From Adaline to Multilayer Neural Networks | by Pan Cretan | Jan, 2024
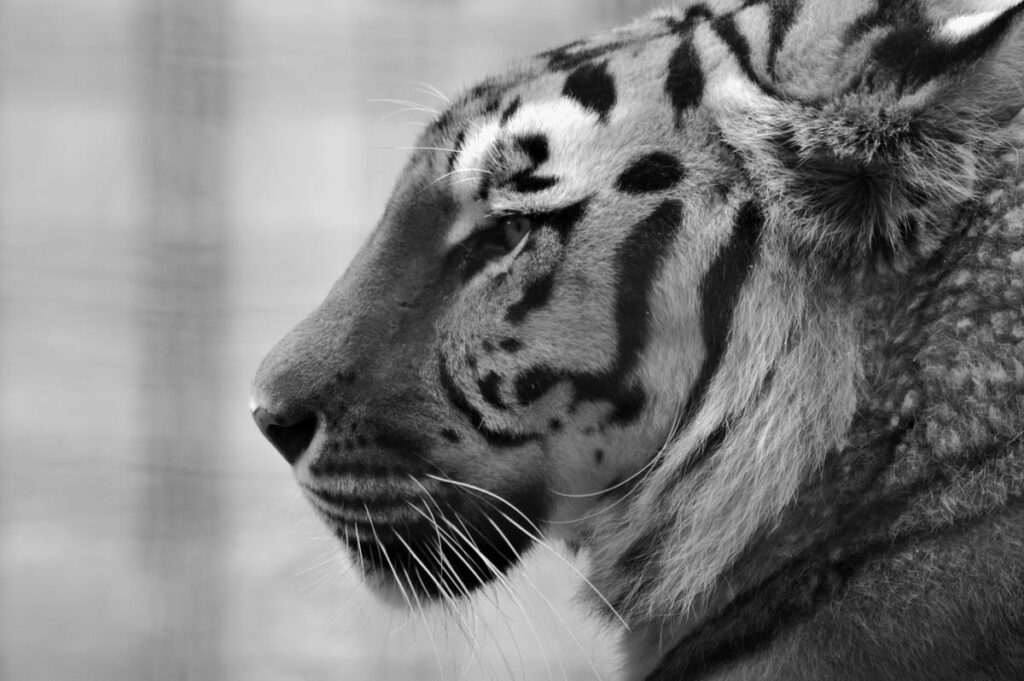
Within the earlier two articles we noticed how we are able to implement a primary classifier primarily based on Rosenblatt’s perceptron and the way this classifier could be improved by utilizing the adaptive linear neuron algorithm (adaline). These two articles cowl the foundations earlier than making an attempt to implement a synthetic neural community with many layers. Transferring from adaline to deep studying is a much bigger leap and plenty of machine studying practitioners will decide immediately for an open supply library like PyTorch. Utilizing such a specialised machine studying library is in fact beneficial for growing a mannequin in manufacturing, however not essentially for studying the elemental ideas of multilayer neural networks. This text builds a multilayer neural community from scratch. As an alternative of fixing a binary classification downside we are going to deal with a multiclass one. We shall be utilizing the sigmoid activation operate after every layer, together with the output one. Basically we prepare a mannequin that for every enter, comprising a vector of options, produces a vector with size equal to the variety of courses to be predicted. Every ingredient of the output vector is within the vary [0, 1] and could be understood because the “likelihood” of every class.
The aim of the article is to turn out to be comfy with the mathematical notation used for describing mathematically neural networks, perceive the position of the varied matrices with weights and biases, and derive the formulation for updating the weights and biases to minimise the loss operate. The implementation permits for any variety of hidden layers with arbitrary dimensions. Most tutorials assume a hard and fast structure however this text makes use of a rigorously chosen mathematical notation that helps generalisation. On this method we are able to additionally run easy numerical experiments to look at the predictive efficiency as a operate of the quantity and measurement of the hidden layers.
As within the earlier articles, I used the web LaTeX equation editor to develop the LaTeX code for the equation after which the chrome plugin Maths Equations Anywhere to render the equation into a picture. All LaTex code is offered on the finish of the article if that you must render it once more. Getting the notation proper is a part of the journey in machine studying, and important for understanding neural networks. It’s important to scrutinise the formulation, and take note of the varied indices and the principles for matrix multiplication. Implementation in code turns into trivial as soon as the mannequin is accurately formulated on paper.
