LLM Positive-Tuning and Mannequin Choice Utilizing Neptune and Transformers
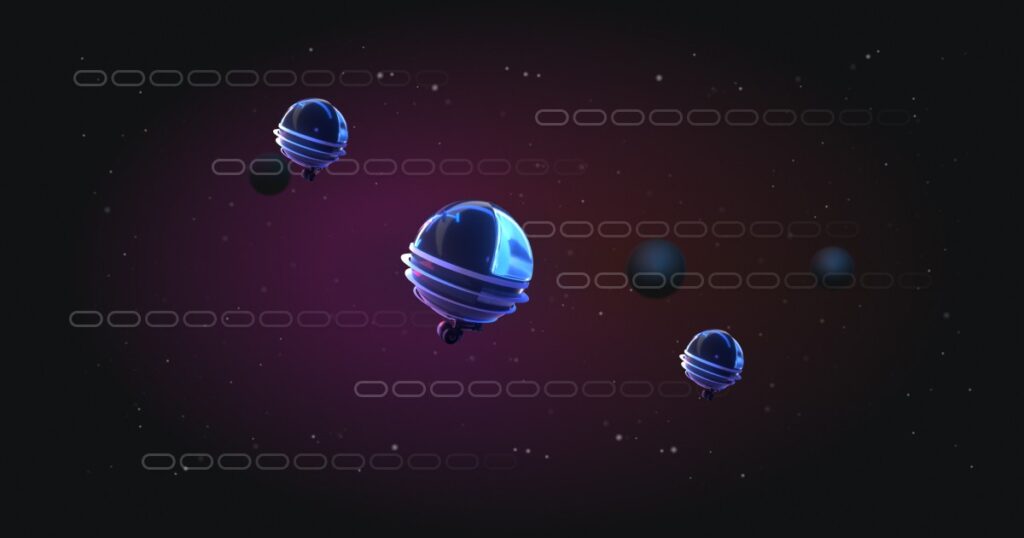
Think about you’re dealing with the next problem: you wish to develop a Massive Language Mannequin (LLM) that may proficiently reply to inquiries in Portuguese. You might have a useful dataset and may select from numerous base fashions. However right here’s the catch — you’re working with restricted computational assets and may’t depend on costly, high-power machines for fine-tuning. How do you determine on the precise mannequin to make use of on this state of affairs?
This put up explores these questions, providing insights and methods for selecting the right mannequin and conducting environment friendly fine-tuning, even when assets are constrained. We’ll have a look at methods to cut back a mannequin’s reminiscence footprint, pace up coaching, and greatest practices for monitoring.

Massive language fashions
Large Language Models (LLMs) are big deep-learning fashions pre-trained on huge knowledge. These fashions are often based mostly on an architecture called transformers. Not like the sooner recurrent neural networks (RNN) that sequentially course of inputs, transformers course of whole sequences in parallel. Initially, the transformer structure was designed for translation duties. However these days, it’s used for numerous duties, starting from language modeling to pc imaginative and prescient and generative AI.
Beneath, you possibly can see a primary transformer structure consisting of an encoder (left) and a decoder (proper). The encoder receives the inputs and generates a contextualized interpretation of the inputs, referred to as embeddings. The decoder makes use of the data within the embeddings to generate the mannequin’s output, one token at a time.

Palms-on: fine-tuning and deciding on an LLM for Brazilian Portuguese
On this mission, we’re taking up the problem of fine-tuning three LLMs: GPT-2, GPT2-medium, GPT2-large, and OPT 125M. The fashions have 137 million, 380 million, 812 million, and 125 million parameters, respectively. The most important one, GPT2-large, takes up over 3GB when saved on disk. All these fashions have been skilled to generate English-language textual content.
