Automate PDF pre-labeling for Amazon Comprehend
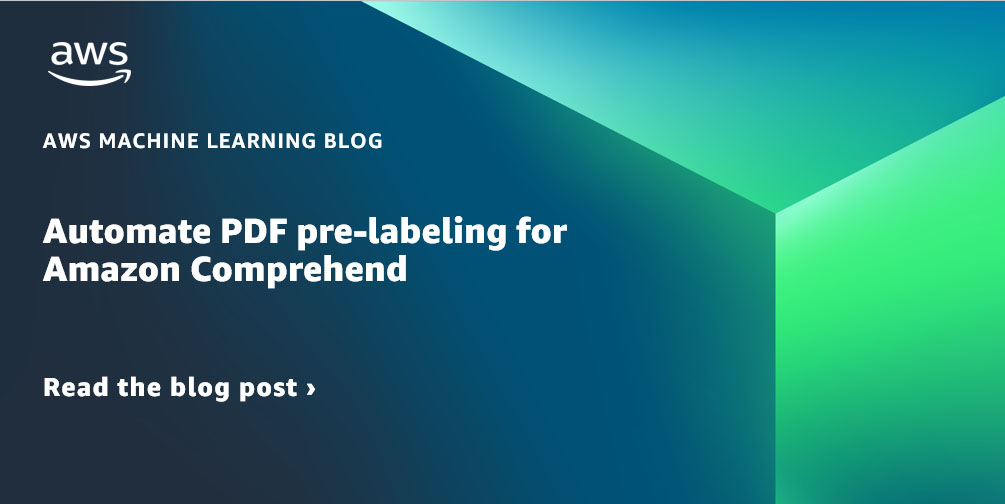
Amazon Comprehend is a natural-language processing (NLP) service that gives pre-trained and customized APIs to derive insights from textual knowledge. Amazon Comprehend clients can prepare customized named entity recognition (NER) fashions to extract entities of curiosity, similar to location, particular person identify, and date, which might be distinctive to their enterprise.
To coach a customized mannequin, you first put together coaching knowledge by manually annotating entities in paperwork. This may be accomplished with the Comprehend Semi-Structured Documents Annotation Tool, which creates an Amazon SageMaker Ground Truth job with a customized template, permitting annotators to attract bounding packing containers across the entities instantly on the PDF paperwork. Nevertheless, for firms with present tabular entity knowledge in ERP methods like SAP, handbook annotation could be repetitive and time-consuming.
To cut back the trouble of making ready coaching knowledge, we constructed a pre-labeling device utilizing AWS Step Functions that mechanically pre-annotates paperwork by utilizing present tabular entity knowledge. This considerably decreases the handbook work wanted to coach correct customized entity recognition fashions in Amazon Comprehend.
On this submit, we stroll you thru the steps of organising the pre-labeling device and present examples of the way it mechanically annotates paperwork from a public dataset of pattern financial institution statements in PDF format. The complete code is obtainable on the GitHub repo.
Resolution overview
On this part, we focus on the inputs and outputs of the pre-labeling device and supply an outline of the answer structure.
Inputs and outputs
As enter, the pre-labeling device takes PDF paperwork that include textual content to be annotated. For the demo, we use simulated financial institution statements like the next instance.
The device additionally takes a manifest file that maps PDF paperwork with the entities that we wish to extract from these paperwork. Entities consists of two issues: the expected_text
to extract from the doc (for instance, AnyCompany Financial institution
) and the corresponding entity_type
(for instance, bank_name
). Later on this submit, we present the right way to assemble this manifest file from a CSV doc like the next instance.
The pre-labeling device makes use of the manifest file to mechanically annotate the paperwork with their corresponding entities. We are able to then use these annotations instantly to coach an Amazon Comprehend mannequin.
Alternatively, you possibly can create a SageMaker Floor Reality labeling job for human overview and modifying, as proven within the following screenshot.
