Unlock ML insights utilizing the Amazon SageMaker Function Retailer Function Processor
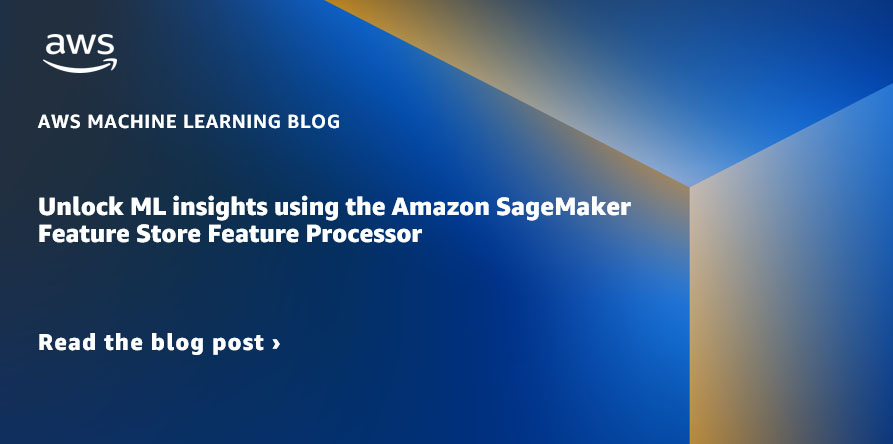
Amazon SageMaker Feature Store offers an end-to-end answer to automate function engineering for machine studying (ML). For a lot of ML use circumstances, uncooked knowledge like log recordsdata, sensor readings, or transaction data must be remodeled into significant options which might be optimized for mannequin coaching.
Function high quality is vital to make sure a extremely correct ML mannequin. Reworking uncooked knowledge into options utilizing aggregation, encoding, normalization, and different operations is commonly wanted and might require vital effort. Engineers should manually write customized knowledge preprocessing and aggregation logic in Python or Spark for every use case.
This undifferentiated heavy lifting is cumbersome, repetitive, and error-prone. The SageMaker Feature Store Feature Processor reduces this burden by robotically reworking uncooked knowledge into aggregated options appropriate for batch coaching ML fashions. It lets engineers present easy knowledge transformation features, then handles working them at scale on Spark and managing the underlying infrastructure. This permits knowledge scientists and knowledge engineers to give attention to the function engineering logic moderately than implementation particulars.
On this submit, we show how a automotive gross sales firm can use the Function Processor to remodel uncooked gross sales transaction knowledge into options in three steps:
- Native runs of information transformations.
- Distant runs at scale utilizing Spark.
- Operationalization through pipelines.
We present how SageMaker Function Retailer ingests the uncooked knowledge, runs function transformations remotely utilizing Spark, and hundreds the ensuing aggregated options right into a feature group. These engineered options are can then be used to coach ML fashions.
For this use case, we see how SageMaker Function Retailer helps convert the uncooked automotive gross sales knowledge into structured options. These options are subsequently used to realize insights like:
- Common and most worth of crimson convertibles from 2010
- Fashions with finest mileage vs. worth
- Gross sales tendencies of recent vs. used automobiles over time
- Variations in common MSRP throughout areas
We additionally see how SageMaker Function Retailer pipelines preserve the options up to date as new knowledge is available in, enabling the corporate to repeatedly achieve insights over time.
Answer overview
We work with the dataset car_data.csv
, which incorporates specs comparable to mannequin, yr, standing, mileage, worth, and MSRP for used and new automobiles offered by the corporate. The next screenshot exhibits an instance of the dataset.
The answer pocket book feature_processor.ipynb
incorporates the next principal steps, which we clarify on this submit:
- Create two function teams: one known as
car-data
for uncooked automotive gross sales data and one other known ascar-data-aggregated
for aggregated automotive gross sales data. - Use the
@feature_processor
decorator to load knowledge into the car-data function group from Amazon Simple Storage Service (Amazon S3). - Run the
@feature_processor code
remotely as a Spark utility to mixture the info. - Operationalize the function processor through SageMaker pipelines and schedule runs.
- Discover the function processing pipelines and lineage in Amazon SageMaker Studio.
- Use aggregated options to coach an ML mannequin.
Conditions
To comply with this tutorial, you want the next:
For this submit, we consult with the next notebook, which demonstrates how you can get began with Function Processor utilizing the SageMaker Python SDK.
Create function teams
To create the function teams, full the next steps:
- Create a function group definition for
car-data
as follows:
The options correspond to every column within the car_data.csv
dataset (Mannequin
, Yr
, Standing
, Mileage
, Worth
, and MSRP
).
