Data extraction with LLMs utilizing Amazon SageMaker JumpStart
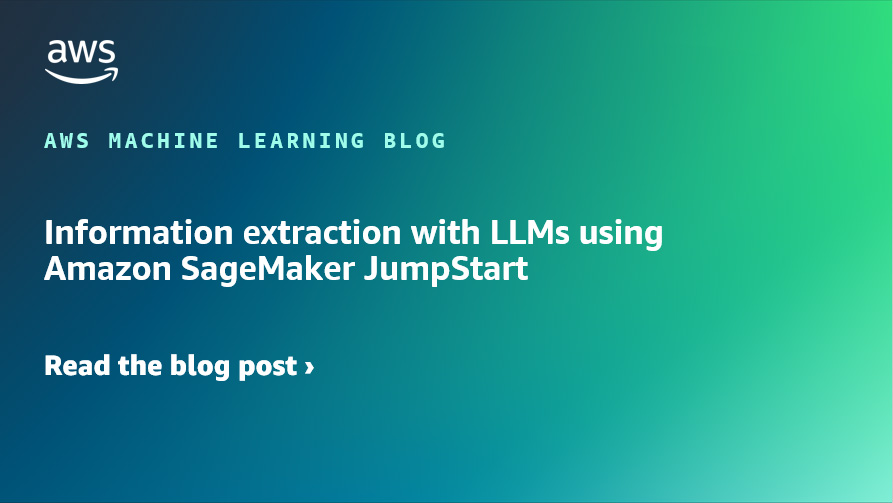
Giant language fashions (LLMs) have unlocked new prospects for extracting info from unstructured textual content knowledge. Though a lot of the present pleasure is round LLMs for generative AI duties, lots of the key use instances that you simply would possibly need to resolve haven’t basically modified. Duties similar to routing help tickets, recognizing clients intents from a chatbot dialog session, extracting key entities from contracts, invoices, and different kind of paperwork, in addition to analyzing buyer suggestions are examples of long-standing wants.
What makes LLMs so transformative, nevertheless, is their skill to attain state-of-the-art outcomes on these frequent duties with minimal knowledge and easy prompting, and their skill to multitask. Moderately than requiring intensive characteristic engineering and dataset labeling, LLMs will be fine-tuned on small quantities of domain-specific knowledge to rapidly adapt to new use instances. By dealing with many of the heavy lifting, providers like Amazon SageMaker JumpStart take away the complexity of fine-tuning and deploying these fashions.
SageMaker JumpStart is a machine studying (ML) hub with basis fashions (FMs), built-in algorithms, and prebuilt ML options which you could deploy with just some clicks. With SageMaker JumpStart, you’ll be able to consider, examine, and choose FMs rapidly based mostly on predefined high quality and duty metrics to carry out duties like article summarization and picture era.
This submit walks by means of examples of constructing info extraction use instances by combining LLMs with immediate engineering and frameworks similar to LangChain. We additionally study the uplift from fine-tuning an LLM for a particular extractive process. Whether or not you’re seeking to classify paperwork, extract key phrases, detect and redact personally identifiable info (PIIs), or parse semantic relationships, you can begin ideating your use case and use LLMs to your pure language processing (NLP).
Immediate engineering
Immediate engineering lets you instruct LLMs to generate options, explanations, or completions of textual content in an interactive means. Immediate engineering depends on massive pretrained language fashions which have been skilled on huge quantities of textual content knowledge. At first look, there may not be one finest technique to design a immediate, and completely different LLMs would possibly work higher or worse with completely different prompts. Subsequently, prompts are sometimes iteratively refined by means of trial and error to provide higher outcomes. As a place to begin, you’ll be able to check with the mannequin documentation which usually consists of suggestions and finest practices for prompting the mannequin, and examples offered in SageMaker JumpStart.
Within the following sections, we give attention to the immediate engineering strategies required for extractive use instances. They assist unlock the facility of LLMs by offering useful constraints and information the mannequin towards its supposed habits. We talk about the next use instances:
- Delicate info detection and redaction
- Entity extraction; generic and particular entities with structured codecs
- Classification, utilizing immediate engineering and fine-tuning
Earlier than we discover these use instances, we have to arrange our growth surroundings.