All code used within the article could be discovered within the accompanying repository. The article covers the next subjects
∘ What is a multilayer neural network?
∘ Activation
∘ Loss function
∘ Backpropagation
∘ Implementation
∘ Dataset
∘ Training the model
∘ Hyperparameter tuning
∘ Conclusions
∘ LaTeX code of equations used in the article
What’s a multilayer neural community?
This part introduces the structure of a generalised, feedforward, fully-connected multilayer neural community. There are a variety of phrases to undergo right here as we work our method by means of Determine 1 under.
For each prediction, the community accepts a vector of options as enter
that may also be understood as a matrix with form (1, n⁰). The community makes use of L layers and produces a vector as an output
that may be understood as a matrix with form (1, nᴸ) the place nᴸ is the variety of courses within the multiclass classification downside we have to clear up. Each float on this matrix lies within the vary [0, 1] and the index of the biggest ingredient corresponds to the expected class. The (L) notation within the superscript is used to seek advice from a specific layer, on this case the final one.
However how will we generate this prediction? Let’s deal with the primary ingredient of the primary layer (the enter just isn’t thought-about a layer)
We first compute the web enter that’s basically an inside product of the enter vector with a set of weights with the addition of a bias time period. The second operation is the appliance of the activation operate σ(z) to which we are going to return later. For now it is very important needless to say the activation operate is actually a scalar operation.
We are able to compute all components of the primary layer in the identical method
From the above we are able to deduce that we now have launched n¹ x n⁰ weights and n¹ bias phrases that may should be fitted when the mannequin is educated. These calculations may also be expressed in matrix type
Pay shut consideration to the form of the matrices. The online output is a results of a matrix multiplication of two matrices with form (1, n⁰) and (n⁰, n¹) that ends in a matrix with form (1, n¹), to which we add one other matrix with the bias phrases that has the identical (1, n¹) form. Word that we launched the transpose of the load matrix. The activation operate applies to each ingredient of this matrix and therefore the activated values of layer 1 are additionally a matrix with form (1, n¹).
The above could be readily generalised for each layer within the neural community. Layer okay accepts as enter nᵏ⁻¹ values and produces nᵏ activated values
Layer okay introduces nᵏ x nᵏ⁻¹ weights and nᵏ bias phrases that may should be fitted when the mannequin is educated. The full variety of weights and bias phrases is
so if we assume an enter vector with 784 components (dimension of a low decision picture in grey scale), a single hidden layer with 50 nodes and 10 courses within the output we have to optimise 785*50+51*10 = 39,760 parameters. The variety of parameters grows additional if we enhance the variety of hidden layers and the variety of nodes in these layers. Optimising an goal operate with so many parameters just isn’t a trivial endeavor and for this reason it took a while from the time adaline was launched till we found how one can prepare deep networks within the mid 80s.
This part basically covers what is called the ahead cross, i.e. how we apply a sequence of matrix multiplications, matrix additions and ingredient sensible activations to transform the enter vector to an output vector. For those who pay shut consideration we assumed that the enter was a single pattern represented as a matrix with form (1, n⁰). The notation holds even when we we feed into the community a batch of samples represented as a matrix with form (N, n⁰). There’s solely small complexity in the case of the bias phrases. If we deal with the primary layer we sum a matrix with form (N, n¹) to a bias matrix with form (1, n¹). For this to work the bias matrix has its first row replicated as many occasions because the variety of samples within the batch we use within the ahead cross. That is such a pure operation that NumPy does it routinely in what known as broadcasting. Once we apply ahead cross to a batch of inputs it’s maybe cleaner to make use of capital letters for all vectors that turn out to be matrices, i.e.
Word that I assumed that broadcasting was utilized to the bias phrases resulting in a matrix with as many rows because the variety of samples within the batch.
Working with batches is typical with deep neural networks. We are able to see that because the variety of samples N will increase we are going to want extra reminiscence to retailer the varied matrices and perform the matrix multiplications. As well as, utilizing solely a part of coaching set for updating the weights means we shall be updating the parameters a number of occasions in every cross of the coaching set (epoch) resulting in quicker convergence. There’s a further profit that’s maybe much less apparent. The community makes use of activation capabilities that, in contrast to the activation in adaline, usually are not the identification. In truth they aren’t even linear, which makes the loss operate non convex. Utilizing batches introduces noise that’s believed to assist escaping shallow native minima. A suitably chosen studying price additional assists with this.