Our objective is to optimize these fashions for enhanced efficiency in Portuguese query answering, addressing the rising demand for AI capabilities in various languages. To perform this, we’ll must have a dataset with inputs and labels and use it to “train” the LLM. Taking a pre-trained mannequin and specializing it to unravel new duties is known as fine-tuning. The principle benefit of this method is you possibly can leverage the information the mannequin has to make use of as a place to begin.
Establishing
I’ve designed this mission to be accessible and reproducible, with a setup that may be replicated on a Colab atmosphere utilizing T4 GPUs. I encourage you to comply with alongside and experiment with the fine-tuning course of your self.
Word that I used a V100 GPU to provide the examples under, which is on the market when you have a Colab Professional subscription. You may see that I’ve already made a primary trade-off between money and time spent right here. Colab doesn’t reveal detailed costs, however a T4 costs $0.35/hour on the underlying Google Cloud Platform, while a V100 costs $2.48/hour. Based on this benchmark, a V100 is 3 times sooner than a T4. Thus, by spending seven occasions extra, we save two-thirds of our time.
You could find all of the code in two Colab notebooks:
We are going to use Python 3.10 in our codes. Earlier than we start, we’ll set up all of the libraries we are going to want. Don’t fear when you’re not accustomed to them but. We’ll go into their objective intimately once we first use them:
Loading and pre-processing the dataset
We’ll use the FaQuAD dataset to fine-tune our fashions. It’s a Portuguese question-answering dataset out there within the Hugging Face dataset assortment.
First, we’ll have a look at the dataset card to know how the dataset is structured. Now we have about 1,000 samples, every consisting of a context, a query, and a solution. Our mannequin’s process is to reply the query based mostly on the context. (The dataset additionally incorporates a title and an ID column, however we gained’t use them to fine-tune our mannequin.)

We will conveniently load the dataset utilizing the Hugging Face `datasets` library:
Our subsequent step is to transform the dataset right into a format our fashions can course of. For our question-answering process, that’s a sequence-to-sequence format: The mannequin receives a sequence of tokens because the enter and produces a sequence of tokens because the output. The enter incorporates the context and the query, and the output incorporates the reply.
For coaching, we’ll create a so-called immediate that incorporates not solely the query and the context but additionally the reply. Utilizing a small helper perform, we concatenate the context, query, and reply, divided by part headings (Later, we’ll miss the reply and ask the mannequin to fill within the “Resposta” part by itself).
We’ll additionally put together a helper perform that wraps the tokenizer. The tokenizer is what turns the textual content right into a sequence of integer tokens. It’s particular to every mannequin, so we’ll need to load and use a distinct tokenizer for every. The helper perform makes that course of extra manageable, permitting us to course of your complete dataset without delay utilizing map. Final, we’ll shuffle the dataset to make sure the mannequin sees it in randomized order.
Right here’s the whole code:
Loading and making ready the fashions
Subsequent, we load and put together the fashions that we’ll fine-tune. LLMs are big fashions. With none type of optimization, for the GPT2-large mannequin in full precision (float32), we now have round 800 million parameters, and we’d like 2.9 GB of reminiscence to load the mannequin and 11.5 GB through the coaching to deal with the gradients. That almost matches within the 16 GB of reminiscence that the T4 within the free tier affords. However we might solely be capable of compute tiny batches, making coaching painfully sluggish.
Confronted with these reminiscence and compute useful resource constraints, we’ll not use the fashions as-is however use quantization and a way referred to as LoRA to cut back their variety of trainable parameters and reminiscence footprint.
Quantization
Quantization is a method used to cut back a mannequin’s measurement in reminiscence by utilizing fewer bits to characterize its parameters. For instance, as an alternative of utilizing 32 bits to characterize a floating level quantity, we’ll use solely 16 and even as little as 4 bits.
This strategy can considerably lower the reminiscence footprint of a mannequin, which is very essential when deploying giant fashions on units with restricted reminiscence or processing energy. By decreasing the precision of the parameters, quantization can result in a sooner inference time and decrease energy consumption. Nonetheless, it’s important to steadiness the extent of quantization with the potential loss within the mannequin’s process efficiency, as extreme quantization can degrade accuracy or effectiveness.