Conditions
The supply code accompanying this instance is offered on this GitHub repo. It consists of a number of Jupyter notebooks and a utils.py
module. The utils.py module homes the shared code that’s used all through the notebooks.
The only technique to run this instance is by utilizing Amazon SageMaker Studio with the Information Science 3.0
kernel or an Amazon SageMaker pocket book occasion with the conda_python3
kernel. For the occasion kind, you’ll be able to select the default settings.
On this instance, we use ml.g5.2xlarge
and ml.g5.48xlarge
situations for endpoint utilization, and ml.g5.24xlarge
for coaching job utilization. Use the Service Quotas console to ensure you have adequate quotas for these situations within the Area the place you’re working this instance.
We use Jupyter notebooks all through this submit. Earlier than we discover the examples, it’s essential to verify that you’ve the newest model of the SageMaker Python SDK. This SDK presents a user-friendly interface for coaching and deploying fashions on SageMaker. To put in or improve to the newest model, run the next command within the first cell of your Jupyter pocket book:
%pip set up --quiet --upgrade sagemaker
Deploy Llama-2-70b-chat utilizing SageMaker JumpStart
There are numerous LLMs accessible in SageMaker JumpStart to select from. On this instance, we use Llama-2-70b-chat, however you would possibly use a unique mannequin relying in your use case. To discover the listing of SageMaker JumpStart fashions, see JumpStart Available Model Table.
To deploy a mannequin from SageMaker JumpStart, you need to use both APIs, as demonstrated on this submit, or use the SageMaker Studio UI. After the mannequin is deployed, you’ll be able to take a look at it by asking a query from the mannequin:
from sagemaker.jumpstart.mannequin import JumpStartModel
model_id, model_version = "meta-textgeneration-llama-2-70b-f", "2.*"
endpoint_name = model_id
instance_type = "ml.g5.48xlarge"
mannequin = JumpStartModel(
model_id=model_id, model_version=model_version, function=role_arn
)
predictor = mannequin.deploy(
endpoint_name=endpoint_name, instance_type=instance_type
)
If no instance_type
is offered, the SageMaker JumpStart SDK will choose the default kind. On this instance, you explicitly set the occasion kind to ml.g5.48xlarge
.
Delicate knowledge extraction and redaction
LLMs present promise for extracting delicate info for redaction. This consists of strategies similar to immediate engineering, which incorporates priming the mannequin to grasp the redaction process, and by offering examples that may enhance the efficiency. For instance, priming the mannequin by stating “redact delicate info” and demonstrating a couple of examples of redacting names, dates, and places will help the LLM infer the foundations of the duty.
Extra in-depth types of priming the mannequin embrace offering constructive and destructive examples, demonstrations of frequent errors, and in-context studying to show the nuances of correct redaction. With cautious immediate design, LLMs can be taught to redact info whereas sustaining readability and utility of the doc. In real-life functions, nevertheless, further analysis is commonly mandatory to enhance the reliability and security of LLMs for dealing with confidential knowledge. That is typically achieved by means of the inclusion of human overview, as a result of no automated method is fully foolproof.
The next are a couple of examples of utilizing immediate engineering for the extraction and redaction of PII. The immediate consists of a number of components: the report_sample
, which incorporates the textual content that you simply need to determine and masks the PII knowledge inside, and directions (or steering) handed on to the mannequin because the system
message.
report_sample = """
This month at AnyCompany, we now have seen a major surge in orders from a various clientele. On November fifth, 2023, buyer Alice from US positioned an order with whole of $2190. Following her, on Nov seventh, Bob from UK ordered a bulk set of twenty-five ergonomic keyboards for his workplace setup with whole of $1000. The pattern continued with Jane from Australia, who on Nov twelfth requested a cargo of ten high-definition screens with whole of $9000, emphasizing the necessity for environmentally pleasant packaging. On the final day of that month, buyer John, positioned in Singapore, finalized an order for fifteen USB-C docking stations, aiming to equip his design studio with the newest expertise for whole of $3600.