As a ultimate be aware earlier than we transfer on, the time period feedforward comes from the truth that every layer is utilizing as enter the output of the earlier layer with out utilizing loops that result in the so-called recurrent neural networks.
Activation
Enabling the neural community to resolve complicated downside requires introducing some type of nonlinearity. That is achieved by utilizing an activation operate in every layer. There are numerous selections. For this text we shall be utilizing the sigmoid (logistic) activation operate that we are able to visualise with
that produces
The code additionally contains all imports we are going to want all through the article.
The activation operate maps any float to the vary 0 to 1. In actuality the sigmoid is a extra appropriate activation of the ultimate layer for binary classification issues. For multiclass issues it might have been extra acceptable to make use of softmax to normalize the output of the neural community to a likelihood distribution over predicted output courses. A method to consider that is that softmax enforces that submit activation the sum of the entries of the output vector should add as much as 1, that isn’t the case with sigmoid. One other method to consider it’s that the sigmoid basically converts the logits (log odds) to a one-versus-all (OvA) likelihood. Nonetheless, we are going to use the sigmoid activation operate to remain as shut as doable to adaline as a result of the softmax just isn’t a component sensible operation and this can introduce some complexities within the again propagation algorithm. I go away this as an train for the reader.
Loss operate
The loss operate used for adaline was the imply sq. error. In observe a multiclass classification downside would use a multiclass cross-entropy loss. To be able to stay as near adaline as doable, and to facilitate the analytical calculation of the gradients of the loss operate with respect to the parameters, we are going to stick on the imply sq. error loss operate. Each pattern within the coaching set, belongs to one of many nᴸ courses and therefore the loss operate could be expressed as
the place the primary summation is over all samples and the second over courses. The above implies that the recognized class for pattern i has been transformed to a one-hot-encoding, i.e. a matrix with form (1, nᴸ) containing zeros aside from the ingredient that corresponds to the pattern class that’s one. We adopted yet one more notation conference in order that [j] within the superscript is used to seek advice from pattern j. The summation above doesn’t want to make use of all samples within the coaching set. In observe will probably be utilized in batches of N’ samples with N’<<N.
Backpropagation
The loss operate is a scalar that depends upon tens or a whole lot of 1000’s of parameters, comprising weights and bias phrases. Sometimes, these parameters are initialised with random numbers and are up to date iteratively in order that the loss operate is minimised utilizing the gradient of the loss operate with regard to every parameter. Within the case of adaline, the analytical derivation of the gradients was easy. For multilayer neural networks the derivation is extra concerned however stays tractable if we undertake a intelligent technique. We enter the world of the again propagation however concern not. Backpropagation basically boils right down to a successive software of the chain differentiation rule from the best to the left.
Let’s come again to the loss operate. It depends upon the activated values of the final layer, so we are able to first compute the derivatives with regard to these
The above could be understood because the (j, i) ingredient of a derivate matrix with form (N, nᴸ) and could be written in matrix type as
the place each matrices in the best hand facet have form (N, nᴸ). The activated values of the final layer are computed by making use of the sigmoid activation operate on every ingredient of the web enter matrix of the final layer. Therefore, to compute the derivatives of the loss operate with regard to every ingredient of this web enter matrix of the final layer we merely must remind ourselves on how one can compute the by-product of a nested operate with the outer one being the sigmoid operate:
The star multiplication denotes ingredient sensible multiplication. The results of this components is a matrix with form (N, nᴸ). In case you have difficulties computing the by-product of the sigmoid operate please verify here.
We at the moment are able to compute the by-product of the loss operate with regard to the weights of the L-1 layer; that is the primary set of weights we encounter after we transfer from proper to left
This results in a matrix with the identical form because the weights of the L-1 layer. We subsequent must compute the by-product of the web enter of the L layer with regard to the weights of the L-1 layer. If we decide one ingredient of the web enter matrix of the final layer and one in all these weights we now have
In case you have hassle to grasp the above, assume that for each pattern j, the i ingredient of the web enter of the L layer solely depends upon the weights of the L-1 layer for which the primary index can be i. Therefore, we are able to get rid of one of many summations within the by-product
We are able to specific all these derivatives in a matrix notation utilizing
Basically the implicit summation within the matrix multiplication absorbs the summation over the samples. Comply with together with the shapes of the multiplied matrices and you will note that the ensuing by-product matrix has the identical form as the load matrix used to calculate the web enter of the L layer. Though the variety of components within the ensuing matrix is restricted to the product of the variety of nodes of the final two layers (the form is (nᴸ, nᴸ⁻¹)), the multiplied matrices are a lot bigger and therefore are sometimes extra reminiscence consuming. Therefore, the necessity to use batches when coaching the mannequin.