The Hugging Face `transformers` library has built-in support for quantization by the `bitsandbytes` library. You may cross
or
mannequin loading strategies to load a mannequin with 8-bit or 4-bit precision, respectively.
After loading the mannequin, we name the wrapper perform `prepare_model_for_kbit_training` from the `peft` library. It prepares the mannequin for coaching in a means that saves reminiscence. It does this by freezing the mannequin parameters, ensuring all elements use the identical sort of knowledge format, and utilizing a particular method referred to as gradient checkpointing if the mannequin can deal with it. This helps in coaching giant AI fashions, even on computer systems with little reminiscence.
After quantizing the mannequin to eight bits, it takes solely a fourth of the reminiscence to load and practice the mannequin, respectively. For GPT2-large, as an alternative of needing 2.9 GB to load, it now takes solely 734 MB.
LoRA
As we all know, Massive Language Fashions have a variety of parameters. Once we wish to fine-tune certainly one of these fashions, we often replace all of the mannequin’s weights. Which means we have to save all of the gradient states in reminiscence throughout fine-tuning, which requires virtually twice the mannequin measurement of reminiscence. Generally, when updating all parameters, we are able to mess up with what the mannequin already discovered, resulting in worse outcomes when it comes to generalization.
Given this context, a staff of researchers proposed a brand new method referred to as Low-Rank Adaptation (LoRA). This reparametrization technique goals to cut back the variety of trainable parameters by low-rank decomposition.
Low-rank decomposition approximates a big matrix right into a product of two smaller matrices, such that multiplying a vector by the 2 smaller matrices yields roughly the identical outcomes as multiplying a vector by the unique matrix. For instance, we may decompose a 3×3 matrix into the product of a 3×1 and a 1×3 matrix in order that as an alternative of getting 9 parameters, we now have solely six.

When fine-tuning a mannequin, we wish to barely change its weights to adapt it to the brand new process. Extra formally, we’re searching for new weights derived from the unique weights: Wnew= Wprevious+ W. Taking a look at this equation, you possibly can see that we maintain the unique weights of their authentic form and simply study W as LoRA matrices.
In different phrases, you possibly can freeze your authentic weights and practice simply the 2 LoRA matrices with considerably fewer parameters in whole. Or, much more merely, you create a set of recent weights in parallel with the unique weights and solely practice the brand new ones. Throughout the inference, you cross your enter to each units of weights and sum them on the finish.

With our base mannequin loaded, we now wish to add the LoRA layers in parallel with the unique mannequin weights for fine-tuning. To do that, we have to outline a `LoraConfig`.
Contained in the `LoraConfig`, we are able to outline the rank of the LoRA matrices (parameter `r`), the dimension of the vector house generated by the matrix columns. We will additionally have a look at the rank as a measure of how a lot compression we’re making use of to our matrices, i.e., how small the bottleneck between A and B within the determine above will likely be.
When selecting the rank, protecting in thoughts the trade-off between the rank of your LoRA matrix and the educational course of is important. Smaller ranks imply much less room to study, i.e., as you have got fewer parameters to replace, it may be more durable to attain important enhancements. Alternatively, increased ranks present extra parameters, permitting for better flexibility and adaptableness throughout coaching. Nonetheless, this elevated capability comes at the price of extra computational assets and doubtlessly longer coaching occasions. Thus, discovering the optimum rank to your LoRA matrix that balances these elements effectively is essential, and one of the best ways to seek out that is by experimenting! A superb strategy is to start out with decrease ranks (8 or 16), as you’ll have fewer parameters to replace, so it will likely be sooner, and enhance it when you see the mannequin just isn’t studying as a lot as you need.
You additionally must outline which modules contained in the mannequin you wish to apply the LoRA method to. You may consider a module as a set of layers (or a constructing block) contained in the mannequin. If you wish to know extra, I’ve ready a deep dive, however be at liberty to skip it.
