From the Perceptron to Adaline. Setting the foundations proper | by Pan Cretan | Nov, 2023
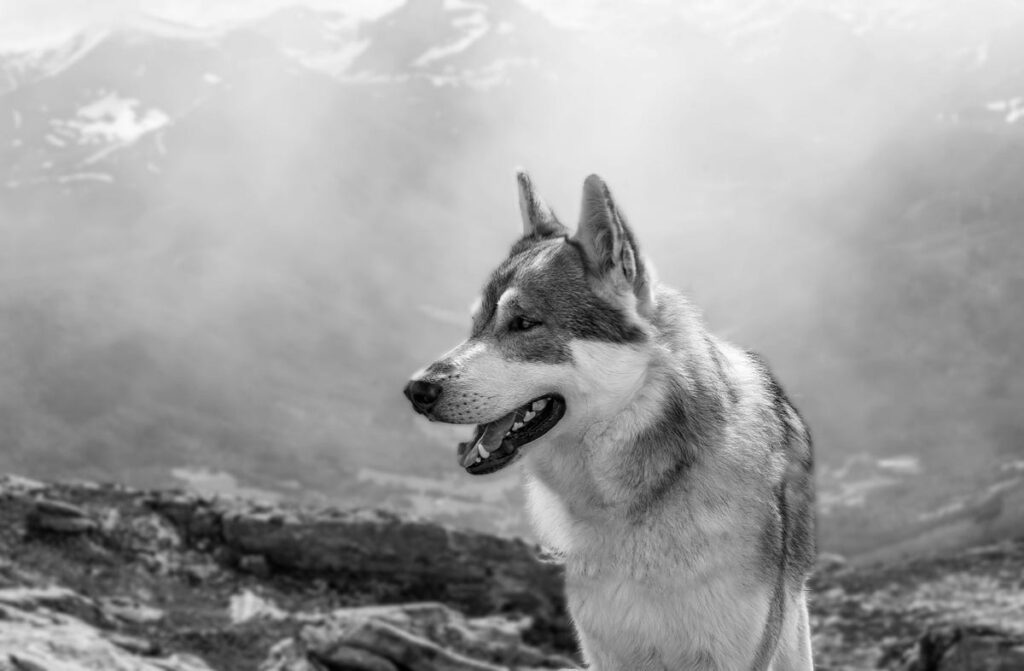
Introduction
In a earlier article I attempted to clarify essentially the most fundamental binary classifier that has doubtless ever existed, Rosenblatt’s perceptron. Understanding this algorithm has instructional worth and may function introduction in elementary machine studying programs. It’s an algorithm that may be coded from scratch in a single afternoon and may spark curiosity, a way of accomplishment and motivation to delve into extra complicated subjects. Nonetheless, as an algorithm it leaves a lot to be desired as a result of convergence is simply assured when the lessons are linearly separable that’s usually not the case.
On this article we’ll proceed the journey on mastering classification ideas. A pure evolution from the Rosenblatt’s perceptron is the adaptive liclose to neuron classifier, or adaline as it’s colloquially recognized. Shifting from the perceptron to adaline just isn’t a giant leap. We merely want to alter the step activation operate to a linear one. This small change results in a steady loss operate that may be robustly minimised. This permits us to introduce many helpful ideas in machine studying, corresponding to vectorisation and optimisation strategies.
In future articles we may even cowl additional refined modifications of the activation and loss capabilities that may take us from adaline to logistic regression, that’s already a helpful algorithm in every day follow. All the above algorithms are primarily single layer neural networks and will be readily prolonged to multilayer ones. On this sense, this text takes the reader a step additional by this evolution and builds the foundations to sort out extra superior ideas.
We are going to want some formulation. I used the web LaTeX equation editor to develop the LaTeX code for the equation after which the chrome plugin Maths Equations Anywhere to render the equation into a picture. The one draw back of this method is that the LaTeX code just isn’t saved in case it’s essential to render it once more. For this function I present the listing of equations on the finish of this text. If you’re not aware of LaTex this will likely have its personal instructional worth. Getting the notation proper is a part of the journey in machine studying.