The derivatives of the loss operate with respect to the bias phrases used for calculating the web enter of the final layer could be computed equally as for the weights to provide
that results in a matrix with form (1, nᴸ).
We’ve simply computed all derivatives of the loss operate with regard to the weights and bias phrases used for computing the web enter of the final layer. We now flip our consideration to the gradients with the regard to the weights and bias phrases of the earlier layer (these parameters could have the superscript index L-2). Hopefully we are able to begin figuring out patterns in order that we are able to apply them to compute the derivates with regard to the weights and bias phrases for okay=0,..,L-2. We might see these patterns emerge if we compute the by-product of the loss operate with regard to the activated values of the L-1 layer. These ought to type a matrix with form (N, nᴸ⁻¹) that’s computed as
As soon as we now have the derivatives of the loss with regard to the activated values of layer L-1 we are able to proceed with calculating the derivatives of the loss operate with regard to the web enter of the layer L-1 after which with regard to the weights and bias phrases with index L-2.
Let’s recap how we backpropagate by one layer. We assume we now have computed the by-product of the loss operate with regard to the weights and bias phrases with index okay and we have to compute the derivates of the loss operate with regard to the weights and bias phrases with index k-1. We have to perform 4 operations:
All operations are vectorised. We are able to already begin imaging how we might implement these operations in a category. My understanding is that when one makes use of a specialised library so as to add a completely related linear layer with an activation operate, that is what occurs behind the scenes! It’s good to not fear in regards to the mathematical notation, however my suggestion could be to undergo these derivations not less than as soon as.
Implementation
On this part we offer the implementation of a generalised, feedforward, multilayer neural community. The API attracts some analogies to the one present in specialised deep studying libraries akin to PyTorch
The code comprises two utility capabilities: sigmoid()
applies the sigmoid (logistic) activation operate to a float (or NumPy array), and int_to_onehot()
takes an inventory of integers with the category of every pattern and returns their one-hot-encoding illustration.
The category MultilayerNeuralNetClassifier
comprises the neural web implementation. The initialisation constructor assigns random numbers to the weights and bias phrases of every layer. For example if we assemble a neural community with layers=[784, 50, 10]
, we shall be utilizing 784 enter options, a hidden layer with 50 nodes and 10 courses as output. This generalised implementation permits altering each the variety of hidden layers and the variety of nodes within the hidden layers. We are going to exploit this after we do hyperparameter tuning in a while. For reproducibility we use a seed for the random quantity generator to initialise the weights.
The ahead
technique returns the activated values for every layer as an inventory of matrices. The strategy works with a single pattern or an array of samples. The final of the returned matrices comprises the mannequin predictions for the category membership of every pattern. As soon as the mannequin is educated solely this matrix is used for making predictions. Nevertheless, while the mannequin is being educated we’d like the activated values for all layers as we are going to see under and for this reason the ahead
technique returns all of them. Assuming that the community was initialised with layers=[784, 50, 10]
, the ahead
technique will return an inventory of two matrices, the primary one with form (N, 50) and the second with form (N, 10), assuming the enter x
has N samples, i.e. it’s a matrix with form (N, 784).
The backward
technique implements backpropagation, i.e. all of the analytically computed derivatives of the loss operate as described within the earlier part. The final layer is particular as a result of we have to compute the derivatives of the loss operate with regard to the mannequin output utilizing the recognized courses. The primary layer is particular as a result of we have to use the enter as a substitute of the activated values of the earlier layer. The center layers are all the identical. We merely iterate over the layers backwards. The code displays totally the analytically derived formulation. Through the use of NumPy we vectorise all operations that hastens execution. The strategy returns a tuple of two lists. The primary record comprises the matrices with the derivatives of the loss operate with regard to the weights of every layer. Assuming that the community was initialised with layers=[784, 50, 10]
, the record will include two matrices with shapes (784, 50) and (50, 10). The second record comprises the vectors with the derivatives of the loss operate with regard to the bias phrases of every layer. Assuming that the community was initialised with layers=[784, 50, 10]
, the record will include two vectors with shapes (50, ) and (10,).