Inside the `LoraConfig`, that you must specify which modules to use LoRA to. You may apply LoRA for many of a mannequin’s modules, however that you must specify the module names that the unique builders assigned at mannequin creation. Which modules exist, and their names are completely different for every mannequin.
The LoRA paper experiences that including LoRA layers solely to the keys and values linear projections is an effective tradeoff in comparison with including LoRA layers to all linear projections in consideration blocks. In our case, for the GPT2 mannequin, we are going to apply LoRA on the `c_attn` layers, as we don’t have the question, worth, and keys weights cut up, and for the OPT mannequin, we are going to apply LoRA on the `q_proj` and `v_proj`.
In the event you use different fashions, you possibly can print the modules’ names and select those you need:
Along with specifying the rank and modules, you need to additionally arrange a hyperparameter referred to as `alpha`, which scales the LoRA matrix:
As a rule of thumb (as mentioned in this article by Sebastian Raschka), you can begin setting this to be two occasions the rank `r`. In case your outcomes aren’t good, you possibly can attempt decrease values.
Right here’s the whole LoRA configuration for our experiments:
We will apply this configuration to our mannequin by calling
Now, simply to point out what number of parameters we’re saving, let’s print the trainable parameters of GPT2-large:
We will see that we’re updating lower than 1% of the parameters! What an effectivity achieve!
Positive-tuning the fashions
With the dataset and fashions ready, it’s time to maneuver on to fine-tuning. Earlier than we begin our experiments, let’s take a step again and think about our strategy. We’ll be coaching 4 completely different fashions with completely different modifications and utilizing completely different coaching parameters. We’re not solely within the mannequin’s efficiency but additionally need to work with constrained assets.
Thus, it will likely be essential that we maintain observe of what we’re doing and progress as systematically as doable. At any time limit, we wish to be certain that we’re shifting in the precise course and spending our money and time properly.
What is important to log and monitor through the fine-tuning course of?
Apart from monitoring customary metrics like coaching and validation loss and coaching parameters resembling the educational price, in our case, we additionally need to have the ability to log and monitor different elements of the fine-tuning:
- Useful resource Utilization: Because you’re working with restricted computational assets, it’s important to maintain a detailed eye on GPU and CPU utilization, reminiscence consumption, and disk utilization. This ensures you’re not overtaxing your system and may help troubleshoot efficiency points.
- Mannequin Parameters and Hyperparameters: To make sure that others can replicate your experiment, storing all the small print concerning the mannequin setup and the coaching script is essential. This consists of the structure of the mannequin, such because the sizes of the layers and the dropout charges, in addition to the hyperparameters, just like the batch measurement and the variety of epochs. Retaining a file of those components is essential to understanding how they have an effect on the mannequin’s efficiency and permitting others to recreate your experiment precisely.
- Epoch Period and Coaching Time: Report the length of every coaching epoch and the full coaching time. This knowledge helps assess the time effectivity of your coaching course of and plan future useful resource allocation.
Arrange logging with neptune.ai
neptune.ai is a machine studying experiment tracker and mannequin registry. It affords a single place to log, evaluate, retailer, and collaborate on experiments and fashions. Neptune is built-in with the `transformers` library’s `Coach` module, permitting you to log and monitor your mannequin coaching seamlessly. This integration was contributed by Neptune’s builders, who keep it to this present day.
To make use of Neptune, you’ll have to join an account first (don’t fear, it’s free for private use) and create a mission in your workspace. Take a look at the Quickstart guide in Neptune’s documentation. There, you’ll additionally discover up-to-date directions for acquiring the mission and token IDs you’ll want to attach your Colab atmosphere to Neptune.
