However What’s Backpropagation, Actually? (Half 1) | by Matthew Chak | Feb, 2024
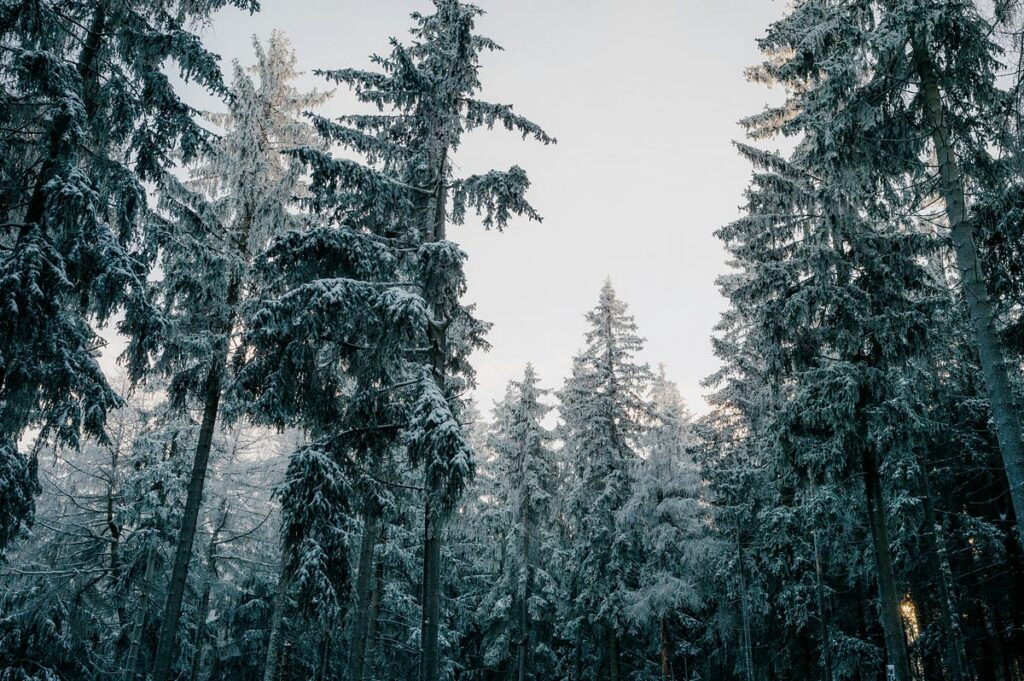
Regardless of performing some work and analysis within the AI ecosystem for a while, I didn’t really cease to consider backpropagation and gradient updates inside neural networks till not too long ago. This text seeks to rectify that and can hopefully present an intensive but easy-to-follow dive into the subject by implementing a easy (but considerably highly effective) neural community framework from scratch.
Essentially, a neural community is only a mathematical operate from our enter area to our desired output area. In truth, we are able to successfully “unwrap” any neural community right into a operate. Contemplate, as an example, the next easy neural community with two layers and one enter:
We are able to now assemble an equal operate by going forwards layer by layer, ranging from the enter. Let’s observe our remaining operate layer by layer:
- On the enter, we begin with the id operate pred(x) = x
- On the first linear layer, we get pred(x) = w₁x + b₁
- The ReLU nets us pred(x) = max(0, w₁x + b₁)
- On the remaining layer, we get pred(x) = w₂(max(0, w₁x + b₁)) + b₂
With extra difficult nets, these features after all get unwieldy, however the level is that we are able to assemble such representations of neural networks.
We are able to go one step additional although — features of this manner usually are not extraordinarily handy for computation, however we are able to parse them right into a extra helpful kind, particularly a syntax tree. For our easy internet, the tree would seem like this:
On this tree kind, our leaves are parameters, constants, and inputs, and the opposite nodes are elementary operations whose arguments are their youngsters. After all, these elementary operations don’t must be binary — the sigmoid operation, as an example, is unary (and so is ReLU if we don’t signify it as a max of 0 and x), and we are able to select to assist multiplication and addition of a couple of enter.
