Create a doc lake utilizing large-scale textual content extraction from paperwork with Amazon Textract
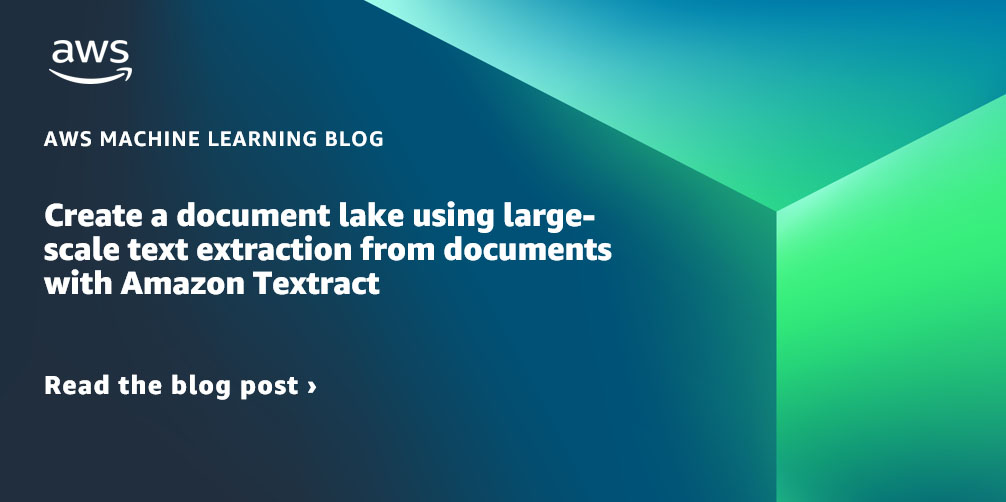
AWS clients in healthcare, monetary providers, the general public sector, and different industries retailer billions of paperwork as photos or PDFs in Amazon Simple Storage Service (Amazon S3). Nevertheless, they’re unable to achieve insights comparable to utilizing the data locked within the paperwork for big language fashions (LLMs) or search till they extract the textual content, kinds, tables, and different structured knowledge. With AWS clever doc processing (IDP) utilizing AI providers comparable to Amazon Textract, you’ll be able to make the most of industry-leading machine studying (ML) expertise to rapidly and precisely course of knowledge from PDFs or doc photos (TIFF, JPEG, PNG). After the textual content is extracted from the paperwork, you should use it to fine-tune a basis mannequin, summarize the data using a foundation model, or ship it to a database.
On this publish, we concentrate on processing a big assortment of paperwork into uncooked textual content information and storing them in Amazon S3. We give you two completely different options for this use case. The primary lets you run a Python script from any server or occasion together with a Jupyter pocket book; that is the quickest method to get began. The second method is a turnkey deployment of assorted infrastructure elements utilizing AWS Cloud Development Kit (AWS CDK) constructs. The AWS CDK assemble offers a resilient and versatile framework to course of your paperwork and construct an end-to-end IDP pipeline. By using the AWS CDK, you’ll be able to prolong its performance to incorporate redaction, store the output in Amazon OpenSearch, or add a customized AWS Lambda perform with your personal enterprise logic.
Each of those options will let you rapidly course of many thousands and thousands of pages. Earlier than working both of those options at scale, we advocate testing with a subset of your paperwork to verify the outcomes meet your expectations. Within the following sections, we first describe the script resolution, adopted by the AWS CDK assemble resolution.
Resolution 1: Use a Python script
This resolution processes paperwork for uncooked textual content via Amazon Textract as rapidly because the service will permit with the expectation that if there’s a failure within the script, the method will choose up from the place it left off. The answer makes use of three completely different providers: Amazon S3, Amazon DynamoDB, and Amazon Textract.
The next diagram illustrates the sequence of occasions throughout the script. When the script ends, a completion standing together with the time taken will probably be returned to the SageMaker studio console.
Now we have packaged this resolution in a .ipynb script and .py script. You should use any of the deployable options as per your necessities.
Conditions
To run this script from a Jupyter pocket book, the AWS Identity and Access Management (IAM) position assigned to the pocket book should have permissions that permit it to work together with DynamoDB, Amazon S3, and Amazon Textract. The final steerage is to offer least-privilege permissions for every of those providers to your AmazonSageMaker-ExecutionRole
position. To study extra, confer with Get started with AWS managed policies and move toward least-privilege permissions.