Reflecting again on my learnings from this text, I felt that the implementation was easy. The toughest half was to give you a sturdy mathematical notation and work out the gradients on paper. Nonetheless, it’s straightforward to make errors that will not be straightforward to detect even when the optimisation appears to converge. This brings me to the particular backward_numerical
technique. This technique is used for neither coaching the mannequin nor making predictions. It makes use of finite (central) variations to estimate the derivatives of the loss operate with regard to the weights and bias phrases of the chosen layer. The numerical derivatives could be in contrast with the analytically computed ones returned by the backward
operate to make sure that the implementation is appropriate. This technique could be too sluggish for use for coaching the mannequin because it requires two ahead passes for every by-product and in our trivial instance with layers=[784, 50, 10]
there are 39,760 such derivatives! However it’s a lifesaver. Personally I’d not have managed to debug the code with out it. If you wish to preserve a key message from this text, it might be the usefulness of numerical differentiation for double checking your analytically derived gradients. We are able to verify the correctness of the gradients with an untrained mannequin
that produces
layer 3: 300 out of 300 weight gradients are numerically equal
layer 3:10 out of 10 bias time period gradients are numerically equal
layer 2: 1200 out of 1200 weight gradients are numerically equal
layer 2:30 out of 30 bias time period gradients are numerically equal
layer 1: 2000 out of 2000 weight gradients are numerically equal
layer 1:40 out of 40 bias time period gradients are numerically equal
Gradients look so as!
Dataset
We are going to want a dataset for constructing our first mannequin. A well-known one usually utilized in sample recognition experiments is the MNIST handwritten digits. We are able to discover extra particulars about this dataset within the OpenML dataset repository. All datasets in OpenML are subject to the CC BY 4.0 license that allows copying, redistributing and reworking the fabric in any medium and for any function.
The dataset comprises 70,000 digit pictures and the corresponding labels. Conveniently, the digits have been size-normalized and centered in a fixed-size 28×28 picture by computing the middle of mass of the pixels, and translating the picture in order to place this level on the middle of the 28×28 subject. The dataset could be conveniently retrieved utilizing scikit-learn
that prints
unique X: X.form=(70000, 784), X.dtype=dtype('int64'), X.min()=0, X.max()=255
unique y: y.form=(70000,), y.dtype=dtype('O')
processed X: X.form=(70000, 784), X.dtype=dtype('float64'), X.min()=-1.0, X.max()=1.0
processed y: y.form=(70000,), y.dtype=dtype('int32')
class counts: 0:6903, 1:7877, 2:6990, 3:7141, 4:6824, 5:6313, 6:6876, 7:7293, 8:6825, 9:6958
We are able to see that every picture is obtainable as a vector with 784 integers between 0 and 255 that have been transformed to floats within the vary [-0.5, 0.5]. That is maybe a bit totally different than the everyday characteristic scaling in scikit-learn the place scaling occurs per characteristic moderately per pattern. The category labels have been retrieved as strings and transformed to integers. The dataset is fairly balanced.
We subsequent visualise ten pictures for every digit to acquire a sense on the variations in hand writing
that produces
We are able to foresee that some digits could also be confused by the mannequin, e.g. the final 9 resembles 8. There may be hand writing variations that aren’t predicted properly, akin to 7 digits written with a horizontal line within the center, relying on how usually such variations are represented within the coaching set. We now have a neural community implementation and a dataset to make use of it with. Within the subsequent part we are going to present the required code for coaching the mannequin earlier than we glance into hyperparameter tuning.
Coaching the mannequin
The primary motion we have to take is to separate the dataset right into a coaching set, and an exterior (hold-out) check set. We are able to readily achieve this utilizing scikit-learn
We use stratification in order that the share of every class is roughly equal in each the coaching set and the exterior (hold-out) dataset. The exterior (hold-out) check set comprises 10,000 samples and won’t be used for something apart from assessing the mannequin efficiency. On this part we are going to use the 60,000 samples for coaching set with none hyperparameter tuning.