When the overview is full, you should utilize the annotated knowledge to coach an Amazon Comprehend customized entity recognizer mannequin.
Structure
The pre-labeling device consists of a number of AWS Lambda features orchestrated by a Step Capabilities state machine. It has two variations that use completely different strategies to generate pre-annotations.
The primary approach is fuzzy matching. This requires a pre-manifest file with anticipated entities. The device makes use of the fuzzy matching algorithm to generate pre-annotations by evaluating textual content similarity.
Fuzzy matching seems to be for strings within the doc which might be related (however not essentially equivalent) to the anticipated entities listed within the pre-manifest file. It first calculates textual content similarity scores between the anticipated textual content and phrases within the doc, then it matches all pairs above a threshold. Subsequently, even when there aren’t any actual matches, fuzzy matching can discover variants like abbreviations and misspellings. This enables the device to pre-label paperwork with out requiring the entities to seem verbatim. For instance, if 'AnyCompany Financial institution'
is listed as an anticipated entity, Fuzzy Matching will annotate occurrences of 'Any Companys Financial institution'
. This gives extra flexibility than strict string matching and permits the pre-labeling device to mechanically label extra entities.
The next diagram illustrates the structure of this Step Capabilities state machine.
The second approach requires a pre-trained Amazon Comprehend entity recognizer model. The device generates pre-annotations utilizing the Amazon Comprehend mannequin, following the workflow proven within the following diagram.
The next diagram illustrates the complete structure.
Within the following sections, we stroll by means of the steps to implement the answer.
Deploy the pre-labeling device
Clone the repository to your native machine:
This repository has been constructed on prime of the Comprehend Semi-Structured Paperwork Annotation Instrument and extends its functionalities by enabling you to start out a SageMaker Floor Reality labeling job with pre-annotations already displayed on the SageMaker Floor Reality UI.
The pre-labeling device contains each the Comprehend Semi-Structured Paperwork Annotation Instrument assets in addition to some assets particular to the pre-labeling device. You possibly can deploy the answer with AWS Serverless Application Model (AWS SAM), an open supply framework that you should utilize to outline serverless software infrastructure code.
You probably have beforehand deployed the Comprehend Semi-Structured Paperwork Annotation Instrument, confer with the FAQ part in Pre_labeling_tool/README.md
for directions on the right way to deploy solely the assets particular to the pre-labeling device.
If you happen to haven’t deployed the device earlier than and are beginning contemporary, do the next to deploy the entire resolution.
Change the present listing to the annotation device folder:
Construct and deploy the answer:
Create the pre-manifest file
Earlier than you should utilize the pre-labeling device, you might want to put together your knowledge. The primary inputs are PDF paperwork and a pre-manifest file. The pre-manifest file incorporates the placement of every PDF doc beneath 'pdf'
and the placement of a JSON file with anticipated entities to label beneath 'expected_entities'
.
The pocket book generate_premanifest_file.ipynb exhibits the right way to create this file. Within the demo, the pre-manifest file exhibits the next code:
Every JSON file listed within the pre-manifest file (beneath expected_entities
) incorporates a listing of dictionaries, one for every anticipated entity. The dictionaries have the next keys:
- ‘expected_texts’ – An inventory of doable textual content strings matching the entity.
- ‘entity_type’ – The corresponding entity kind.
- ‘ignore_list’ (elective) – The listing of phrases that needs to be ignored within the match. These parameters needs to be used to stop fuzzy matching from matching particular mixtures of phrases that you recognize are mistaken. This may be helpful if you wish to ignore some numbers or e mail addresses when names.
For instance, the expected_entities
of the PDF proven beforehand seems to be like the next:
Run the pre-labeling device
With the pre-manifest file that you just created within the earlier step, begin operating the pre-labeling device. For extra particulars, confer with the pocket book start_step_functions.ipynb.