We’ll set these as atmosphere variables:
There are two choices for logging data from `transformer` coaching to Neptune: You may both set `report_to=”neptune”` within the `TrainingArguments` or cross an occasion of `NeptuneCallback` to the `Coach`’s `callbacks` parameter. I favor the second choice as a result of it provides me extra management over what I log. Word that when you cross a logging callback, you need to set `report_to=”none` within the `TrainingArguments` to keep away from duplicate knowledge being reported.
Beneath, you possibly can see how I sometimes instantiate the `NeptuneCallback`. I specified a reputation for my experiment run and requested Neptune to log all parameters used and the {hardware} metrics. Setting `log_checkpoints=”final”` ensures that the final mannequin checkpoint can even be saved on Neptune.
Coaching a mannequin
Because the final step earlier than configuring the `Coach`, it’s time to tokenize the dataset with the mannequin’s tokenizer. Since we’ve loaded the tokenizer along with the mannequin, we are able to now put the helper perform we ready earlier into motion:
The coaching is managed by a `Coach` object. The `Coach` makes use of a `DataCollatorForLanguageModeling`, which prepares the info in a means appropriate for language mannequin coaching.
Right here’s the total setup of the `Coach`:
That’s a variety of code, so let’s undergo it intimately:
- The coaching course of is outlined to run for 20 epochs (EPOCHS = 20). You’ll doubtless discover that coaching for much more epochs will result in higher outcomes.
- We’re utilizing a method referred to as gradient accumulation, set right here to eight steps (GRADIENT_ACCUMULATION_STEPS = 8), which helps deal with bigger batch sizes successfully, particularly when reminiscence assets are restricted. In easy phrases, gradient accumulation is a method to deal with giant batches. As an alternative of getting a batch of 64 samples and updating the weights for each step, we are able to have a batch measurement of 8 samples and carry out eight steps, simply updating the weights within the final step. It generates the identical outcome as a batch of 64 however saves reminiscence.
- The MICRO_BATCH_SIZE is about to eight, indicating the variety of samples processed every step. This can be very essential to seek out an quantity of samples that may slot in your GPU reminiscence through the coaching to keep away from out-of-memory points (Take a look at the `transformers` documentation to study extra about this).
- The training price, an important hyperparameter in coaching neural networks, is about to 0.002 (LEARNING_RATE = 2e-3), figuring out the step measurement at every iteration when shifting towards a minimal of the loss perform. To facilitate a smoother and more practical coaching course of, the mannequin will regularly enhance its studying price for the primary 100 steps (WARMUP_STEPS = 100), serving to to stabilize early coaching phases.
- The coach is about to not use the mannequin’s cache (mannequin.config.use_cache = False) to handle reminiscence extra effectively.
With all of that in place, we are able to launch the coaching:
Whereas coaching is working, head over to Neptune, navigate to your mission, and click on on the experiment that’s working. There, click on on `Charts` to see how your coaching progresses (loss and studying price). To see useful resource utilization, click on the `Monitoring` tab and comply with how GPU and CPU utilization and reminiscence utilization change over time. When the coaching finishes, you possibly can see different data like coaching samples per second, coaching steps per second, and extra.
On the finish of the coaching, we seize the output of this course of in `trainer_output`, which usually consists of particulars concerning the coaching efficiency and metrics that we are going to later use to save lots of the mannequin on the mannequin registry.
However first, we’ll need to verify whether or not our coaching was profitable.
Evaluating the fine-tuned LLMs
Mannequin analysis in AI, significantly for language fashions, is a posh and multifaceted process. It entails navigating a sequence of trade-offs amongst value, knowledge applicability, and alignment with human preferences. This course of is crucial in making certain that the developed fashions aren’t solely technically proficient but additionally sensible and user-centric.
LLM analysis approaches

The chart above exhibits that the least costly (and mostly used) strategy is to make use of public benchmarks. On the one hand, this strategy is very cost-effective and straightforward to check. Nonetheless, alternatively, it’s much less more likely to resemble manufacturing knowledge. An alternative choice, barely extra pricey than benchmarks, is AutoEval, the place different language fashions are used to judge the goal mannequin. For these with a better price range, consumer testing, the place the mannequin is made accessible to customers, or human analysis, which entails a devoted staff of people centered on assessing the mannequin, is an choice.