By pondering of our community as a tree of those elementary operations, we are able to now do a number of issues very simply with recursion, which can kind the premise of each our backpropagation and ahead propagation algorithms. In code, we are able to outline a recursive neural community class that appears like this:
from dataclasses import dataclass, discipline
from typing import Listing@dataclass
class NeuralNetNode:
"""A node in our neural community tree"""
youngsters: Listing['NeuralNetNode'] = discipline(default_factory=checklist)
def op(self, x: Listing[float]) -> float:
"""The operation that this node performs"""
increase NotImplementedError
def ahead(self) -> float:
"""Consider this node on the given enter"""
return self.op([child.forward() for child in self.children])
# That is only for comfort
def __call__(self) -> Listing[float]:
return self.ahead()
def __repr__(self):
return f'{self.__class__.__name__}({self.youngsters})'
Suppose now that we’ve a differentiable loss operate for our neural community, say MSE. Recall that MSE (for one pattern) is outlined as follows:
We now want to replace our parameters (the inexperienced circles in our tree illustration) given the worth of our loss. To do that, we want the spinoff of our loss operate with respect to every parameter. Calculating this immediately from the loss is extraordinarily tough although — in spite of everything, our MSE is calculated by way of the worth predicted by our neural internet, which might be a very difficult operate.
That is the place very helpful piece of arithmetic — the chain rule — comes into play. As a substitute of being pressured to compute our extremely complicated derivatives from the get-go, we are able to as a substitute compute a collection of less complicated derivatives.
It seems that the chain rule meshes very effectively with our recursive tree construction. The thought mainly works as follows: assuming that we’ve easy sufficient elementary operations, every elementary operation is aware of its spinoff with respect to all of its arguments. Given the spinoff from the father or mother operation, we are able to thus compute the spinoff of every baby operation with respect to the loss operate by way of easy multiplication. For a easy linear regression mannequin utilizing MSE, we are able to diagram it as follows:
After all, a few of our nodes don’t do something with their derivatives — particularly, solely our leaf nodes care. However now every node can get the spinoff of its output with respect to the loss operate by way of this recursive course of. We are able to thus add the next strategies to our NeuralNetNode class:
def grad(self) -> Listing[float]:
"""The gradient of this node with respect to its inputs"""
increase NotImplementedErrordef backward(self, derivative_from_parent: float):
"""Propagate the spinoff from the father or mother to the kids"""
self.on_backward(derivative_from_parent)
deriv_wrt_children = self.grad()
for baby, derivative_wrt_child in zip(self.youngsters, deriv_wrt_children):
baby.backward(derivative_from_parent * derivative_wrt_child)
def on_backward(self, derivative_from_parent: float):
"""Hook for subclasses to override. Issues like updating parameters"""
cross
Train 1: Strive creating considered one of these bushes for a easy linear regression mannequin and carry out the recursive gradient updates by hand for a few steps.
Observe: For simplicity’s sake, we require our nodes to have just one father or mother (or none in any respect). If every node is allowed to have a number of dad and mom, our backwards() algorithm turns into considerably extra difficult as every baby must sum the spinoff of its dad and mom to compute its personal. We are able to do that iteratively with a topological kind (e.g. see here) or nonetheless recursively, i.e. with reverse accumulation (although on this case we would wish to do a second cross to really replace the entire parameters). This isn’t terribly tough, so I’ll go away it as an train to the reader (and can speak about it extra partially 2, keep tuned).
Constructing Fashions
The remainder of our code actually simply entails implementing parameters, inputs, and operations, and naturally working our coaching. Parameters and inputs are pretty easy constructs:
import random@dataclass
class Enter(NeuralNetNode):
"""A leaf node that represents an enter to the community"""
worth: float=0.0
def op(self, x):
return self.worth
def grad(self) -> Listing[float]:
return [1.0]
def __repr__(self):
return f'{self.__class__.__name__}({self.worth})'
@dataclass
class Parameter(NeuralNetNode):
"""A leaf node that represents a parameter to the community"""
worth: float=discipline(default_factory=lambda: random.uniform(-1, 1))
learning_rate: float=0.01
def op(self, x):
return self.worth
def grad(self):
return [1.0]
def on_backward(self, derivative_from_parent: float):
self.worth -= derivative_from_parent * self.learning_rate
def __repr__(self):
return f'{self.__class__.__name__}({self.worth})'
Operations are barely extra difficult, although not an excessive amount of so — we simply have to calculate their gradients correctly. Under are implementations of some helpful operations:
import math@dataclass
class Operation(NeuralNetNode):
"""A node that performs an operation on its inputs"""
cross
@dataclass
class Add(Operation):
"""A node that provides its inputs"""
def op(self, x):
return sum(x)
def grad(self):
return [1.0] * len(self.youngsters)
@dataclass
class Multiply(Operation):
"""A node that multiplies its inputs"""
def op(self, x):
return math.prod(x)
def grad(self):
grads = []
for i in vary(len(self.youngsters)):
cur_grad = 1
for j in vary(len(self.youngsters)):
if i == j:
proceed
cur_grad *= self.youngsters[j].ahead()
grads.append(cur_grad)
return grads
@dataclass
class ReLU(Operation):
"""
A node that applies the ReLU operate to its enter.