"""
system = """
Your process is to exactly determine Personally Identifiable Data (PII) and identifiable particulars, together with title, handle, and the particular person's nation, within the offered textual content. Change these particulars with precisely 4 asterisks (****) because the masking characters. Use '****' for masking textual content of any size. Solely write the masked textual content within the response.
"""
Within the following instance, you outline the llama2_chat
perform that encapsulates sending the immediate to the Llama-2 mannequin. You reuse this perform all through the examples.
def llama2_chat(
predictor,
person,
temperature=0.1,
max_tokens=512,
top_p=0.9,
system=None,
):
"""Constructs the payload for the llama2 mannequin, sends it to the endpoint,
and returns the response."""
inputs = []
if system:
inputs.append({"function": "system", "content material": system})
if person:
inputs.append({"function": "person", "content material": person})
payload = {
"inputs": [inputs],
"parameters": {
"max_new_tokens": max_tokens,
"top_p": top_p,
"temperature": temperature,
},
}
response = predictor.predict(payload, custom_attributes="accept_eula=true")
return response
Use the next code to name the perform, passing your parameters:
response = utils.llama2_chat(
predictor,
system=system,
person=report_sample,
)
print(utils.llama2_parse_output(response))
You get the next output:
Entity extraction
Entity extraction is the method of figuring out and extracting key info entities from unstructured textual content. This system helps create structured knowledge from unstructured textual content and offers helpful contextual info for a lot of downstream NLP duties. Widespread functions for entity extraction embrace constructing a information base, extracting metadata to make use of for personalization or search, and enhancing person inputs and dialog understanding inside chatbots.
You’ll be able to successfully use LLMs for entity extraction duties by means of cautious immediate engineering. With a couple of examples of extracting entities from textual content, explanatory prompts, and the specified output format, the mannequin can be taught to determine and extract entities similar to folks, organizations, and places from new enter texts. Within the following examples, we exhibit a couple of completely different entity extraction duties starting from less complicated to extra advanced utilizing immediate engineering with the Llama-2-70b-chat mannequin you deployed earlier.
Extract generic entities
Use the next code to extract particular entities:
email_sample = "Howdy, My title is John. Your AnyCompany Monetary Providers, LLC bank card account 1111-0000-1111-0008 has a minimal cost of $24.53 that's due by July thirty first. Primarily based in your autopay settings, we are going to withdraw your cost on the due date out of your checking account quantity XXXXXX1111 with the routing quantity XXXXX0000. Buyer suggestions for Sunshine Spa, 123 Most important St, Anyplace. Ship feedback to Alice at alice_aa@anycompany.com and Bob at bob_bb@anycompany.com. I loved visiting the spa. It was very snug but it surely was additionally very costly. The facilities have been okay however the service made the spa an awesome expertise."
system = """
Your process is to exactly determine any e-mail addresses from the given textual content after which write them, one per line. Keep in mind to ONLY write an e-mail handle if it is exactly spelled out within the enter textual content. If there are not any e-mail addresses within the textual content, write "N/A". DO NOT write anything.
"""
consequence = utils.llama2_chat(predictor, system=system, person=email_sample)
print(utils.llama2_parse_output(consequence))
You get the next output:
alice_aa@anycompany.com
bob_bb@anycompany.com
Extract particular entities in a structured format
Utilizing the earlier pattern report, you’ll be able to extract extra advanced info in a structured method. This time, you present a JSON template for the mannequin to make use of and return the output in JSON format.
With LLMs producing JSON paperwork as output, you’ll be able to effortlessly parse them into a spread of different knowledge buildings. This permits easy conversions to dictionaries, YAML, and even Pydantic fashions utilizing third-party libraries, similar to LangChain’s PydanticOutputParser. You’ll be able to see the implementation within the GitHub repo.
import json
system = """
Your process is to exactly extract info from the textual content offered, and format it based on the given JSON schema delimited with triple backticks. Solely embrace the JSON output in your response. If a particular discipline has no accessible knowledge, point out this by writing `null` as the worth for that discipline within the output JSON. In instances the place there is no such thing as a knowledge accessible in any respect, return an empty JSON object. Keep away from together with every other statements within the response.