Adaptive linear neuron classifier (adaline)
So what’s the adaline algorithm? Adaline is a binary classifier because the perceptron. A prediction is made through the use of a set of enter values for the options [x₁, .. , xₘ] the place m is the variety of options. The enter values are multiplied with the weights [w₁, .. , wₘ] and the bias is added to acquire the web enter z = w₁x₁ + .. + wₘxₘ + b. The online enter is handed to the linear activation operate σ(z) that’s then used to make a prediction utilizing a step operate as with the perceptron:
A key distinction with the perceptron is that the linear activation operate is used for studying the weights, while the step operate is simply used for making the prediction on the finish. This feels like a small factor, however it’s of great significance. The linear activation operate is differentiable while the step operate just isn’t! The edge 0.5 above just isn’t written in stone. By adjusting the edge we are able to regulate the precision and recall in line with our use case, i.e. primarily based on what’s the price of false positives and false negatives.
Within the case of adaline the linear activation operate is solely the identification, i.e. σ(z) = z. The target operate (also called loss operate) that must be minimised within the coaching course of is
the place w are the weights
and b is the bias. The summation is over all the examples within the coaching set. In some implementations the loss operate additionally features a 1/2 coefficient for comfort. This cancels out as soon as we take the gradients of the loss operate with respect to the weights and bias and, as we’ll see beneath, has no impact aside from scaling the educational price by an element of two. On this article we don’t use the 1/2 coefficient.
For every instance, we compute the sq. distinction between the calculated final result
and the true class label. Be aware that the enter vector is known to be a matrix with form (1, m), i.e. as we’ll see later is one row of our function matrix x with form (n, m).
The coaching is nothing else than an optimisation drawback. We have to modify the weights and bias in order that the loss operate is minimised. As with all minimisation drawback we have to compute the gradients of the target operate with respect to the impartial variables that in our case would be the weights and the bias. The partial spinoff of the loss operate with regard to the burden wⱼ is
The final row introduces vital matrix notation. The function matrix x has form (n, m) and we take the transpose of its column j, i.e. a matrix with form (1, n). The true class labels y is a matrix with form (n, 1). The online output of all samples z can be a matrix with form (n, 1), that doesn’t change after the activation that’s understood to use to every of its parts. The ultimate results of the above method is a scalar. Are you able to guess how we may categorical the gradients with respect to all weights utilizing the matrix notation?
the place the transpose of the function matrix has form (m, n). The tip results of this operation is a matrix with form (m, 1). This notation is vital. As a substitute of utilizing loops, we will likely be utilizing precisely this matrix multiplication utilizing numpy. Within the period of neural networks and GPUs, the power to use vectorization is crucial!
What in regards to the gradient of the loss operate with respect to the bias?
the place the overbar denotes the imply of the vector beneath it. As soon as extra, computing the imply with numpy is a vectorised operation, i.e. summation doesn’t should be applied utilizing a loop.
As soon as we have now the gradients we are able to make use of the gradient descent optimisation methodology to minimise the loss. The weights and bias phrases are iteratively up to date utilizing
the place η is an acceptable chosen studying price. Too small values can delay convergence, while too excessive values can stop convergence altogether. Some experimentation is required, as is mostly the case with the parameters of machine studying algorithms.
Within the above implementation we assume that the weights and bias are up to date primarily based on all examples without delay. This is called full batch gradient descent and is one excessive. The opposite excessive is to replace the weights and bias after every coaching instance, that is called stochastic gradient descent (SGD). In actuality there may be additionally some center floor, often known as mini batch gradient descent, the place the weights and bias are up to date primarily based on a subset of the examples. Convergence is often reached quicker on this approach, i.e. we don’t must run as many iterations over the entire coaching set, while vectorisation continues to be (a minimum of partially) potential. If the coaching set may be very giant (or the mannequin may be very complicated as is these days the case with the transformers in NLP) full batch gradient descent might merely be not an choice.
Different formulation and closed kind answer
Earlier than we proceed with the implementation of adaline in Python, we’ll make a fast digression. We may take up the bias b within the weight vector as
by which case the web output for all samples within the coaching set turns into
that means that the function matrix has been prepended with a column full of 1, resulting in a form (n, m+1). The gradient with regard to the mixed weights set turns into
In precept we may derive a closed kind answer provided that on the minimal all gradients will likely be zero
In actuality the inverse of the matrix within the above equation might not exist due to singularities or it can’t be computed sufficiently precisely. Therefore, such closed kind answer just isn’t utilized in follow neither in machine studying nor in numerical strategies normally. Nonetheless, it’s helpful to understand that adaline resembles linear regression and as such it has a closed kind answer.