- Add the file identifier
id
and occasion timeingest_time
to the function group:
- Create a function group definition for
car-data-aggregated
as follows:
For the aggregated function group, the options are mannequin yr standing, common mileage, max mileage, common worth, max worth, common MSRP, max MSRP, and ingest time. We add the file identifier model_year_status
and occasion time ingest_time
to this function group.
- Now, create the
car-data
function group:
- Create the
car-data-aggregated
function group:
You’ll be able to navigate to the SageMaker Function Retailer possibility beneath Knowledge on the SageMaker Studio Residence menu to see the function teams.
Use the @feature_processor decorator to load knowledge
On this part, we domestically remodel the uncooked enter knowledge (car_data.csv
) from Amazon S3 into the car-data
function group utilizing the Function Retailer Function Processor. This preliminary native run permits us to develop and iterate earlier than working remotely, and may very well be executed on a pattern of the info if desired for sooner iteration.
With the @feature_processor
decorator, your transformation perform runs in a Spark runtime surroundings the place the enter arguments supplied to your perform and its return worth are Spark DataFrames.
- Set up the Feature Processor SDK from the SageMaker Python SDK and its extras utilizing the next command:
The variety of enter parameters in your transformation perform should match the variety of inputs configured within the @feature_processor
decorator. On this case, the @feature_processor
decorator has car-data.csv
as enter and the car-data
function group as output, indicating this can be a batch operation with the target_store
as OfflineStore
:
- Outline the
remodel()
perform to remodel the info. This perform performs the next actions:- Convert column names to lowercase.
- Add the occasion time to the
ingest_time
column. - Take away punctuation and change lacking values with NA.
- Name the
remodel()
perform to retailer the info within thecar-data
function group:
The output exhibits that the info is ingested efficiently into the car-data function group.
The output of the transform_df.present()
perform is as follows:
We now have efficiently remodeled the enter knowledge and ingested it within the car-data
function group.
Run the @feature_processor code remotely
On this part, we show working the function processing code remotely as a Spark utility utilizing the @distant
decorator described earlier. We run the function processing remotely utilizing Spark to scale to massive datasets. Spark offers distributed processing on clusters to deal with knowledge that’s too large for a single machine. The @distant
decorator runs the native Python code as a single or multi-node SageMaker coaching job.
- Use the
@distant
decorator together with the@feature_processor
decorator as follows:
The spark_config
parameter signifies that is run as a Spark utility
. The SparkConfig occasion configures the Spark configuration and dependencies.
- Outline the
mixture()
perform to mixture the info utilizing PySpark SQL and user-defined features (UDFs). This perform performs the next actions:- Concatenate
mannequin
,yr
, andstanding
to createmodel_year_status
. - Take the typical of
worth
to createavg_price
. - Take the max worth of
worth
to createmax_price
. - Take the typical of
mileage
to createavg_mileage
. - Take the max worth of
mileage
to createmax_mileage
. - Take the typical of
msrp
to createavg_msrp
. - Take the max worth of
msrp
to createmax_msrp
. - Group by
model_year_status
.
- Concatenate
- Run the
mixture()
perform, which creates a SageMaker coaching job to run the Spark utility:
In consequence, SageMaker creates a coaching job to the Spark utility outlined earlier. It can create a Spark runtime surroundings utilizing the sagemaker-spark-processing picture
.
We use SageMaker Coaching jobs right here to run our Spark function processing utility. With SageMaker Coaching, you’ll be able to scale back startup occasions to 1 minute or much less through the use of heat pooling, which is unavailable in SageMaker Processing. This makes SageMaker Coaching higher optimized for brief batch jobs like function processing the place startup time is necessary.
- To view the small print, on the SageMaker console, select Coaching jobs beneath Coaching within the navigation pane, then select the job with the identify
aggregate-<timestamp>
.
The output of the mixture() perform generates telemetry code. Contained in the output, you will notice the aggregated knowledge as follows:
When the coaching job is full, it’s best to see following output:
Operationalize the function processor through SageMaker pipelines
On this part, we show how you can operationalize the function processor by selling it to a SageMaker pipeline and scheduling runs.
