Fast and Soiled Strategy to Match Regression Fashions Utilizing (Solely) SQL.
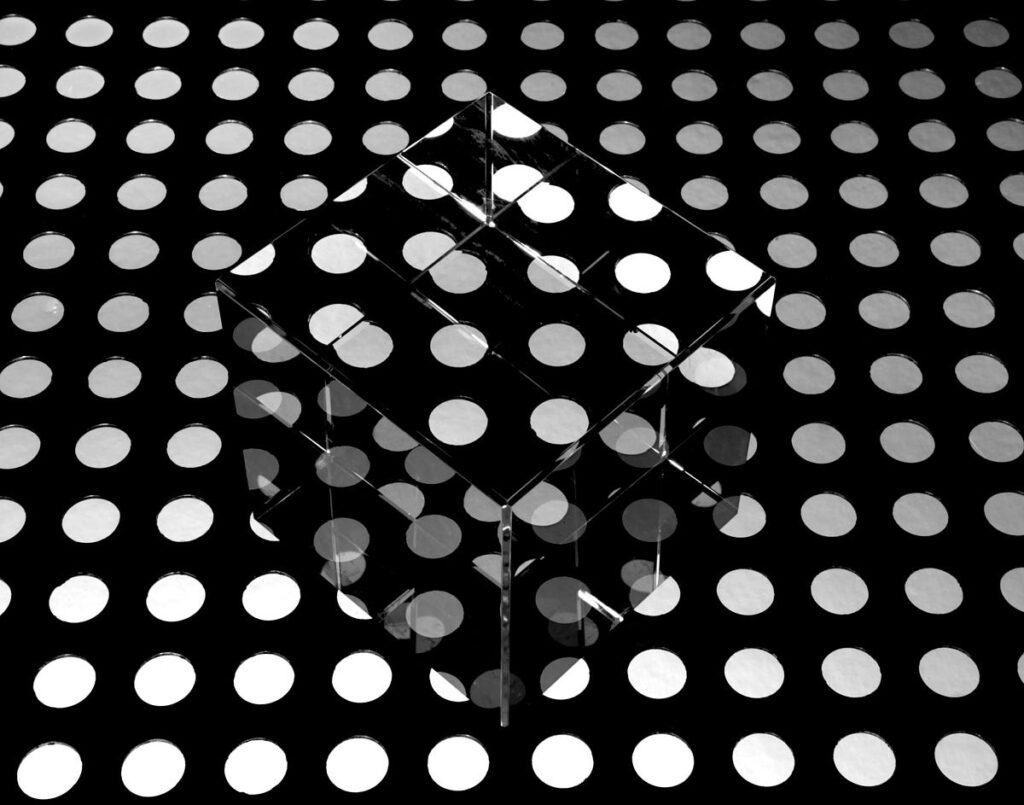
You don’t all the time want Python or R to suit fashions — Postgresql has coated the fundamentals
SQL programmers hardly match any ML fashions.
Another person will do it until they’ve both Python or R information. Whereas Python and scikit-learn are sometimes my go-to instruments for machine studying, it’s price noting that SQL may do some fast and soiled mannequin becoming.
Regression fashions are a typical one nearly everybody wants. I bear in mind utilizing it in highschool physics for the primary time.
In such conditions, you probably have your information in a Postgres desk, you don’t have to go away your SQL surroundings to suit such trivial fashions.
Right here’s how we do that.
Regression modeling in SQL
Postgres has built-in utilities to work with regression fashions. You don’t have to put in or activate any particular modules.
We are able to simply match linear regression fashions shortly and make predictions utilizing them.
A linear regression mannequin is about discovering the equation of a line that generalizes the dataset. Thus, we solely want to search out the road’s intercept and slope.
The regr_slope
and regr_intercept
capabilities assist us with this process.
Let’s suppose we now have a desk with the rainfall and temperature columns. And we have to predict the lacking values within the temperature column utilizing the rainfall data.
Here is a SELECT assertion that retrieves the rainfall and temperature values from the climate desk, the place lacking temperature values are stuffed with predictions utilizing the regr_slope
and regr_intercept
capabilities:
SELECT
rainfall,
CASE
WHEN temperature IS NULL
THEN regr_slope(temperature, rainfall) * rainfall
+ regr_intercept(temperature, rainfall)
ELSE temperature
END AS temperature
FROM
climate;
We use a CASE
assertion to verify if the temperature worth is lacking (i.e., NULL). Whether it is lacking, we use the regr_slope
and regr_intercept
Features to foretell the temperature worth primarily based on the corresponding rainfall worth. In any other case, we use the unique temperature worth.
Persisting the predictions
If I wish to fill within the lacking values within the desk completely, I can use a barely modified model of the above code. You may create a materialized view for the predictions or insert the forecasts into a distinct desk.
-- Populate the desk with predicted temperature values
INSERT INTO predicted_temperature (rainfall, temperature)
SELECT
t1.rainfall,
CASE
WHEN t1.temperature IS NULL
THEN regr_slope(t2.temperature, t2.rainfall) * t1.rainfall
+ regr_intercept(t2.temperature, t2.rainfall)
ELSE t1.temperature
END AS temperature
FROM
climate t1
LEFT JOIN climate t2 ON t1.rainfall = t2.rainfall
WHERE
t1.temperature IS NULL;
And right here’s the identical however making a materialized view. Materialized views are merely queries saved within the database together with their outcomes from the most recent run.
CREATE MATERIALIZED VIEW predicted_temperature_mv AS
SELECT
rainfall,
CASE
WHEN temperature IS NULL
THEN regr_slope(temperature, rainfall) * rainfall
+ regr_intercept(temperature, rainfall)
ELSE temperature
END AS temperature
FROM
climate;
Limitations of becoming regression fashions in SQL
Anybody who labored on regression fashions earlier than might attest that the above examples nonetheless must be accomplished. Becoming an accurate mannequin is extra complicated than what we’ve mentioned right here.
Particularly in real-life conditions, we must always contemplate a couple of impartial variable to foretell the dependent one. Typically we now have to make use of polynomial order in becoming the regression fashions slightly than the linear one. We may want to make use of them each.
Postgres’s regression utilities aren’t able to dealing with such complicated modeling. We are able to solely construct a linea regressor with one impartial variable.
Conclusion
Linear regression fashions are most likely essentially the most used ones for predicting steady information. Knowledge scientists usually use it as a place to begin for extra complicated ML modeling.
Though we want the assist of programming languages equivalent to Python for extra subtle machine-learning duties, easy duties like linear regressions will be executed inside SQL itself.
I hope this little method mentioned on this publish will aid you in your each day work.