Implementing adaline in Python
Our implementation will use mini batch gradient descent. Nonetheless, the implementation is versatile and permits optimising the loss operate utilizing each stochastic gradient descent and full batch gradient descent as the 2 extremes. We are going to study the convergence behaviour by various the batch measurement.
We implement adaline utilizing a category that exposes a match and a predict operate within the typical scikit-learn API type.
Upon initialisation the adaline classifier units the batch measurement for the mini batch gradient descent. If batch measurement is about to None, the entire coaching set is used (full batch gradient descent), in any other case the coaching set is utilized in batches (mini batch gradient descent). If the batch measurement is one we primarily revert to stochastic gradient descent. The coaching set is shuffled earlier than every move by the coaching set to keep away from repetitive cycles, however this solely has an impact if mini batch gradient descent is used. The essence of the algorithm is within the _update_weights_bias
operate that carries out a full move by the coaching set and returns the corresponding loss. This operate applies the gradient descent with the analytically computed gradients utilizing the derivations as within the earlier part. Be aware the usage of the numpy matmul
and dot
capabilities that keep away from the usage of express loops. If the batch_size is about to None then there aren’t any loops in any way and the implementation is totally vectorised.
Utilizing adaline in follow
We make the required imports and create an artificial dataset as within the earlier perceptron article
that produces
The one distinction with the sooner article is that we tweaked the gaussian means and covariances in order that the lessons aren’t linearly separable as we’d anticipate adaline to beat this. Furthermore, the 2 impartial variables have on function totally different scales to debate the significance of function scaling.
Let’s attempt to match a primary mannequin and visualise convergence. Previous to becoming we normalise the options in order that they each have zero imply and unit commonplace deviation
This produces the convergence plot
Adaline slowly converges, however the loss operate doesn’t change into zero. With a view to confirm the profitable coaching we visualise the choice boundary utilizing the identical method as within the earlier article
that produces
There are some misclassified factors provided that the 2 lessons within the coaching set weren’t linearly separable and we used a linear choice boundary. Nonetheless, the algorithm converged properly. The answer is deterministic. With enough variety of passes by the coaching set we get hold of numerically equal weights and bias, no matter their preliminary values.
Mini batch vs. full batch gradient descent
The above numerical experiment used full batch gradient descent that partially explains the gradual convergence. We are going to use the identical dataset and random state as earlier than, however this time we’ll match the adaline classifier utilizing totally different batch sizes, starting from 20 to 400 that’s the variety of examples in our coaching set.
that produces
We will clearly see that the smaller the batch measurement the quicker the convergence, however there are additionally some oscillations. These oscillation might destabilise the convergence with bigger studying charges. If we double the educational price to 0.002 this turns into evident
Growing the educational price additional will finally stop convergence with the smaller batch sizes. With even bigger studying charges even the complete batch gradient descent will fail to converge as we’d overshoot the worldwide minimal.
Conclusions
Adaline is a major enchancment over the perceptron. The weights and bias are obtained through the minimisation of a steady loss operate that as well as is convex (and therefore doesn’t have native minima). With a enough small studying price the algorithm converges even when the lessons aren’t linearly separable. When utilizing gradient descent in any of its variants the convergence price is affected by the scaling of the options. On this article we used easy standardisation that shifts the imply of each function to change into zero, while the unfold is adjusted to unit variance. On this approach it’s potential to pick out a studying price that works effectively for all weights and bias, that means that the worldwide minimal will be obtained in fewer epochs.
Acquiring understanding on learn how to construct a binary classifier utilizing vectorisation is essential earlier than delving into extra complicated subjects, corresponding to help vector machines and multilayer neural networks. In every day follow, one would use scikit-learn that gives superior classification algorithms that enable for nonlinear choice boundaries, while supporting environment friendly and systematic hyper parameter tuning and cross validation. Nonetheless, constructing easy binary classifiers from scratch presents a deep understanding, will increase confidence and offers a way of possession. Though constructing every part from scratch is after all not practical, deeply understanding the less complicated algorithms gives the required expertise and insights in order that extra superior algorithms included in off-the-shelf libraries really feel much less opaque.
LaTeX code of equations used within the article
The equations used within the article will be discovered within the gist beneath, in case you wish to render them once more.