Alternatively, you’ll be able to run this script from different environments comparable to an Amazon Elastic Compute Cloud (Amazon EC2) occasion or container that you’d handle, supplied that Python, Pip3, and the AWS SDK for Python (Boto3) are put in. Once more, the identical IAM polices should be utilized that permit the script to work together with the varied managed providers.
Walkthrough
To implement this resolution, you first must clone the repository GitHub.
You have to set the next variables within the script earlier than you’ll be able to run it:
- tracking_table – That is the identify of the DynamoDB desk that will probably be created.
- input_bucket – That is your supply location in Amazon S3 that comprises the paperwork that you just wish to ship to Amazon Textract for textual content detection. For this variable, present the identify of the bucket, comparable to
mybucket
. - output_bucket – That is for storing the situation of the place you need Amazon Textract to jot down the outcomes to. For this variable, present the identify of the bucket, comparable to
myoutputbucket
. - _input_prefix (elective) – If you wish to choose sure information from inside a folder in your S3 bucket, you’ll be able to specify this folder identify because the enter prefix. In any other case, depart the default as empty to pick all.
The script is as follows:
The next DynamoDB desk schema will get created when the script is run:
When the script is run for the primary time, it is going to examine to see if the DynamoDB desk exists and can routinely create it if wanted. After the desk is created, we have to populate it with an inventory of doc object references from Amazon S3 that we wish to course of. The script by design will enumerate over objects within the specified input_bucket
and routinely populate our desk with their names when ran. It takes roughly 10 minutes to enumerate over 100,000 paperwork and populate these names into the DynamoDB desk from the script. When you’ve got thousands and thousands of objects in a bucket, you could possibly alternatively use the stock function of Amazon S3 that generates a CSV file of names, then populate the DynamoDB desk from this listing with your personal script prematurely and never use the perform known as fetchAllObjectsInBucketandStoreName
by commenting it out. To study extra, confer with Configuring Amazon S3 Inventory.
As talked about earlier, there’s each a pocket book model and a Python script model. The pocket book is probably the most simple method to get began; merely run every cell from begin to end.
In the event you resolve to run the Python script from a CLI, it’s endorsed that you just use a terminal multiplexer comparable to tmux. That is to stop the script from stopping ought to your SSH session end. For instance: tmux new -d ‘python3 textractFeeder.py’
.
The next is the script’s entry point; from right here you’ll be able to remark out strategies not wanted:
The next fields are set when the script is populating the DynamoDB desk:
- objectName – The identify of the doc positioned in Amazon S3 that will probably be despatched to Amazon Textract
- bucketName – The bucket the place the doc object is saved
These two fields should be populated when you resolve to make use of a CSV file from the S3 stock report and skip the auto populating that occurs throughout the script.
Now that the desk is created and populated with the doc object references, the script is able to begin calling the Amazon Textract StartDocumentTextDetection
API. Amazon Textract, much like different managed providers, has a default limit on the APIs known as transactions per second (TPS). If required, you’ll be able to request a quota improve from the Amazon Textract console. The code is designed to make use of a number of threads concurrently when calling Amazon Textract to maximise the throughput with the service. You’ll be able to change this throughout the code by modifying the threadCountforTextractAPICall
variable. By default, that is set to twenty threads. The script will initially learn 200 rows from the DynamoDB desk and retailer these in an in-memory listing that’s wrapped with a category for thread security. Every caller thread is then began and runs inside its personal swim lane. Principally, the Amazon Textract caller thread will retrieve an merchandise from the in-memory listing that comprises our object reference. It’s going to then name the asynchronous start_document_text_detection
API and watch for the acknowledgement with the job ID. The job ID is then up to date again to the DynamoDB row for that object, and the thread will repeat by retrieving the subsequent merchandise from the listing.
The next is the principle orchestration code script:
The caller threads will proceed repeating till there are not any gadgets throughout the listing, at which level the threads will every cease. When all threads working inside their swim lanes have stopped, the subsequent 200 rows from DynamoDB are retrieved and a brand new set of 20 threads are began, and the entire course of repeats till each row that doesn’t include a job ID is retrieved from DynamoDB and up to date. Ought to the script crash attributable to some surprising downside, then the script might be run once more from the orchestrate()
technique. This makes certain that the threads will proceed processing rows that include empty job IDs. Observe that when rerunning the orchestrate()
technique after the script has stopped, there’s a potential that just a few paperwork will get despatched to Amazon Textract once more. This quantity will probably be equal to or lower than the variety of threads that have been working on the time of the crash.