```
{json_schema}
```
"""
json_schema = """
{
"orders":
[
{
"name": "<customer_name>",
"location": "<customer_location>",
"order_date": "<order_date in format YYYY-MM-DD>",
"order_total": "<order_total>",
"order_items": [
{
"item_name": "<item_name>",
"item_quantity": "<item_quantity>"
}
]
}
]
}
"""
response = utils.llama2_chat(
predictor,
system=system.format(json_schema=json_schema),
person=report_sample,
)
json_str = utils.llama2_parse_output(response)
print(json_str)
You get the next output:
{
"orders": [
{
"name": "Alice",
"location": "US",
"order_date": "2023-11-05",
"order_total": 2190,
"order_items": [
{
"item_name": null,
"item_quantity": null
}
]
},
{
"title": "Bob",
"location": "UK",
"order_date": "2023-11-07",
"order_total": 1000,
"order_items": [
{
"item_name": "ergonomic keyboards",
"item_quantity": 25
}
]
},
{
"title": "Jane",
"location": "Australia",
"order_date": "2023-11-12",
"order_total": 9000,
"order_items": [
{
"item_name": "high-definition monitors",
"item_quantity": 10
}
]
},
{
"title": "John",
"location": "Singapore",
"order_date": "2023-11-30",
"order_total": 3600,
"order_items": [
{
"item_name": "USB-C docking stations",
"item_quantity": 15
}
]
}
]
}
Classification utilizing immediate engineering
LLMS generally is a useful gizmo for info extraction duties similar to textual content classification. Widespread functions embrace classifying the intents of person interactions through channels similar to e-mail, chatbots, voice, and others, or categorizing paperwork to route their requests to downstream methods. The preliminary step includes figuring out the intent or class of the person’s request or the doc. These intents or lessons may take many types—from quick single phrases to hundreds of hierarchical lessons and sub-classes.
Within the following examples, we exhibit immediate engineering on artificial dialog knowledge to extract intents. Moreover, we present how pre-trained fashions will be assessed to find out if fine-tuning is required.
Let’s begin with the next instance. You’ve got a listing of buyer interactions with an imaginary well being and life insurance coverage firm. To start out, use the Llama-2-70b-chat mannequin you deployed within the earlier part:
inference_instance_type = "ml.g5.48xlarge"
# Llama-2-70b chat
model_id, model_version = "meta-textgeneration-llama-2-70b-f", "2.*"
endpoint_name = model_id
predictor = utils.get_predictor(
endpoint_name=endpoint_name,
model_id=model_id,
model_version=model_version,
inference_instance_type=inference_instance_type,
)
The get_predictor
perform is a helper perform that creates a predictor object from a mannequin ID and model. If the required endpoint doesn’t exist, it creates a brand new endpoint and deploy the mannequin. If the endpoint already exists, it makes use of the present endpoint.
customer_interactions = [
"""Hello, I've recently moved to a new state and I need to update my address for my health insurance policy.
Can you assist me with that?
""",
"""Good afternoon! I'm interested in adding dental coverage to my existing health plan.
Could you provide me the options and prices?
""",
"""I had a disappointing experience with the customer service yesterday regarding my claim.
I want to file a formal complaint and speak with a supervisor.
""",
]
system = """
Your process is to determine the client intent from their interactions with help bot within the offered textual content. The intent output should no more than 4 phrases. If the intent just isn't clear, please present a fallback intent of "unknown".
"""
def get_intent(system, customer_interactions):
for customer_interaction in customer_interactions:
response = utils.llama2_chat(
predictor,
system=system,
person=customer_interaction,
)
content material = utils.llama2_parse_output(response)
print(content material)
get_intent(system, customer_interactions)
You get the next output:
Replace Handle
Intent: Informational
Intent: Escalate difficulty
Trying on the output, these appear affordable because the intents. Nonetheless, the format and elegance of the intents can fluctuate relying on the language mannequin. One other limitation of this method is that intents should not confined to a predefined listing, which implies the language mannequin would possibly generate and phrase the intents in another way every time you run it.
