.
Tremendous tuning embedding fashions utilizing SageMaker
SageMaker is a totally managed machine studying service that simplifies all the machine studying workflow, from knowledge preparation and mannequin coaching to deployment and monitoring. It supplies a seamless and built-in setting that abstracts away the complexities of infrastructure administration, permitting builders and knowledge scientists to focus solely on constructing and iterating their machine studying fashions.
One of many key strengths of SageMaker is its native help for fashionable open supply frameworks akin to TensorFlow, PyTorch, and Hugging Face transformers. This integration allows seamless mannequin coaching and deployment utilizing these frameworks, their highly effective capabilities and in depth ecosystem of libraries and instruments.
SageMaker additionally gives a spread of built-in algorithms for frequent use instances like pc imaginative and prescient, pure language processing, and tabular knowledge, making it straightforward to get began with pre-built fashions for varied duties. SageMaker additionally helps distributed coaching and hyperparameter tuning, permitting for environment friendly and scalable mannequin coaching.
Stipulations
For this walkthrough, it’s best to have the next stipulations:
Steps to effective tune embedding fashions on Amazon SageMaker
Within the following sections, we use a SageMaker JupyterLab to stroll via the steps of knowledge preparation, making a coaching script, coaching the mannequin, and deploying it as a SageMaker endpoint.
We are going to effective tune the embedding mannequin sentence-transformers, all-MiniLM-L6-v2, which is an open supply Sentence Transformers mannequin effective tuned on a 1B sentence pairs dataset. It maps sentences and paragraphs to a 384-dimensional dense vector area and can be utilized for duties like clustering or semantic search. To effective tune it, we are going to use the Amazon Bedrock FAQs, a dataset of query and reply pairs, utilizing the MultipleNegativesRankingLoss function.
In Losses, you’ll find the totally different loss capabilities that can be utilized to fine-tune embedding fashions on coaching knowledge. The selection of loss operate performs a crucial function when effective tuning the mannequin. It determines how effectively our embedding mannequin will work for the particular downstream process.
The MultipleNegativesRankingLoss
operate is beneficial once you solely have optimistic pairs in your coaching knowledge, for instance, solely pairs of comparable texts like pairs of paraphrases, pairs of duplicate questions, pairs of question and response, or pairs of (source_language
and target_language
).
In our case, contemplating that we’re utilizing Amazon Bedrock FAQs as coaching knowledge, which consists of pairs of questions and solutions, the MultipleNegativesRankingLoss
operate may very well be match.
The next code snippet demonstrates easy methods to load a coaching dataset from a JSON file, prepares the info for coaching, after which effective tunes the pre-trained mannequin. After effective tuning, the up to date mannequin is saved.
The EPOCHS
variable determines the variety of occasions the mannequin will iterate over all the coaching dataset in the course of the fine-tuning course of. The next variety of epochs usually results in higher convergence and doubtlessly improved efficiency however may also improve the danger of overfitting if not correctly regularized.
On this instance, we’ve a small coaching set consisting of solely 100 data. Consequently, we’re utilizing a excessive worth for the EPOCHS
parameter. Sometimes, in real-world eventualities, you’ll have a a lot bigger coaching set. In such instances, the EPOCHS
worth must be a single- or two-digit quantity to keep away from overfitting the mannequin to the coaching knowledge.
To deploy and serve the fine-tuned embedding mannequin for inference, we create an inference.py
Python script that serves because the entry level. This script implements two important capabilities: model_fn
and predict_fn
, as required by SageMaker for deploying and utilizing machine studying fashions.
The model_fn
operate is accountable for loading the fine-tuned embedding mannequin and the related tokenizer. The predict_fn
operate takes enter sentences, tokenizes them utilizing the loaded tokenizer, and computes their sentence embeddings utilizing the fine-tuned mannequin. To acquire a single vector illustration for every sentence, it performs imply pooling over the token embeddings adopted by normalization of the ensuing embedding. Lastly, predict_fn
returns the normalized embeddings as an inventory, which might be additional processed or saved as required.