When deriving the gradients of the loss operate with regard to the mannequin parameters we present that it’s crucial to hold out a number of matrix multiplications and a few of these matrices have as many rows because the variety of samples. On condition that sometimes the variety of samples is sort of massive we are going to want a big quantity of reminiscence. To alleviate this we shall be utilizing mini batches in the identical method we used mini batches in the course of the gradient descent optimisation of the adaline mannequin. Sometimes, every batch can include 100–500 samples. Decreasing the batch measurement will increase the convergence velocity as a result of we make extra parameter updates throughout the the identical cross of the coaching set (epoch), however we additionally enhance the noise. We have to strike a steadiness. First we offer a generator that accepts the coaching set and the batch measurement and returns the batches
The generator returns batches of equal measurement that by default include 100 samples. The full variety of samples will not be a a number of of the batch measurement and therefore some samples is not going to be returned in a given cross by means of the coaching set. Th variety of skipped samples is smaller than the batch measurement and the set of samples not noted modifications each time the generator is used, assuming we don’t reset the random quantity generator. Therefore, this isn’t vital. As we shall be passing although the coaching units a number of occasions within the totally different epochs we are going to ultimately use the coaching set totally. The rationale for utilizing batches of a relentless measurement is that we are going to be updating the mannequin parameters after every batch and a small batch can enhance the noise and forestall convergence, particularly if the samples within the batch occur to be outliers.
When the mannequin is initiated we count on a low accuracy that we are able to verify with
that provides an accuracy of roughly 9.5%. This is kind of anticipated for a fairly balanced dataset as there are 10 courses. We now have the means to observe the loss and the accuracy of every batch handed to the ahead cross that we are going to exploit throughout coaching. Let’s write the ultimate piece of code to iterate over the epochs and mini batches, replace the mannequin parameters and monitor how the loss and accuracy evolves in each the coaching set and exterior (hold-out) check set.
Utilizing this operate coaching turns into a single line of code
that produces
epoch 0: loss_training=0.096 | accuracy_training=0.236 | loss_test=0.088 | accuracy_test=0.285
epoch 1: loss_training=0.086 | accuracy_training=0.333 | loss_test=0.085 | accuracy_test=0.367
epoch 2: loss_training=0.083 | accuracy_training=0.430 | loss_test=0.081 | accuracy_test=0.479
epoch 3: loss_training=0.078 | accuracy_training=0.532 | loss_test=0.075 | accuracy_test=0.568
epoch 4: loss_training=0.072 | accuracy_training=0.609 | loss_test=0.069 | accuracy_test=0.629
epoch 5: loss_training=0.066 | accuracy_training=0.657 | loss_test=0.063 | accuracy_test=0.673
epoch 6: loss_training=0.060 | accuracy_training=0.691 | loss_test=0.057 | accuracy_test=0.701
epoch 7: loss_training=0.055 | accuracy_training=0.717 | loss_test=0.052 | accuracy_test=0.725
epoch 8: loss_training=0.050 | accuracy_training=0.739 | loss_test=0.049 | accuracy_test=0.742
epoch 9: loss_training=0.047 | accuracy_training=0.759 | loss_test=0.045 | accuracy_test=0.765
We are able to see that after the ten epochs the accuracy for the coaching set has reached roughly 76%, while the accuracy of the exterior (hold-out) check set is barely larger, indicating that the mannequin has not been overfitted.
The lack of the coaching set retains reducing and therefore convergence has not been reached but. The mannequin permits sizzling beginning so we might run one other ten epochs by repeating the one line of code above. As an alternative, we are going to provoke the mannequin once more and run it for 100 epochs, rising the batch measurement to 200 on the similar time. We offer the entire code for doing so.
We first plot the coaching loss and its price of change as a operate of the epoch quantity
that produces
We are able to see the mannequin has converged moderately properly as the speed of the change of the coaching loss has turn out to be greater than two orders of magnitude smaller in comparison with its worth at first of the coaching. I’m not certain why we observe a discount in convergence velocity at round epoch 10; I can solely speculate that the optimiser escaped an area minimal.
We are able to additionally plot the accuracy of the coaching set and the check set as a operate of the epoch quantity
that produces
The accuracy reaches roughly 90% after about 50 epochs for each the coaching set and exterior (hold-out) check set, suggesting that there is no such thing as a/little overfitting. We simply educated our first, customized constructed multilayer neural community with one hidden layer!
