Personalize your generative AI purposes with Amazon SageMaker Function Retailer
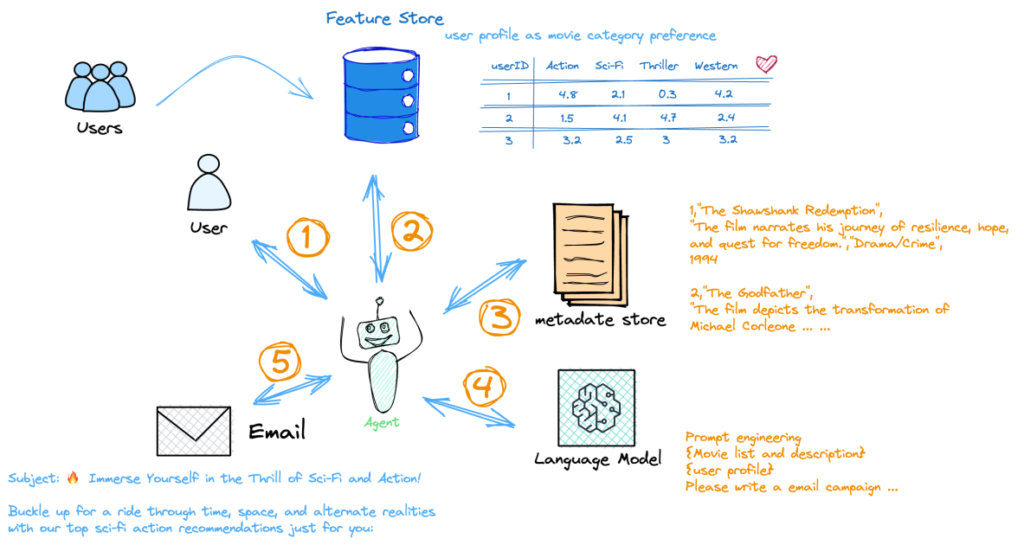
Massive language fashions (LLMs) are revolutionizing fields like serps, pure language processing (NLP), healthcare, robotics, and code era. The purposes additionally lengthen into retail, the place they’ll improve buyer experiences by way of dynamic chatbots and AI assistants, and into digital advertising and marketing, the place they’ll set up buyer suggestions and suggest merchandise primarily based on descriptions and buy behaviors.
The personalization of LLM purposes will be achieved by incorporating up-to-date person data, which usually includes integrating a number of elements. One such element is a characteristic retailer, a instrument that shops, shares, and manages options for machine studying (ML) fashions. Options are the inputs used throughout coaching and inference of ML fashions. For example, in an utility that recommends films, options may embrace earlier rankings, choice classes, and demographics. Amazon SageMaker Feature Store is a totally managed repository designed particularly for storing, sharing, and managing ML mannequin options. One other important element is an orchestration instrument appropriate for immediate engineering and managing completely different sort of subtasks. Generative AI builders can use frameworks like LangChain, which presents modules for integrating with LLMs and orchestration instruments for process administration and immediate engineering.
Constructing on the idea of dynamically fetching up-to-date knowledge to supply personalised content material, the usage of LLMs has garnered vital consideration in latest analysis for recommender programs. The underlying precept of those approaches includes the development of prompts that encapsulate the advice process, person profiles, merchandise attributes, and user-item interactions. These task-specific prompts are then fed into the LLM, which is tasked with predicting the chance of interplay between a specific person and merchandise. As said within the paper Personalized Recommendation via Prompting Large Language Models, recommendation-driven and engagement-guided prompting elements play a vital position in enabling LLMs to give attention to related context and align with person preferences.
On this submit, we elucidate the easy but highly effective thought of mixing person profiles and merchandise attributes to generate personalised content material suggestions utilizing LLMs. As demonstrated all through the submit, these fashions maintain immense potential in producing high-quality, context-aware enter textual content, which ends up in enhanced suggestions. For example this, we information you thru the method of integrating a characteristic retailer (representing person profiles) with an LLM to generate these personalised suggestions.
Answer overview
Let’s think about a state of affairs the place a film leisure firm promotes films to completely different customers by way of an electronic mail marketing campaign. The promotion comprises 25 well-known films, and we need to choose the highest three suggestions for every person primarily based on their pursuits and former score behaviors.
For instance, given a person’s curiosity in numerous film genres like motion, romance, and sci-fi, we may have an AI system decide the highest three advisable films for that specific person. As well as, the system may generate personalised messages for every person in a tone tailor-made to their preferences. We embrace some examples of personalised messages later on this submit.