After creating the inference.py
script, we package deal it along with the fine-tuned embedding mannequin right into a single mannequin.tar.gz
file. This compressed file can then be uploaded to an S3 bucket, making it accessible for deployment as a SageMaker endpoint.
Lastly, we will deploy our fine-tuned mannequin in a SageMaker endpoint.
After the deployment is accomplished, you’ll find the deployed SageMaker endpoint within the AWS Administration Console for SageMaker by selecting the Inference from the navigation pane, after which selecting Endpoints.
You have got a number of choices to invoke you endpoint. For instance, in your SageMaker JupyterLab, you possibly can invoke it with the next code snippet:
It returns the vector containing the embedding of the inputs key:
For example the affect of effective tuning, we will evaluate the cosine similarity scores between two semantically associated sentences utilizing each the unique pre-trained mannequin and the fine-tuned mannequin. The next cosine similarity rating signifies that the 2 sentences are extra semantically comparable, as a result of their embeddings are nearer within the vector area.
Let’s think about the next pair of sentences:
- What are brokers, and the way can they be used?
- Brokers for Amazon Bedrock are totally managed capabilities that mechanically break down duties, create an orchestration plan, securely connect with firm knowledge via APIs, and generate correct responses for advanced duties like automating stock administration or processing insurance coverage claims.
These sentences are associated to the idea of brokers within the context of Amazon Bedrock, though with totally different ranges of element. By producing embeddings for these sentences utilizing each fashions and calculating their cosine similarity, we will consider how effectively every mannequin captures the semantic relationship between them.
The unique pre-trained mannequin returns a similarity rating of solely 0.54.
The fine-tuned mannequin returns a similarity rating of 0.87.
We are able to observe how the fine-tuned mannequin was in a position to establish a a lot greater semantic similarity between the ideas of agents and Brokers for Amazon Bedrock when in comparison with the pre-trained mannequin. This enchancment is attributed to the fine-tuning course of, which uncovered the mannequin to the domain-specific language and ideas current within the Amazon Bedrock FAQs knowledge, enabling it to raised seize the connection between these phrases.
Clear up
To keep away from future fees in your account, delete the assets you created on this walkthrough. The SageMaker endpoint and the SageMaker JupyterLab occasion will incur fees so long as the cases are lively, so once you’re executed delete the endpoint and resources that you created whereas operating the walkthrough.
Conclusion
On this weblog put up, we’ve explored the significance of effective tuning embedding fashions to enhance the accuracy of RAG methods in particular domains or duties. We mentioned the restrictions of pre-trained embeddings, that are educated on general-purpose datasets and may not seize the nuances and domain-specific semantics required for specialised domains or duties.
We highlighted the necessity for domain-specific embeddings, which might be obtained by effective tuning the embedding mannequin on knowledge consultant of the goal area or process. This course of permits the mannequin to seize the related semantics, jargon, and contextual relationships which are essential for correct vector representations and, consequently, higher retrieval efficiency in RAG methods.
We then demonstrated easy methods to effective tune embedding fashions on Amazon SageMaker utilizing the favored Sentence Transformers library.
By effective tuning embeddings on domain-specific knowledge utilizing SageMaker, you possibly can unlock the complete potential of RAG methods, enabling extra correct and related responses tailor-made to your particular area or process. This strategy might be notably invaluable in domains like authorized, medical, or technical fields the place capturing domain-specific nuances is essential for producing high-quality and reliable outputs.
This and extra examples can be found within the GitHub repo. Attempt it out at the moment utilizing the Set up for single users (Quick setup) on Amazon SageMaker and tell us what you suppose within the feedback.
Concerning the Authors
Ennio Emanuele Pastore is a Senior Architect on the AWS GenAI Labs group. He’s an fanatic of every little thing associated to new applied sciences which have a optimistic affect on companies and common livelihood. He helps organizations in reaching particular enterprise outcomes through the use of knowledge and AI and accelerating their AWS Cloud adoption journey.