To start out the pre-labeling device, present an occasion
with the next keys:
- Premanifest – Maps every PDF doc to its
expected_entities
file. This could include the Amazon Simple Storage Service (Amazon S3) bucket (beneathbucket
) and the important thing (beneathkey
) of the file. - Prefix – Used to create the
execution_id
, which names the S3 folder for output storage and the SageMaker Floor Reality labeling job identify. - entity_types – Displayed within the UI for annotators to label. These ought to embody all entity sorts within the anticipated entities recordsdata.
- work_team_name (elective) – Used for creating the SageMaker Floor Reality labeling job. It corresponds to the non-public workforce to make use of. If it’s not offered, solely a manifest file might be created as an alternative of a SageMaker Floor Reality labeling job. You should utilize the manifest file to create a SageMaker Floor Reality labeling job in a while. Word that as of this writing, you possibly can’t present an exterior workforce when creating the labeling job from the pocket book. Nevertheless, you possibly can clone the created job and assign it to an exterior workforce on the SageMaker Floor Reality console.
- comprehend_parameters (elective) – Parameters to instantly prepare an Amazon Comprehend customized entity recognizer mannequin. If omitted, this step might be skipped.
To start out the state machine, run the next Python code:
This can begin a run of the state machine. You possibly can monitor the progress of the state machine on the Step Capabilities console. The next diagram illustrates the state machine workflow.
When the state machine is full, do the next:
- Examine the next outputs saved within the
prelabeling/
folder of thecomprehend-semi-structured-docs
S3 bucket:- Particular person annotation recordsdata for every web page of the paperwork (one per web page per doc) in
temp_individual_manifests/
- A manifest for the SageMaker Floor Reality labeling job in
consolidated_manifest/consolidated_manifest.manifest
- A manifest that can be utilized to coach a customized Amazon Comprehend mannequin in
consolidated_manifest/consolidated_manifest_comprehend.manifest
- Particular person annotation recordsdata for every web page of the paperwork (one per web page per doc) in
- On the SageMaker console, open the SageMaker Floor Reality labeling job that was created to overview the annotations
- Examine and check the customized Amazon Comprehend mannequin that was skilled
As talked about beforehand, the device can solely create SageMaker Floor Reality labeling jobs for personal workforces. To outsource the human labeling effort, you possibly can clone the labeling job on the SageMaker Floor Reality console and connect any workforce to the brand new job.
Clear up
To keep away from incurring extra prices, delete the assets that you just created and delete the stack that you just deployed with the next command:
Conclusion
The pre-labeling device gives a strong manner for firms to make use of present tabular knowledge to speed up the method of coaching customized entity recognition fashions in Amazon Comprehend. By mechanically pre-annotating PDF paperwork, it considerably reduces the handbook effort required within the labeling course of.
The device has two variations: fuzzy matching and Amazon Comprehend-based, giving flexibility on the right way to generate the preliminary annotations. After paperwork are pre-labeled, you possibly can shortly overview them in a SageMaker Floor Reality labeling job and even skip the overview and instantly prepare an Amazon Comprehend customized mannequin.
The pre-labeling device lets you shortly unlock the worth of your historic entity knowledge and use it in creating customized fashions tailor-made to your particular area. By rushing up what is often probably the most labor-intensive a part of the method, it makes customized entity recognition with Amazon Comprehend extra accessible than ever.
For extra details about the right way to label PDF paperwork utilizing a SageMaker Floor Reality labeling job, see Custom document annotation for extracting named entities in documents using Amazon Comprehend and Use Amazon SageMaker Ground Truth to Label Data.
Concerning the authors
Oskar Schnaack is an Utilized Scientist on the Generative AI Innovation Middle. He’s enthusiastic about diving into the science behind machine studying to make it accessible for patrons. Exterior of labor, Oskar enjoys biking and maintaining with tendencies in info idea.
Romain Besombes is a Deep Studying Architect on the Generative AI Innovation Middle. He’s enthusiastic about constructing modern architectures to deal with clients’ enterprise issues with machine studying.