This AI utility would come with a number of elements working collectively, as illustrated within the following diagram:
- A person profiling engine takes in a person’s earlier behaviors and outputs a person profile reflecting their pursuits.
- A characteristic retailer maintains person profile knowledge.
- A media metadata retailer retains the promotion film checklist updated.
- A language mannequin takes the present film checklist and person profile knowledge, and outputs the highest three advisable films for every person, written of their most well-liked tone.
- An orchestrating agent coordinates the completely different elements.
In abstract, clever brokers may assemble prompts utilizing user- and item-related knowledge and ship custom-made pure language responses to customers. This is able to characterize a typical content-based suggestion system, which recommends gadgets to customers primarily based on their profiles. The person’s profile is saved and maintained within the characteristic retailer and revolves round their preferences and tastes. It’s generally derived primarily based on their earlier behaviors, resembling rankings.
The next diagram illustrates the way it works.
The applying follows these steps to offer responses to a person’s suggestion:
- The person profiling engine that takes a person’s historic film score as enter, outputs person curiosity, and shops the characteristic in SageMaker Function Retailer. This course of will be up to date in a scheduling method.
- The agent takes the person ID as enter, searches for the person curiosity, and completes the immediate template following the person’s pursuits.
- The agent takes the promotion merchandise checklist (film identify, description, style) from a media metadata retailer.
- The pursuits immediate template and promotion merchandise checklist are fed into an LLM for electronic mail marketing campaign messages.
- The agent sends the personalised electronic mail marketing campaign to the top person.
The person profiling engine builds a profile for every person, capturing their preferences and pursuits. This profile will be represented as a vector with components mapping to options like film genres, with values indicating the person’s stage of curiosity. The person profiles within the characteristic retailer enable the system to recommend personalised suggestions matching their pursuits. Person profiling is a well-studied area inside suggestion programs. To simplify, you possibly can construct a regression algorithm utilizing a person’s earlier rankings throughout completely different classes to deduce their total preferences. This may be performed with algorithms like XGBoost.
Code walkthrough
On this part, we offer examples of the code. The complete code walkthrough is on the market within the GitHub repo.
After acquiring the person pursuits characteristic from the person profiling engine, we will retailer the ends in the characteristic retailer. SageMaker Function Retailer helps batch characteristic ingestion and on-line storage for real-time inference. For ingestion, knowledge will be up to date in an offline mode, whereas inference must occur in milliseconds. SageMaker Function Retailer ensures that offline and on-line datasets stay in sync.
For knowledge ingestion, we use the next code:
For real-time on-line storage, we may use the next code to extract the person profile primarily based on the person ID:
Then we rank the highest three film classes to feed the downstream suggestion engine:
Person ID: 42
Top3 Classes: [‘Animation’, ‘Thriller’, ‘Adventure’]
Our utility employs two major elements. The primary element retrieves knowledge from a characteristic retailer, and the second element acquires a listing of film promotions from the metadata retailer. The coordination between these elements is managed by Chains from LangChain, which characterize a sequence of calls to elements.
It’s price mentioning that in complicated eventualities, the appliance may have greater than a hard and fast sequence of calls to LLMs or different instruments. Agents, outfitted with a set of instruments, use an LLM to find out the sequence of actions to be taken. Whereas Chains encode a hardcoded sequence of actions, brokers use the reasoning energy of a language mannequin to dictate the order and nature of actions.
The connection between completely different knowledge sources, together with SageMaker Function Retailer, is demonstrated within the following code. All of the retrieved knowledge is consolidated to assemble an in depth immediate, serving as enter for the LLM. We dive deep into the specifics of immediate design within the subsequent part. The next is a immediate template definition that interfaces with a number of knowledge sources:
As well as, we use Amazon SageMaker to host our LLM mannequin and expose it because the LangChain SageMaker endpoint. To deploy the LLM, we use Amazon SageMaker JumpStart (for extra particulars, seek advice from Llama 2 foundation models from Meta are now available in Amazon SageMaker JumpStart). After the mannequin is deployed, we will create the LLM module:
Within the context of our utility, the agent runs a sequence of steps, referred to as an LLMChain. It integrates a immediate template, mannequin, and guardrails to format the person enter, cross it to the mannequin, get a response, after which validate (and, if crucial, rectify) the mannequin output.
Within the subsequent part, we stroll by way of the immediate engineering for the LLM to output anticipated outcomes.