Evaluating question-answering fashions with F1 scores and the precise match metric
In our mission, contemplating the necessity to steadiness cost-effectiveness with sustaining analysis requirements for the dataset, we are going to make use of two particular metrics: precise match and F1 rating. We’ll use the `validation` set supplied together with the FaQuAD dataset. Therefore, our analysis technique falls into the `Public Benchmarks` class, because it depends on a well known dataset to judge PTBR fashions.
The precise match metric determines if the response given by the mannequin exactly aligns with the goal reply. It is a simple and efficient method to assess the mannequin’s accuracy in replicating anticipated responses. We’ll additionally calculate the F1 rating, which mixes precision and recall, of the returned tokens. It will give us a extra nuanced analysis of the mannequin’s efficiency. By adopting these metrics, we intention to evaluate our mannequin’s capabilities reliably with out incurring important bills.
As we stated beforehand, there are numerous methods to judge an LLM, and we select this fashion, utilizing customary metrics, as a result of it’s quick and low cost. Nonetheless, there are some trade-offs when selecting “arduous” metrics to judge outcomes that may be appropriate, even when the metrics say it isn’t good.
One instance is: think about the goal reply for some query is “The rat discovered the cheese and ate it.” and the mannequin’s prediction is “The mouse found the cheese and consumed it.” Each examples have virtually the identical which means, however the phrases chosen differ. For metrics like precise match and F1, the scores will likely be actually low. A greater – however extra pricey – analysis strategy can be to have people annotate or use one other LLM to confirm if each sentences have the identical which means.
Implementing the analysis capabilities
Let’s return to our code. I’ve determined to create my very own analysis capabilities as an alternative of utilizing the `Coach`’s built-in capabilities to carry out the analysis. On the one hand, this provides us extra management. Alternatively, I regularly encountered out-of-memory (OOM) errors whereas doing evaluations straight with the `Coach`.
For our analysis, we’ll want two capabilities:
- `get_logits_and_labels`: Processes a pattern, generates a immediate from it, passes this immediate by a mannequin, and returns the mannequin’s logits (scores) together with the token IDs of the goal reply.
- `compute_metrics`: Evaluates a mannequin on a dataset, calculating precise match (EM) and F1 scores. It iterates by the dataset, utilizing the get_logits_and_labels perform to generate mannequin predictions and corresponding labels. Predictions are decided by deciding on the almost certainly token indices from the logits. For the EM rating, it decodes these predictions and labels into textual content and computes the EM rating. For the F1 rating, it maintains the unique token IDs and calculates the rating for every pattern, averaging them on the finish.
Right here’s the whole code:
Earlier than assessing our mannequin, we should swap it to analysis mode, which deactivates dropout. Moreover, we must always re-enable the mannequin’s cache to preserve reminiscence throughout prediction.
Following this setup, merely execute the `compute_metrics` perform on the analysis dataset and specify the specified variety of generated tokens to make use of (Word that utilizing extra tokens will enhance processing time).
Storing the fashions and analysis outcomes
Now that we’ve completed fine-tuning and evaluating a mannequin, we must always put it aside and transfer on to the following mannequin. To this finish, we’ll create a `model_version` to retailer in Neptune’s mannequin registry.
Intimately, we’ll save the most recent mannequin checkpoint together with the loss, the F1 rating, and the precise match metric. These metrics will later enable us to pick the optimum mannequin. To create a mannequin and a mannequin model, you have to to outline the mannequin key, which is the mannequin identifier and should be uppercase and distinctive inside the mission. After defining the mannequin key, to make use of this mannequin to create a mannequin model, that you must concatenate it with the mission identifier that yow will discover on Neptune below “All tasks” – “Edit mission data” – “Venture key”.
