In right this moment’s panorama of one-on-one buyer interactions for putting orders, the prevailing follow continues to depend on human attendants, even in settings like drive-thru espresso retailers and fast-food institutions. This conventional strategy poses a number of challenges: it closely depends upon handbook processes, struggles to effectively scale with rising buyer calls for, introduces the potential for human errors, and operates inside particular hours of availability. Moreover, in aggressive markets, companies adhering solely to handbook processes may discover it difficult to ship environment friendly and aggressive service. Regardless of technological developments, the human-centric mannequin stays deeply ingrained so as processing, main to those limitations.
The prospect of using know-how for one-on-one order processing help has been out there for a while. Nevertheless, current options can usually fall into two classes: rule-based methods that demand substantial effort and time for setup and maintenance, or inflexible methods that lack the flexibleness required for human-like interactions with clients. Consequently, companies and organizations face challenges in swiftly and effectively implementing such options. Happily, with the appearance of generative AI and large language models (LLMs), it’s now potential to create automated methods that may deal with pure language effectively, and with an accelerated on-ramping timeline.
Amazon Bedrock is a totally managed service that gives a selection of high-performing basis fashions (FMs) from main AI corporations like AI21 Labs, Anthropic, Cohere, Meta, Stability AI, and Amazon through a single API, together with a broad set of capabilities you’ll want to construct generative AI functions with safety, privateness, and accountable AI. Along with Amazon Bedrock, you need to use different AWS providers like Amazon SageMaker JumpStart and Amazon Lex to create totally automated and simply adaptable generative AI order processing brokers.
On this put up, we present you easy methods to construct a speech-capable order processing agent utilizing Amazon Lex, Amazon Bedrock, and AWS Lambda.
Resolution overview
The next diagram illustrates our resolution structure.
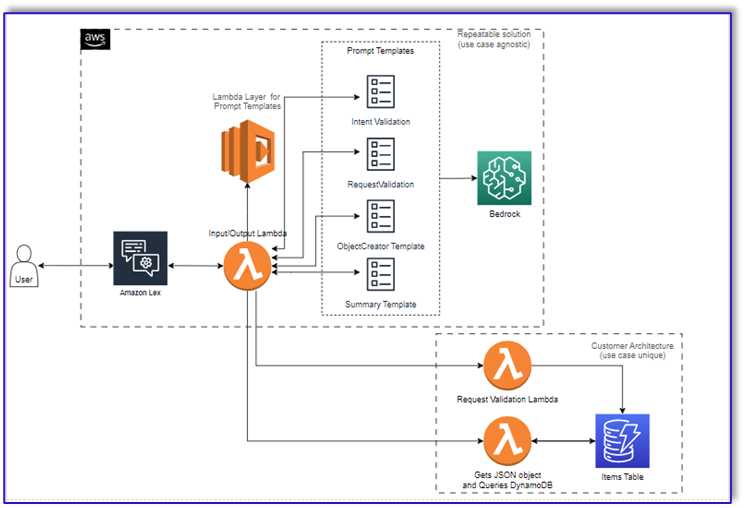
The workflow consists of the next steps:
- A buyer locations the order utilizing Amazon Lex.
- The Amazon Lex bot interprets the shopper’s intents and triggers a
DialogCodeHook
.
- A Lambda perform pulls the suitable immediate template from the Lambda layer and codecs mannequin prompts by including the shopper enter within the related immediate template.
- The
RequestValidation
immediate verifies the order with the menu merchandise and lets the shopper know through Amazon Lex if there’s one thing they need to order that isn’t a part of the menu and can present suggestions. The immediate additionally performs a preliminary validation for order completeness.
- The
ObjectCreator
immediate converts the pure language requests into an information construction (JSON format).
- The shopper validator Lambda perform verifies the required attributes for the order and confirms if all mandatory info is current to course of the order.
- A buyer Lambda perform takes the info construction as an enter for processing the order and passes the order whole again to the orchestrating Lambda perform.
- The orchestrating Lambda perform calls the Amazon Bedrock LLM endpoint to generate a closing order abstract together with the order whole from the shopper database system (for instance, Amazon DynamoDB).
- The order abstract is communicated again to the shopper through Amazon Lex. After the shopper confirms the order, the order can be processed.
Stipulations
This put up assumes that you’ve an energetic AWS account and familiarity with the next ideas and providers:
Additionally, as a way to entry Amazon Bedrock from the Lambda features, you’ll want to make sure that the Lambda runtime has the next libraries:
- boto3>=1.28.57
- awscli>=1.29.57
- botocore>=1.31.57
This may be completed with a Lambda layer or through the use of a particular AMI with the required libraries.
Moreover, these libraries are required when calling the Amazon Bedrock API from Amazon SageMaker Studio. This may be completed by working a cell with the next code:
%pip set up --no-build-isolation --force-reinstall
"boto3>=1.28.57"
"awscli>=1.29.57"
"botocore>=1.31.57"
Lastly, you create the next coverage and later connect it to any function accessing Amazon Bedrock:
{
"Model": "2012-10-17",
"Assertion": [
{
"Sid": "Statement1",
"Effect": "Allow",
"Action": "bedrock:*",
"Resource": "*"
}
]
}
Create a DynamoDB desk
In our particular state of affairs, we’ve created a DynamoDB desk as our buyer database system, however you can additionally use Amazon Relational Database Service (Amazon RDS). Full the next steps to provision your DynamoDB desk (or customise the settings as wanted to your use case):
- On the DynamoDB console, select Tables within the navigation pane.
- Select Create desk.
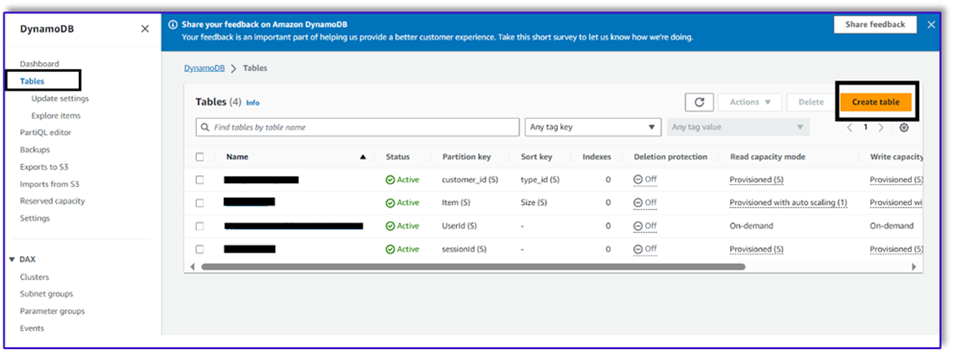
- For Desk title, enter a reputation (for instance,
ItemDetails
).
- For Partition key, enter a key (for this put up, we use
Merchandise
).
- For Type key, enter a key (for this put up, we use
Dimension
).
- Select Create desk.
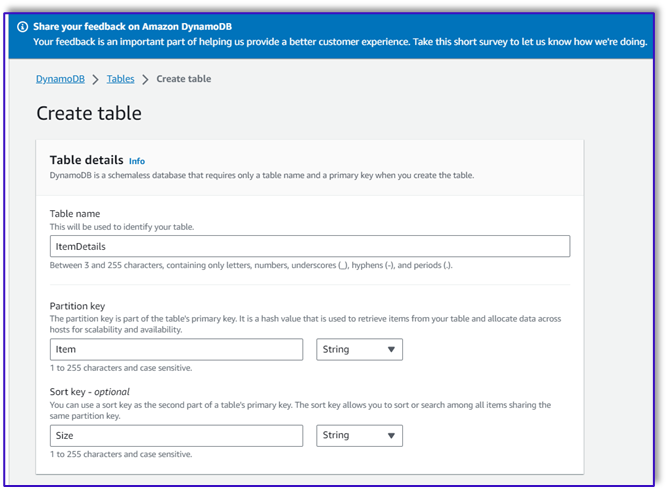
Now you possibly can load the info into the DynamoDB desk. For this put up, we use a CSV file. You’ll be able to load the info to the DynamoDB desk utilizing Python code in a SageMaker pocket book.
First, we have to arrange a profile named dev.
- Open a brand new terminal in SageMaker Studio and run the next command:
aws configure --profile dev
This command will immediate you to enter your AWS entry key ID, secret entry key, default AWS Area, and output format.
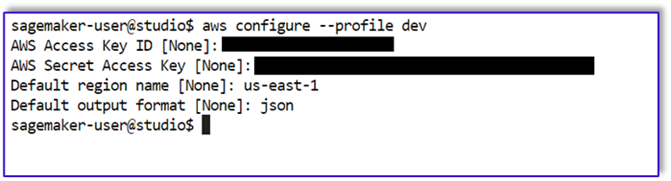
- Return to the SageMaker pocket book and write a Python code to arrange a connection to DynamoDB utilizing the Boto3 library in Python. This code snippet creates a session utilizing a particular AWS profile named dev after which creates a DynamoDB consumer utilizing that session. The next is the code pattern to load the info:
%pip set up boto3
import boto3
import csv
# Create a session utilizing a profile named 'dev'
session = boto3.Session(profile_name="dev")
# Create a DynamoDB useful resource utilizing the session
dynamodb = session.useful resource('dynamodb')
# Specify your DynamoDB desk title
table_name="your_table_name"
desk = dynamodb.Desk(table_name)
# Specify the trail to your CSV file
csv_file_path="path/to/your/file.csv"
# Learn CSV file and put gadgets into DynamoDB
with open(csv_file_path, 'r', encoding='utf-8-sig') as csvfile:
csvreader = csv.reader(csvfile)
# Skip the header row
subsequent(csvreader, None)
for row in csvreader:
# Extract values from the CSV row
merchandise = {
'Merchandise': row[0], # Modify the index based mostly in your CSV construction
'Dimension': row[1],
'Worth': row[2]
}
# Put merchandise into DynamoDB
response = desk.put_item(Merchandise=merchandise)
print(f"Merchandise added: {response}")
print(f"CSV information has been loaded into the DynamoDB desk: {table_name}")
Alternatively, you need to use NoSQL Workbench or different instruments to rapidly load the info to your DynamoDB desk.
The next is a screenshot after the pattern information is inserted into the desk.