To deal with this, you need to use the in-context studying approach in immediate engineering to steer the mannequin in the direction of choosing from a predefined set of intents, or class labels, that you simply present. Within the following instance, alongside the client dialog, you embrace a listing of potential intents and ask the mannequin to select from this listing:
system = """
Your process is to determine the intent from the client interplay with the help bot. Choose from the intents offered within the following listing delimited with ####. If the intent just isn't clear, please present a fallback intent of "unknown". ONLY write the intent.
####
- info change
- add protection
- criticism
- portal navigation
- free product improve
####
"""
get_intent(system, customer_interactions)
You get the next output:
info change
add protection
criticism
Reviewing the outcomes, it’s evident that the language mannequin performs properly in choosing the suitable intent within the desired format.
Sub-intents and intent bushes
In case you make the previous situation extra advanced, as in lots of real-life use instances, intents will be designed in numerous classes and likewise in a hierarchical vogue, which can make the classification duties tougher for the mannequin. Subsequently, you’ll be able to additional enhance and modify your immediate to supply an instance to the mannequin, also called n-shot studying, k-shot studying, or few-shot studying.
The next is the intent tree to make use of on this instance. You will discover its supply code within the utils.py
file within the code repository.
INTENTS = [
{
"main_intent": "profile_update",
"sub_intents": [
"contact_info",
"payment_info",
"members",
],
},
{
"main_intent": "health_cover",
"sub_intents": [
"add_extras",
"add_hospital",
"remove_extras",
"remove_hospital",
"new_policy",
"cancel_policy",
],
},
{
"main_intent": "life_cover",
"sub_intents": [
"new_policy",
"cancel_policy",
"beneficiary_info",
],
},
{
"main_intent": "customer_retention",
"sub_intents": [
"complaint",
"escalation",
"free_product_upgrade",
],
},
{
"main_intent": "technical_support",
"sub_intents": [
"portal_navigation",
"login_issues",
],
},
]
Utilizing the next immediate (which incorporates the intents), you’ll be able to ask the mannequin to choose from the offered listing of intents:
system = """
Your process is to determine the intent from the client interplay with the help bot. Determine the intent of the offered textual content utilizing the listing of offered intent tree delimited with ####. The intents are outlined in lessons and sub-classes. Write the intention with this format: <main-intent>:<sub-intent>. ONLY write the intent.
OUTPUT EXAMPLE:
profile_update:contact_info
OUTPUT EXAMPLE:
customer_retention:criticism
####
{intents}
####
"""
intents_json = json.dumps(utils.INTENTS, indent=4)
system = system.format(intents=intents_json)
get_intent(system, customer_interactions)
You get the next output:
profile_update:contact_info
health_cover:add_extras
customer_retention:criticism
Though LLMs can typically accurately determine intent from a listing of attainable intents, they could typically produce further outputs or fail to stick to the precise intent construction and output schema. There are additionally eventualities the place intents should not as simple as they initially appear or are extremely particular to a enterprise area context that the mannequin doesn’t absolutely comprehend.
For example, within the following pattern interplay, the client in the end needs to vary their protection, however their fast query and interplay intent is to get assist with portal navigation. Equally, within the second interplay, the extra acceptable intent is “free product improve” which the client is requesting. Nonetheless, the mannequin is unable to detect these nuanced intents as precisely as desired.
customer_interactions = [
"I want to change my coverage plan. But I'm not seeing where to do this on the online website. Could you please point me to it?",
"I'm unhappy with the current benefits of my plan and I'm considering canceling unless there are better alternatives. What can you offer?",
]
get_intent(system, customer_interactions)
You get the next output:
profile_update:contact_info
customer_retention:criticism
Immediate engineering can typically efficiently extract particular intents from textual content. Nonetheless, for some use instances, relying solely on immediate engineering has limitations. Situations the place further strategies past immediate engineering could also be wanted embrace:
- Conversations with numerous intent lessons or lengthy contexts that exceed the language mannequin’s context window dimension, or making queries extra computationally costly
- Desired outputs in particular codecs that the mannequin struggles to undertake
- Enhancing mannequin understanding of the area or process to spice up efficiency
Within the following part, we exhibit how fine-tuning can increase the accuracy of the LLM for the intent classification process tried earlier.