Mannequin choice
As soon as we’re performed with all our mannequin coaching and experiments, it’s time to collectively consider them. That is doable as a result of we monitored the coaching and saved all the data on Neptune. Now, we’ll use the platform to check completely different runs and fashions to decide on the very best one for our use case.
After finishing all of your runs, you possibly can click on `Examine runs` on the high of the mission’s web page and allow the “small eye” for the runs you wish to evaluate. Then, you possibly can go to the `Charts` tab, and you’ll find a joint plot of the losses for all of the experiments. Here’s how it looks in my project. In purple, we are able to see the loss for the gpt2-large mannequin. As we skilled for fewer epochs, we are able to see that we now have a shorter curve, which however achieved a greater loss.
The loss perform just isn’t but saturated, indicating that our fashions nonetheless have room for progress and will doubtless obtain increased ranges of efficiency with extra coaching time.
Go to the `Fashions` web page and click on on the mannequin you created. You will notice an summary of all of the variations you skilled and uploaded. It’s also possible to see the metrics reported and the mannequin title.
You’ll discover that not one of the mannequin variations have been assigned to a “Stage” but. Neptune means that you can assign models to different stages, particularly “Staging,” “Manufacturing,” and “Archived.”
Whereas we are able to promote a mannequin by the UI, we’ll return to our code and robotically determine the very best mannequin. For this, we first fetch all mannequin variations’ metadata, kind by the precise match and f1 scores, and promote the very best mannequin in accordance with these metrics to manufacturing:
After executing this, we are able to see, as anticipated, that gpt2-large (our largest mannequin) was the very best mannequin and was chosen to go to manufacturing:
As soon as extra, we’ll return to our code and eventually use our greatest mannequin to reply questions in Brazilian Portuguese:

Let’s evaluate the prediction with out fine-tuning and the prediction after fine-tuning. As demonstrated, earlier than fine-tuning, the mannequin didn’t know learn how to deal with Brazilian Portuguese in any respect and answered by repeating some a part of the enter or returning particular characters like “##########.” Nonetheless, after fine-tuning, it turns into evident that the mannequin handles the enter a lot better, answering the query appropriately (it solely added a “?” on the finish, however the remainder is precisely the reply we’d count on).
We will additionally have a look at the metrics earlier than and after fine-tuning and confirm how a lot it improved:
Given the metrics and the prediction instance, we are able to conclude that the fine-tuning was in the precise course, regardless that we now have room for enchancment.
Do you’re feeling like experimenting with neptune.ai?
Methods to enhance the answer?
On this article, we’ve detailed a easy and environment friendly method for fine-tuning LLMs.
In fact, we nonetheless have some method to go to attain good efficiency and consistency. There are numerous extra, extra superior methods you possibly can make use of, resembling:
- Extra Information: Add extra high-quality, various, and related knowledge to the coaching set to enhance the mannequin’s studying and generalization.
- Tokenizer Merging: Mix tokenizers for higher enter processing, particularly for multilingual fashions.
- Mannequin-Weight Tuning: Straight regulate the pre-trained mannequin weights to suit the brand new knowledge higher, which will be more practical than tuning adapter weights.
- Reinforcement Studying with Human Suggestions: Make use of human raters to supply suggestions on the mannequin’s outputs, which is used to fine-tune the mannequin by reinforcement studying, aligning it extra intently with advanced targets.
- Extra Coaching Steps: Growing the variety of coaching steps can additional improve the mannequin’s understanding and adaptation to the info.
Conclusion
We engaged in 4 distinct trials all through our experiments, every using a distinct mannequin. We’ve used quantization and LoRA to cut back the reminiscence and compute useful resource necessities. All through the coaching and analysis, we’ve used Neptune to log metrics and retailer and handle the completely different mannequin variations.
I hope this text impressed you to discover the probabilities of LLMs additional. Specifically, when you’re a local speaker of a language that’s not English, I’d prefer to encourage you to discover fine-tuning LLMs in your native tongue.