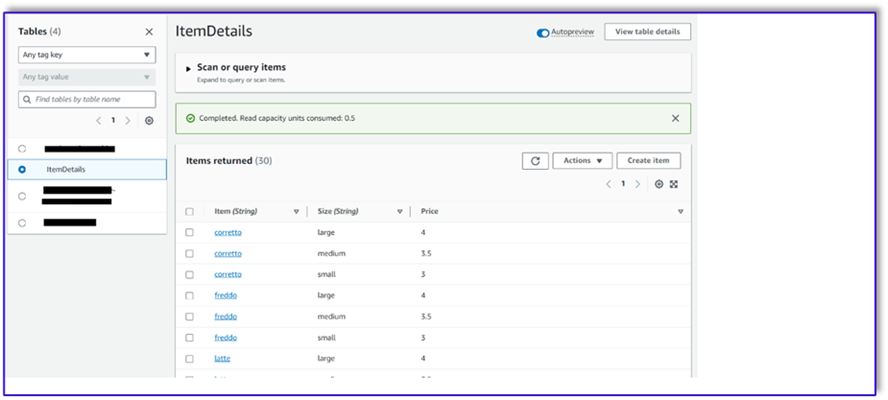
Create templates in a SageMaker pocket book utilizing the Amazon Bedrock invocation API
To create our immediate template for this use case, we use Amazon Bedrock. You’ll be able to entry Amazon Bedrock from the AWS Management Console and through API invocations. In our case, we entry Amazon Bedrock through API from the comfort of a SageMaker Studio pocket book to create not solely our immediate template, however our full API invocation code that we are able to later use on our Lambda perform.
- On the SageMaker console, entry an current SageMaker Studio area or create a brand new one to entry Amazon Bedrock from a SageMaker pocket book.
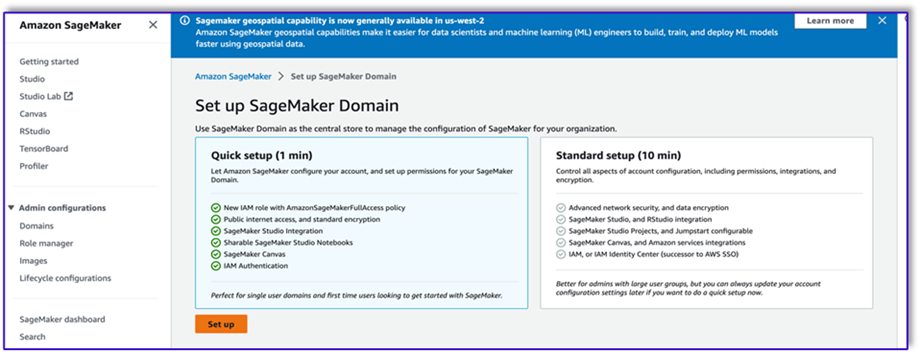
- After you create the SageMaker area and person, select the person and select Launch and Studio. It will open a JupyterLab atmosphere.
- When the JupyterLab atmosphere is prepared, open a brand new pocket book and start importing the required libraries.
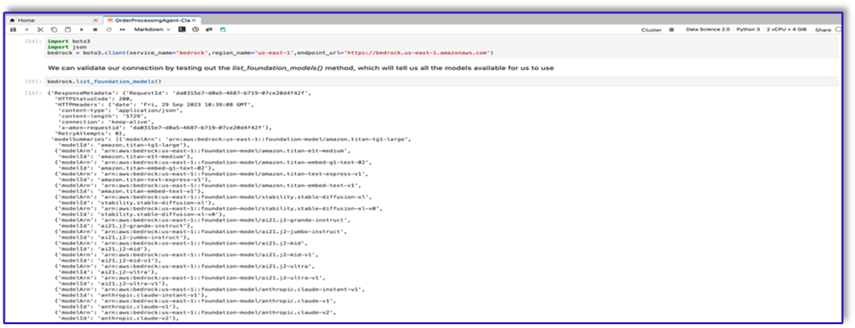
There are numerous FMs out there through the Amazon Bedrock Python SDK. On this case, we use Claude V2, a robust foundational mannequin developed by Anthropic.
The order processing agent wants just a few totally different templates. This will change relying on the use case, however we’ve got designed a basic workflow that may apply to a number of settings. For this use case, the Amazon Bedrock LLM template will accomplish the next:
- Validate the shopper intent
- Validate the request
- Create the order information construction
- Cross a abstract of the order to the shopper
- To invoke the mannequin, create a bedrock-runtime object from Boto3.

#Mannequin api request parameters
modelId = 'anthropic.claude-v2' # change this to make use of a special model from the mannequin supplier
settle for="utility/json"
contentType="utility/json"
import boto3
import json
bedrock = boto3.consumer(service_name="bedrock-runtime")
Let’s begin by engaged on the intent validator immediate template. That is an iterative course of, however due to Anthropic’s immediate engineering information, you possibly can rapidly create a immediate that may accomplish the duty.
- Create the primary immediate template together with a utility perform that can assist put together the physique for the API invocations.
The next is the code for prompt_template_intent_validator.txt:
"{"immediate": "Human: I provides you with some directions to finish my request.n<directions>Given the Dialog between Human and Assistant, you'll want to establish the intent that the human needs to perform and reply appropriately. The legitimate intents are: Greeting,Place Order, Complain, Communicate to Somebody. At all times put your response to the Human inside the Response tags. Additionally add an XML tag to your output figuring out the human intent.nHere are some examples:n<instance><Dialog> H: hello there.nnA: Hello, how can I assist you to right this moment?nnH: Sure. I would love a medium mocha please</Dialog>nnA:<intent>Place Order</intent><Response>nGot it.</Response></instance>n<instance><Dialog> H: heynnA: Hello, how can I assist you to right this moment?nnH: my espresso doesn't style effectively are you able to please re-make it?</Dialog>nnA:<intent>Complain</intent><Response>nOh, I'm sorry to listen to that. Let me get somebody that can assist you.</Response></instance>n<instance><Dialog> H: hellonnA: Hello, how can I assist you to right this moment?nnH: I want to communicate to another person please</Dialog>nnA:<intent>Communicate to Somebody</intent><Response>nSure, let me get somebody that can assist you.</Response></instance>n<instance><Dialog> H: howdynnA: Hello, how can I assist you to right this moment?nnH:can I get a big americano with sugar and a couple of mochas with no whipped cream</Dialog>nnA:<intent>Place Order</intent><Response>nSure factor! Please give me a second.</Response></instance>n<instance><Dialog> H: hellonn</Dialog>nnA:<intent>Greeting</intent><Response>nHi there, how can I assist you to right this moment?</Response></instance>n</directions>nnPlease full this request in response to the directions and examples supplied above:<request><Dialog>REPLACEME</Dialog></request>nnAssistant:n", "max_tokens_to_sample": 250, "temperature": 1, "top_k": 250, "top_p": 0.75, "stop_sequences": ["nnHuman:", "nnhuman:", "nnCustomer:", "nncustomer:"]}"
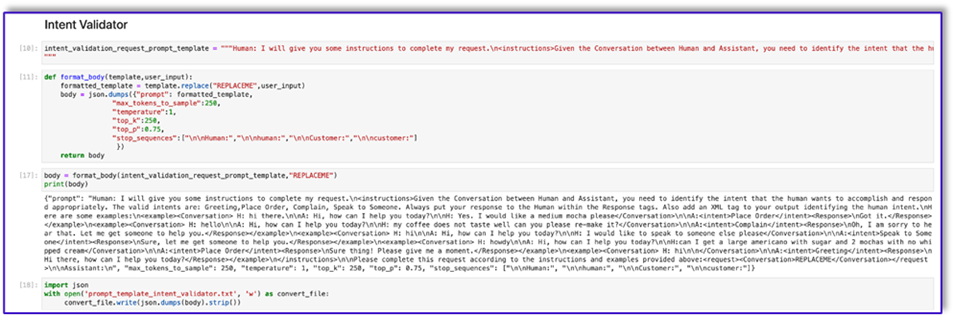
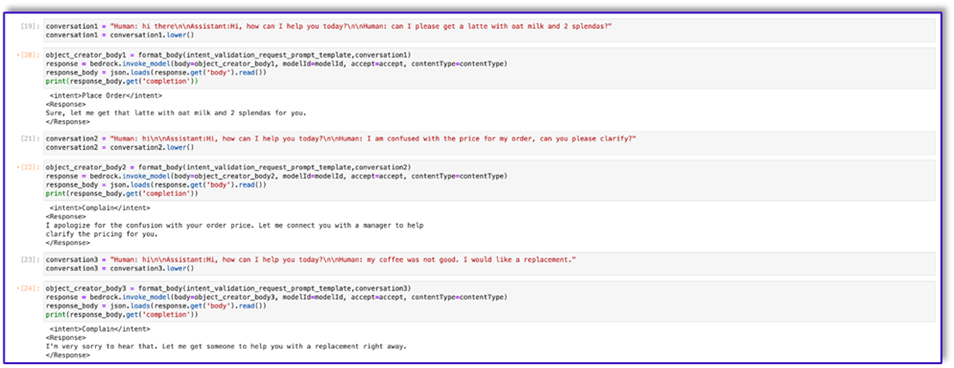
- Save this template right into a file as a way to add to Amazon S3 and name from the Lambda perform when wanted. Save the templates as JSON serialized strings in a textual content file. The earlier screenshot reveals the code pattern to perform this as effectively.
- Repeat the identical steps with the opposite templates.
The next are some screenshots of the opposite templates and the outcomes when calling Amazon Bedrock with a few of them.