Hyperparameter tuning
On this earlier part we selected an arbitrary community structure and fitted the mannequin parameters. On this part we proceed with a primary hyperparameter tuning by various the variety of hidden layers (starting from 1 to three), the variety of nodes within the hidden layers (starting from 10 to 50 in increments of 10) and the training price (utilizing the values 0.1, 0.2 and 0.3). We stored the batch measurement fixed at 200 samples per batch. General, we tried 45 parameter combos. We are going to make use of 6-fold cross validation (not nested) which suggests 6 mannequin trainings per parameter mixture, which interprets to 270 mannequin trainings in whole. In every fold we shall be utilizing 50,000 samples for coaching and 10,000 samples for measuring the accuracy (referred to as validation within the code). To reinforce the probabilities to attain convergence we are going to carry out 250 epochs for every mannequin becoming. The full execution time was ~12 hours on a single processor (Intel Xeon Gold 3.5GHz). This is kind of what we are able to moderately run on a CPU. The coaching velocity may very well be elevated utilizing multiprocessing. In truth, the coaching could be method quicker utilizing a specialised deep studying library like PyTorch on GPUs, such because the freely accessible T4 GPUs on Google Colab.
This code iterates over all hyperparameter values and folds and shops the loss and accuracy for each the coaching (50,000 samples) and validation (10,000 samples) in a pandas dataframe. The dataframe is used to search out the optimum hyperparameters
that produces
optimum parameters: n_hidden_layers=1, n_hidden_nodes=50, studying price=0.3
finest imply cross validation accuracy: 0.944
| n_hidden_layers | 10 | 20 | 30 | 40 | 50 |
|------------------:|---------:|---------:|---------:|---------:|--------:|
| 1 | 0.905217 | 0.927083 | 0.936883 | 0.939067 | 0.9441 |
| 2 | 0.8476 | 0.925567 | 0.933817 | 0.93725 | 0.9415 |
| 3 | 0.112533 | 0.305133 | 0.779133 | 0.912867 | 0.92285 |
We are able to see that there’s little profit in rising the variety of layers. Maybe we might have gained barely higher efficiency utilizing a bigger first hidden layer because the hyperparameter tuning hit the certain of fifty nodes. Some imply cross-validation accuracies are fairly low that may very well be indicative of poor convergence (e.g. when utilizing 3 hidden layers with 10 nodes every). We didn’t examine additional however this might be sometimes required earlier than concluding on the optimum community geometry. I’d count on that permitting for extra epochs would enhance accuracy additional specific with the bigger networks.
A ultimate step is to retrain the mannequin with all samples apart from the exterior (hold-out) set which might be solely used for the ultimate analysis
The final 5 epochs are
epoch 245: loss_training=0.008 | accuracy_training=0.958 | loss_test=0.009 | accuracy_test=0.946
epoch 246: loss_training=0.008 | accuracy_training=0.958 | loss_test=0.009 | accuracy_test=0.947
epoch 247: loss_training=0.008 | accuracy_training=0.958 | loss_test=0.009 | accuracy_test=0.947
epoch 248: loss_training=0.008 | accuracy_training=0.958 | loss_test=0.009 | accuracy_test=0.946
epoch 249: loss_training=0.008 | accuracy_training=0.958 | loss_test=0.009 | accuracy_test=0.946
We achieved ~95% accuracy with the exterior (hold-out) check set. That is magical if we take into account that we began with a clean piece of paper!
Conclusions
This text demonstrated how we are able to construct a multilayer, feedforward, totally related neural community from scratch. The community was used for fixing a multiclass classification downside. The implementation has been generalised to permit for any variety of hidden layers with any variety of nodes. This facilitates hyperparameter tuning by various the variety of layers and models in them. Nevertheless, we have to needless to say the loss gradients turn out to be smaller and smaller because the depth of the neural community will increase. This is called the vanishing gradient downside and requires utilizing specialised coaching algorithms as soon as the depth exceeds a sure threshold, which is out of the scope of this text.
Our vanilla implementation of a multilayer neural community has hopefully instructional worth. Utilizing it in observe would require a number of enhancements although. To start with, overfitting would should be addressed, by using some type of drop out. Different enhancements, such because the addition of skip-connections and the variation of the training price throughout coaching, could also be helpful too. As well as, the community structure itself could be optimised, e.g. by utilizing a convolutional neural community that may be extra acceptable for classifying pictures. Such enhancements are finest tried utilizing a specialised library like PyTorch. When growing algorithms from scratch one must be cautious of the time it takes and the place to attract the road in order that the endeavour stays instructional with out being extraordinarily time consuming. I hope this text strikes a very good steadiness on this sense. In case you are intrigued I’d advocate this book for additional research.
LaTeX code of equations used within the article
The equations used within the article could be discovered within the gist under, in case you want to render them once more.