Observe that this could solely have one baby.
"""
def op(self, x):
return max(0, x[0])
def grad(self):
return [1.0 if self.children[0].ahead() > 0 else 0.0]
@dataclass
class Sigmoid(Operation):
"""
A node that applies the sigmoid operate to its enter.
Observe that this could solely have one baby.
"""
def op(self, x):
return 1 / (1 + math.exp(-x[0]))
def grad(self):
return [self.forward() * (1 - self.forward())]
The operation superclass right here isn’t helpful but, although we are going to want it to extra simply discover our mannequin’s inputs later.
Discover how usually the gradients of the features require the values from their youngsters, therefore we require calling the kid’s ahead() methodology. We’ll contact upon this extra in a little bit bit.
Defining a neural community in our framework is a bit verbose however is similar to developing a tree. Right here, as an example, is code for a easy linear classifier in our framework:
linear_classifier = Add([
Multiply([
Parameter(),
Input()
]),
Parameter()
])
Utilizing Our Fashions
To run a prediction with our mannequin, we’ve to first populate the inputs in our tree after which name ahead() on the father or mother. To populate the inputs although, we first want to seek out them, therefore we add the next methodology to our Operation class (we don’t add this to our NeuralNetNode class because the Enter sort isn’t outlined there but):
def find_input_nodes(self) -> Listing[Input]:
"""Discover the entire enter nodes within the subtree rooted at this node"""
input_nodes = []
for baby in self.youngsters:
if isinstance(baby, Enter):
input_nodes.append(baby)
elif isinstance(baby, Operation):
input_nodes.lengthen(baby.find_input_nodes())
return input_nodes
We are able to now add the predict() methodology to the Operation class:
def predict(self, inputs: Listing[float]) -> float:
"""Consider the community on the given inputs"""
input_nodes = self.find_input_nodes()
assert len(input_nodes) == len(inputs)
for input_node, worth in zip(input_nodes, inputs):
input_node.worth = worth
return self.ahead()
Train 2: The present means we applied predict() is considerably inefficient since we have to traverse the tree to seek out all of the inputs each time we run predict(). Write a compile() methodology that caches the operation’s inputs when it’s run.
Coaching our fashions is now very easy:
from typing import Callable, Tupledef train_model(
mannequin: Operation,
loss_fn: Callable[[float, float], float],
loss_grad_fn: Callable[[float, float], float],
information: Listing[Tuple[List[float], float]],
epochs: int=1000,
print_every: int=100
):
"""Prepare the given mannequin on the given information"""
for epoch in vary(epochs):
total_loss = 0.0
for x, y in information:
prediction = mannequin.predict(x)
total_loss += loss_fn(y, prediction)
mannequin.backward(loss_grad_fn(y, prediction))
if epoch % print_every == 0:
print(f'Epoch {epoch}: loss={total_loss/len(information)}')
Right here, as an example, is how we might practice a linear Fahrenheit to Celsius classifier utilizing our framework:
def mse_loss(y_true: float, y_pred: float) -> float:
return (y_true - y_pred) ** 2def mse_loss_grad(y_true: float, y_pred: float) -> float:
return -2 * (y_true - y_pred)
def fahrenheit_to_celsius(x: float) -> float:
return (x - 32) * 5 / 9
def generate_f_to_c_data() -> Listing[List[float]]:
information = []
for _ in vary(1000):
f = random.uniform(-1, 1)
information.append([[f], fahrenheit_to_celsius(f)])
return information
linear_classifier = Add([
Multiply([
Parameter(),
Input()
]),
Parameter()
])
train_model(linear_classifier, mse_loss, mse_loss_grad, generate_f_to_c_data())
After working this, we get
print(linear_classifier)
print(linear_classifier.predict([32]))>> Add(youngsters=[Multiply(children=[Parameter(0.5555555555555556), Input(0.8930639016107234)]), Parameter(-17.777777777777782)])
>> -1.7763568394002505e-14
Which appropriately corresponds to a linear classifier with weight 0.56, bias -17.78 (which is the Fahrenheit to Celsius formulation)
We are able to, after all, additionally practice far more complicated fashions, e.g. right here is one for predicting if a degree (x, y) is above or under the road y = x:
def bce_loss(y_true: float, y_pred: float, eps: float=0.00000001) -> float:
y_pred = min(max(y_pred, eps), 1 - eps)
return -y_true * math.log(y_pred) - (1 - y_true) * math.log(1 - y_pred)def bce_loss_grad(y_true: float, y_pred: float, eps: float=0.00000001) -> float:
y_pred = min(max(y_pred, eps), 1 - eps)
return (y_pred - y_true) / (y_pred * (1 - y_pred))
def generate_binary_data():
information = []
for _ in vary(1000):
x = random.uniform(-1, 1)
y = random.uniform(-1, 1)
information.append([(x, y), 1 if y > x else 0])
return information
model_binary = Sigmoid(
[
Add(
[
Multiply(
[
Parameter(),
ReLU(
[
Add(