The next is the code for prompt_template_request_validator.txt:
"{"immediate": "Human: I provides you with some directions to finish my request.n<directions>Given the context do the next steps: 1. confirm that the gadgets within the enter are legitimate. If buyer supplied an invalid merchandise, advocate changing it with a legitimate one. 2. confirm that the shopper has supplied all the data marked as required. If the shopper missed a required info, ask the shopper for that info. 3. When the order is full, present a abstract of the order and ask for affirmation at all times utilizing this phrase: 'is that this right?' 4. If the shopper confirms the order, Don't ask for affirmation once more, simply say the phrase contained in the brackets [Great, Give me a moment while I try to process your order]</directions>n<context>nThe VALID MENU ITEMS are: [latte, frappe, mocha, espresso, cappuccino, romano, americano].nThe VALID OPTIONS are: [splenda, stevia, raw sugar, honey, whipped cream, sugar, oat milk, soy milk, regular milk, skimmed milk, whole milk, 2 percent milk, almond milk].nThe required info is: dimension. Dimension might be: small, medium, massive.nHere are some examples: <instance>H: I would love a medium latte with 1 Splenda and a small romano with no sugar please.nnA: <Validation>:nThe Human is ordering a medium latte with one splenda. Latte is a legitimate menu merchandise and splenda is a legitimate choice. The Human can also be ordering a small romano with no sugar. Romano is a legitimate menu merchandise.</Validation>n<Response>nOk, I bought: nt-Medium Latte with 1 Splenda and.nt-Small Romano with no Sugar.nIs this right?</Response>nnH: yep.nnA:n<Response>nGreat, Give me a second whereas I attempt to course of your order</instance>nn<instance>H: I would love a cappuccino and a mocha please.nnA: <Validation>:nThe Human is ordering a cappuccino and a mocha. Each are legitimate menu gadgets. The Human didn't present the dimensions for the cappuccino. The human didn't present the dimensions for the mocha. I'll ask the Human for the required lacking info.</Validation>n<Response>nSure factor, however are you able to please let me know the dimensions for the Cappuccino and the dimensions for the Mocha? We've Small, Medium, or Giant.</Response></instance>nn<instance>H: I would love a small cappuccino and a big lemonade please.nnA: <Validation>:nThe Human is ordering a small cappuccino and a big lemonade. Cappuccino is a legitimate menu merchandise. Lemonade will not be a legitimate menu merchandise. I'll recommend the Human a alternative from our legitimate menu gadgets.</Validation>n<Response>nSorry, we do not have Lemonades, would you wish to order one thing else as an alternative? Maybe a Frappe or a Latte?</Response></instance>nn<instance>H: Can I get a medium frappuccino with sugar please?nnA: <Validation>:n The Human is ordering a Frappuccino. Frappuccino will not be a legitimate menu merchandise. I'll recommend a alternative from the legitimate menu gadgets in my context.</Validation>n<Response>nI am so sorry, however Frappuccino will not be in our menu, would you like a frappe or a cappuccino as an alternative? maybe one thing else?</Response></instance>nn<instance>H: I need two massive americanos and a small latte please.nnA: <Validation>:n The Human is ordering 2 Giant Americanos, and a Small Latte. Americano is a legitimate menu merchandise. Latte is a legitimate menu merchandise.</Validation>n<Response>nOk, I bought: nt-2 Giant Americanos and.nt-Small Latte.nIs this right?</Response>nnH: appears to be like right, sure.nnA:n<Response>nGreat, Give me a second whereas I attempt to course of your order.</Response></instance>nn</Context>nnPlease full this request in response to the directions and examples supplied above:<request>REPLACEME</request>nnAssistant:n", "max_tokens_to_sample": 250, "temperature": 0.3, "top_k": 250, "top_p": 0.75, "stop_sequences": ["nnHuman:", "nnhuman:", "nnCustomer:", "nncustomer:"]}"
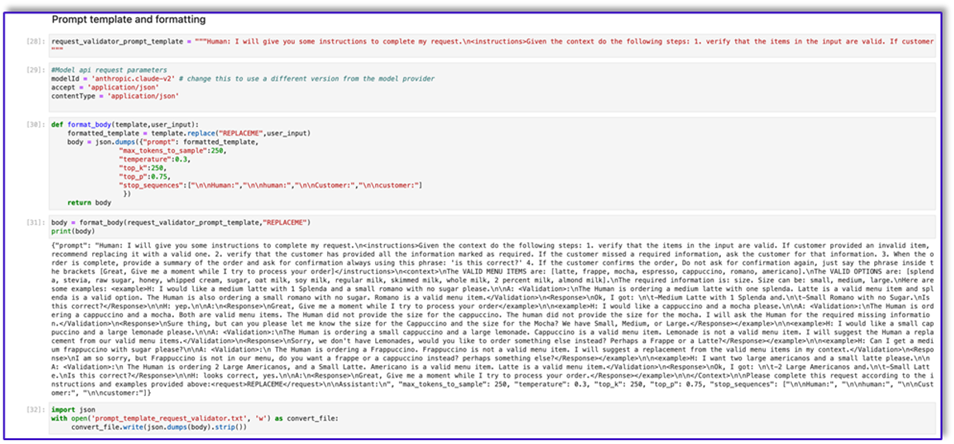
The next is our response from Amazon Bedrock utilizing this template.
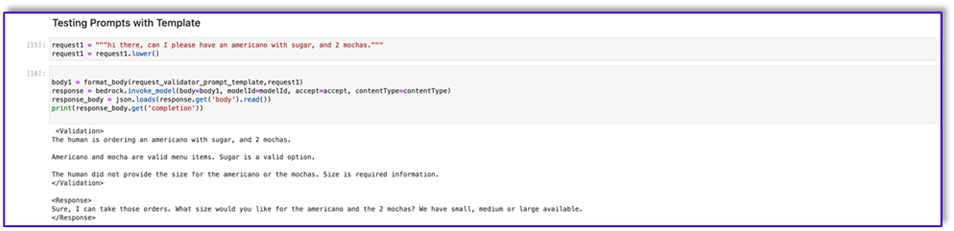
The next is the code for prompt_template_object_creator.txt
:
"{"immediate": "Human: I provides you with some directions to finish my request.n<directions>Given the Dialog between Human and Assistant, you'll want to create a json object in Response with the suitable attributes.nHere are some examples:n<instance><Dialog> H: I need a latte.nnA:nCan I've the dimensions?nnH: Medium.nnA: So, a medium latte.nIs this Appropriate?nnH: Sure.</Dialog>nnA:<Response>{"1":{"merchandise":"latte","dimension":"medium","addOns":[]}}</Response></instance>n<instance><Dialog> H: I need a big frappe and a couple of small americanos with sugar.nnA: Okay, let me verify:nn1 massive frappenn2 small americanos with sugarnnIs this right?nnH: Sure.</Dialog>nnA:<Response>{"1":{"merchandise":"frappe","dimension":"massive","addOns":[]},"2":{"merchandise":"americano","dimension":"small","addOns":["sugar"]},"3":{"merchandise":"americano","dimension":"small","addOns":["sugar"]}}</Response>n</instance>n<instance><Dialog> H: I need a medium americano.nnA: Okay, let me verify:nn1 medium americanonnIs this right?nnH: Sure.</Dialog>nnA:<Response>{"1":{"merchandise":"americano","dimension":"medium","addOns":[]}}</Response></instance>n<instance><Dialog> H: I need a big latte with oatmilk.nnA: Okay, let me verify:nnLarge latte with oatmilknnIs this right?nnH: Sure.</Dialog>nnA:<Response>{"1":{"merchandise":"latte","dimension":"massive","addOns":["oatmilk"]}}</Response></instance>n<instance><Dialog> H: I need a small mocha with no whipped cream please.nnA: Okay, let me verify:nnSmall mocha with no whipped creamnnIs this right?nnH: Sure.</Dialog>nnA:<Response>{"1":{"merchandise":"mocha","dimension":"small","addOns":["no whipped cream"]}}</Response>nn</instance></directions>nnPlease full this request in response to the directions and examples supplied above:<request><Dialog>REPLACEME</Dialog></request>nnAssistant:n", "max_tokens_to_sample": 250, "temperature": 0.3, "top_k": 250, "top_p": 0.75, "stop_sequences": ["nnHuman:", "nnhuman:", "nnCustomer:", "nncustomer:"]}"

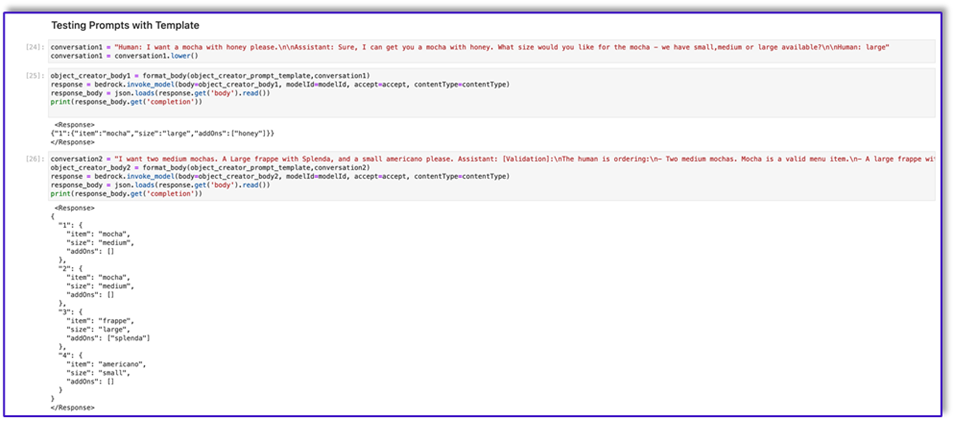
The next is the code for prompt_template_order_summary.txt:
"{"immediate": "Human: I provides you with some directions to finish my request.n<directions>Given the Dialog between Human and Assistant, you'll want to create a abstract of the order with bullet factors and embody the order whole.nHere are some examples:n<instance><Dialog> H: I need a big frappe and a couple of small americanos with sugar.nnA: Okay, let me verify:nn1 massive frappenn2 small americanos with sugarnnIs this right?nnH: Sure.</Dialog>nn<OrderTotal>10.50</OrderTotal>nnA:<Response>nHere is a abstract of your order together with the whole:nn1 massive frappenn2 small americanos with sugar.nYour Order whole is $10.50</Response></instance>n<instance><Dialog> H: I need a medium americano.nnA: Okay, let me verify:nn1 medium americanonnIs this right?nnH: Sure.</Dialog>nn<OrderTotal>3.50</OrderTotal>nnA:<Response>nHere is a abstract of your order together with the whole:nn1 medium americano.nYour Order whole is $3.50</Response></instance>n<instance><Dialog> H: I need a big latte with oat milk.nnA: Okay, let me verify:nnLarge latte with oat milknnIs this right?nnH: Sure.</Dialog>nn<OrderTotal>6.75</OrderTotal>nnA:<Response>nHere is a abstract of your order together with the whole:nnLarge latte with oat milk.nYour Order whole is $6.75</Response></instance>n<instance><Dialog> H: I need a small mocha with no whipped cream please.nnA: Okay, let me verify:nnSmall mocha with no whipped creamnnIs this right?nnH: Sure.</Dialog>nn<OrderTotal>4.25</OrderTotal>nnA:<Response>nHere is a abstract of your order together with the whole:nnSmall mocha with no whipped cream.nYour Order whole is $6.75</Response>nn</instance>n</directions>nnPlease full this request in response to the directions and examples supplied above:<request><Dialog>REPLACEME</Dialog>nn<OrderTotal>REPLACETOTAL</OrderTotal></request>nnAssistant:n", "max_tokens_to_sample": 250, "temperature": 0.3, "top_k": 250, "top_p": 0.75, "stop_sequences": ["nnHuman:", "nnhuman:", "nnCustomer:", "nncustomer:", "[Conversation]"]}"
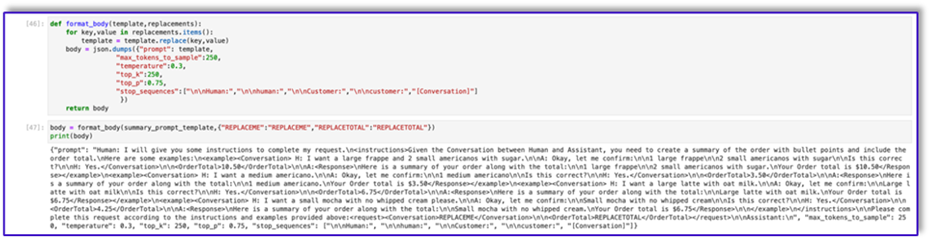
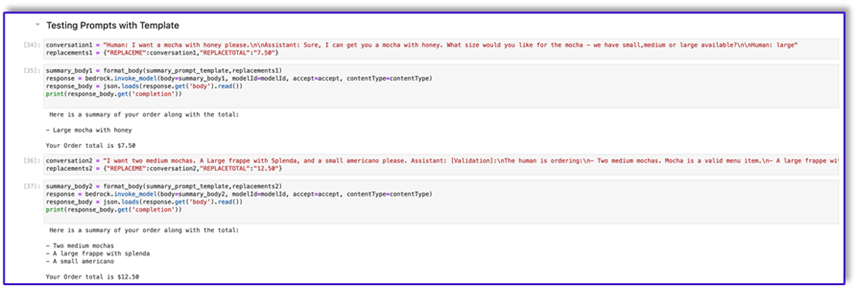
As you possibly can see, we’ve got used our immediate templates to validate menu gadgets, establish lacking required info, create an information construction, and summarize the order. The foundational fashions out there on Amazon Bedrock are very highly effective, so you can accomplish much more duties through these templates.