LLM suggestion prompting and outcomes
Following the high-level idea of engagement-guided prompting as described within the analysis examine Personalized Recommendation via Prompting Large Language Models, the basic precept of our prompting technique is to combine person preferences in creating prompts. These prompts are designed to information the LLM in the direction of extra successfully figuring out attributes throughout the content material description that align with person preferences. To elaborate additional, our immediate includes a number of elements:
- Contextual relevance – The preliminary a part of our immediate template incorporates media metadata resembling merchandise identify (film title), description (film synopsis), and attribute (film style). By incorporating this data, the immediate offers the LLM with a broader context and a extra complete understanding of the content material. This contextual data aids the LLM in higher understanding the merchandise by way of its description and attributes, thereby enhancing its utility in content material suggestion eventualities.
- Person choice alignment – By bearing in mind a person profile that signifies person preferences, potential suggestions are higher positioned to determine content material traits and options that resonate with goal customers. This alignment augments the utility of the merchandise descriptions as a result of it enhances the effectivity of recommending gadgets which can be related and according to person preferences.
- Enhanced suggestion high quality – The engagement-guided immediate makes use of person preferences to determine related promotional gadgets. We will additionally use person choice to regulate the tone of the LLM for the ultimate output. This can lead to an correct, informative, and personalised expertise, thereby bettering the general efficiency of the content material suggestion system.
The next code reveals an instance immediate template:
prompt_template = """
Our firm, "Basic Cinema" regularly promotes films that we purpose to suggest to our clients. This month, we have now a number of widespread films on promotion.
As an AI agent, you might be tasked to help "Basic Cinema" in crafting an electronic mail marketing campaign to suggest related films to customers. The suggestions ought to adhere to a number of pointers, together with contextual relevance, guaranteeing the suggestions are strictly from our promotional film checklist. Moreover, the suggestions ought to align with person preferences, suggesting gadgets which can be related and in concord with the person's most well-liked classes. You might be to offer exactly three high advisable films. Lastly, please draft the e-mail to mirror the tone of the person's most well-liked classes. The e-mail mustn't exceed 100 phrases.
The advisable films ought to be sourced from this contextual relevance film checklist:
{promotion_movie_list}.
The person has expressed curiosity in {user_preference}.
Please make sure the suggestions are related, and the tone of the e-mail displays the tastes of these within the {user_preference} film class.
Make sure the letter appeals to these within the {user_preference} film class, and hold the e-mail marketing campaign inside a 100-word restrict. """
The next is an instance outcome with person preferences of sci-fi, journey, and struggle genres:
Topic: Discover the Frontier of Basic Cinema with Our Sci-Fi, Journey, and Struggle Motion pictures!
Pricey [Name],
Are you able to embark on a journey by way of time and area, expertise heart-pumping motion, and witness the bravery of heroes on the battlefield? Look no additional! Basic Cinema proudly presents our fastidiously curated number of films, tailor-made particularly to your pursuits in Sci-Fi, Journey, and Struggle.First up, we have now the long-lasting “Star Wars: Episode V – The Empire Strikes Again” (1980). This basic installment within the Star Wars saga follows Luke Skywalker’s journey to changing into a Jedi Grasp, whereas his buddies face off in opposition to the evil Empire. With its groundbreaking particular results and memorable characters, this film is a must-see for any fan of the style.
Subsequent, we suggest “Interstellar” (2014), a thought-provoking and visually gorgeous movie that delves into the mysteries of time and area. As a gaggle of explorers embark on a quest to save lots of humanity, they encounter breathtaking landscapes and uncover the secrets and techniques of the universe.
Lastly, we have now “Saving Non-public Ryan” (1998), a gripping and intense struggle drama that follows a gaggle of troopers on a harmful mission to seek out and rescue a paratrooper whose brothers have been killed in motion. This critically acclaimed movie is a robust tribute to the heroes of World Struggle II.
Don’t miss out on these cinematic masterpieces! Watch them now and expertise the fun of journey, the surprise of sci-fi, and the bravery of struggle heroes.
Blissful viewing, and should the drive be with you!Greatest regards,
Basic Cinema Crew
The next is one other instance outcome with a person choice of documentary, musical, and drama:
Topic: Basic Cinema’s Suggestions for Documentary, Musical, and Drama Lovers
Pricey [Name],
We hope this electronic mail finds you nicely and that you simply’re having fun with the number of films obtainable on our platform. At Basic Cinema, we take satisfaction in catering to the various tastes of our clients, and we’ve chosen three distinctive films that we consider will resonate together with your curiosity in Documentary, Musical, and Drama.
First up, we have now “The Shawshank Redemption” (1994), a robust and uplifting drama that follows the journey of two prisoners as they discover hope and redemption in a corrupt and unforgiving jail system. With its gripping storyline, excellent performances, and timeless themes, this film is a must-see for anybody who loves a well-crafted drama.