[
Multiply(
[
Parameter(),
Input()
]
),
Multiply(
[
Parameter(),
Input()
]
),
Parameter()
]
)
]
)
]
),
Parameter()
]
)
]
)
train_model(model_binary, bce_loss, bce_loss_grad, generate_binary_data())
Then we fairly get
print(model_binary.predict([1, 0]))
print(model_binary.predict([0, 1]))
print(model_binary.predict([0, 1000]))
print(model_binary.predict([-5, 3]))
print(model_binary.predict([0, 0]))>> 3.7310797619230176e-66
>> 0.9997781079343139
>> 0.9997781079343139
>> 0.9997781079343139
>> 0.23791579184662365
Although this has affordable runtime, it’s considerably slower than we might count on. It is because we’ve to name ahead() and re-calculate the mannequin inputs rather a lot within the name to backwards(). As such, have the next train:
Train 3: Add caching to our community. That’s, on the decision to ahead(), the mannequin ought to return the cached worth from the earlier name to ahead() if and provided that the inputs haven’t modified because the final name. Make sure that you run ahead() once more if the inputs have modified.
And that’s about it! We now have a working neural community framework wherein we are able to practice simply a number of fascinating fashions (although not networks with nodes that feed into a number of different nodes. This isn’t too tough so as to add — see the be aware within the dialogue of the chain rule), although granted it’s a bit verbose. In case you’d wish to make it higher, attempt among the following:
Train 4: When you concentrate on it, extra “complicated” nodes in our community (e.g. Linear layers) are actually simply “macros” in a way — that’s, if we had a neural internet tree that regarded, say, as follows:
what you might be actually doing is that this:
In different phrases, Linear(inp) is de facto only a macro for a tree containing |inp| + 1 parameters, the primary of that are weights in multiplication and the final of which is a bias. Every time we see Linear(inp), we are able to thus substitute it for an equal tree composed solely of elementary operations.
For this train, your job is thus to implement the Macro class. The category needs to be an Operation that recursively replaces itself with elementary operations
Observe: this step might be performed each time, although it’s seemingly best so as to add a compile() methodology to the Operation class that it’s important to name earlier than coaching (or add it to your present methodology from Train 2). We are able to, after all, additionally implement extra complicated nodes in different (maybe extra environment friendly) methods, however that is nonetheless train.
Train 5: Although we don’t actually ever want inner nodes to supply something apart from one quantity as their output, it’s typically good for the basis of our tree (that’s, our output layer) to supply one thing else (e.g. an inventory of numbers within the case of a Softmax). Implement the Output class and permit it to supply a Listof[float] as a substitute of only a float. As a bonus, attempt implementing the SoftMax output.
Observe: there are a number of methods of doing this. You may make Output lengthen Operation, after which modify the NeuralNetNode class’ op() methodology to return a Listing[float] as a substitute of only a float. Alternatively, you might create a brand new Node superclass that each Output and Operation lengthen. That is seemingly simpler.
Observe additional that though these outputs can produce lists, they may nonetheless solely get one spinoff again from the loss operate — the loss operate will simply occur to take an inventory of floats as a substitute of a float (e.g. the Categorical Cross Entropy loss)
Train 6: Bear in mind how earlier within the article we mentioned that neural nets are simply mathematical features comprised of elementary operations? Add the funcify() methodology to the NeuralNetNode class that turns it into such a operate written in human-readable notation (add parentheses as you please). For instance, the neural internet Add([Parameter(0.1), Parameter(0.2)]) ought to collapse to “0.1 + 0.2” (or “(0.1 + 0.2)”).
Observe: For this to work, inputs needs to be named. In case you did train 2, identify your inputs within the compile() operate. If not, you’ll have to determine a strategy to identify your inputs — writing a compile() operate remains to be seemingly the simplest means.
Train 7: Modify our framework to permit nodes to have a number of dad and mom. I’ll remedy this partially 2.
That’s all for now! In case you’d like to take a look at the code, you’ll be able to take a look at this google colab that has all the things (apart from options to each train however #6, although I could add these partially 2).
Contact me at mchak@calpoly.edu for any inquiries.
Except in any other case specified, all pictures are by the creator.