You will have accomplished engineering the prompts and saved the templates to textual content information. Now you can start creating the Amazon Lex bot and the related Lambda features.
Create a Lambda layer with the immediate templates
Full the next steps to create your Lambda layer:
- In SageMaker Studio, create a brand new folder with a subfolder named
python
.
- Copy your immediate information to the
python
folder.
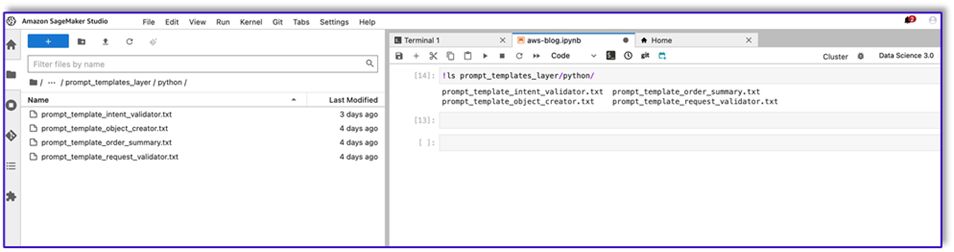
- You’ll be able to add the ZIP library to your pocket book occasion by working the next command.
!conda set up -y -c conda-forge zip
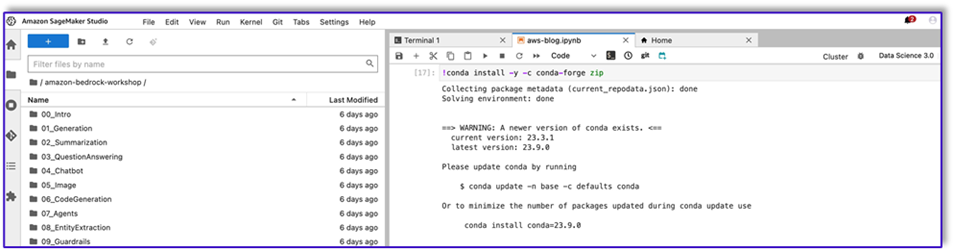
- Now, run the next command to create the ZIP file for importing to the Lambda layer.
!zip -r prompt_templates_layer.zip prompt_templates_layer/.
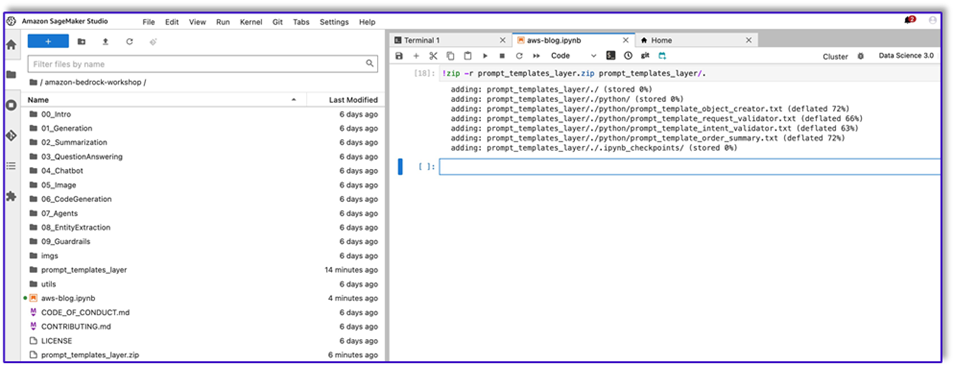
- After you create the ZIP file, you possibly can obtain the file. Go to Lambda, create a brand new layer by importing the file instantly or by importing to Amazon S3 first.
- Then connect this new layer to the orchestration Lambda perform.
Now your immediate template information are regionally saved in your Lambda runtime atmosphere. It will pace up the method throughout your bot runs.
Create a Lambda layer with the required libraries
Full the next steps to create your Lambda layer with the required librarues:
- Open an AWS Cloud9 occasion atmosphere, create a folder with a subfolder referred to as
python
.
- Open a terminal contained in the
python
folder.
- Run the next instructions from the terminal:
pip set up “boto3>=1.28.57” -t .
pip set up “awscli>=1.29.57" -t .
pip set up “botocore>=1.31.57” -t .
- Run
cd ..
and place your self inside your new folder the place you even have the python
subfolder.
- Run the next command:
- After you create the ZIP file, you possibly can obtain the file. Go to Lambda, create a brand new layer by importing the file instantly or by importing to Amazon S3 first.
- Then connect this new layer to the orchestration Lambda perform.
Create the bot in Amazon Lex v2
For this use case, we construct an Amazon Lex bot that may present an enter/output interface for the structure as a way to name Amazon Bedrock utilizing voice or textual content from any interface. As a result of the LLM will deal with the dialog piece of this order processing agent, and Lambda will orchestrate the workflow, you possibly can create a bot with three intents and no slots.
- On the Amazon Lex console, create a brand new bot with the strategy Create a clean bot.
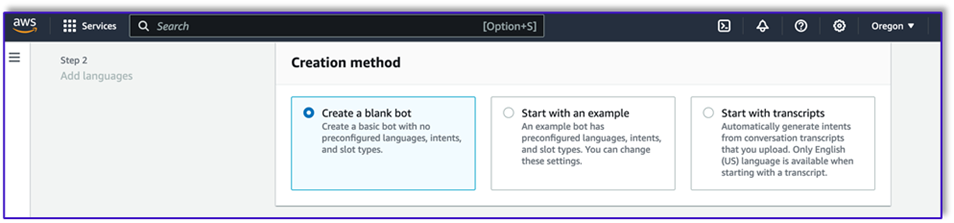
Now you possibly can add an intent with any applicable preliminary utterance for the end-users to start out the dialog with the bot. We use easy greetings and add an preliminary bot response so end-users can present their requests. When creating the bot, make sure that to make use of a Lambda code hook with the intents; this can set off a Lambda perform that can orchestrate the workflow between the shopper, Amazon Lex, and the LLM.
- Add your first intent, which triggers the workflow and makes use of the intent validation immediate template to name Amazon Bedrock and establish what the shopper is attempting to perform. Add just a few easy utterances for end-users to start out dialog.
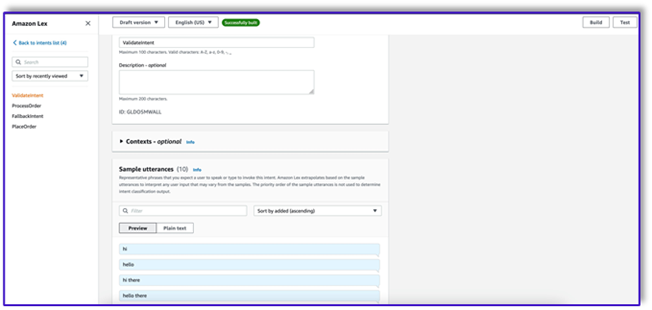
You don’t want to make use of any slots or preliminary studying in any of the bot intents. The truth is, you don’t want so as to add utterances to the second or third intents. That’s as a result of the LLM will information Lambda all through the method.
- Add a affirmation immediate. You’ll be able to customise this message within the Lambda perform later.
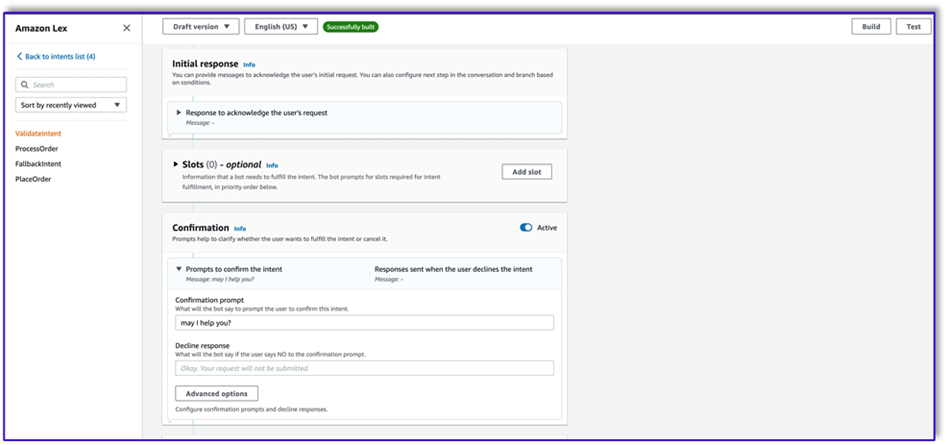
- Underneath Code hooks, choose Use a Lambda perform for initialization and validation.