Fantastic-tuning an LLM for classification
The next sections element the fine-tuning strategy of the FlanT5-XL and Mistral 7B mannequin utilizing SageMaker JumpStart. We use the FlanT5-XL and Mistral 7B fashions to check their accuracy. Each fashions are considerably smaller in comparison with the Llama-2-70b-Chat. The purpose is to find out whether or not smaller fashions can obtain state-of-the-art efficiency on particular duties after they’re fine-tuned.
We have now fine-tuned each Mitral 7B and FlanT5-XL fashions. You’ll be able to see the small print of the Mistral 7b fine-tuning within the code repository. Within the following, we define the steps for fine-tuning and evaluating of FlanT5-XL.
Initially, you deploy (or reuse) the FlanT5 endpoint because the base_predictor
, which represents the bottom mannequin previous to any fine-tuning. Subsequently, you assess the efficiency of the fashions by evaluating them after the fine-tuning course of.
inference_instance_type = "ml.g5.2xlarge"
model_id , model_version= "huggingface-text2text-flan-t5-xl", "2.0.0"
base_endpoint_name = model_id
base_predictor = utils.get_predictor(
endpoint_name=base_endpoint_name,
model_id=model_id,
model_version=model_version,
inference_instance_type=inference_instance_type,
)
Put together coaching knowledge for fine-tuning
Getting ready for fine-tuning requires organizing a number of information, together with the dataset and template information. The dataset is structured to align with the required enter format for fine-tuning. For instance, every report in our coaching dataset adheres to the next construction:
{"question": "buyer question", "response": "main-intent:sub-intent"}
On this instance, you utilize a synthesized dataset comprising buyer interactions with a fictional insurance coverage firm. To be taught extra concerning the knowledge and achieve entry to it, check with the source code.
intent_dataset_file = "knowledge/intent_dataset.jsonl"
intent_dataset_train_file = "knowledge/intent_dataset_train.jsonl"
intent_dataset_test_file = "knowledge/intent_dataset_test.jsonl"
ft_template_file = "knowledge/template.json"
The next is the immediate for fine-tuning. The immediate has the question
parameter, which is ready through the fine-tuning utilizing the SageMaker JumpStart SDK.
FT_PROMPT = """Determine the intent lessons from the given person question, delimited with ####. Intents are categorized into two ranges: important intent and sub intent. In your response, present solely ONE set of important and sub intents that's most related to the question. Write your response ONLY on this format <main-intent>:<sub-intent>. ONLY Write the intention.
OUTPUT EXAMPLE:
profile_update:contact_info
OUTPUT EXAMPLE:
technical_support:portal_navigation
#### QUERY:
{question}
####
"""
The next creates a template file that will probably be utilized by the SageMaker JumpStart framework to fine-tune the mannequin. The template has two fields, immediate
and completion
. These fields are used to go labeled knowledge to the mannequin for the fine-tuning course of.
template = {
"immediate": utils.FT_PROMPT,
"completion": "{response}",
}
with open(ft_template_file, "w") as f:
json.dump(template, f)
The coaching knowledge is uploaded to an Amazon Simple Storage Service (Amazon S3) bucket, setting the stage for the precise fine-tuning course of.
train_data_location = utils.upload_train_and_template_to_s3(
bucket_prefix="intent_dataset_flant5",
train_path=intent_dataset_train_file,
template_path=ft_template_file,
)
Fantastic-tune the mannequin
Configure the JumpStartEstimator
, specifying your chosen mannequin and different parameters like occasion kind and hyperparameters (on this instance, you utilize 5 epochs for the coaching). This estimator drives the fine-tuning course of.