- First, add the transformation_code.py file containing the function processing logic to Amazon S3:
- Subsequent, create a Function Processor pipeline car_data_pipeline utilizing the .to_pipeline() perform:
- To run the pipeline, use the next code:
- Equally, you’ll be able to create a pipeline for aggregated options known as
car_data_aggregated_pipeline
and begin a run. - Schedule the
car_data_aggregated_pipeline
to run each 24 hours:
Within the output part, you will notice the ARN of pipeline and the pipeline execution function, and the schedule particulars:
- To get all of the Function Processor pipelines on this account, use the
list_pipelines()
perform on the Function Processor:
The output might be as follows:
We now have efficiently created SageMaker Function Processor pipelines.
Discover function processing pipelines and ML lineage
In SageMaker Studio, full the next steps:
- On the SageMaker Studio console, on the Residence menu, select Pipelines.
It’s best to see two pipelines created: car-data-ingestion-pipeline
and car-data-aggregated-ingestion-pipeline
.
- Select the
car-data-ingestion-pipeline
.
It exhibits the run particulars on the Executions tab.
- To view the function group populated by the pipeline, select Function Retailer beneath Knowledge and select
car-data
.
You will note the 2 function teams we created within the earlier steps.
- Select the
car-data
function group.
You will note the options particulars on the Options tab.
View pipeline runs
To view the pipeline runs, full the next steps:
- On the Pipeline Executions tab, choose
car-data-ingestion-pipeline
.
It will present all of the runs.
- Select one of many hyperlinks to see the small print of the run.
- To view lineage, select Lineage.
The total lineage for car-data
exhibits the enter knowledge supply car_data.csv
and upstream entities. The lineage for car-data-aggregated
exhibits the enter car-data
function group.
- Select Load options after which select Question upstream lineage on
car-data
andcar-data-ingestion-pipeline
to see all of the upstream entities.
The total lineage for car-data
function group ought to appear to be the next screenshot.
Equally, the lineage for the car-aggregated-data
function group ought to appear to be the next screenshot.
SageMaker Studio offers a single surroundings to trace scheduled pipelines, view runs, discover lineage, and look at the function processing code.
The aggregated options comparable to common worth, max worth, common mileage, and extra within the car-data-aggregated
function group present perception into the character of the info. You can too use these options as a dataset to coach a mannequin to foretell automotive costs, or for different operations. Nevertheless, coaching the mannequin is out of scope for this submit, which focuses on demonstrating the SageMaker Function Retailer capabilities for function engineering.
Clear up
Don’t overlook to scrub up the assets created as a part of this submit to keep away from incurring ongoing costs.
- Disable the scheduled pipeline through the
fp.schedule()
technique with the state parameter asDisabled
:
- Delete each function teams:
The info residing within the S3 bucket and offline function retailer can incur prices, so it’s best to delete them to keep away from any costs.
- Delete the S3 objects.
- Delete the records from the function retailer.
Conclusion
On this submit, we demonstrated how a automotive gross sales firm used SageMaker Function Retailer Function Processor to realize invaluable insights from their uncooked gross sales knowledge by:
- Ingesting and remodeling batch knowledge at scale utilizing Spark
- Operationalizing function engineering workflows through SageMaker pipelines
- Offering lineage monitoring and a single surroundings to observe pipelines and discover options
- Getting ready aggregated options optimized for coaching ML fashions
By following these steps, the corporate was in a position to remodel beforehand unusable knowledge into structured options that might then be used to coach a mannequin to foretell automotive costs. SageMaker Function Retailer enabled them to give attention to function engineering moderately than the underlying infrastructure.
We hope this submit helps you unlock invaluable ML insights from your personal knowledge utilizing SageMaker Function Retailer Function Processor!
For extra data on this, consult with Feature Processing and the SageMaker instance on Amazon SageMaker Feature Store: Feature Processor Introduction.
In regards to the Authors
Dhaval Shah is a Senior Options Architect at AWS, specializing in Machine Studying. With a powerful give attention to digital native companies, he empowers prospects to leverage AWS and drive their enterprise progress. As an ML fanatic, Dhaval is pushed by his ardour for creating impactful options that deliver constructive change. In his leisure time, he indulges in his love for journey and cherishes high quality moments along with his household.
Ninad Joshi is a Senior Options Architect at AWS, serving to world AWS prospects design safe, scalable, and value efficient options in cloud to resolve their complicated real-world enterprise challenges. His work in Machine Studying (ML) covers a variety of AI/ML use circumstances, with a main give attention to Finish-to-Finish ML, Pure Language Processing, and Laptop Imaginative and prescient. Previous to becoming a member of AWS, Ninad labored as a software program developer for 12+ years. Exterior of his skilled endeavors, Ninad enjoys enjoying chess and exploring totally different gambits.