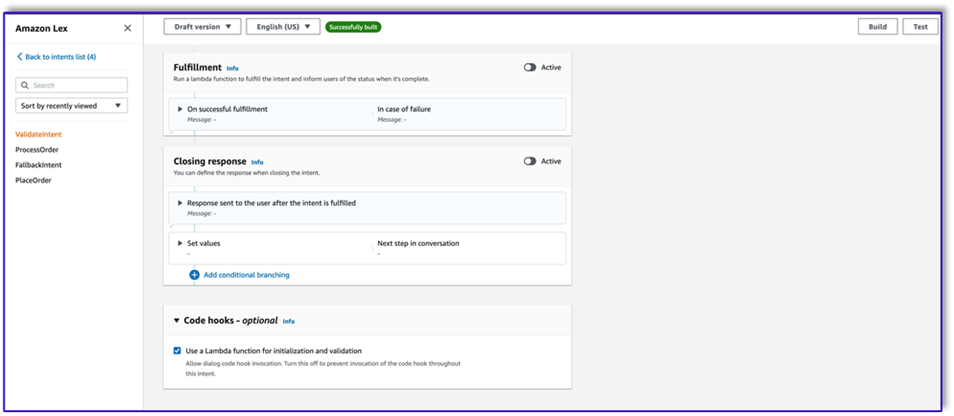
- Create a second intent with no utterance and no preliminary response. That is the
PlaceOrder
intent.
When the LLM identifies that the shopper is attempting to position an order, the Lambda perform will set off this intent and validate the shopper request in opposition to the menu, and ensure that no required info is lacking. Keep in mind that all of that is on the immediate templates, so you possibly can adapt this workflow for any use case by altering the immediate templates.
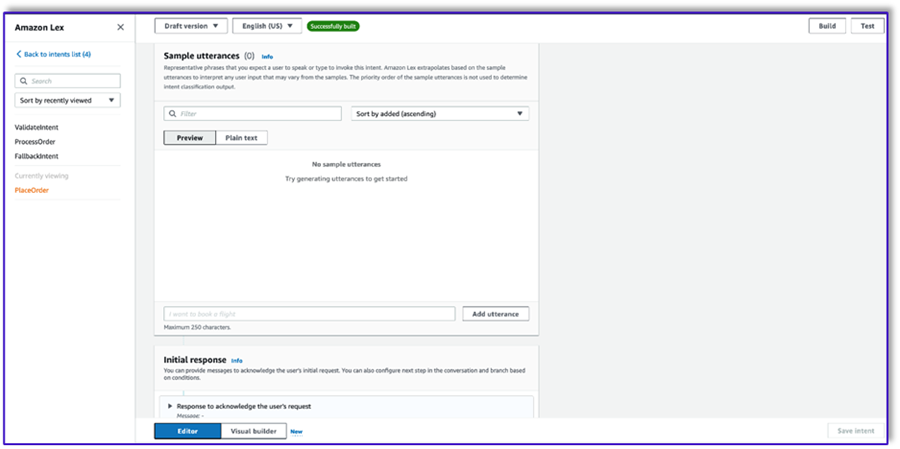
- Don’t add any slots, however add a affirmation immediate and decline response.
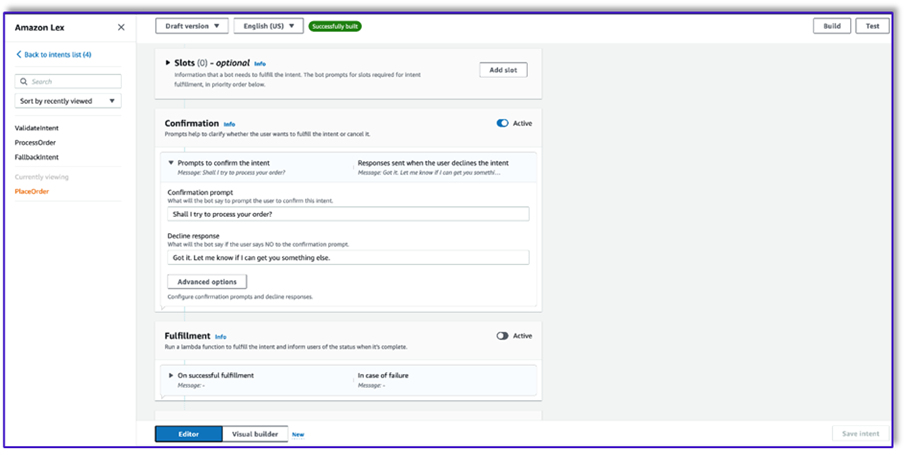
- Choose Use a Lambda perform for initialization and validation.
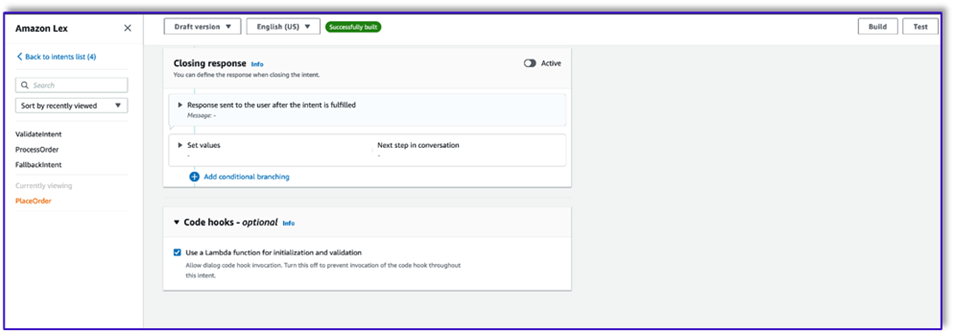
- Create a 3rd intent named
ProcessOrder
with no pattern utterances and no slots.
- Add an preliminary response, a affirmation immediate, and a decline response.
After the LLM has validated the shopper request, the Lambda perform triggers the third and final intent to course of the order. Right here, Lambda will use the thing creator template to generate the order JSON information construction to question the DynamoDB desk, after which use the order abstract template to summarize the entire order together with the whole so Amazon Lex can go it to the shopper.
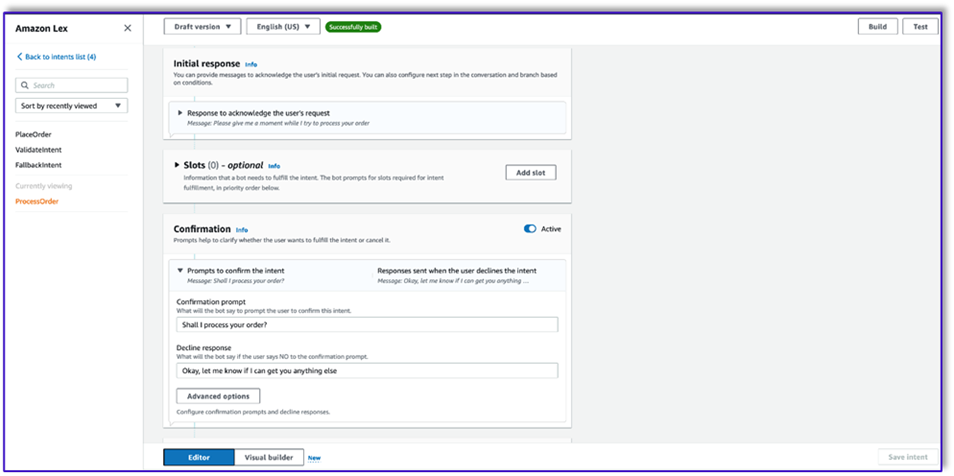
- Choose Use a Lambda perform for initialization and validation. This will use any Lambda perform to course of the order after the shopper has given the ultimate affirmation.
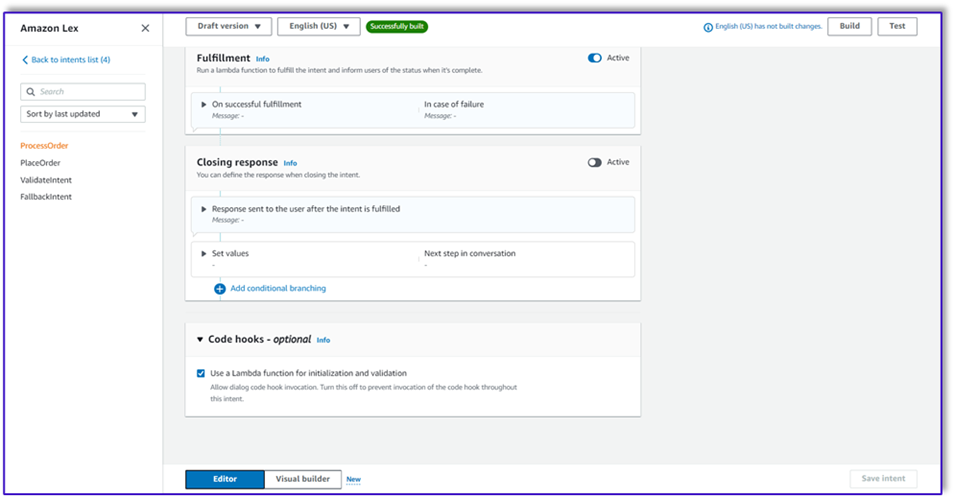
- After you create all three intents, go to the Visible builder for the
ValidateIntent
, add a go-to intent step, and join the output of the constructive affirmation to that step.
- After you add the go-to intent, edit it and select the PlaceOrder intent because the intent title.
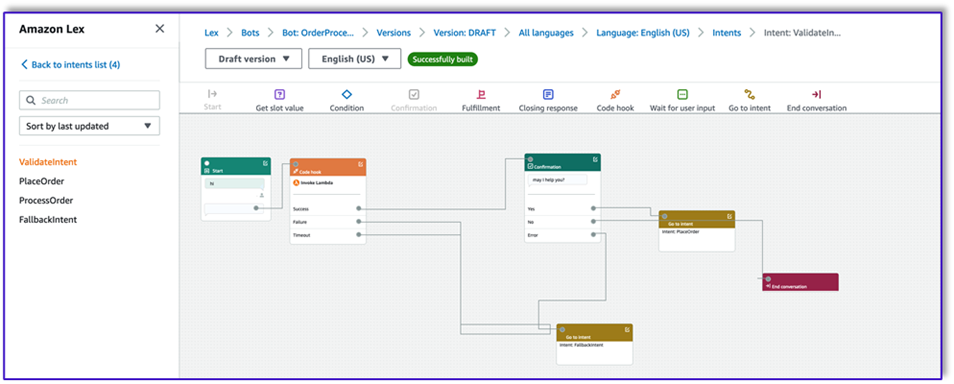
- Equally, to go the Visible builder for the
PlaceOrder
intent and join the output of the constructive affirmation to the ProcessOrder
go-to intent. No modifying is required for the ProcessOrder
intent.