from sagemaker.jumpstart.estimator import JumpStartEstimator
estimator = JumpStartEstimator(
model_id=model_id,
disable_output_compression=True,
instance_type="ml.g5.24xlarge",
function=utils.get_role_arn(),
)
estimator.set_hyperparameters(
instruction_tuned="True", epochs="5", max_input_length="1024"
)
estimator.match({"coaching": train_data_location})
Deploy the fine-tuned mannequin
After fine-tuning, deploy the fine-tuned mannequin:
finetuned_endpoint_name = "flan-t5-xl-ft-infoext"
finetuned_model_name = finetuned_endpoint_name
# Deploying the finetuned mannequin to an endpoint
finetuned_predictor = estimator.deploy(
endpoint_name=finetuned_endpoint_name,
model_name=finetuned_model_name,
)
Use the next code to check the fine-tuned mannequin towards its base mannequin with ambiguous queries, which you noticed within the earlier part:
ambiguous_queries = [
{
"query": "I want to change my coverage plan. But I'm not seeing where to do this on the online site. Could you please show me how?",
"main_intent": "techincal_support",
"sub_intent": "portal_navigation",
},
{
"query": "I'm unhappy with the current benefits of my plan and I'm considering canceling unless there are better alternatives. What can you offer?",
"main_intent": "customer_retention",
"sub_intent": "free_product_upgrade",
},
]
for question in ambiguous_queries:
query = question["query"]
print("question:", query, "n")
print(
"anticipated intent: ", f"{question['main_intent']}:{question['sub_intent']}"
)
immediate = utils.FT_PROMPT.format(question=query)
response = utils.flant5(base_predictor, person=immediate, max_tokens=13)
print("base mannequin: ", utils.parse_output(response))
response = utils.flant5(finetuned_predictor, person=immediate, max_tokens=13)
print("finetuned mannequin: ", utils.parse_output(response))
print("-" * 80)
You get the next output:
question: I need to change my protection plan. However I am not seeing the place to do that on the net web site. Might you please present me how?
anticipated intent: techincal_support:portal_navigation
base mannequin: main_intent>:sub_intent> change
finetuned mannequin: technical_support:portal_navigation
--------------------------------------------------------------------------------
question: I am sad with the present advantages of my plan and I am contemplating canceling until there are higher alternate options. What are you able to supply?
anticipated intent: customer_retention:free_product_upgrade
base mannequin: main_intent>:sub_intent> cancel
finetuned mannequin: customer_retention:free_product_upgrade
--------------------------------------------------------------------------------
As proven on this instance, the fine-tuned mannequin is ready to classify the ambiguous queries accurately.
In evaluations, fine-tuned fashions carried out higher in figuring out the proper class for each clear and ambiguous intents. The next part particulars the benchmark’s efficiency total, and towards every intent.
Efficiency comparisons and issues
On this part, we now have gathered the analysis outcomes and efficiency benchmarks for every mannequin, earlier than and after fine-tuning, in addition to a comparability between the immediate engineering and fine-tuning the LLM. The dataset consists of seven,824 examples, with a 90% break up for coaching (together with validation) and 10% for testing.
Mannequin | General Accuracy | Fantastic-tuning Period (minutes) | Notes |
Mistral-7b (fine-tuned 5 epochs, with out lessons within the immediate) | 98.97% | 720 |
Given Mistral-7b’s nature as a textual content era mannequin, parsing its output to extract intent will be difficult as a result of tendencies for character repetition and era of further characters. Improved efficiency with extra epochs: 98% accuracy for 5 epochs in comparison with 92% for one epoch. |
Flan-T5-XL (fine-tuned one epochs, with out lessons within the immediate) | 98.46% | 150 | Marginal enchancment in accuracy with elevated epochs: from 97.5% (one epoch) to 98.46% (5 epochs). |
Llama-2-70b-chat (With lessons within the immediate) | 78.42% | N/A | Low accuracy in ambiguous eventualities. |
Llama-2-70b-chat (With out lessons within the immediate) | 10.85% | N/A | . |
Flan-T5-XL (base mannequin, with out lessons within the immediate) | 0.0% | N/A | Unable to determine any of the intent lessons with the anticipated format. |
Mistral-7b (base mannequin, with out lessons within the immediate) | 0.0% | N/A | Unable to determine any of the intent lessons with the anticipated format. |
The next desk incorporates a breakdown of fashions’ accuracy for every intent class.