Subsequent, we suggest “The Lord of the Rings: The Fellowship of the Ring” (2001), an epic journey that mixes breathtaking visuals, memorable characters, and a richly detailed world. This film is a masterclass in storytelling, with a deep sense of historical past and tradition that can transport you to Center-earth and depart you wanting extra.
Lastly, we recommend “The Pianist” (2002), a profound and shifting documentary that tells the true story of Władysław Szpilman, a Polish Jewish pianist who struggled to outlive the destruction of the Warsaw ghetto throughout World Struggle II. This movie is a robust reminder of the human spirit’s capability for resilience and hope, even within the face of unimaginable tragedy.
We hope these suggestions resonate together with your pursuits and offer you an satisfying and enriching film expertise. Don’t miss out on these timeless classics – watch them now and uncover the magic of Basic Cinema!
Greatest regards,
The Basic Cinema Crew
We’ve carried out exams with each Llama 2 7B-Chat (see the next code pattern) and Llama 70B for comparability. Each fashions carried out nicely, yielding constant conclusions. By utilizing a immediate template crammed with up-to-date knowledge, we discovered it simpler to check arbitrary LLMs, serving to us select the correct steadiness between efficiency and price. We’ve additionally made a number of shared observations which can be price noting.
Firstly, we will see that the suggestions offered genuinely align with person preferences. The film suggestions are guided by varied elements inside our utility, most notably the person profile saved within the characteristic retailer.
Moreover, the tone of the emails corresponds to person preferences. Because of the superior language understanding capabilities of LLM, we will customise the film descriptions and electronic mail content material, tailoring them to every particular person person.
Moreover, the ultimate output format will be designed into the immediate. For instance, in our case, the salutation “Pricey [Name]” must be crammed by the e-mail service. It’s necessary to notice that though we keep away from exposing personally identifiable data (PII) inside our generative AI utility, there’s the chance to reintroduce this data throughout postprocessing, assuming the correct stage of permissions are granted.
Clear up
To keep away from pointless prices, delete the sources you created as a part of this answer, together with the characteristic retailer and LLM inference endpoint deployed with SageMaker JumpStart.
Conclusion
The facility of LLMs in producing personalised suggestions is immense and transformative, notably when coupled with the correct instruments. By integrating SageMaker Function Retailer and LangChain for immediate engineering, builders can assemble and handle extremely tailor-made person profiles. This ends in high-quality, context-aware inputs that considerably improve suggestion efficiency. In our illustrative state of affairs, we noticed how this may be utilized to tailor film suggestions to particular person person preferences, leading to a extremely personalised expertise.
Because the LLM panorama continues to evolve, we anticipate seeing extra progressive purposes that use these fashions to ship much more partaking, personalised experiences. The chances are boundless, and we’re excited to see what you’ll create with these instruments. With sources resembling SageMaker JumpStart and Amazon Bedrock now obtainable to speed up the event of generative AI purposes, we strongly suggest exploring the development of advice options utilizing LLMs on AWS.
In regards to the Authors
Yanwei Cui, PhD, is a Senior Machine Studying Specialist Options Architect at AWS. He began machine studying analysis at IRISA (Analysis Institute of Laptop Science and Random Techniques), and has a number of years of expertise constructing AI-powered industrial purposes in laptop imaginative and prescient, pure language processing, and on-line person habits prediction. At AWS, he shares his area experience and helps clients unlock enterprise potentials and drive actionable outcomes with machine studying at scale. Exterior of labor, he enjoys studying and touring.
Gordon Wang is a Senior AI/ML Specialist TAM at AWS. He helps strategic clients with AI/ML greatest practices cross many industries. He’s enthusiastic about laptop imaginative and prescient, NLP, generative AI, and MLOps. In his spare time, he loves operating and climbing.
Michelle Hong, PhD, works as Prototyping Options Architect at Amazon Internet Providers, the place she helps clients construct progressive purposes utilizing a wide range of AWS elements. She demonstrated her experience in machine studying, notably in pure language processing, to develop data-driven options that optimize enterprise processes and enhance buyer experiences.
Bin Wang, PhD, is a Senior Analytic Specialist Options Architect at AWS, boasting over 12 years of expertise within the ML business, with a specific give attention to promoting. He possesses experience in pure language processing (NLP), recommender programs, numerous ML algorithms, and ML operations. He’s deeply enthusiastic about making use of ML/DL and massive knowledge methods to resolve real-world issues. Exterior of his skilled life, he enjoys music, studying, and touring.