- You now have to create the Lambda perform that orchestrates Amazon Lex and calls the DynamoDB desk, as detailed within the following part.
Create a Lambda perform to orchestrate the Amazon Lex bot
Now you can construct the Lambda perform that orchestrates the Amazon Lex bot and workflow. Full the next steps:
- Create a Lambda perform with the usual execution coverage and let Lambda create a task for you.
- Within the code window of your perform, add just a few utility features that can assist: format the prompts by including the lex context to the template, name the Amazon Bedrock LLM API, extract the specified textual content from the responses, and extra. See the next code:
import json
import re
import boto3
import logging
logger = logging.getLogger()
logger.setLevel(logging.DEBUG)
bedrock = boto3.consumer(service_name="bedrock-runtime")
def CreatingCustomPromptFromLambdaLayer(object_key,replace_items):
folder_path="/choose/order_processing_agent_prompt_templates/python/"
strive:
file_path = folder_path + object_key
with open(file_path, "r") as file1:
raw_template = file1.learn()
# Modify the template with the customized enter immediate
#template['inputs'][0].insert(1, {"function": "person", "content material": '### Enter:n' + user_request})
for key,worth in replace_items.gadgets():
worth = json.dumps(json.dumps(worth).substitute('"','')).substitute('"','')
raw_template = raw_template.substitute(key,worth)
modified_prompt = raw_template
return modified_prompt
besides Exception as e:
return {
'statusCode': 500,
'physique': f'An error occurred: {str(e)}'
}
def CreatingCustomPrompt(object_key,replace_items):
logger.debug('replace_items is: {}'.format(replace_items))
#retrieve person request from intent_request
#we first propmt the mannequin with present order
bucket_name="your-bucket-name"
#object_key = 'prompt_template_order_processing.txt'
strive:
s3 = boto3.consumer('s3')
# Retrieve the present template from S3
response = s3.get_object(Bucket=bucket_name, Key=object_key)
raw_template = response['Body'].learn().decode('utf-8')
raw_template = json.masses(raw_template)
logger.debug('uncooked template is {}'.format(raw_template))
#template_json = json.masses(raw_template)
#logger.debug('template_json is {}'.format(template_json))
#template = json.dumps(template_json)
#logger.debug('template is {}'.format(template))
# Modify the template with the customized enter immediate
#template['inputs'][0].insert(1, {"function": "person", "content material": '### Enter:n' + user_request})
for key,worth in replace_items.gadgets():
raw_template = raw_template.substitute(key,worth)
logger.debug("Changing: {} nwith: {}".format(key,worth))
modified_prompt = json.dumps(raw_template)
logger.debug("Modified template: {}".format(modified_prompt))
logger.debug("Modified template kind is: {}".format(print(kind(modified_prompt))))
#modified_template_json = json.masses(modified_prompt)
#logger.debug("Modified template json: {}".format(modified_template_json))
return modified_prompt
besides Exception as e:
return {
'statusCode': 500,
'physique': f'An error occurred: {str(e)}'
}
def validate_intent(intent_request):
logger.debug('beginning validate_intent: {}'.format(intent_request))
#retrieve person request from intent_request
user_request="Human: " + intent_request['inputTranscript'].decrease()
#getting present context variable
current_session_attributes = intent_request['sessionState']['sessionAttributes']
if len(current_session_attributes) > 0:
full_context = current_session_attributes['fullContext'] + 'nn' + user_request
dialog_context = current_session_attributes['dialogContext'] + 'nn' + user_request
else:
full_context = user_request
dialog_context = user_request
#Getting ready validation immediate by including context to immediate template
object_key = 'prompt_template_intent_validator.txt'
#replace_items = {"REPLACEME":full_context}
#replace_items = {"REPLACEME":dialog_context}
replace_items = {"REPLACEME":dialog_context}
#validation_prompt = CreatingCustomPrompt(object_key,replace_items)
validation_prompt = CreatingCustomPromptFromLambdaLayer(object_key,replace_items)
#Prompting mannequin for request validation
intent_validation_completion = prompt_bedrock(validation_prompt)
intent_validation_completion = re.sub(r'["]','',intent_validation_completion)
#extracting response from response completion and eradicating some particular characters
validation_response = extract_response(intent_validation_completion)
validation_intent = extract_intent(intent_validation_completion)
#enterprise logic relying on intents
if validation_intent == 'Place Order':
return validate_request(intent_request)
elif validation_intent in ['Complain','Speak to Someone']:
##including session attributes to maintain present context
full_context = full_context + 'nn' + intent_validation_completion
dialog_context = dialog_context + 'nnAssistant: ' + validation_response
intent_request['sessionState']['sessionAttributes']['fullContext'] = full_context
intent_request['sessionState']['sessionAttributes']['dialogContext'] = dialog_context
intent_request['sessionState']['sessionAttributes']['customerIntent'] = validation_intent
return shut(intent_request['sessionState']['sessionAttributes'],intent_request['sessionState']['intent']['name'],'Fulfilled','Shut',validation_response)
if validation_intent == 'Greeting':
##including session attributes to maintain present context
full_context = full_context + 'nn' + intent_validation_completion
dialog_context = dialog_context + 'nnAssistant: ' + validation_response
intent_request['sessionState']['sessionAttributes']['fullContext'] = full_context
intent_request['sessionState']['sessionAttributes']['dialogContext'] = dialog_context
intent_request['sessionState']['sessionAttributes']['customerIntent'] = validation_intent
return shut(intent_request['sessionState']['sessionAttributes'],intent_request['sessionState']['intent']['name'],'InProgress','ConfirmIntent',validation_response)
def validate_request(intent_request):
logger.debug('beginning validate_request: {}'.format(intent_request))
#retrieve person request from intent_request
user_request="Human: " + intent_request['inputTranscript'].decrease()
#getting present context variable
current_session_attributes = intent_request['sessionState']['sessionAttributes']
if len(current_session_attributes) > 0:
full_context = current_session_attributes['fullContext'] + 'nn' + user_request
dialog_context = current_session_attributes['dialogContext'] + 'nn' + user_request
else:
full_context = user_request
dialog_context = user_request
#Getting ready validation immediate by including context to immediate template
object_key = 'prompt_template_request_validator.txt'
replace_items = {"REPLACEME":dialog_context}
#validation_prompt = CreatingCustomPrompt(object_key,replace_items)
validation_prompt = CreatingCustomPromptFromLambdaLayer(object_key,replace_items)
#Prompting mannequin for request validation
request_validation_completion = prompt_bedrock(validation_prompt)
request_validation_completion = re.sub(r'["]','',request_validation_completion)
#extracting response from response completion and eradicating some particular characters
validation_response = extract_response(request_validation_completion)
##including session attributes to maintain present context
full_context = full_context + 'nn' + request_validation_completion
dialog_context = dialog_context + 'nnAssistant: ' + validation_response
intent_request['sessionState']['sessionAttributes']['fullContext'] = full_context
intent_request['sessionState']['sessionAttributes']['dialogContext'] = dialog_context
return shut(intent_request['sessionState']['sessionAttributes'],'PlaceOrder','InProgress','ConfirmIntent',validation_response)
def process_order(intent_request):
logger.debug('beginning process_order: {}'.format(intent_request))
#retrieve person request from intent_request
user_request="Human: " + intent_request['inputTranscript'].decrease()
#getting present context variable
current_session_attributes = intent_request['sessionState']['sessionAttributes']
if len(current_session_attributes) > 0:
full_context = current_session_attributes['fullContext'] + 'nn' + user_request
dialog_context = current_session_attributes['dialogContext'] + 'nn' + user_request
else:
full_context = user_request
dialog_context = user_request
# Getting ready object creator immediate by including context to immediate template
object_key = 'prompt_template_object_creator.txt'
replace_items = {"REPLACEME":dialog_context}
#object_creator_prompt = CreatingCustomPrompt(object_key,replace_items)
object_creator_prompt = CreatingCustomPromptFromLambdaLayer(object_key,replace_items)
#Prompting mannequin for object creation
object_creation_completion = prompt_bedrock(object_creator_prompt)
#extracting response from response completion
object_creation_response = extract_response(object_creation_completion)
inputParams = json.masses(object_creation_response)
inputParams = json.dumps(json.dumps(inputParams))
logger.debug('inputParams is: {}'.format(inputParams))
consumer = boto3.consumer('lambda')
response = consumer.invoke(FunctionName="arn:aws:lambda:us-east-1:<AccountNumber>:perform:aws-blog-order-validator",InvocationType="RequestResponse",Payload = inputParams)
responseFromChild = json.load(response['Payload'])
validationResult = responseFromChild['statusCode']
if validationResult == 205:
order_validation_error = responseFromChild['validator_response']
return shut(intent_request['sessionState']['sessionAttributes'],'PlaceOrder','InProgress','ConfirmIntent',order_validation_error)
#invokes Order Processing lambda to question DynamoDB desk and returns order whole
response = consumer.invoke(FunctionName="arn:aws:lambda:us-east-1: <AccountNumber>:perform:aws-blog-order-processing",InvocationType="RequestResponse",Payload = inputParams)
responseFromChild = json.load(response['Payload'])
orderTotal = responseFromChild['body']
###Prompting the mannequin to summarize the order together with order whole
object_key = 'prompt_template_order_summary.txt'
replace_items = {"REPLACEME":dialog_context,"REPLACETOTAL":orderTotal}
#order_summary_prompt = CreatingCustomPrompt(object_key,replace_items)
order_summary_prompt = CreatingCustomPromptFromLambdaLayer(object_key,replace_items)
order_summary_completion = prompt_bedrock(order_summary_prompt)
#extracting response from response completion
order_summary_response = extract_response(order_summary_completion)
order_summary_response = order_summary_response + '. Shall I finalize processing your order?'