When there aren’t any extra rows containing a clean job ID within the DynamoDB desk, the script will cease. All of the JSON output from Amazon Textract for all of the objects will probably be discovered within the output_bucket
by default underneath the textract_output
folder. Every subfolder inside textract_output
will probably be named with the job ID that corresponds to the job ID that was saved within the DynamoDB desk for that object. Throughout the job ID folder, you can find the JSON, which will probably be numerically named beginning at 1 and might doubtlessly span extra JSON information that might be labeled 2, 3, and so forth. Spanning JSON information is a results of dense or multi-page paperwork, the place the quantity of content material extracted exceeds the Amazon Textract default JSON measurement of 1,000 blocks. Seek advice from Block for extra data on blocks. These JSON information will include all of the Amazon Textract metadata, together with the textual content that was extracted from throughout the paperwork.
Yow will discover the Python code pocket book model and script for this resolution in GitHub.
Clear up
When the Python script is full, it can save you prices by shutting down or stopping the Amazon SageMaker Studio pocket book or container that you just spun up.
Now on to our second resolution for paperwork at scale.
Resolution 2: Use a serverless AWS CDK assemble
This resolution makes use of AWS Step Functions and Lambda capabilities to orchestrate the IDP pipeline. We use the IDP AWS CDK constructs, which make it simple to work with Amazon Textract at scale. Moreover, we use a Step Functions distributed map to iterate over all of the information within the S3 bucket and provoke processing. The primary Lambda perform determines what number of pages your paperwork has. This permits the pipeline to routinely use both the synchronous (for single-page paperwork) or asynchronous (for multi-page paperwork) API. When utilizing the asynchronous API, a further Lambda perform is known as to all of the JSON information that Amazon Textract will produce for all your pages into one JSON file to make it simple in your downstream purposes to work with the data.
This resolution additionally comprises two extra Lambda capabilities. The primary perform parses the textual content from the JSON and saves it as a textual content file in Amazon S3. The second perform analyzes the JSON and shops that for metrics on the workload.
The next diagram illustrates the Step Features workflow.
Conditions
This code base makes use of the AWS CDK and requires Docker. You’ll be able to deploy this from an AWS Cloud9 occasion, which has the AWS CDK and Docker already arrange.
Walkthrough
To implement this resolution, you first must clone the repository.
After you clone the repository, set up the dependencies:
Then use the next code to deploy the AWS CDK stack:
You need to present each the supply bucket and supply prefix (the situation of the information you wish to course of) for this resolution.
When the deployment is full, navigate to the Step Features console, the place it is best to see the state machine ServerlessIDPArchivePipeline
.
Open the state machine particulars web page and on the Executions tab, select Begin execution.
Select Begin execution once more to run the state machine.
After you begin the state machine, you’ll be able to monitor the pipeline by trying on the map run. You will note an Merchandise processing standing part like the next screenshot. As you’ll be able to see, that is constructed to run and monitor what was profitable and what failed. This course of will proceed to run till all paperwork have been learn.
With this resolution, it is best to have the ability to course of thousands and thousands of information in your AWS account with out worrying about the way to correctly decide which information to ship to which API or corrupt information failing your pipeline. By the Step Features console, it is possible for you to to look at and monitor your information in actual time.
Clear up
After your pipeline is completed working, to wash up, you’ll be able to return into your challenge and enter the next command:
This may delete any providers that have been deployed for this challenge.
Conclusion
On this publish, we offered an answer that makes it simple to transform your doc photos and PDFs to textual content information. This can be a key prerequisite to utilizing your paperwork for generative AI and search. To study extra about utilizing textual content to coach or fine-tune your basis fashions, confer with Fine-tune Llama 2 for text generation on Amazon SageMaker JumpStart. To make use of with search, confer with Implement smart document search index with Amazon Textract and Amazon OpenSearch. To study extra about superior doc processing capabilities provided by AWS AI providers, confer with Guidance for Intelligent Document Processing on AWS.
In regards to the Authors
Tim Condello is a senior synthetic intelligence (AI) and machine studying (ML) specialist options architect at Amazon Internet Providers (AWS). His focus is pure language processing and laptop imaginative and prescient. Tim enjoys taking buyer concepts and turning them into scalable options.
David Girling is a senior AI/ML options architect with over twenty years of expertise in designing, main and growing enterprise techniques. David is a part of a specialist crew that focuses on serving to clients study, innovate and make the most of these extremely succesful providers with their knowledge for his or her use circumstances.