Most important Intent | Sub-intent | Instance Depend | Llama2-70b (with out lessons in immediate) | Llama2-70b (with lessons in immediate) | Flant5-XL Fantastic-tuned |
Mistral-7b Fantastic-tuned |
Buyer Retention | Grievance | 63 | 7.94% | 44.44% | 98.41% | 98.41% |
Buyer Retention | Escalation | 49 | 91.84% | 100% | 100% | 100% |
Buyer Retention | Free Product Improve | 50 | 0.00% | 64.00% | 100% | 100% |
Well being Cowl | Add Extras | 38 | 0.00% | 100% | 97.37% | 100% |
Well being Cowl | Add Hospital | 44 | 0.00% | 81.82% | 100% | 97.73% |
Well being Cowl | Cancel Coverage | 43 | 0.00% | 100% | 100% | 97.67% |
Well being Cowl | New Coverage | 41 | 0.00% | 82.93% | 100% | 100% |
Well being Cowl | Take away Extras | 47 | 0.00% | 85.11% | 100% | 100% |
Well being Cowl | Take away Hospital | 53 | 0.00% | 84.90% | 100% | 100% |
Life Cowl | Beneficiary Data | 45 | 0.00% | 100% | 97.78% | 97.78% |
Life Cowl | Cancel Coverage | 47 | 0.00% | 55.32% | 100% | 100% |
Life Cowl | New Coverage | 40 | 0.00% | 90.00% | 92.50% | 100% |
Profile Replace | Contact Data | 45 | 35.56% | 95.56% | 95.56% | 95.56% |
Profile Replace | Members | 52 | 0.00% | 36.54% | 98.08% | 98.08% |
Profile Replace | Fee Data | 47 | 40.43% | 97.87% | 100% | 100% |
Technical Help | Login Points | 39 | 0.00% | 92.31% | 97.44% | 100% |
Technical Help | Portal Navigation | 40 | 0.00% | 45.00% | 95.00% | 97.50% |
This comparative evaluation illustrates the trade-offs between fine-tuning time and mannequin accuracy. It highlights the flexibility of fashions like Mistral-7b and FlanT5-XL to attain greater classification accuracy by means of fine-tuning. Moreover, it reveals how smaller fashions can match or surpass the efficiency of bigger fashions on particular duties when fine-tuned, contrasted with utilizing immediate engineering alone on the bigger fashions.
Clear up
Full the next steps to scrub up your sources:
- Delete the SageMaker endpoints, configuration, and fashions.
- Delete the S3 bucket created for this instance.
- Delete the SageMaker pocket book occasion (in case you used one to run this instance).
Abstract
Giant language fashions have revolutionized info extraction from unstructured textual content knowledge. These fashions excel in duties similar to classifying info and extracting key entities from varied paperwork, reaching state-of-the-art outcomes with minimal knowledge.
This submit demonstrated using massive language fashions for info extraction by means of immediate engineering and fine-tuning. Whereas efficient, relying solely on immediate engineering can have limitations for advanced duties that require inflexible output codecs or numerous lessons. In these eventualities, fine-tuning even smaller fashions on domain-specific knowledge can considerably enhance efficiency past what immediate engineering alone can obtain.
The submit included sensible examples highlighting how fine-tuned smaller fashions can surpass immediate engineering with bigger fashions for such advanced use instances. Though immediate engineering is an efficient start line for less complicated use instances, fine-tuning presents a extra strong answer for advanced info extraction duties, guaranteeing greater accuracy and adaptableness to particular use instances. SageMaker JumpStart instruments and providers facilitate this course of, making it accessible for people and groups throughout all ranges of ML experience.
Further studying
You’ll be able to learn extra on utilizing SageMaker JumpStart for clever doc processing, fine-tuning, and analysis of LLMs within the following sources:
In regards to the Authors
Pooya Vahidi is a Senior Options Architect at AWS, captivated with laptop science, synthetic intelligence, and cloud computing. As an AI skilled, he’s an energetic member of the AWS AI/ML Space-of-Depth staff. With a background spanning over 20 years of experience in main the structure and engineering of large-scale options, he helps clients on their transformative journeys by means of cloud and AI/ML applied sciences.
Dr. Romina Sharifpour is a Senior Machine Studying and Synthetic Intelligence Options Architect at Amazon Net Providers (AWS). She has spent over 10 years main the design and implementation of progressive end-to-end options enabled by developments in ML and AI. Romina’s areas of curiosity are pure language processing, massive language fashions, and MLOps.