##including session attributes to maintain present context
full_context = full_context + 'nn' + order_summary_completion
dialog_context = dialog_context + 'nnAssistant: ' + order_summary_response
intent_request['sessionState']['sessionAttributes']['fullContext'] = full_context
intent_request['sessionState']['sessionAttributes']['dialogContext'] = dialog_context
return shut(intent_request['sessionState']['sessionAttributes'],'ProcessOrder','InProgress','ConfirmIntent',order_summary_response)
""" --- Principal handler and Workflow features --- """
def lambda_handler(occasion, context):
"""
Route the incoming request based mostly on intent.
The JSON physique of the request is supplied within the occasion slot.
"""
logger.debug('occasion is: {}'.format(occasion))
return dispatch(occasion)
def dispatch(intent_request):
"""
Referred to as when the person specifies an intent for this bot. If intent will not be legitimate then returns error title
"""
logger.debug('intent_request is: {}'.format(intent_request))
intent_name = intent_request['sessionState']['intent']['name']
confirmation_state = intent_request['sessionState']['intent']['confirmationState']
# Dispatch to your bot's intent handlers
if intent_name == 'ValidateIntent' and confirmation_state == 'None':
return validate_intent(intent_request)
if intent_name == 'PlaceOrder' and confirmation_state == 'None':
return validate_request(intent_request)
elif intent_name == 'PlaceOrder' and confirmation_state == 'Confirmed':
return process_order(intent_request)
elif intent_name == 'PlaceOrder' and confirmation_state == 'Denied':
return shut(intent_request['sessionState']['sessionAttributes'],intent_request['sessionState']['intent']['name'],'Fulfilled','Shut','Obtained it. Let me know if I may also help you with one thing else.')
elif intent_name == 'PlaceOrder' and confirmation_state not in ['Denied','Confirmed','None']:
return shut(intent_request['sessionState']['sessionAttributes'],intent_request['sessionState']['intent']['name'],'Fulfilled','Shut','Sorry. I'm having bother finishing the request. Let me get somebody that can assist you.')
logger.debug('exiting intent {} right here'.format(intent_request['sessionState']['intent']['name']))
elif intent_name == 'ProcessOrder' and confirmation_state == 'None':
return validate_request(intent_request)
elif intent_name == 'ProcessOrder' and confirmation_state == 'Confirmed':
return shut(intent_request['sessionState']['sessionAttributes'],intent_request['sessionState']['intent']['name'],'Fulfilled','Shut','Excellent! Your order has been processed. Please proceed to cost.')
elif intent_name == 'ProcessOrder' and confirmation_state == 'Denied':
return shut(intent_request['sessionState']['sessionAttributes'],intent_request['sessionState']['intent']['name'],'Fulfilled','Shut','Obtained it. Let me know if I may also help you with one thing else.')
elif intent_name == 'ProcessOrder' and confirmation_state not in ['Denied','Confirmed','None']:
return shut(intent_request['sessionState']['sessionAttributes'],intent_request['sessionState']['intent']['name'],'Fulfilled','Shut','Sorry. I'm having bother finishing the request. Let me get somebody that can assist you.')
logger.debug('exiting intent {} right here'.format(intent_request['sessionState']['intent']['name']))
increase Exception('Intent with title ' + intent_name + ' not supported')
def prompt_bedrock(formatted_template):
logger.debug('immediate bedrock enter is:'.format(formatted_template))
physique = json.masses(formatted_template)
modelId = 'anthropic.claude-v2' # change this to make use of a special model from the mannequin supplier
settle for="utility/json"
contentType="utility/json"
response = bedrock.invoke_model(physique=physique, modelId=modelId, settle for=settle for, contentType=contentType)
response_body = json.masses(response.get('physique').learn())
response_completion = response_body.get('completion')
logger.debug('response is: {}'.format(response_completion))
#print_ww(response_body.get('completion'))
#print(response_body.get('outcomes')[0].get('outputText'))
return response_completion
#perform to extract textual content between the <Response> and </Response> tags inside mannequin completion
def extract_response(response_completion):
if '<Response>' in response_completion:
customer_response = response_completion.substitute('<Response>','||').substitute('</Response>','').cut up('||')[1]
logger.debug('modified response is: {}'.format(response_completion))
return customer_response
else:
logger.debug('modified response is: {}'.format(response_completion))
return response_completion
#perform to extract textual content between the <Response> and </Response> tags inside mannequin completion
def extract_intent(response_completion):
if '<intent>' in response_completion:
customer_intent = response_completion.substitute('<intent>','||').substitute('</intent>','||').cut up('||')[1]
return customer_intent
else:
return customer_intent
def shut(session_attributes, intent, fulfillment_state, action_type, message):
#This perform prepares the response within the appropiate format for Lex V2
response = {
"sessionState": {
"sessionAttributes":session_attributes,
"dialogAction": {
"kind": action_type
},
"intent": {
"title":intent,
"state":fulfillment_state
},
},
"messages":
[{
"contentType":"PlainText",
"content":message,
}]
,
}
return response
- Connect the Lambda layer you created earlier to this perform.
- Moreover, connect the layer to the immediate templates you created.
- Within the Lambda execution function, connect the coverage to entry Amazon Bedrock, which was created earlier.

The Lambda execution function ought to have the next permissions.
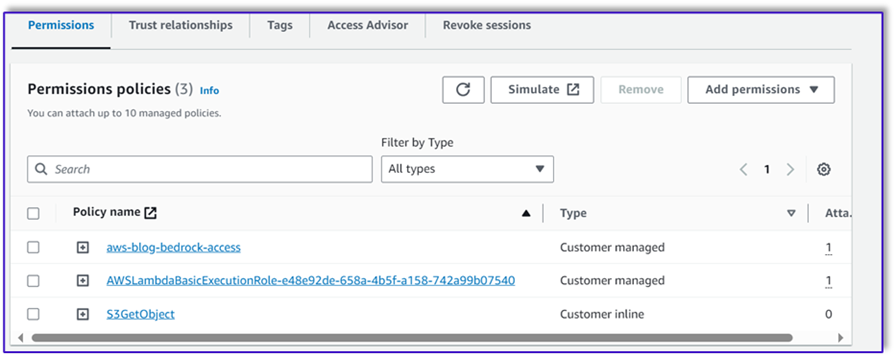
Connect the Orchestration Lambda perform to the Amazon Lex bot
- After you create the perform within the earlier part, return to the Amazon Lex console and navigate to your bot.
- Underneath Languages within the navigation pane, select English.
- For Supply, select your order processing bot.
- For Lambda perform model or alias, select $LATEST.
- Select Save.
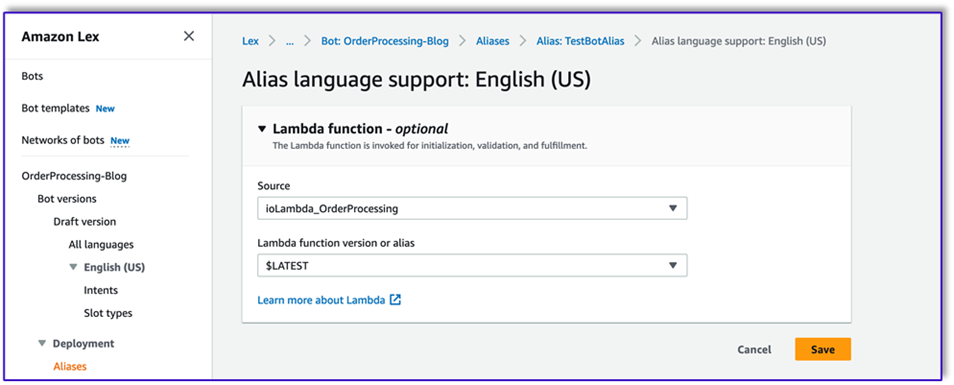
Create aiding Lambda features
Full the next steps to create extra Lambda features:
- Create a Lambda perform to question the DynamoDB desk that you simply created earlier:
import json
import boto3
import logging
logger = logging.getLogger()
logger.setLevel(logging.DEBUG)
# Initialize the DynamoDB consumer
dynamodb = boto3.useful resource('dynamodb')
desk = dynamodb.Desk('your-table-name')
def calculate_grand_total(input_data):
# Initialize the whole value
total_price = 0
strive:
# Loop by every merchandise within the enter JSON
for item_id, item_data in input_data.gadgets():
item_name = item_data['item'].decrease() # Convert merchandise title to lowercase
item_size = item_data['size'].decrease() # Convert merchandise dimension to lowercase
# Question the DynamoDB desk for the merchandise based mostly on Merchandise and Dimension
response = desk.get_item(
Key={'Merchandise': item_name,
'Dimension': item_size}
)
# Examine if the merchandise was discovered within the desk
if 'Merchandise' in response:
merchandise = response['Item']
value = float(merchandise['Price'])
total_price += value # Add the merchandise's value to the whole
return total_price
besides Exception as e:
increase Exception('An error occurred: {}'.format(str(e)))
def lambda_handler(occasion, context):
strive:
# Parse the enter JSON from the Lambda occasion
input_json = json.masses(occasion)
# Calculate the grand whole
grand_total = calculate_grand_total(input_json)
# Return the grand whole within the response
return {'statusCode': 200,'physique': json.dumps(grand_total)}
besides Exception as e:
return {
'statusCode': 500,
'physique': json.dumps('An error occurred: {}'.format(str(e)))
- Navigate to the Configuration tab within the Lambda perform and select Permissions.
- Connect a resource-based coverage assertion permitting the order processing Lambda perform to invoke this perform.
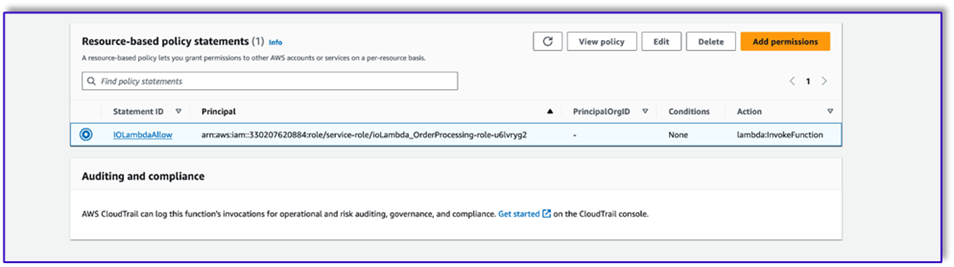
- Navigate to the IAM execution function for this Lambda perform and add a coverage to entry the DynamoDB desk.
