Complete Information to Datasets and Dataloaders in PyTorch | by Ryan D’Cunha | Jun, 2024
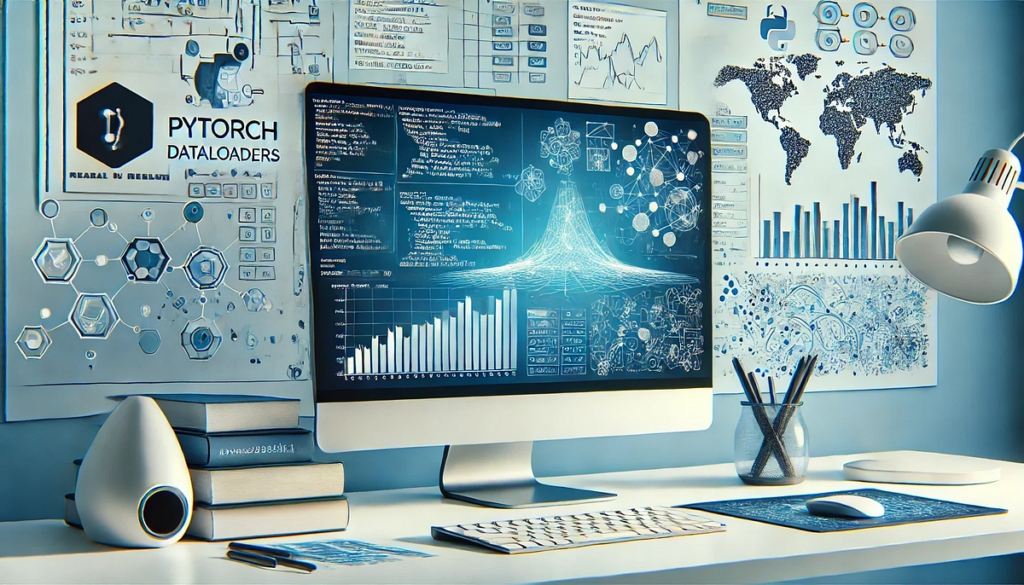
The total information to creating customized datasets and dataloaders for various fashions in PyTorch
Earlier than you’ll be able to construct a machine studying mannequin, you might want to load your information right into a dataset. Fortunately, PyTorch has many instructions to assist with this complete course of (if you’re not aware of PyTorch I like to recommend refreshing on the fundamentals here).
PyTorch has good documentation to assist with this course of, however I’ve not discovered any complete documentation or tutorials in direction of customized datasets. I’m first going to begin with creating fundamental premade datasets after which work my method as much as creating datasets from scratch for various fashions!
Earlier than we dive into code for various use instances, let’s perceive the distinction between the 2 phrases. Usually, you first create your dataset after which create a dataloader. A dataset comprises the options and labels from every information level that can be fed into the mannequin. A dataloader is a customized PyTorch iterable that makes it straightforward to load information with added options.
DataLoader(dataset, batch_size=1, shuffle=False, sampler=None,
batch_sampler=None, num_workers=0, collate_fn=None,
pin_memory=False, drop_last=False, timeout=0,
worker_init_fn=None, *, prefetch_factor=2,
persistent_workers=False)
The commonest arguments within the dataloader are batch_size, shuffle (normally just for the coaching information), num_workers (to multi-process loading the info), and pin_memory (to place the fetched information Tensors in pinned reminiscence and allow quicker information switch to CUDA-enabled GPUs).
It is strongly recommended to set pin_memory = True as an alternative of specifying num_workers attributable to multiprocessing issues with CUDA.
Within the case that your dataset is downloaded from on-line or domestically, it is going to be very simple to create the dataset. I feel PyTorch has good documentation on this, so I can be temporary.
If you already know the dataset is both from PyTorch or PyTorch-compatible, merely name the required imports and the dataset of selection:
from torch.utils.information import Dataset
from torchvision import datasets
from torchvision.transforms imports ToTensorinformation = torchvision.datasets.CIFAR10('path', practice=True, remodel=ToTensor())
Every dataset can have distinctive arguments to cross into it (discovered here). Generally, it is going to be the trail the dataset is saved at, a boolean indicating if it must be downloaded or not (conveniently known as obtain), whether or not it’s coaching or testing, and if transforms should be utilized.
I dropped in that transforms may be utilized to a dataset on the finish of the final part, however what really is a remodel?
A remodel is a technique of manipulating information for preprocessing a picture. There are a lot of completely different sides to transforms. The commonest remodel, ToTensor(), will convert the dataset to tensors (wanted to enter into any mannequin). Different transforms constructed into PyTorch (torchvision.transforms) embrace flipping, rotating, cropping, normalizing, and shifting photos. These are sometimes used so the mannequin can generalize higher and doesn’t overfit to the coaching information. Information augmentations may also be used to artificially enhance the scale of the dataset if wanted.
Beware most torchvision transforms solely settle for Pillow picture or tensor codecs (not numpy). To transform, merely use
To transform from numpy, both create a torch tensor or use the next:
From PIL import Picture
# assume arr is a numpy array
# you might have to normalize and forged arr to np.uint8 relying on format
img = Picture.fromarray(arr)
Transforms may be utilized concurrently utilizing torchvision.transforms.compose. You possibly can mix as many transforms as wanted for the dataset. An instance is proven beneath:
import torchvision.transforms.Composedataset_transform = transforms.Compose([
transforms.RandomResizedCrop(256),
transforms.RandomHorizontalFlip(),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])
])
Be sure you cross the saved remodel as an argument into the dataset for it to be utilized within the dataloader.
Generally of growing your personal mannequin, you will have a customized dataset. A typical use case can be switch studying to use your personal dataset on a pretrained mannequin.
There are 3 required elements to a PyTorch dataset class: initialization, size, and retrieving a component.
__init__: To initialize the dataset, cross within the uncooked and labeled information. One of the best apply is to cross within the uncooked picture information and labeled information individually.
__len__: Return the size of the dataset. Earlier than creating the dataset, the uncooked and labeled information needs to be checked to be the identical measurement.
__getitem__: That is the place all the info dealing with happens to return a given index (idx) of the uncooked and labeled information. If any transforms should be utilized, the info have to be transformed to a tensor and remodeled. If the initialization contained a path to the dataset, the trail have to be opened and information accessed/preprocessed earlier than it may be returned.
Instance dataset for a semantic segmentation mannequin:
from torch.utils.information import Dataset
from torchvision import transformsclass ExampleDataset(Dataset):
"""Instance dataset"""
def __init__(self, raw_img, data_mask, remodel=None):
self.raw_img = raw_img
self.data_mask = data_mask
self.remodel = remodel
def __len__(self):
return len(self.raw_img)
def __getitem__(self, idx):
if torch.is_tensor(idx):
idx = idx.tolist()
picture = self.raw_img[idx]
masks = self.data_mask[idx]
pattern = {'picture': picture, 'masks': masks}
if self.remodel:
pattern = self.remodel(pattern)
return pattern