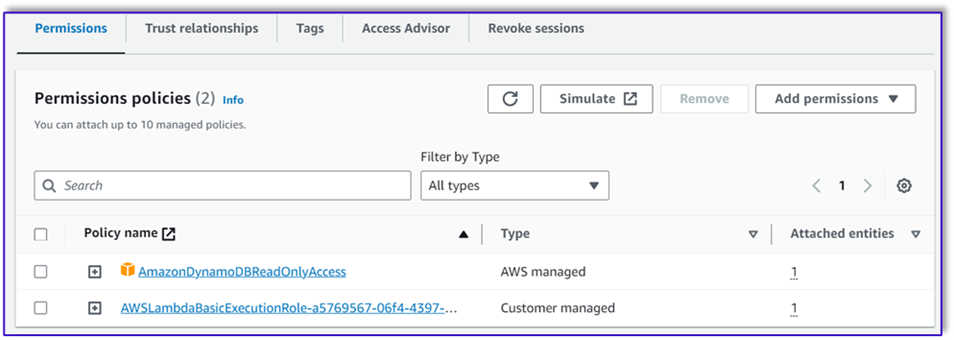
- Create one other Lambda perform to validate if all required attributes had been handed from the shopper. Within the following instance, we validate if the dimensions attribute is captured for an order:
import json
import logging
logger = logging.getLogger()
logger.setLevel(logging.DEBUG)
def lambda_handler(occasion, context):
# Outline buyer orders from the enter occasion
customer_orders = json.masses(occasion)
# Initialize a listing to gather error messages
order_errors = {}
missing_size = []
error_messages = []
# Iterate by every order in customer_orders
for order_id, order in customer_orders.gadgets():
if "dimension" not so as or order["size"] == "":
missing_size.append(order['item'])
order_errors['size'] = missing_size
if order_errors:
items_missing_size = order_errors['size']
error_message = f"might you please present the dimensions for the next gadgets: {', '.be part of(items_missing_size)}?"
error_messages.append(error_message)
# Put together the response message
if error_messages:
response_message = "n".be part of(error_messages)
return {
'statusCode': 205,
'validator_response': response_message
}
else:
response_message = "Order is validated efficiently"
return {
'statusCode': 200,
'validator_response': response_message
}
- Navigate to the Configuration tab within the Lambda perform and select Permissions.
- Connect a resource-based coverage assertion permitting the order processing Lambda perform to invoke this perform.
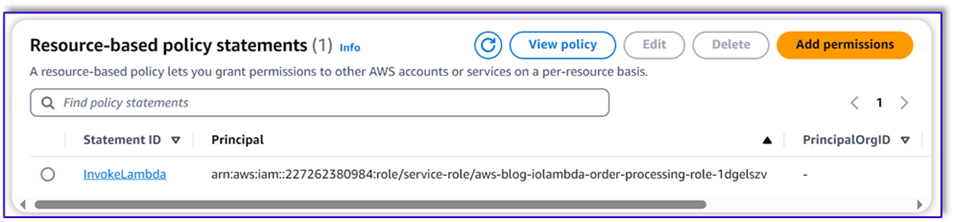
Check the answer
Now we are able to take a look at the answer with instance orders that clients place through Amazon Lex.
For our first instance, the shopper requested for a frappuccino, which isn’t on the menu. The mannequin validates with the assistance of order validator template and suggests some suggestions based mostly on the menu. After the shopper confirms their order, they’re notified of the order whole and order abstract. The order can be processed based mostly on the shopper’s closing affirmation.
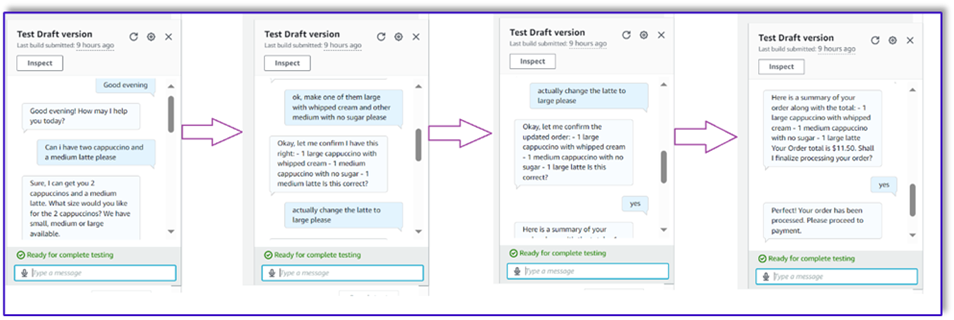
In our subsequent instance, the shopper is ordering for big cappuccino after which modifying the dimensions from massive to medium. The mannequin captures all mandatory modifications and requests the shopper to substantiate the order. The mannequin presents the order whole and order abstract, and processes the order based mostly on the shopper’s closing affirmation.
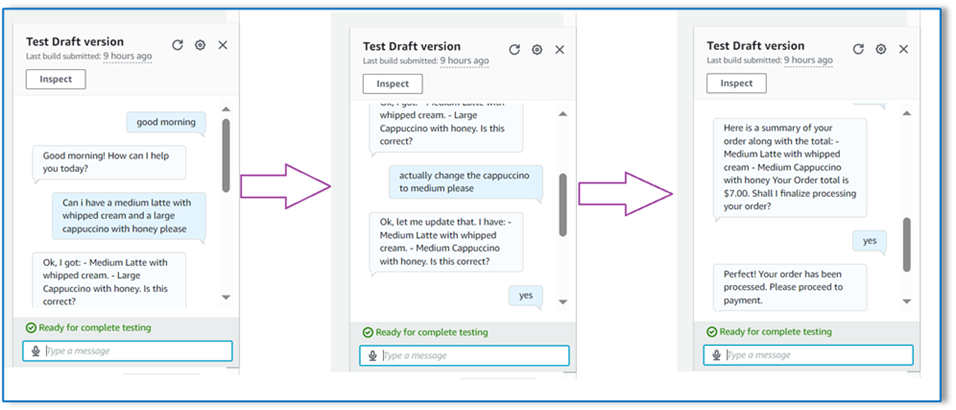
For our closing instance, the shopper positioned an order for a number of gadgets and the dimensions is lacking for a few gadgets. The mannequin and Lambda perform will confirm if all required attributes are current to course of the order after which ask the shopper to supply the lacking info. After the shopper supplies the lacking info (on this case, the dimensions of the espresso), they’re proven the order whole and order abstract. The order can be processed based mostly on the shopper’s closing affirmation.
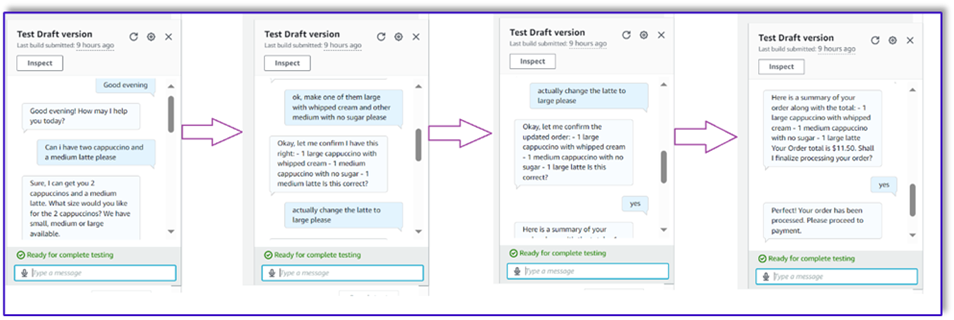
LLM limitations
LLM outputs are stochastic by nature, which implies that the outcomes from our LLM can fluctuate in format, and even within the type of untruthful content material (hallucinations). Subsequently, builders have to depend on a superb error dealing with logic all through their code as a way to deal with these eventualities and keep away from a degraded end-user expertise.
Clear up
If you happen to now not want this resolution, you possibly can delete the next assets:
- Lambda features
- Amazon Lex field
- DynamoDB desk
- S3 bucket
Moreover, shut down the SageMaker Studio occasion if the applying is now not required.
Value evaluation
For pricing info for the primary providers utilized by this resolution, see the next:
Notice that you need to use Claude v2 with out the necessity for provisioning, so total prices stay at a minimal. To additional scale back prices, you possibly can configure the DynamoDB desk with the on-demand setting.
Conclusion
This put up demonstrated easy methods to construct a speech-enabled AI order processing agent utilizing Amazon Lex, Amazon Bedrock, and different AWS providers. We confirmed how immediate engineering with a robust generative AI mannequin like Claude can allow sturdy pure language understanding and dialog flows for order processing with out the necessity for in depth coaching information.
The answer structure makes use of serverless elements like Lambda, Amazon S3, and DynamoDB to allow a versatile and scalable implementation. Storing the immediate templates in Amazon S3 permits you to customise the answer for various use circumstances.
Subsequent steps might embody increasing the agent’s capabilities to deal with a wider vary of buyer requests and edge circumstances. The immediate templates present a option to iteratively enhance the agent’s abilities. Further customizations might contain integrating the order information with backend methods like stock, CRM, or POS. Lastly, the agent may very well be made out there throughout varied buyer touchpoints like cellular apps, drive-thru, kiosks, and extra utilizing the multi-channel capabilities of Amazon Lex.
To be taught extra, confer with the next associated assets:
- Deploying and managing multi-channel bots:
- Immediate engineering for Claude and different fashions:
- Serverless architectural patterns for scalable AI assistants:
In regards to the Authors
Moumita Dutta is a Accomplice Resolution Architect at Amazon Internet Companies. In her function, she collaborates intently with companions to develop scalable and reusable belongings that streamline cloud deployments and improve operational effectivity. She is a member of AI/ML neighborhood and a Generative AI knowledgeable at AWS. In her leisure, she enjoys gardening and biking.
Fernando Lammoglia is a Accomplice Options Architect at Amazon Internet Companies, working intently with AWS companions in spearheading the event and adoption of cutting-edge AI options throughout enterprise items. A strategic chief with experience in cloud structure, generative AI, machine studying, and information analytics. He focuses on executing go-to-market methods and delivering impactful AI options aligned with organizational targets. On his free time he likes to spend time together with his household and journey to different international locations.
Mitul Patel is a Senior Resolution Architect at Amazon Internet Companies. In his function as a cloud know-how enabler, he works with clients to know their targets and challenges, and supplies prescriptive steering to realize their goal with AWS choices. He’s a member of AI/ML neighborhood and a Generative AI ambassador at AWS. In his free time, he enjoys climbing and enjoying soccer.